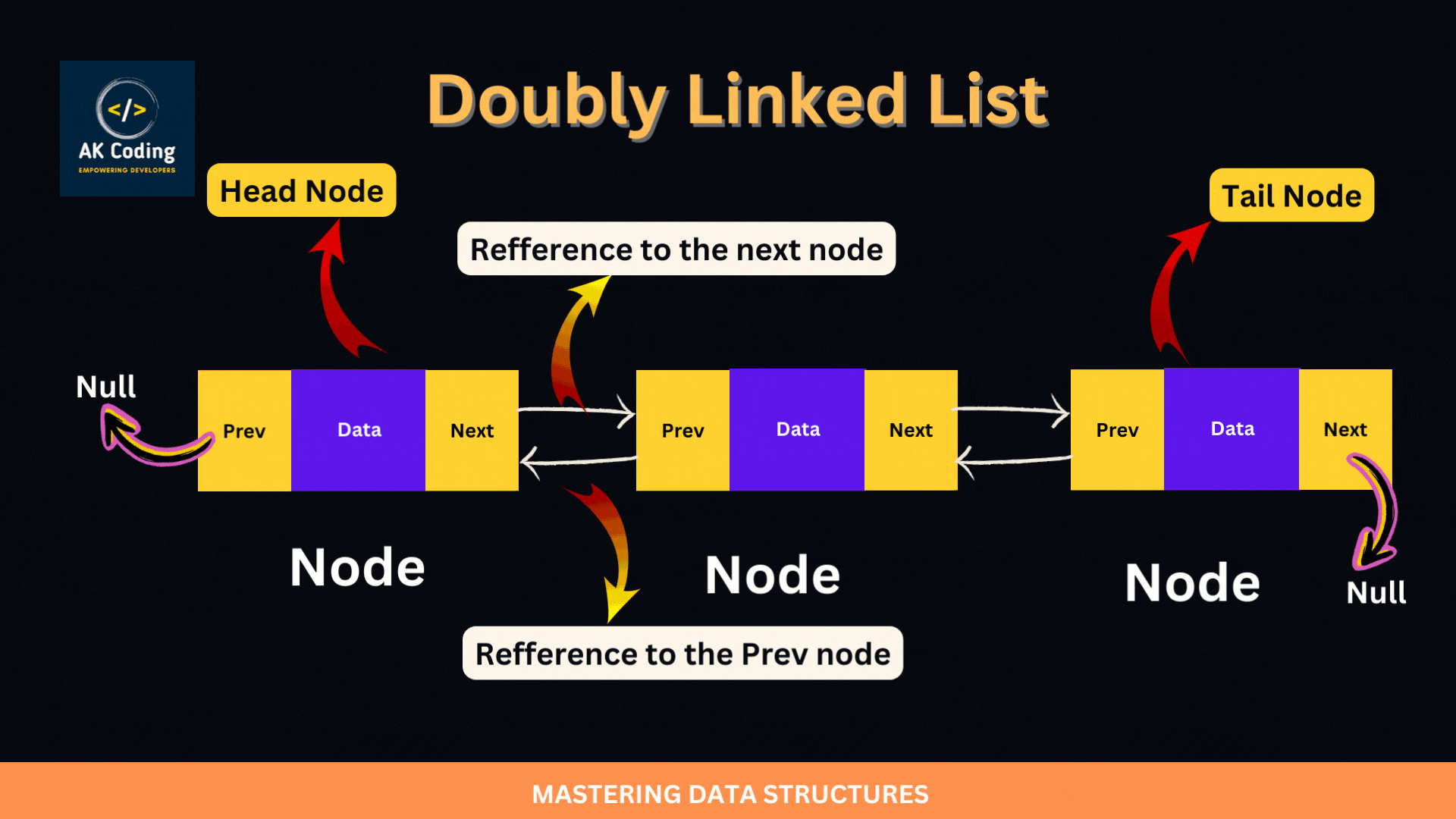
Table of Contents
Introduction to Doubly Linked List in Data Structure
Doubly Linked List stand as a fundamental data structure in computer science, offering enhanced functionality compared to their singly linked counterparts. In this comprehensive introduction, we delve into the intricacies of doubly linked list, exploring their structure, operations, and real-world applications.
