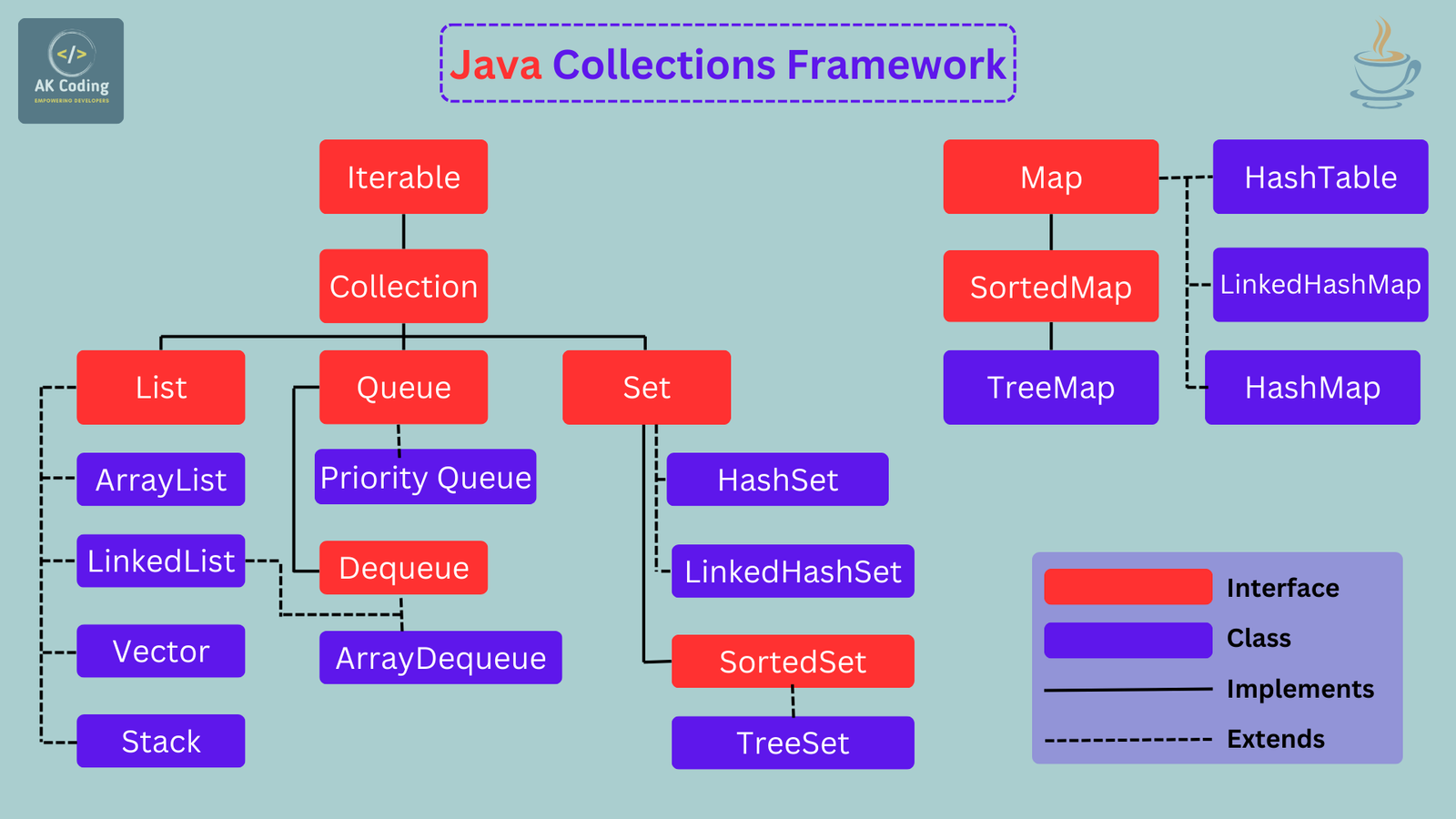
Table of Contents
Java Collections Framework
Introduction
Java Collections Framework provides a unified architecture for representing and manipulating collections of objects. It offers a set of classes and interfaces that serve as building blocks for creating, managing, and manipulating collections of objects in Java applications. These collections can be used to store, retrieve, and manipulate groups of objects efficiently.
