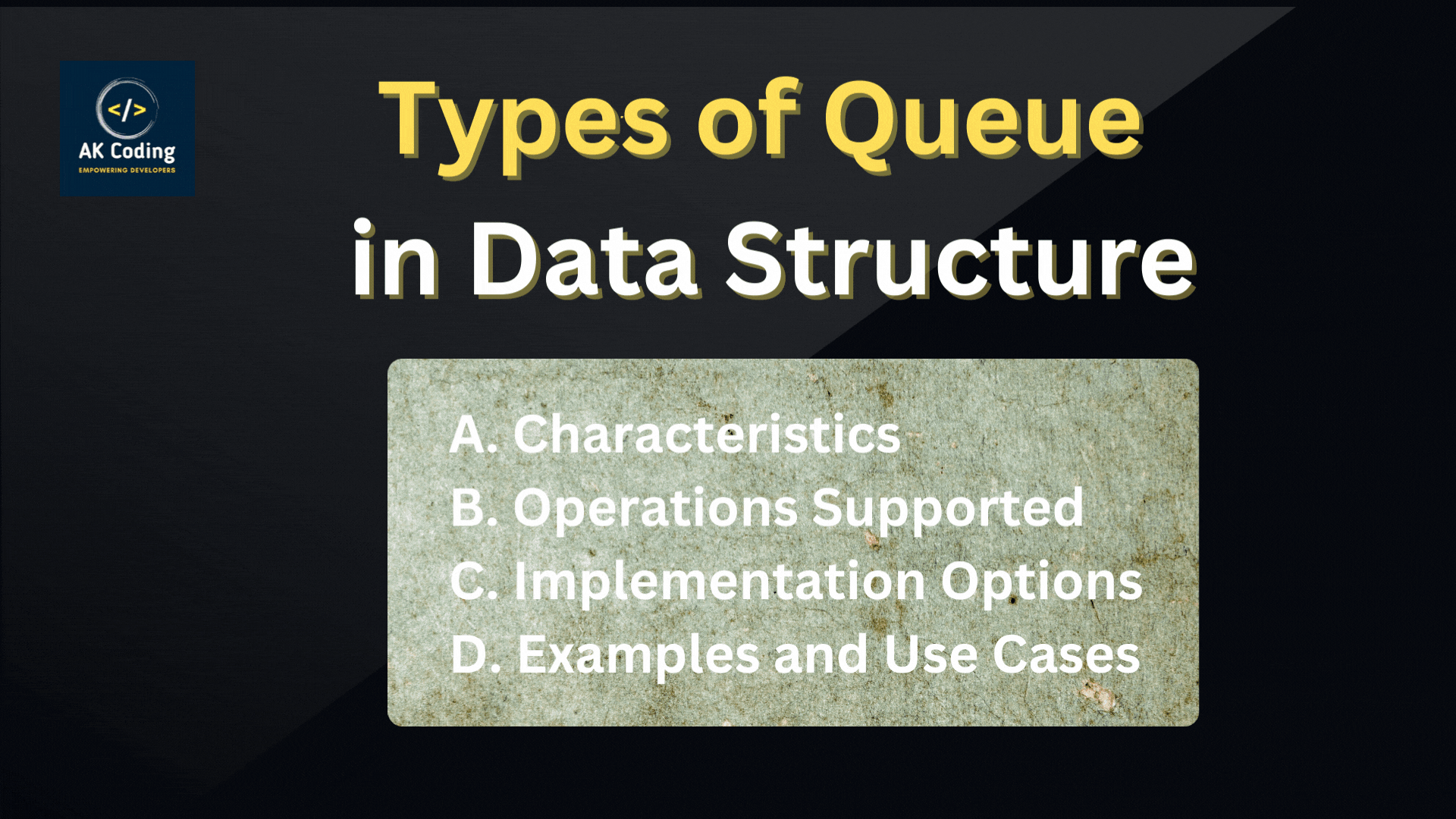
Table of Contents
Introduction
Definition of a Queue
A queue is a linear data structure that follows the First-In-First-Out (FIFO) principle, where elements are inserted at the rear and removed from the front. It resembles a queue of people waiting for a service, where the first person who enters the queue is the first to be served.