Unlike singly linked lists, doubly linked list feature nodes with two pointers, allowing traversal both forwards and backwards. This bidirectional traversal capability empowers efficient insertion, deletion, and searching operations within the list, making doubly linked list a versatile tool in various programming scenarios.
Join us as we embark on a journey to unravel the complexities of doubly linked list, uncovering their advantages, implementation techniques, and best practices. Whether you’re a seasoned programmer or a newcomer to data structures, this guide promises to provide valuable insights into harnessing the power of doubly linked list in your projects.
Advantages and disadvantages
Advantages of Doubly Linked List
- Bidirectional Traversal: Doubly linked list allow traversal in both forward and backward directions, facilitating efficient operations such as searching, insertion, and deletion from both ends of the list.
- Dynamic Size: Similar to singly linked lists, doubly linked list support dynamic resizing, enabling the list to grow or shrink as needed without the need for contiguous memory allocation.
- Enhanced Operations: Doubly linked list offer enhanced operations compared to singly linked lists. For instance, deleting a node requires only constant time complexity O(1) when given a reference to the node, which is not possible in singly linked lists without traversing from the beginning.
- Traversal Flexibility: Bidirectional traversal provides flexibility in scenarios where backward traversal is required, such as implementing undo functionality in applications.
- Memory Efficiency: Although doubly linked list require additional memory for storing the previous pointer, they offer efficient memory utilization by enabling fast and flexible data manipulation.
Disadvantages of Doubly Linked List
- Memory Overhead: Doubly linked list incur extra memory overhead compared to singly linked lists due to the additional storage required for the previous pointer in each node.
- Complexity: Managing doubly linked list can be more complex than singly linked lists due to the bidirectional pointers, which increases the likelihood of bugs and requires careful handling of edge cases.
- Insertion and Deletion Overhead: While doubly linked list offer efficient insertion and deletion at both ends of the list, operations involving nodes in the middle of the list require extra pointer manipulation, leading to increased complexity and potential performance overhead.
- Traversal Complexity: Although doubly linked list support bidirectional traversal, traversing the entire list still requires visiting each node individually, resulting in linear time complexity O(n) for traversal.
- Implementation Complexity: Implementing doubly linked list may require additional effort compared to singly linked lists, especially for beginners, due to the complexity associated with managing bidirectional pointers and ensuring proper synchronization of references.
In summary, while doubly linked list offer enhanced functionality and flexibility compared to singly linked lists, they also come with additional memory overhead and complexity. Understanding the trade-offs between the advantages and disadvantages is crucial when deciding whether to use doubly linked list in specific programming scenarios.
Node Structure of Doubly Linked List
In a Doubly Linked List, each node contains three components:
- Data: This field holds the actual data or value associated with the node.
- Previous Pointer: This pointer/reference points to the previous node in the list. It allows bidirectional traversal, enabling movement from the current node to its predecessor.
- Next Pointer: This pointer/reference points to the next node in the list. Similar to the previous pointer, it facilitates bidirectional traversal by allowing movement from the current node to its successor.
