Introduction to Data Structures, unlocking the power of data structures essential foundations for efficient algorithms and problem solving
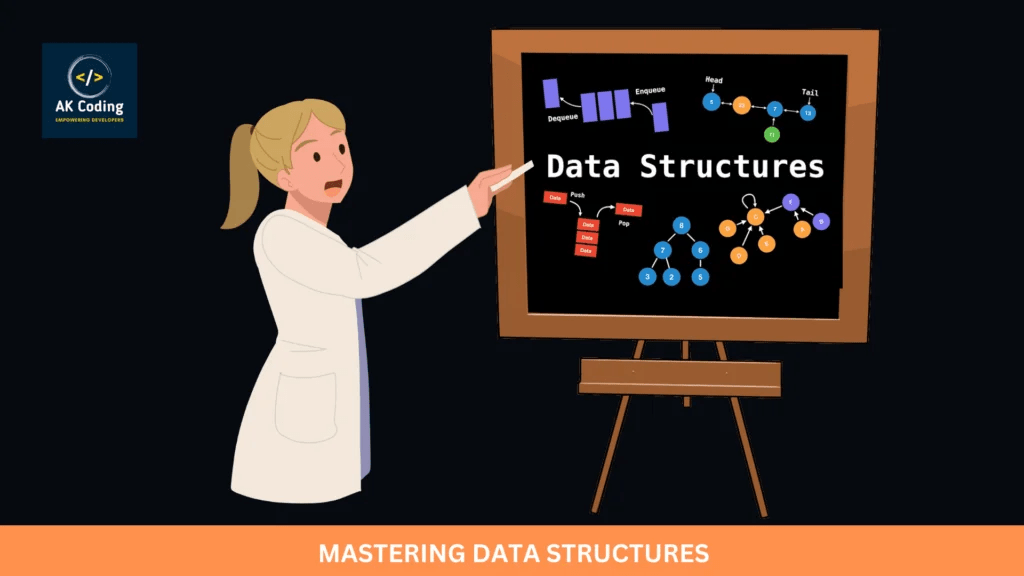
Table of Contents
1. Introduction to Data Structures
Data structures are fundamental components of computer science that organize and store data in a way that enables efficient access, manipulation, and retrieval. They provide a framework for representing and managing information within computer programs, facilitating tasks such as searching, sorting, and processing data. In essence, data structures serve as the building blocks upon which algorithms operate, playing a critical role in problem-solving and software development.
Basic Concepts of Data Structures
- Elements: Data structures consist of individual elements or data items, which can be of various types, such as integers, characters, strings, or custom-defined objects.
- Operations: Data structures support a set of operations or actions that can be performed on the stored data, including insertion, deletion, traversal, search, and sorting. The choice of operations depends on the specific type and requirements of the data structure.
- Memory Representation: Data structures are implemented using memory storage mechanisms, which determine how data is organized and accessed within a computer’s memory. Common memory representations include arrays, linked lists, trees, and hash tables.
- Abstraction: Data structures provide an abstraction layer that hides the underlying implementation details, allowing developers to focus on the logical structure and behavior of the data rather than the specific memory layout or storage mechanism.
- Efficiency: The efficiency of data structures is measured based on factors such as time complexity (the amount of time required to perform operations) and space complexity (the amount of memory required to store data). Well-designed data structures optimize these factors to achieve fast and resource-efficient operations.
- Complexity Analysis: Analyzing the time and space complexity of data structures and algorithms is essential for evaluating their performance characteristics and making informed design decisions. Techniques like Big O notation are commonly used to quantify the scalability and efficiency of data structures.
In summary, data structures are essential components of computer science that provide a means of organizing and managing data effectively. Understanding the basic concepts of data structures is crucial for developing efficient algorithms, optimizing program performance, and solving a wide range of computational problems.
2. Why Study Data Structures?
Role in Computer Science and Software Engineering
Data structures play a pivotal role in computer science and software engineering for several reasons:
- Efficient Data Management: Data structures provide organized ways to store, retrieve, and manipulate data efficiently. They enable programmers to manage complex data sets and perform operations on them with optimal time and space complexity.
- Foundation for Algorithms: Data structures serve as the foundation upon which algorithms are built. Many algorithms rely on specific data structures to achieve their intended functionality. Understanding data structures is therefore essential for understanding and implementing algorithms effectively.