Overview of Queue Data Structure
- Queues are commonly used to model real-world scenarios such as waiting lines, job scheduling, and task processing.
- They are characterized by two primary operations: enqueue (adding an element to the rear of the queue) and dequeue (removing an element from the front of the queue).
- Queues can be implemented using arrays, linked lists, or other data structures, each with its own advantages and disadvantages.
- In addition to enqueue and dequeue, other operations supported by queues include peeking (viewing the element at the front of the queue without removing it) and checking whether the queue is empty or full.
Importance and Applications in Computer Science:
- Job Scheduling: Queues are used in operating systems to manage processes waiting to be executed by the CPU. Jobs are placed in a queue based on priority or arrival time.
- Breadth-First Search (BFS) in Graphs: Queues are essential for implementing BFS algorithm, which explores vertices in a graph level by level. It uses a queue to store and process vertices.
- Buffering in I/O Operations: Queues are used to buffer input/output data in computer systems, ensuring smooth and efficient processing of data streams.
- Network Routing: In computer networks, queues are used for packet switching and routing. Data packets are placed in queues at routers and switches before being forwarded to their destinations.
- Multithreading and Synchronization: Queues are used for inter-thread communication and synchronization in concurrent programming. Blocking queues ensure safe access to shared resources among multiple threads.
- Print Queue Management: Printers use queues to manage print jobs. Print requests are queued up, and the printer processes them one by one based on their order of arrival.
In computer science, queues play a fundamental role in various algorithms, data structures, and system designs, contributing to the efficiency, reliability, and scalability of software and hardware systems.
Types of queue in data structure
Linear Queue
Characteristics of Linear Queue
- Follows the First-In-First-Out (FIFO) principle, where the first element inserted is the first one to be removed.
- Elements are added at the rear (enqueue) and removed from the front (dequeue).
- Linear queues can be implemented using arrays or linked lists.
Operations Supported in Linear Queue
- Enqueue: Adds an element to the rear of the queue.
- Dequeue: Removes and returns the element at the front of the queue.
- Front: Returns the element at the front of the queue without removing it.
- Rear: Returns the element at the rear of the queue without removing it.
- isEmpty: Checks if the queue is empty.
- isFull: Checks if the queue is full (applicable only for bounded queues).
Linear Queue Implementation Options
- Array-based Implementation:
- Uses an array to store queue elements.
- Requires maintaining front and rear pointers to keep track of the queue’s boundaries.
- Enqueue and dequeue operations have O(1) time complexity.
- However, resizing the array may require O(n) time if the array needs to be copied to a larger one.
- Linked List-based Implementation:
- Uses a linked list to store queue elements.
- Requires maintaining references to the front and rear nodes.
- Enqueue and dequeue operations have O(1) time complexity, as adding or removing elements from the ends of a linked list is constant time.
- Does not have a fixed size limit like array-based implementation, allowing for dynamic resizing.
Examples and Use Cases:
- Job Scheduling: Linear queues are used in operating systems for job scheduling. Processes waiting to be executed are placed in a queue and processed in the order they arrive.
- Breadth-First Search (BFS): BFS algorithm uses a queue to store and process vertices level by level in a graph traversal. It explores neighboring vertices before moving to the next level.
- Print Queue Management: Printers use linear queues to manage print jobs. Print requests are queued up, and the printer processes them one by one based on their order of arrival.
- Buffering in I/O Operations: Linear queues are used to buffer input/output data in computer systems, ensuring smooth and efficient processing of data streams.
- Network Packet Switching: Queues are used in computer networks for packet switching and routing. Data packets are placed in queues at routers and switches before being forwarded to their destinations.
Linear queues are versatile data structures widely used in computer science and software engineering for managing and processing data in various applications, ranging from operating systems to networking and algorithm design.
Circular Queue
Characteristics of Circular Queue
- Circular queues are a type of queue data structure where the last element is connected to the first element, forming a circular structure.
- They optimize space utilization in array-based implementations by reusing empty slots after dequeue operations.
- Circular queues prevent unnecessary shifting of elements during enqueue and dequeue operations, leading to improved performance.
Operations Supported in Circular Queue
- Enqueue: Adds an element to the rear of the circular queue.