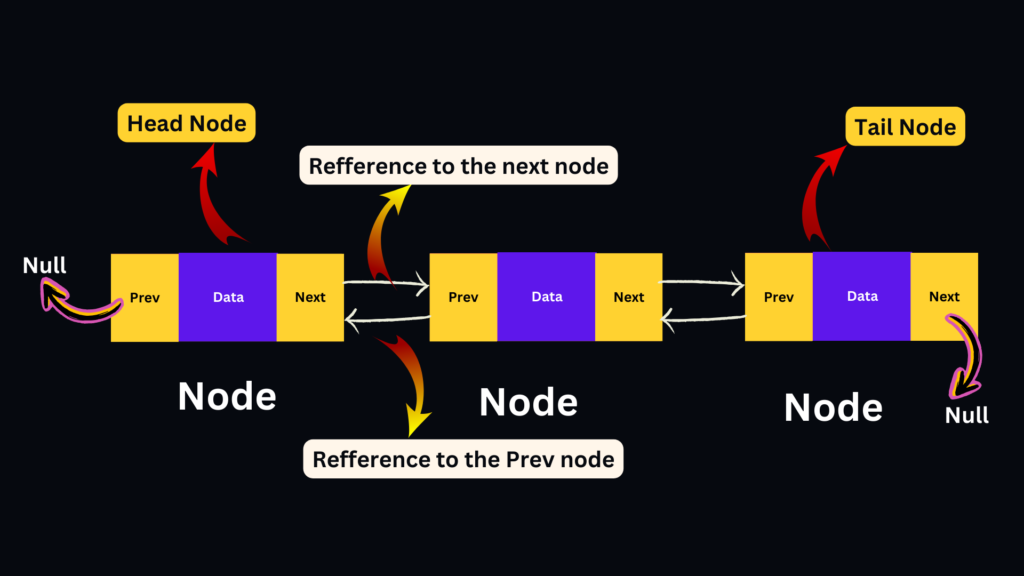
Here’s a basic representation of the node structure of a Doubly Linked List in pseudocode:
Node:
data // Data or value stored in the node
prev // Pointer to the previous node in the list
next // Pointer to the next node in the list
In Java, this node structure can be implemented as follows:
// Node class for Doubly Linked List
private static class Node {
int data; // Data stored in the node
Node prev; // Pointer to the previous node
Node next; // Pointer to the next node
// Constructor to initialize node with data
Node(int data) {
this.data = data;
this.prev = null;
this.next = null;
}
}
Each node in the Doubly Linked List holds a piece of data and maintains references to both the previous and next nodes in the list, enabling efficient bidirectional traversal and manipulation of the list’s elements.
Operations on Doubly Linked List
Insertion
In a Doubly Linked List, insertion operations can be performed at various positions within the list, including at the beginning, end, or middle. Here’s how each insertion operation is typically implemented:
Insertion at the Beginning
- Create a new node with the given data.
- Set the
next
pointer of the new node to point to the current head of the list. - If the list is not empty, update the
prev
pointer of the current head to point to the new node. - Update the head of the list to point to the new node.
Insertion at the End
- Create a new node with the given data.
- Traverse the list to find the last node.
- Set the
next
pointer of the last node to point to the new node. - Set the
prev
pointer of the new node to point to the last node.
Insertion at a Specific Position (Middle)
- Create a new node with the given data.
- Traverse the list to find the node at the desired position.
- Adjust the
next
andprev
pointers of the nodes before and after the desired position to incorporate the new node.
Below is the Java code demonstrating these insertion operations:
// Insertion at the beginning of the Doubly Linked List
public void insertAtBeginning(int data) {
Node newNode = new Node(data);
newNode.next = head;
if (head != null) {
head.prev = newNode;
}
head = newNode;
}
// Insertion at the end of the Doubly Linked List
public void insertAtEnd(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
return;
}
Node last = head;
while (last.next != null) {
last = last.next;
}
last.next = newNode;
newNode.prev = last;
}
// Insertion at a specific position in the Doubly Linked List
public void insertAtPosition(int data, int position) {
if (position < 0) {
System.out.println("Invalid position");
return;
}
if (position == 0) {
insertAtBeginning(data);
return;
}
Node newNode = new Node(data);
Node current = head;
int currentPosition = 0;
while (current != null && currentPosition < position - 1) {
current = current.next;
currentPosition++;
}
if (current == null) {
System.out.println("Invalid position");
return;
}
newNode.next = current.next;
if (current.next != null) {
current.next.prev = newNode;
}
current.next = newNode;
newNode.prev = current;
}
These methods allow insertion of new nodes at the beginning, end, or a specific position within the Doubly Linked List, providing flexibility in managing the list’s elements.
Deletion
In a Doubly Linked List, deletion operations can be performed at various positions within the list, including at the beginning, end, or middle. Here’s how each deletion operation is typically implemented:
Deletion at the Beginning
- Update the head pointer to point to the next node in the list.
- If the list is not empty, set the
prev
pointer of the new head to null.
Deletion at the End
- Traverse the list to find the last node.
- Set the
next
pointer of the second-to-last node to null.