- Software Design and Architecture: Data structures influence the design and architecture of software systems. Choosing the right data structures can significantly impact the performance, scalability, and maintainability of software applications.
- Abstraction and Modularity: Data structures allow programmers to abstract away the details of data organization and access, providing modular components that can be reused across different parts of a program or even in different projects.
Importance in Problem Solving and Algorithm Design:
The study of data structures is crucial for problem-solving and algorithm design due to the following reasons:
- Algorithm Efficiency: The choice of data structure can greatly influence the efficiency of algorithms. Different data structures have different time and space complexity characteristics, which can significantly impact the performance of algorithms.
- Problem Classification: Many computational problems can be classified based on the types of data structures and algorithms required to solve them. Understanding data structures helps in recognizing problem patterns and selecting appropriate algorithmic approaches.
- Optimization Opportunities: Knowledge of data structures enables programmers to identify optimization opportunities in algorithmic solutions. By choosing the right data structures and algorithmic techniques, it is often possible to improve the efficiency and scalability of solutions to computational problems.
- Algorithmic Paradigms: Different data structures lend themselves to different algorithmic paradigms, such as divide and conquer, dynamic programming, or greedy algorithms. Understanding data structures provides insight into which paradigm is most suitable for a given problem.
In summary, studying data structures is essential for computer scientists and software engineers as it provides the foundation for efficient data management, algorithm design, and problem-solving. Mastery of data structures enables programmers to develop optimized, scalable, and maintainable software solutions for a wide range of computational problems.
3. Classification of Data Structures
Data structures can be classified based on various criteria, including their organization, accessibility, and relationship between elements. Here’s a breakdown of the classification of data structures:
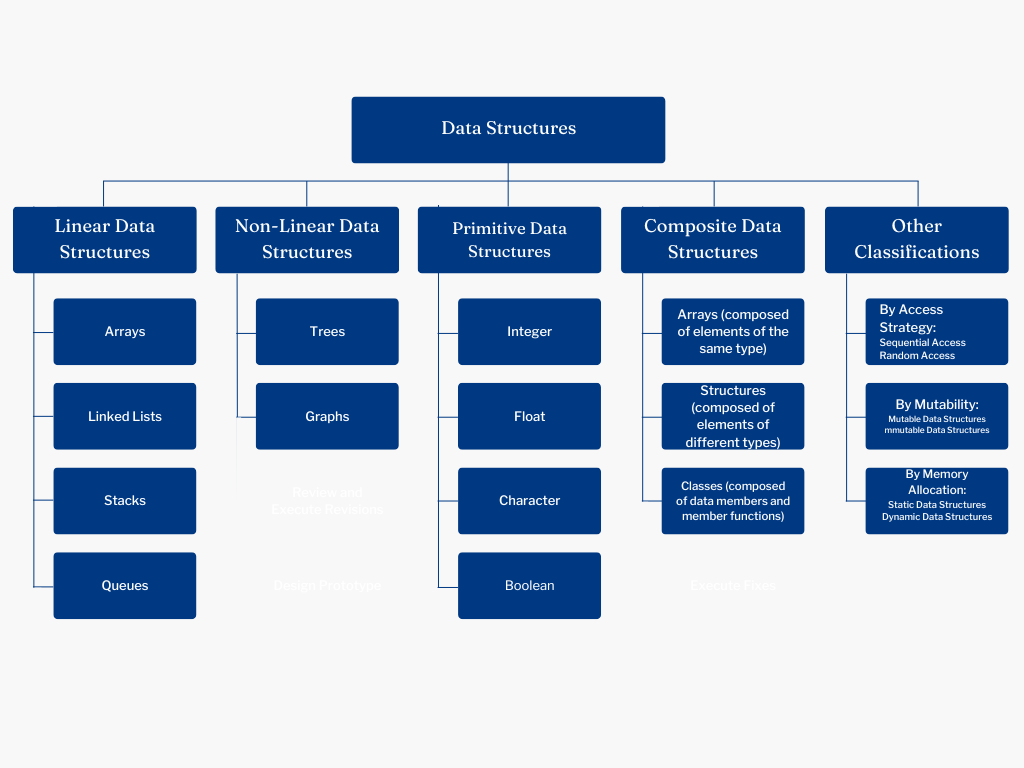
1. Linear vs Non-Linear Data Structures
Linear Data Structures:
Elements are arranged in a sequential manner, with each element having a unique predecessor and successor.