- Dequeue: Removes and returns the element from the front of the circular queue.
- Front: Returns the element at the front of the circular queue without removing it.
- Rear: Returns the element at the rear of the circular queue without removing it.
- IsEmpty: Checks if the circular queue is empty.
- IsFull: Checks if the circular queue is full.
Circular Queue Implementation Options
- Array-based Implementation:
- Circular queues can be implemented using arrays by maintaining two pointers: front and rear.
- After each dequeue operation, the front pointer is incremented, and after each enqueue operation, the rear pointer is incremented.
- When the rear pointer reaches the end of the array, it wraps around to the beginning of the array (circular behavior).
- The circular queue is considered full when
(rear + 1) % capacity == front
, and empty whenfront == rear
.
Examples and Use Cases:
- Buffering in Data Streams:
- Circular queues are used in buffering input/output data streams in computer systems.
- They efficiently manage data flow by cyclically reusing buffer space, ensuring smooth processing of data.
- Memory Management:
- Circular queues are employed in memory management systems to allocate and deallocate memory blocks.
- They enable efficient reuse of memory space by recycling freed memory blocks in a circular manner.
- Task Scheduling:
- In real-time systems and task scheduling algorithms, circular queues are used to manage tasks waiting to be executed.
- Tasks are enqueued based on their priority or arrival time, and dequeued in a circular fashion for execution.
- Resource Allocation:
- Circular queues are utilized in resource allocation scenarios, such as CPU scheduling and disk management.
- Resources are allocated to processes or applications in a circular manner, ensuring fair access and utilization.
- Buffering in Communication Systems:
- Circular queues are employed in communication systems for buffering data packets in routers and switches.
- They prevent packet loss and ensure efficient data transmission by cyclically managing data flow.
Priority Queue
Characteristics of Priority Queue
- A priority queue is a type of queue where each element has a priority associated with it.
- Elements are dequeued based on their priority, not the order in which they were enqueued.
- Higher-priority elements are dequeued before lower-priority ones.
- It does not follow the FIFO (First-In-First-Out) principle like a regular queue.
Operations Supported in Priority Queue
- Enqueue: Adds an element to the priority queue while preserving the order based on priority.
- Dequeue: Removes and returns the highest-priority element from the priority queue.
- Peek: Retrieves the highest-priority element from the priority queue without removing it.
- IsEmpty: Checks if the priority queue is empty.
- Size: Returns the number of elements in the priority queue.
Priority Queue Implementation Options
- Using Heaps:
- Binary heaps, such as min heap or max heap, are commonly used to implement priority queues.
- Min heaps are used for priority queues where lower values represent higher priorities, while max heaps are used for the opposite scenario.
- Operations like enqueue, dequeue, and peek can be efficiently performed in O(log n) time complexity using heaps.
- Using Balanced Binary Search Trees:
- Balanced binary search trees, such as AVL trees or Red-Black trees, can also be used to implement priority queues.
- These trees maintain the order of elements based on priority, allowing for efficient insertion, deletion, and retrieval operations.
Priority Queue Examples and Use Cases
- Job Scheduling:
- Priority queues are used in operating systems for job scheduling, where processes are executed based on their priority levels.
- High-priority tasks are given preference over lower-priority ones, ensuring timely execution of critical tasks.
- Dijkstra’s Shortest Path Algorithm:
- Priority queues are used in Dijkstra’s algorithm for finding the shortest paths in a weighted graph.
- The algorithm maintains a priority queue of vertices based on their tentative distances from the source vertex, allowing it to explore vertices with lower distances first.
- Huffman Coding:
- Priority queues are used in Huffman coding, a lossless data compression algorithm.
- The algorithm builds a binary tree of characters based on their frequencies, with higher-frequency characters having lower depths in the tree.
- Task Scheduling in Real-time Systems:
- Priority queues are used in real-time systems for scheduling tasks with deadlines.
- Tasks with higher-priority deadlines are scheduled first to ensure timely completion and meet the system’s real-time constraints.
- Network Routing:
- Priority queues are used in network routing algorithms for packet switching and forwarding.
- Packets are assigned priorities based on factors like Quality of Service (QoS) requirements or network congestion levels, allowing routers to prioritize critical traffic.