Deletion of a Specific Node (Middle)
- Traverse the list to find the node to be deleted.
- Adjust the
next
andprev
pointers of the nodes before and after the node to be deleted to bypass it.
Below is the Java code demonstrating these deletion operations:
// Deletion at the beginning of the Doubly Linked List
public void deleteAtBeginning() {
if (head == null) {
return;
}
head = head.next;
if (head != null) {
head.prev = null;
}
}
// Deletion at the end of the Doubly Linked List
public void deleteAtEnd() {
if (head == null || head.next == null) {
head = null;
return;
}
Node last = head;
while (last.next != null) {
last = last.next;
}
last.prev.next = null;
}
// Deletion of a specific node in the Doubly Linked List
public void deleteAtPosition(int position) {
if (head == null || position < 0) {
return;
}
if (position == 0) {
deleteAtBeginning();
return;
}
Node current = head;
int currentPosition = 0;
while (current != null && currentPosition < position) {
current = current.next;
currentPosition++;
}
if (current == null) {
return;
}
if (current.next != null) {
current.next.prev = current.prev;
}
if (current.prev != null) {
current.prev.next = current.next;
}
}
These methods allow deletion of nodes from the Doubly Linked List at the beginning, end, or a specific position, providing flexibility in managing the list’s elements.
Searching
In a Doubly Linked List, searching for a specific element involves traversing the list from either the beginning or the end, depending on the desired direction. Here’s how the search operation is typically implemented:
Search from the Beginning
- Start traversing the list from the head node.
- Compare the data of each node with the target element.
- If a node with the target element is found, return the node or its position.
- If the end of the list is reached without finding the target element, return null or -1 to indicate that the element was not found.
Search from the End
- Start traversing the list from the last node.
- Compare the data of each node with the target element.
- If a node with the target element is found, return the node or its position.
- If the beginning of the list is reached without finding the target element, return null or -1 to indicate that the element was not found.
Below is the Java code demonstrating the search operation from the beginning of the Doubly Linked List:
// Search for a specific element in the Doubly Linked List from the beginning
public Node searchFromBeginning(int target) {
Node current = head;
while (current != null) {
if (current.data == target) {
return current; // Element found, return the node
}
current = current.next; // Move to the next node
}
return null; // Element not found
}
And here is the code demonstrating the search operation from the end of the Doubly Linked List:
// Search for a specific element in the Doubly Linked List from the end
public Node searchFromEnd(int target) {
Node current = head;
while (current != null && current.next != null) {
current = current.next; // Move to the last node
}
while (current != null) {
if (current.data == target) {
return current; // Element found, return the node
}
current = current.prev; // Move to the previous node
}
return null; // Element not found
}
These methods allow searching for a specific element in the Doubly Linked List from either the beginning or the end, providing flexibility in accessing and manipulating the list’s elements.
Traversal
Traversal in a Doubly Linked List involves visiting each node in the list sequentially, either from the beginning to the end or from the end to the beginning. Here’s how traversal is typically implemented:
Traversal from the Beginning (Forward Traversal)
- Start traversing the list from the head node.
- Visit each node and perform the desired operation (e.g., printing the data).
- Move to the next node using the
next
pointer. - Continue traversing until reaching the end of the list (i.e., until reaching a node where the
next
pointer is null).
Traversal from the End (Reverse Traversal):
- Start traversing the list from the last node.
- Visit each node and perform the desired operation.
- Move to the previous node using the
prev
pointer. - Continue traversing until reaching the beginning of the list (i.e., until reaching a node where the
prev
pointer is null).
Below is the Java code demonstrating traversal of a Doubly Linked List in both forward and reverse directions:
// Forward traversal of the Doubly Linked List
public void forwardTraversal() {
Node current = head;
while (current != null) {
System.out.print(current.data + " "); // Print the data of the current node
current = current.next; // Move to the next node
}
System.out.println(); // Print a newline after traversing all nodes
}
// Reverse traversal of the Doubly Linked List
public void reverseTraversal() {
if (head == null) {
return;
}
Node current = head;
while (current.next != null) {
current = current.next; // Move to the last node
}
while (current != null) {
System.out.print(current.data + " "); // Print the data of the current node
current = current.prev; // Move to the previous node
}
System.out.println(); // Print a newline after traversing all nodes
}
These methods allow traversing a Doubly Linked List in both forward and reverse directions, providing flexibility in accessing and processing the list’s elements.