Examples:
- Arrays
- Linked Lists
- Stacks
- Queues
Non-Linear Data Structures
Elements are not arranged in a sequential manner and may have multiple predecessors and successors.
Examples:
- Trees
- Graphs
Comparisons of Linear Data Structures and Non-Linear Data Structures
Below is a comparison of linear and non-linear data structures
Aspect | Linear Data Structures | Non-Linear Data Structures |
---|---|---|
Organization | Elements arranged sequentially | Elements arranged in complex relationships |
Access Pattern | Sequential access | Requires specialized traversal algorithms |
Examples | Arrays, Linked Lists, Stacks, Queues | Trees, Graphs |
Properties | Simple organization, easy traversal | Complex organization, specialized traversal methods |
Applications | Ordered collections, sequential processing | Hierarchical data representation, network analysis |
Usage | Simple data storage, basic algorithms | Complex data relationships, advanced algorithms |
Traversal | Linear traversal (forward or backward) | Tree traversal (pre-order, in-order, post-order), Graph traversal (depth-first, breadth-first) |
Efficiency | Generally efficient for linear operations | Efficiency varies based on the complexity of relationships and traversal requirements |
In summary, linear data structures are characterized by sequential organization and simple access patterns, making them suitable for basic data storage and processing tasks. Non-linear data structures, on the other hand, represent complex relationships between elements and require specialized traversal algorithms for analysis and manipulation. Both types of data structures have unique properties and are used in various applications based on the nature of the data and the requirements of the problem at hand.
2. Primitive vs Composite Data Structures
Primitive Data Structures:
Fundamental data types provided by the programming language.
Examples:
- Integer
- Float
- Character
- Boolean
Composite Data Structures:
- Combinations of primitive data types and other composite data structures.
- Examples:
- Arrays (composed of elements of the same type)
- Structures (composed of elements of different types)
- Classes (composed of data members and member functions)
3. Other Classifications of Data Structures
By Access Strategy:
- Sequential Access: Elements accessed one after the other in a predetermined order (e.g., arrays).
- Random Access: Elements accessed directly by their position or index (e.g., arrays, hash tables).
By Mutability:
- Mutable Data Structures: Allow modifications to the structure and content of the data (e.g., arrays, linked lists).
- Immutable Data Structures: Once created, cannot be modified (e.g., strings in some programming languages).
By Memory Allocation:
- Static Data Structures: Memory allocation fixed at compile time (e.g., arrays).
- Dynamic Data Structures: Memory allocation can change dynamically during runtime (e.g., linked lists, trees).
By Purpose:
- Linear Data Structures: Optimized for storing and accessing data sequentially.
- Non-Linear Data Structures: Optimized for representing hierarchical relationships or arbitrary connections between data elements.
By Complexity:
- Simple Data Structures: Basic building blocks used to construct more complex data structures (e.g., arrays, linked lists).
- Complex Data Structures: Higher-level structures built upon simpler ones, providing advanced functionalities and optimizations (e.g., trees, hash maps).
Understanding the classification of data structures is essential for selecting the appropriate structure for solving specific problems and optimizing algorithmic solutions. Each type of data structure has its own advantages, disadvantages, and optimal use cases, making it important to choose wisely based on the requirements of the problem at hand.
4. Key Terminology
Key Terminology in Data Structures
1. Elements:
- Elements refer to the individual data items or entities stored within a data structure.
- Example: In an array, each element represents a distinct value.
2. Operations:
- Operations are the actions that can be performed on a data structure to manipulate its contents.
- Common operations include insertion, deletion, searching, traversal, and sorting.
- Example: In a stack, push and pop are the primary operations.
3. Memory Representation:
- Memory representation refers to how data is organized and stored within the computer’s memory.
- Different data structures use different memory representations to store and access elements efficiently.
- Example: Arrays use contiguous memory allocation, while linked lists use dynamic memory allocation for individual nodes.
Elements in Data Structures
1. Definition:
- Elements are the individual data items or entities stored within a data structure.
- Each element can be of a specific data type, such as integer, character, string, or user-defined object.
2. Properties:
- Elements may have associated properties or attributes that define their characteristics or behavior.
- Example: In a student record data structure, elements may include properties such as name, age, gender, and grade.