What is the Collections Framework?
The Collections Framework in Java is a comprehensive set of classes and interfaces that provide reusable data structures to store and manipulate groups of objects. It consists of interfaces, implementations, and algorithms that enable developers to work with collections of objects in a consistent and efficient manner. Collections in Java can store elements of different types, including primitive types, and can dynamically grow and shrink as needed.
Purpose and Benefits
The primary purpose of the Java Collections Framework is to provide a standard way to work with collections of objects in Java applications. Some key benefits include:
- Uniform Interface: The framework offers a unified set of interfaces for working with different types of collections, making it easier to write code that is independent of specific implementations.
- Efficiency: The collections provided by the framework are designed for efficiency in terms of performance and memory usage. They offer various implementations optimized for different use cases.
- Type Safety: Java Collections Framework ensures type safety by using generics, allowing developers to specify the types of elements stored in a collection at compile-time.
- Flexibility: The framework provides a wide range of collection classes and interfaces, enabling developers to choose the most suitable data structure for their specific requirements.
- Interoperability: Collections Framework seamlessly integrates with other parts of the Java platform, such as the Java Stream API, making it easier to work with collections in conjunction with other Java features.
Historical Background
The Java Collections Framework was introduced as part of the Java 2 Platform, Standard Edition (J2SE) in Java 1.2. It was developed to address the limitations of the earlier collection classes provided by the Java platform, such as arrays, vectors, and hashtables, which lacked a unified interface and were not as flexible or efficient. The introduction of the Collections Framework marked a significant milestone in Java’s evolution, providing a modern, standardized approach to working with collections of objects. Since its inception, the framework has undergone several enhancements and updates, including the addition of new collection types and performance improvements, to meet the evolving needs of Java developers. Today, the Collections Framework is an integral part of the Java platform and is widely used in a variety of Java applications ranging from desktop to enterprise-level systems.
Core Interface
- Collection Interface
1. Methods(add, addAll, clear etc.)
2. Subinterfaces (List, Set, Queue) - List Interface
1. Methods(get, indexOf, lastIndexOf)
2. Implementations (ArrayList, LinkedList, etc.) - Set Interface
1. Methods(add, clear, contains)
2. Implementations (HashSet, TreeSet, etc.) - Queue Interface
1. Methods(peek,poll,offer etc.)
2. Implementations (PriorityQueue, ArrayDeque, etc.) - Map Interface
1. Methods(get, put, putAll, keySet, etc.)
2. Implementations (HashMap, TreeMap, etc.)
Common Utility Classes
- Collections Class
- Utility methods for collections
- Arrays Class
- Utility methods for arrays
Collection Implementations
- ArrayList
- LinkedList
- HashSet
- TreeSet
- HashMap
- TreeMap
- PriorityQueue
- ArrayDeque
- LinkedHashMap
- WeakHashMap
- IdentityHashMap
- EnumMap
- ConcurrentHashMap
Iterators
- Iterator Interface
- ListIterator Interface
Ordering and Sorting
- Comparable Interface
- Comparator Interface
- Sorting collections
Concurrent Collections
- ConcurrentHashMap
- CopyOnWriteArrayList
- CopyOnWriteArraySet
Specialized Collections
- EnumSet
- EnumMap
- BitSet
Performance Considerations
Time Complexities
- ArrayList:
- Access (get): O(1)
- Insertion (add): O(n)
- Deletion (remove): O(n)
- LinkedList:
- Access (get): O(n)
- Insertion (add): O(1)
- Deletion (remove): O(1)
- HashSet:
- Add, Contains, Remove: O(1) average case, O(n) worst case
- TreeSet:
- Add, Contains, Remove: O(log n) time complexity
- HashMap:
- Add, Contains, Remove: O(1) average case, O(n) worst case for individual operations
- Iteration: O(n)
- TreeMap:
- Add, Contains, Remove: O(log n) time complexity for individual operations
- Iteration: O(n)
- PriorityQueue:
- Insertion (add): O(log n)
- Removal (poll): O(log n)
- Peek: O(1)
- ArrayDeque:
- Add, Remove: O(1) amortized time complexity
- LinkedHashMap:
- Add, Remove, Get: O(1)
- Iteration: O(n)
- ConcurrentHashMap:
- Most operations are O(1) under normal operating conditions
- Some operations may be O(log n) in rare conditions
Space Complexities
- ArrayList:
- O(n) space complexity
- LinkedList:
- O(n) space complexity
- HashSet:
- O(n) space complexity
- TreeSet:
- O(n) space complexity
- HashMap:
- O(n) space complexity
- TreeMap:
- O(n) space complexity
- PriorityQueue:
- O(n) space complexity
- ArrayDeque:
- O(n) space complexity
- LinkedHashMap:
- O(n) space complexity
- ConcurrentHashMap:
- The space complexity varies depending on the number of elements and concurrency level. It generally occupies more space than a regular HashMap due to the additional overhead for handling concurrency.