Reversal
To reverse a Doubly Linked List, you need to swap the prev
and next
pointers of each node in the list. Additionally, you will need to update the head pointer to point to the new last node (which was the first node before reversal) and the tail pointer to point to the new first node (which was the last node before reversal).
Here’s how you can implement the reversal of a Doubly Linked List in Java:
public void reverse() {
if (head == null || head.next == null) {
return; // List is empty or has only one node, no need to reverse
}
Node current = head;
Node temp = null;
// Swap the prev and next pointers of each node
while (current != null) {
temp = current.prev;
current.prev = current.next;
current.next = temp;
current = current.prev; // Move to the next node in the original direction
}
// Update the head pointer to point to the new last node
if (temp != null) {
head = temp.prev;
}
// Update the tail pointer to point to the new first node
if (head != null) {
tail = head.prev;
}
}
In this implementation, we traverse the list from the head node and swap the prev
and next
pointers of each node. After completing the traversal, we update the head pointer to point to the new last node (which was the first node before reversal) and the tail pointer to point to the new first node (which was the last node before reversal).
This method reverses the entire Doubly Linked List in place, without requiring additional memory or creating a new list. After calling this method, the original list will be reversed.
Applications of Doubly Linked List
Doubly Linked List find applications in various scenarios where bidirectional traversal and efficient insertion and deletion operations are required. Some common applications include:
- Implementation of Undo Functionality: Doubly Linked List are commonly used in applications that require undo functionality, such as text editors or graphic design software. Each node in the list represents a state change, and users can navigate through the list to undo or redo actions.
- Cache Implementation: Doubly Linked List are used in cache implementations where recently accessed items need to be quickly retrieved and removed. Nodes can be added to the front of the list when an item is accessed, and the least recently used item can be removed from the end of the list.
- Browser History: Doubly Linked List are used in web browsers to implement the forward and backward navigation functionality. Each node in the list represents a visited webpage, and users can navigate through their browsing history using the forward and backward pointers.
- Music Playlist: Doubly Linked List are used in music player applications to implement playlists. Each node in the list represents a song, and users can navigate through the playlist in both forward and backward directions.
- Deque (Double-Ended Queue) Implementation: Doubly Linked List are used to implement double-ended queues, where elements can be inserted and deleted from both ends of the queue efficiently.
- Symbol Table Implementation: Doubly Linked List are used in compilers and interpreters to implement symbol tables, which store information about variables, functions, and other identifiers in a program.
- Dynamic Memory Allocation: Doubly Linked List are used in dynamic memory allocation systems, where memory blocks of varying sizes need to be efficiently managed and allocated.
- Database Management Systems: Doubly Linked List are used in database management systems to implement indexes and linked lists of records for efficient data retrieval and manipulation.
These are just a few examples of the many applications of Doubly Linked List. Their versatility and ability to support bidirectional traversal make them valuable data structures in a wide range of programming scenarios.