3. Access:
- Elements can be accessed and manipulated using various operations provided by the data structure.
- Accessing elements typically involves specifying an index, key, or position within the data structure.
Operations on Data Structures
1. Insertion:
- Insertion involves adding a new element to the data structure.
- Depending on the type of data structure, insertion may occur at the beginning, end, or middle of the structure.
2. Deletion:
- Deletion involves removing an existing element from the data structure.
- Deletion may require reorganizing the structure to maintain its integrity and efficiency.
3. Searching:
- Searching involves finding a specific element within the data structure.
- Different search algorithms may be used based on the organization and properties of the data structure.
4. Traversal:
- Traversal involves visiting each element in the data structure in a systematic manner.
- Traversal is often used to perform operations on each element or to collect information from the structure.
5. Sorting:
- Sorting involves arranging the elements of the data structure in a specific order.
- Sorting algorithms can be applied to various data structures to order elements based on specific criteria.
Memory Representation of Data Structures
1. Contiguous Memory Allocation:
- Elements are stored in adjacent memory locations, allowing for direct access and efficient traversal.
- Examples: Arrays, matrices.
2. Dynamic Memory Allocation:
- Elements are allocated memory dynamically as needed, using pointers or references to link them together.
- Examples: Linked lists, trees.
3. Indexed Access:
- Elements are accessed using an index or position within the structure.
- Indexed access allows for efficient random access to elements.
- Examples: Arrays, hash tables.
3. Pointers or References:
- Pointers or references are used to establish relationships between elements within the structure.
- Pointers enable dynamic linking and traversal of data elements.
- Examples: Linked lists, trees, graphs.
Understanding these key terminology and concepts is essential for designing, implementing, and using data structures effectively in software development and problem-solving. Each concept provides insights into how data is organized, accessed, and manipulated within different types of data structures.
5. Real-world Applications of Data Structures
Data structures find applications in various domains and everyday scenarios, contributing to technological advancements and innovation. Here are examples of data structures in everyday life and their impact on technology:
1. Social Media Networks:
- Data structures like graphs are used to represent connections between users in social media networks.
- Algorithms based on graph theory enable recommendations, friend suggestions, and content personalization.
- Impact: Enhances user engagement, fosters connections, and drives content discovery.
2. Online Shopping Platforms:
- Data structures such as hash tables and trees are employed to organize and index product information.
- Efficient search and retrieval algorithms enable users to find products quickly based on various criteria.
- Impact: Streamlines the shopping experience, supports personalized recommendations, and facilitates inventory management.
3. Navigation Systems:
- Graph data structures are utilized to represent road networks, with nodes representing intersections and edges representing roads.
- Pathfinding algorithms like Dijkstra’s algorithm and A* search are applied to find optimal routes between locations.
- Impact: Enables real-time navigation, minimizes travel time, and optimizes resource utilization.
4. Healthcare Systems:
- Data structures such as trees and graphs are used to organize patient records, medical histories, and treatment plans.
- Algorithms support decision-making processes, patient monitoring, and analysis of medical data.
- Impact: Improves patient care, facilitates medical research, and enhances diagnostic accuracy.
5. Search Engines:
- Hash tables and inverted index data structures are employed to index and store web pages and documents.
- Ranking algorithms like PageRank use graph structures to assess the relevance and authority of web pages.
- Impact: Facilitates information retrieval, supports content discovery, and enhances user experience.
6. Financial Systems:
- Data structures like trees and priority queues are used to manage financial transactions, customer accounts, and investment portfolios.
- Algorithms support risk assessment, fraud detection, and algorithmic trading strategies.
- Impact: Enhances financial security, enables real-time transaction processing, and optimizes investment decisions.
7. Smartphones and Wearable Devices:
- Data structures such as arrays, linked lists, and stacks are employed to manage sensor data, user inputs, and application state.
- Efficient data structures and algorithms prolong battery life, optimize memory usage, and ensure responsive user interfaces.
- Impact: Enables seamless integration of hardware and software, supports advanced features like gesture recognition and health tracking.
8. E-commerce Recommendation Systems:
- Data structures like collaborative filtering matrices and recommendation graphs are used to analyze user behavior and preferences.
- Machine learning algorithms leverage these structures to generate personalized product recommendations.
- Impact: Drives sales, enhances customer satisfaction, and fosters customer loyalty.