These time and space complexities provide insights into the performance characteristics of different collections in the Java Collections Framework, helping developers choose the most appropriate data structure based on their specific requirements and usage scenarios.
Best Practices
- Use the Appropriate Collection Type:
- Choose the collection type that best fits your requirements (e.g., List, Set, Map) based on factors such as access patterns, duplicates, and ordering.
- Prefer Interfaces Over Concrete Implementations:
- Program to interfaces (e.g., List, Set, Map) rather than concrete implementations (e.g., ArrayList, HashSet, HashMap) to allow for flexibility and easier switching between implementations.
- Specify the Initial Capacity and Load Factor:
- When creating collections that are expected to hold a large number of elements, consider specifying an initial capacity and load factor for better performance and memory usage.
- Use Generics for Type Safety:
- Utilize generics to specify the types of elements stored in a collection, ensuring type safety and avoiding runtime errors.
- Be Mindful of Thread Safety:
- Choose thread-safe collection implementations (e.g., ConcurrentHashMap, CopyOnWriteArrayList) when working with concurrent environments to prevent data corruption and race conditions.
- Handle Concurrent Modifications Safely:
- When iterating over collections, use iterators or enhanced for-loops to avoid concurrent modification exceptions. If modifications are required during iteration, use Iterator’s methods for safe modification.
- Optimize Iteration Performance:
- Prefer enhanced for-loops or iterators over manual index-based iteration for better readability and performance, especially for collections like LinkedList.
- Use Immutable Collections for Unmodifiable Data:
- Utilize immutable collection implementations (e.g., Collections.unmodifiableList) when dealing with read-only or unmodifiable data to prevent accidental modifications.
- Avoid Unnecessary Conversions:
- Minimize conversions between different collection types to avoid unnecessary overhead and potential loss of performance.
- Be Aware of Performance Characteristics:
- Understand the time and space complexities of different collection operations to make informed decisions about choosing the most suitable collection type for specific use cases.
- Consider Memory Footprint:
- Be mindful of the memory footprint of collections, especially when dealing with large datasets, and choose implementations that offer efficient memory usage.
- Handle Null Values Appropriately:
- Ensure consistent handling of null values based on the requirements of your application to prevent unexpected behavior and NullPointerExceptions.
- Use Comparator for Custom Sorting:
- When sorting collections of custom objects, implement the Comparable interface for natural ordering or provide a custom Comparator for customized sorting logic.
- Document Usage Assumptions:
- Document assumptions and constraints about the usage of collections, especially in shared codebases, to facilitate better understanding and collaboration among developers.
By adhering to these best practices, developers can effectively leverage the Java Collections Framework to build efficient, robust, and maintainable Java applications.
Examples and Use Cases
Demonstrations of Common Tasks Using Collections
- Storing and Retrieving Data:
- Creating a list of strings and adding, removing, or accessing elements using methods like
add
,remove
, andget
. - Example: Maintaining a list of user names in a social media application.