Priority queues are versatile data structures widely used in various applications where elements need to be processed based on their priority levels rather than their order of arrival. They provide efficient solutions for managing and processing prioritized data, contributing to the optimization and reliability of software systems.
Double-Ended Queue (Deque)
Characteristics of Double-Ended Queue
- A double-ended queue, often abbreviated as deque, is a versatile linear data structure that allows insertion and deletion of elements from both the front and the rear ends.
- It provides operations similar to both stacks and queues, allowing elements to be added or removed from either end of the deque.
- Deques can grow or shrink dynamically based on the number of elements they contain.
Operations Supported in Double-Ended Queue
- Insertion Operations:
- InsertFront: Add an element to the front of the deque.
- InsertRear: Add an element to the rear of the deque.
- Deletion Operations:
- DeleteFront: Remove an element from the front of the deque.
- DeleteRear: Remove an element from the rear of the deque.
- Access Operations:
- GetFront: Retrieve the element at the front of the deque without removing it.
- GetRear: Retrieve the element at the rear of the deque without removing it.
- Other Operations:
- IsEmpty: Check if the deque is empty.
- Size: Get the number of elements in the deque.
Double-Ended Queue Implementation Options
- Doubly Linked List-based Implementation:
- In this implementation, each element of the deque is represented as a node in a doubly linked list.
- Operations like insertion and deletion can be performed efficiently by manipulating pointers.
- Array-based Implementation:
- Deques can also be implemented using arrays with dynamic resizing to accommodate changing sizes.
- Circular arrays or dynamic arrays can be used to optimize performance and memory usage.
Double-Ended Queue Examples and Use Cases
- Deque as a Queue:
- Deques can be used as a general-purpose queue where elements are added to the rear and removed from the front.
- Example: Job scheduling in an operating system, where processes are queued up for execution.
- Deque as a Stack:
- Deques can be used as a stack where elements are added and removed from the same end (either front or rear).
- Example: Undo/Redo functionality in text editors or graphics software.
- Deque for Input Buffering:
- Deques can be used to buffer input data, allowing efficient processing of streams or packets.
- Example: Buffering input from a keyboard or network socket.
- Deque for Algorithmic Problems:
- Deques are used in algorithmic problems such as sliding window problems, where elements are added and removed from both ends during processing.
- Example: Implementing a data structure for the maximum sliding window problem.
Double-ended queues offer flexibility and efficiency in various scenarios where elements need to be added or removed from both ends, making them a valuable tool in algorithm design, system development, and software engineering.
Blocking Queue
Characteristics of Blocking Queue
- Thread-Safe: Blocking queues are designed to be thread-safe, meaning they support concurrent access by multiple threads without the risk of data corruption or inconsistency.
- Blocking Operations: Blocking queues support blocking operations, where certain operations (such as enqueue and dequeue) may block the calling thread if the queue is empty or full, until the condition is satisfied.
- Synchronization: Internally, blocking queues use synchronization mechanisms such as locks, conditions, or semaphores to ensure thread safety and proper synchronization between producer and consumer threads.
- Bounded or Unbounded: Blocking queues can be either bounded (with a fixed capacity) or unbounded (with no fixed capacity).
Operations Supported in Blocking Queue
- Enqueue (Put): Adds an element to the rear of the queue. If the queue is full, the operation may block until space becomes available.
- Dequeue (Take): Removes and returns the element at the front of the queue. If the queue is empty, the operation may block until an element is available.
- Peek: Returns the element at the front of the queue without removing it. This operation typically does not block.
- Size: Returns the number of elements currently in the queue.
- Empty / Full Check: Determines whether the queue is empty or full.
Blocking Queue Implementation Options
- Using Locks and Conditions: Blocking queues can be implemented using low-level synchronization primitives such as locks and conditions provided by the language’s concurrency utilities.
- Using Semaphores: Semaphores can be used to implement blocking operations in the queue, where semaphores are used to signal availability of elements or space in the queue.
- Using Built-in Libraries: Most programming languages offer built-in libraries or frameworks that provide high-level implementations of blocking queues, abstracting away the low-level synchronization details.