Implementation of Doubly Linked List in Java
Here’s a basic implementation of a Doubly Linked List in Java:
public class DoublyLinkedList {
private Node head;
private Node tail;
private static class Node {
int data;
Node prev;
Node next;
Node(int data) {
this.data = data;
}
}
// Constructor
public DoublyLinkedList() {
this.head = null;
this.tail = null;
}
// Method to check if the list is empty
public boolean isEmpty() {
return head == null;
}
// Method to insert a node at the beginning of the list
public void insertAtBeginning(int data) {
Node newNode = new Node(data);
if (isEmpty()) {
head = tail = newNode;
} else {
newNode.next = head;
head.prev = newNode;
head = newNode;
}
}
// Method to insert a node at the end of the list
public void insertAtEnd(int data) {
Node newNode = new Node(data);
if (isEmpty()) {
head = tail = newNode;
} else {
tail.next = newNode;
newNode.prev = tail;
tail = newNode;
}
}
// Method to display the list in forward direction
public void displayForward() {
if (isEmpty()) {
System.out.println("List is empty");
return;
}
Node current = head;
while (current != null) {
System.out.print(current.data + " ");
current = current.next;
}
System.out.println();
}
// Method to display the list in backward direction
public void displayBackward() {
if (isEmpty()) {
System.out.println("List is empty");
return;
}
Node current = tail;
while (current != null) {
System.out.print(current.data + " ");
current = current.prev;
}
System.out.println();
}
// Main method for testing
public static void main(String[] args) {
DoublyLinkedList dll = new DoublyLinkedList();
dll.insertAtBeginning(10);
dll.insertAtBeginning(20);
dll.insertAtEnd(30);
dll.insertAtEnd(40);
System.out.println("Doubly Linked List in forward direction:");
dll.displayForward();
System.out.println("Doubly Linked List in backward direction:");
dll.displayBackward();
}
}
This implementation includes methods to insert nodes at the beginning and end of the list, as well as to display the list in both forward and backward directions. You can further extend this implementation by adding methods for deletion, searching, and other operations as needed.
Operations implementation
Here’s a more complete implementation of a Doubly Linked List in Java, including operations for insertion, deletion, searching, and traversal:
public class DoublyLinkedList {
private Node head;
private Node tail;
private static class Node {
int data;
Node prev;
Node next;
Node(int data) {
this.data = data;
}
}
// Constructor
public DoublyLinkedList() {
this.head = null;
this.tail = null;
}
// Method to check if the list is empty
public boolean isEmpty() {
return head == null;
}
// Method to insert a node at the beginning of the list
public void insertAtBeginning(int data) {
Node newNode = new Node(data);
if (isEmpty()) {
head = tail = newNode;
} else {
newNode.next = head;
head.prev = newNode;
head = newNode;
}
}
// Method to insert a node at the end of the list
public void insertAtEnd(int data) {
Node newNode = new Node(data);
if (isEmpty()) {
head = tail = newNode;
} else {
tail.next = newNode;
newNode.prev = tail;
tail = newNode;
}
}
// Method to delete a node from the beginning of the list
public void deleteAtBeginning() {
if (isEmpty()) {
System.out.println("List is empty, nothing to delete");
return;
}
head = head.next;
if (head != null) {
head.prev = null;
} else {
tail = null;
}
}
// Method to delete a node from the end of the list
public void deleteAtEnd() {
if (isEmpty()) {
System.out.println("List is empty, nothing to delete");
return;
}
tail = tail.prev;
if (tail != null) {
tail.next = null;
} else {
head = null;
}
}
// Method to search for a node with given data
public boolean search(int data) {
Node current = head;
while (current != null) {
if (current.data == data) {
return true; // Element found
}
current = current.next;
}
return false; // Element not found
}
// Method to display the list in forward direction
public void displayForward() {
if (isEmpty()) {
System.out.println("List is empty");
return;
}
Node current = head;
while (current != null) {
System.out.print(current.data + " ");
current = current.next;
}
System.out.println();
}
// Method to display the list in backward direction
public void displayBackward() {
if (isEmpty()) {
System.out.println("List is empty");
return;
}
Node current = tail;
while (current != null) {
System.out.print(current.data + " ");
current = current.prev;
}
System.out.println();
}
// Main method for testing
public static void main(String[] args) {
DoublyLinkedList dll = new DoublyLinkedList();
dll.insertAtBeginning(10);
dll.insertAtEnd(20);
dll.insertAtBeginning(5);
dll.insertAtEnd(30);
System.out.println("Doubly Linked List in forward direction:");
dll.displayForward();
System.out.println("Doubly Linked List in backward direction:");
dll.displayBackward();
System.out.println("Searching for 20: " + dll.search(20));
System.out.println("Searching for 25: " + dll.search(25));
dll.deleteAtBeginning();
dll.deleteAtEnd();
System.out.println("Doubly Linked List after deletion:");
dll.displayForward();
}
}
This implementation includes methods for insertion at the beginning and end of the list, deletion from the beginning and end of the list, searching for a specific node, and traversal in both forward and backward directions.