- Iterating Over Collections:
- Using enhanced for-loops or iterators to iterate over elements in a collection.
- Example: Displaying the contents of a list of products in an e-commerce application.
- Searching and Filtering:
- Performing searches or filters based on specific criteria using methods like
contains
or custom predicates. - Example: Filtering a list of email addresses to find those belonging to a specific domain.
- Sorting Collections:
- Sorting elements in a collection either using natural ordering (Comparable) or a custom comparator.
- Example: Sorting a list of students based on their grades in descending order.
- Mapping Keys to Values:
- Storing key-value pairs in a map and performing operations like adding, removing, or retrieving values based on keys.
- Example: Maintaining a map of employee IDs to their corresponding names in a company directory.
- Counting Occurrences:
- Counting the occurrences of elements in a collection using techniques like frequency counting.
- Example: Counting the number of times each word appears in a text document.
- Grouping Elements:
- Grouping elements of a collection based on specific criteria using techniques like partitioning or grouping by.
- Example: Grouping a list of students based on their grades into different categories (e.g., A, B, C).
- Performing Set Operations:
- Performing set operations like union, intersection, or difference on sets.
- Example: Finding the common interests between two sets of users in a social networking platform.
Real-world Scenarios Where Collections are Employed
- Data Processing Pipelines:
- Collections are often used to represent intermediate or final data states in data processing pipelines, such as in ETL (Extract, Transform, Load) processes.
- Caching and Memoization:
- Collections are employed in caching systems to store frequently accessed data for quick retrieval, reducing the need for expensive computations or database queries.
- Session Management:
- Collections are used to manage user sessions in web applications, storing session attributes and managing their lifecycle.
- Resource Allocation:
- Collections are utilized in resource allocation algorithms, such as scheduling processes in an operating system or allocating tasks in distributed computing systems.
- Inventory Management:
- Collections are employed in inventory management systems to track and manage stock levels, orders, and product information.
- Graph and Network Analysis:
- Collections are used to represent graphs and networks in graph theory and network analysis applications, storing vertices, edges, and their associated attributes.
- Event Handling and Pub/Sub Systems:
- Collections are utilized in event handling systems and publish-subscribe (pub/sub) architectures to manage subscribers, events, and their relationships.
- Algorithmic Problem Solving:
- Collections are used extensively in algorithmic problem-solving tasks, such as implementing data structures like stacks, queues, and priority queues.
- Machine Learning and Data Mining:
- Collections are employed in machine learning and data mining applications for storing training data, feature vectors, and model outputs.
By understanding these examples and use cases, developers can effectively leverage the Java Collections Framework to solve a wide range of problems in various domains, from simple data management tasks to complex algorithmic challenges.
Comparison with Legacy Collection Classes
- Synchronization:
- Legacy Classes (e.g., Vector, Hashtable): Legacy collection classes such as Vector and Hashtable are synchronized by default, meaning they are thread-safe. Every method is synchronized, which can lead to performance overhead in multi-threaded environments.
- Java Collections Framework: Many classes in the Java Collections Framework are not synchronized by default (e.g., ArrayList, HashMap). Instead, if synchronization is required, developers can use wrapper classes like
Collections.synchronizedList
orCollections.synchronizedMap
.
- Iterator Support:
- Legacy Classes: Older collection classes typically provide enumeration interfaces for iteration, which have limited functionality compared to iterators. For example, they lack methods for safely removing elements during iteration.
- Java Collections Framework: The Collections Framework introduces iterators, which provide more functionality compared to enumerations. Iterators allow bidirectional traversal, safer removal of elements during iteration, and fail-fast behavior.
- Performance and Scalability:
- Legacy Classes: The synchronization of legacy classes like Vector and Hashtable can introduce performance overhead, especially in multi-threaded scenarios where synchronization is unnecessary.
- Java Collections Framework: Many classes in the Collections Framework are not synchronized by default, resulting in better performance in single-threaded scenarios. Additionally, the Collections Framework provides concurrent collection classes like ConcurrentHashMap for efficient concurrent access.