Blocking Queue Examples and Use Cases
- Producer-Consumer Problem: Blocking queues are commonly used to solve the producer-consumer problem, where one or more threads (producers) produce data items, and one or more threads (consumers) consume these items. Blocking queues facilitate safe and efficient communication and synchronization between producers and consumers.
- Thread Pool Management: Blocking queues are used in thread pool implementations to manage tasks awaiting execution. Worker threads dequeue tasks from the queue and execute them, ensuring proper load balancing and resource utilization.
- Event Handling: In graphical user interfaces (GUIs) and event-driven applications, blocking queues are used to handle and process user events. Events are enqueued into the queue and processed sequentially by event-handling threads.
- Parallel Programming: In parallel and distributed computing, blocking queues facilitate coordination and communication between parallel tasks and distributed components, ensuring proper synchronization and data exchange.
- Task Scheduling: Blocking queues are used in task scheduling systems to manage and prioritize tasks awaiting execution. Tasks with higher priority are dequeued first, ensuring timely execution of critical tasks.
Concurrent Queue
Characteristics of Concurrent Queue
- Thread-Safety: Concurrent queues are designed to support multiple threads accessing the queue concurrently without causing data corruption or inconsistencies.
- Synchronization: They employ synchronization mechanisms to ensure atomicity of operations and prevent race conditions.
- Blocking Operations: Concurrent queues may support blocking operations, where threads may block or wait for certain conditions to be met before proceeding.
Operations Supported in Concurrent Queue
- Enqueue: Adds an element to the rear of the queue.
- Dequeue: Removes and returns the element from the front of the queue.
- Peek: Retrieves the element at the front of the queue without removing it.
- Size: Returns the number of elements currently in the queue.
- isEmpty: Checks if the queue is empty.
Concurrent Queue Implementation Options
- BlockingQueue Interface (Java): Java provides the
BlockingQueue
interface in thejava.util.concurrent
package, which defines a set of methods for concurrent queue operations. Implementations such asArrayBlockingQueue
,LinkedBlockingQueue
, andPriorityBlockingQueue
are available. - ConcurrentLinkedQueue (Java): This class in Java is an unbounded, thread-safe queue implementation based on a linked structure. It supports high-concurrency scenarios with efficient non-blocking algorithms.
- Lock-based Implementations: Concurrent queues can be implemented using locks, such as mutexes or semaphores, to provide thread-safe access to the underlying data structure.
- Atomic Operations: Some languages and libraries offer atomic operations for concurrent queue implementations, ensuring that operations are performed atomically without requiring explicit locking.
Concurrent Queue Examples and Use Cases
- Producer-Consumer Problem: Concurrent queues are commonly used to solve the producer-consumer problem, where multiple producer threads produce data items and multiple consumer threads consume them. Blocking queues ensure proper synchronization and efficient resource utilization.
- Task Scheduling: In concurrent programming, task scheduling systems often use concurrent queues to manage tasks awaiting execution by worker threads. Tasks are enqueued into the queue and dequeued by available worker threads for processing.
- Thread Pool Management: Concurrent queues are integral to managing thread pools, where tasks are submitted to the pool and executed by worker threads. Blocking queues ensure that tasks are processed efficiently and that worker threads are not overwhelmed.
- Event Handling: In event-driven systems, concurrent queues are used to manage event queues, ensuring proper handling of events by multiple event-processing threads without data corruption or race conditions.
Concurrent queues are essential in concurrent and parallel programming scenarios, providing efficient and thread-safe access to shared resources and facilitating synchronization between multiple threads.
Conclusion
In conclusion, queues are fundamental data structures that play a crucial role in computer science and software development. This article explored various types of queues, each with its own characteristics, operations, implementation options, and use cases. From basic linear queues to advanced concurrent queues, the diversity of queue types allows for efficient data handling, synchronization, and task management in a wide range of applications.
Summary of Key Points:
- Queues follow the First-In-First-Out (FIFO) principle, where elements are added to the rear and removed from the front.
- Linear queues, circular queues, priority queues, double-ended queues (deques), blocking queues, and concurrent queues are among the common types of queues.
- Linear queues are simple and widely used, while circular queues optimize space utilization.
- Priority queues prioritize elements based on certain criteria, and double-ended queues support insertion and deletion from both ends.