Difference between singly and doubly linked list
Here’s a comparison between Singly Linked Lists and Doubly Linked Lists in tabular format:
Feature | Singly Linked List | Doubly Linked List |
---|---|---|
Structure | Each node contains data and a reference to the next node. | Each node contains data and references to both the next and previous nodes. |
Traversal | Forward traversal only | Forward and backward traversal |
Memory Requirement | Requires less memory (only one reference per node) | Requires more memory (two references per node) |
Insertion | Efficient insertion at the beginning | Efficient insertion at both beginning and end |
Deletion | Efficient deletion at the beginning | Efficient deletion at both beginning and end |
Reversal | Requires extra effort for reversal | Can be reversed efficiently |
Operations | Limited operations due to forward traversal | Supports bidirectional traversal and operations like reversal |
Complexity | Generally simpler to implement and manage | Slightly more complex due to additional references |
Use Cases | Suitable for scenarios requiring forward traversal only, memory optimization, or simplicity | Suitable for scenarios requiring bidirectional traversal, efficient insertion/deletion at both ends, or reversal operations |
This comparison highlights the key differences between Singly Linked Lists and Doubly Linked Lists, including their structure, traversal capabilities, memory requirements, efficiency in insertion and deletion, support for reversal operations, complexity in implementation, and use cases.
Conclusion
In conclusion, the Doubly Linked List is a fundamental data structure with several key features and applications. Let’s recap the key points, discuss its importance, and consider future advancements:
Recap of Key Points
- Doubly Linked List consists of nodes where each node contains data and references to both the next and previous nodes.
- Nodes can be efficiently inserted and deleted from both ends of the list, enabling bidirectional traversal.
- It offers advantages over singly linked lists, such as easier deletion of nodes, efficient traversal in both directions, and support for operations like reversing the list.
Importance of Doubly Linked List
- Doubly Linked List are crucial in scenarios where efficient insertion, deletion, and traversal operations in both directions are required.
- They find applications in various domains, including text editors, cache implementations, browser history, and symbol tables in compilers.
- Their flexibility and versatility make them indispensable in designing efficient data structures and algorithms.
Future Considerations and Advancements
- Future advancements in Doubly Linked List may focus on optimizing memory usage and improving performance for specific applications.
- Research into advanced techniques for memory management, such as memory pooling and efficient garbage collection, could enhance the efficiency of Doubly Linked List.
- Integration with modern programming paradigms and frameworks, such as functional programming and reactive programming, may lead to innovative use cases and optimizations.
In summary, the Doubly Linked List remains a foundational data structure with significant relevance in software development. Understanding its principles, applications, and potential advancements is essential for building efficient and scalable systems in the future.
FAQs
What is Doubly Linked List?
A Doubly Linked List is a linear data structure consisting of nodes where each node contains data and references to both the next and previous nodes. This structure allows bidirectional traversal, making it efficient for insertion, deletion, and traversal operations in both directions.
How is a Doubly Linked List different from a Singly Linked List?
In a Singly Linked List, each node contains a reference to the next node only, while in a Doubly Linked List, each node contains references to both the next and previous nodes. This difference allows for bidirectional traversal and more efficient deletion operations in Doubly Linked List compared to Singly Linked Lists.
What are the advantages of using a Doubly Linked List?
Advantages of Doubly Linked List include efficient insertion and deletion operations at both ends of the list, bidirectional traversal, and support for operations like reversing the list. These features make Doubly Linked List suitable for applications requiring frequent insertion, deletion, and traversal in both directions.
What are the disadvantages of Doubly Linked List?
Disadvantages of Doubly Linked List include higher memory overhead due to storing references to both next and previous nodes, increased complexity in implementation compared to Singly Linked Lists, and the potential for inconsistency if not properly managed (e.g., dangling references).
When should I use a Doubly Linked List instead of other data structures?
Doubly Linked List are suitable for scenarios where bidirectional traversal, efficient insertion/deletion at both ends, and support for operations like reversing the list are required. Common use cases include implementing undo functionality, managing browser history, and implementing double-ended queues (dequeues).
Read other awesome articles in Medium.com