- Type Safety:
- Legacy Classes: Legacy collection classes such as Vector and Hashtable are not type-safe since they were introduced before the advent of generics in Java.
- Java Collections Framework: The Collections Framework introduced generics, allowing developers to specify the type of elements stored in a collection at compile-time. This improves type safety and reduces the likelihood of runtime errors.
- Fail-fast vs. Enumeration Fail-safe:
- Legacy Classes: The legacy collection classes provide fail-safe enumeration, meaning they do not throw ConcurrentModificationException if the collection is modified during iteration. Instead, they may produce unexpected results.
- Java Collections Framework: The Collections Framework introduces fail-fast iterators, which throw ConcurrentModificationException if the collection is structurally modified during iteration. This behavior helps detect and prevent concurrent modifications, ensuring data integrity.
- Extensibility and Flexibility:
- Legacy Classes: Legacy collection classes like Vector and Hashtable have limited extensibility and flexibility, with fixed functionality and interface.
- Java Collections Framework: The Collections Framework provides a more extensive set of interfaces and classes, allowing for greater extensibility and flexibility. Developers can easily create custom collection implementations or adapt existing ones to suit specific requirements.
- Null Handling:
- Legacy Classes: Legacy collection classes like Vector and Hashtable allow null elements and keys.
- Java Collections Framework: The Collections Framework generally allows null elements in collections but may have specific restrictions for certain implementations, such as TreeSet not allowing null elements.
- Performance Characteristics:
- Legacy Classes: Performance characteristics of legacy classes like Vector and Hashtable may not be as optimized compared to their counterparts in the Java Collections Framework.
- Java Collections Framework: The Collections Framework offers a wider range of collection implementations with different performance characteristics, allowing developers to choose the most suitable one based on their specific requirements.
Understanding these differences helps developers make informed decisions when selecting collection classes for their applications, considering factors such as performance, thread safety, type safety, and extensibility.
Future of the Collections Framework
Potential Additions or Enhancements
- Integration with Reactive Programming:
- Enhance the Collections Framework to better integrate with reactive programming paradigms, enabling developers to handle asynchronous and event-driven data streams more efficiently.
- Support for Immutable Collections:
- Introduce built-in support for immutable collections to promote safer concurrency and facilitate functional programming practices.
- Enhanced Support for Big Data:
- Develop specialized collection implementations optimized for handling large-scale data processing tasks, such as distributed computing and big data analytics.
- Advanced Data Structures:
- Explore the addition of advanced data structures like Bloom filters, trie structures, or skip lists to the Collections Framework, providing more options for specialized use cases.
- Native Support for Data Serialization:
- Improve support for data serialization and deserialization within the Collections Framework, enabling seamless integration with external storage systems and distributed computing frameworks.
- Efficient Stream Processing:
- Enhance support for stream processing operations within the Collections Framework, leveraging parallel processing capabilities for improved performance on multi-core systems.
- Extension of Functional Programming Features:
- Extend functional programming features within the Collections Framework, allowing developers to express complex data transformations and operations using concise and declarative syntax.
- Enhancements for Memory Efficiency:
- Implement optimizations to reduce memory overhead and improve memory efficiency in collection implementations, especially for scenarios involving large datasets or constrained environments.
- Integration with Java Language Features:
- Leverage new language features introduced in recent Java versions (e.g., records, pattern matching) to simplify collection manipulation and improve developer productivity.
- Native Support for Persistent Data Structures:
- Investigate the inclusion of persistent data structures in the Collections Framework, enabling efficient creation and manipulation of immutable data structures for persistent storage and data sharing.
Evolving Needs and Technologies
- Cloud-Native Applications:
- Adapt the Collections Framework to meet the requirements of cloud-native applications, such as scalability, resilience, and support for containerized deployments.
- Microservices Architecture:
- Provide support for microservices architectures by optimizing collection implementations for lightweight and efficient communication between distributed components.
- Machine Learning and AI:
- Align the Collections Framework with the needs of machine learning and artificial intelligence applications, including efficient handling of large datasets and integration with popular ML frameworks.