In summary, data structures play a crucial role in diverse real-world applications, powering technological innovations and driving advancements across various industries. Their efficient organization and manipulation of data enable the development of sophisticated systems and solutions that improve efficiency, enhance user experiences, and drive business success.
6. Challenges and Considerations in Data Structures
Efficiency vs. Simplicity, and Trade-offs between Time and Space Complexity are key challenges and considerations in data structure design. Here’s a closer look:
1. Efficiency vs. Simplicity:
- Efficiency: Data structures need to be optimized for efficient operations, minimizing time and space complexity. This involves selecting the right structure and algorithm for the problem at hand, considering factors like access patterns, expected data size, and performance requirements.
- Simplicity: Simplicity in data structures ensures ease of understanding, implementation, and maintenance. While complex structures may offer higher efficiency, they can also introduce greater complexity in code, making it harder to debug, extend, and modify.
2. Trade-offs between Time and Space Complexity:
- Time Complexity: Refers to the computational time required to perform operations on the data structure. Algorithms with lower time complexity execute faster but may require more memory or storage.
- Space Complexity: Refers to the amount of memory or storage space required by the data structure. Structures with lower space complexity consume less memory but may execute slower due to more computationally intensive operations.
- Trade-offs: Data structures often involve trade-offs between time and space complexity. For example, a hash table offers constant-time average-case lookup but may consume more memory due to collision resolution. On the other hand, a binary search tree offers logarithmic-time lookup but may require less memory compared to a hash table.
Considerations:
- Problem Requirements: Understanding the specific requirements of the problem is crucial for selecting the appropriate data structure. Consider factors like data size, frequency of operations, expected usage patterns, and performance constraints.
- Scalability: Data structures should be scalable to handle growing data sets without significant degradation in performance. Structures that offer efficient amortized time complexity over large datasets are preferred.
- Resource Constraints: Consider the available hardware resources, such as memory, CPU, and storage capacity, when designing data structures. Optimize structures to minimize resource consumption while meeting performance goals.
- Flexibility: Choose data structures that offer flexibility to adapt to changing requirements and accommodate future enhancements or modifications. Structures should support efficient insertion, deletion, and updates without compromising performance or stability.
In summary, addressing the challenges of efficiency vs. simplicity and trade-offs between time and space complexity requires careful consideration of problem requirements, scalability, resource constraints, and flexibility. By striking the right balance between these factors, developers can design data structures that offer optimal performance, reliability, and maintainability for a wide range of applications and scenarios.
Conclusion
In conclusion, understanding data structures is essential for anyone venturing into the world of programming and computer science. We’ve explored the fundamental concepts, importance, and applications of data structures in this introduction.
By organizing and structuring data effectively, data structures enable us to manage information efficiently, optimize memory usage, and streamline problem-solving processes. Whether it’s storing data in arrays, organizing it in linked lists, or managing hierarchical relationships in trees, data structures provide the foundational building blocks for developing robust and scalable software solutions.
Moreover, mastering data structures empowers us to tackle complex computational problems, design efficient algorithms, and build innovative applications across various domains. Whether you’re a beginner or an experienced programmer, delving deeper into data structures opens up a world of possibilities for creating impactful and transformative technologies.
As we embark on this journey into the realm of data structures, let’s embrace curiosity, persistence, and creativity. With each concept we explore and every problem we solve, we deepen our understanding of how data structures shape the way we interact with information and navigate the digital landscape. Together, let’s continue to harness the power of data structures to drive innovation, solve challenges, and shape the future of technology.
FAQs
What are some tips for mastering data structures?
Practice regularly by implementing different data structures in code.
Experiment with different use cases and scenarios to understand when to use each data structure.
Study algorithms and data structure concepts in-depth to gain a deeper understanding of how they work.
Why is it important to understand data structures as a programmer?
Understanding data structures is essential for becoming a proficient programmer because they are fundamental building blocks used in almost every software application. Proficiency in data structures enables programmers to write more efficient, scalable, and maintainable code.
How can I learn more about data structures?
There are many resources available for learning about data structures, including textbooks, online courses, tutorials, and coding exercises. Start by understanding the basic concepts and then practice implementing different data structures in code.
What are the trade-offs between different data structures?
The choice of data structure depends on the specific requirements of the application. Some data structures may offer faster access times but require more memory, while others may be more memory-efficient but have slower access times.
Read other awesome articles in Medium.com