- Edge Computing:
- Address the challenges of edge computing environments by optimizing collection implementations for low-latency, resource-constrained devices, and intermittent connectivity scenarios.
- Data Privacy and Security:
- Introduce features for ensuring data privacy and security within the Collections Framework, such as encryption, access control, and compliance with regulatory standards.
- Continuous Integration and Delivery (CI/CD):
- Streamline development and deployment processes for the Collections Framework by adopting CI/CD practices and automation tools to ensure faster release cycles and improved reliability.
- Community Feedback and Contributions:
- Continuously engage with the Java developer community to gather feedback, identify pain points, and prioritize enhancements or additions to the Collections Framework based on real-world usage and evolving needs.
By focusing on these potential additions, enhancements, and evolving needs, the Java Collections Framework can remain a versatile and indispensable tool for Java developers in building modern, scalable, and efficient applications across a wide range of domains and industries.
Conclusion
In conclusion, the Java Collections Framework stands as a cornerstone of Java programming, offering a comprehensive set of classes and interfaces for managing collections of objects. Throughout its evolution, the framework has continuously adapted to meet the changing needs of developers and advancements in technology. From its introduction in Java 1.2 to its current state, the Collections Framework has played a pivotal role in simplifying data manipulation, enhancing code reusability, and promoting best practices in software development.
Recap of Key Points
- The Java Collections Framework provides a unified architecture for representing and manipulating collections of objects.
- It offers a wide range of interfaces and implementations for lists, sets, maps, queues, and more.
- Key considerations include time and space complexities, performance characteristics, thread safety, and type safety.
- Best practices include choosing the appropriate collection type, using generics for type safety, and handling concurrent modifications safely.
- The framework has evolved to support modern programming paradigms such as functional programming and reactive programming.
Importance and Ubiquity of the Collections Framework
The Collections Framework holds immense importance and ubiquity in Java programming due to several reasons:
- Standardization: It provides a standardized approach to working with collections, allowing developers to write code that is reusable, interoperable, and easily understandable.
- Efficiency: The framework offers efficient data structures and algorithms for common operations, enabling developers to build high-performance applications.
- Flexibility: With a wide range of collection types and implementations, developers have the flexibility to choose the most suitable data structure for their specific requirements.
- Integration: The Collections Framework seamlessly integrates with other parts of the Java ecosystem, such as streams, lambdas, and concurrency utilities, enabling developers to leverage its capabilities in various contexts.
Final Thoughts
As Java continues to evolve, the Collections Framework will likely continue to evolve alongside it, incorporating new features, optimizations, and enhancements to meet the demands of modern software development. By understanding and mastering the Collections Framework, Java developers can unlock the full potential of the language and build robust, scalable, and maintainable applications for a wide range of domains and industries.
FAQs
1. What is the Java Collections Framework?
- The Java Collections Framework is a set of classes and interfaces in Java that provides reusable data structures to store and manipulate groups of objects. It offers a unified architecture for representing collections such as lists, sets, maps, and queues.
2. What are the core interfaces in the Java Collections Framework?
- The core interfaces in the Java Collections Framework include Collection, List, Set, Map, Queue, and their respective subinterfaces. These interfaces define common methods and behaviors for working with collections of objects.
3. How do I choose the right collection type for my application?
- The choice of collection type depends on factors such as the required ordering, presence of duplicates, access patterns, and performance considerations. For example, use List if you need ordered elements with duplicates, Set for unique elements without a specific order, and Map for key-value pairs.
4. How can I ensure thread safety when using collections in a multi-threaded environment?
- To ensure thread safety, you can use synchronized collection implementations or concurrent collection classes provided by the Java Collections Framework, such as ConcurrentHashMap and CopyOnWriteArrayList. Alternatively, you can use explicit synchronization using synchronized blocks or locks.
5. What are some common pitfalls to avoid when working with collections?
- Common pitfalls include not handling null values properly, not understanding the performance characteristics of collection operations, not handling concurrent modifications during iteration, and choosing inappropriate collection types for specific use cases. It’s essential to understand these pitfalls and follow best practices to avoid them.
Read other awesome articles in Medium.com