- Blocking queues ensure thread-safe access and synchronization, while concurrent queues facilitate concurrent access by multiple threads.
- Queues find applications in job scheduling, breadth-first search, network routing, multithreading, and more.
Future Developments and Applications of Queue Data Structures:
- Optimization for High-Performance Systems: Continued research and development aim to optimize queue implementations for high-performance computing and real-time systems.
- Integration with Distributed Systems: Queues are increasingly used in distributed systems and cloud computing architectures for efficient message passing and workload distribution.
- Enhanced Synchronization Mechanisms: Future developments may focus on advanced synchronization mechanisms and lock-free algorithms for concurrent queues to improve scalability and performance.
- Application in Emerging Technologies: Queue data structures will continue to play a crucial role in emerging technologies such as Internet of Things (IoT), edge computing, and artificial intelligence (AI) for efficient data processing and resource management.
In summary, the evolution and advancement of queue data structures will continue to drive innovation and enable the development of efficient, scalable, and reliable software systems across various domains and industries.
FAQs
Advantages of circular queue over linear queue
Here’s a comparison of the advantages of circular queue over linear queue
Advantages | Circular Queue | Linear Queue |
---|---|---|
Efficient Space Utilization | Circular queue optimizes space utilization by reusing empty spaces after dequeue operations. | Linear queue may suffer from wasted space due to empty slots left after dequeue operations. |
Reduced Memory Overhead | Circular queue reduces memory overhead as it does not need to resize the underlying array frequently. | Linear queue may require resizing the array when it becomes full, leading to additional memory overhead. |
Improved Performance | Circular queue offers better performance for enqueue and dequeue operations as it avoids shifting elements during dequeue. | Linear queue may incur higher time complexity for dequeue operations due to shifting of elements. |
Simplified Implementation | Circular queue simplifies implementation as it does not require additional logic to handle wraparound when reaching the end of the array. | Linear queue may require extra logic to handle wraparound or resizing of the underlying array. |
Suitable for Applications with Fixed Buffer Size | Circular queue is suitable for applications with a fixed buffer size, such as in embedded systems or hardware queues. | Linear queue may not be as efficient for fixed-size buffer applications due to potential wasted space. |
Better for Real-time Systems | Circular queue is preferred in real-time systems where predictable and consistent performance is critical. | Linear queue may introduce unpredictable latency during enqueue and dequeue operations, affecting real-time systems. |
In summary, circular queues offer several advantages over linear queues, including efficient space utilization, reduced memory overhead, improved performance, simplified implementation, suitability for fixed-size buffer applications, and better performance in real-time systems. These advantages make circular queues a preferred choice in various scenarios where efficient data handling and predictable performance are crucial.
Difference between circular queue and linear queue
Here’s a comparison of circular queue and linear queue
Feature | Circular Queue | Linear Queue |
---|---|---|
Data Structure | Circular buffer with a fixed-size array or linked list | Linear array or linked list |
Structure | Circular arrangement, where the last element points to the first element, forming a loop | Linear arrangement, where elements are stored sequentially |
Enqueue Operation | Inserts elements at the rear end of the queue | Inserts elements at the rear end of the queue |
Dequeue Operation | Removes elements from the front end of the queue | Removes elements from the front end of the queue |
Space Utilization | Optimizes space utilization by reusing empty slots after dequeuing elements | May suffer from wasted space due to empty slots left after dequeuing elements |
Memory Overhead | May have lower memory overhead as resizing of the underlying array is not required frequently | May require resizing of the underlying array when it becomes full, leading to additional memory overhead |
Performance | Offers better performance for enqueue and dequeue operations as it avoids shifting elements | May incur higher time complexity for dequeue operations due to shifting of elements |
Implementation Complexity | Simplifies implementation as it does not require additional logic to handle wraparound when reaching the end of the array | May require extra logic to handle wraparound or resizing of the underlying array |
Suitability for Fixed-size Buffers | Suitable for applications with a fixed buffer size, such as in embedded systems or hardware queues | May not be as efficient for fixed-size buffer applications due to potential wasted space |
Real-time Systems | Preferred in real-time systems where predictable and consistent performance is critical | May introduce unpredictable latency during enqueue and dequeue operations, affecting real-time systems |
In summary, circular queues and linear queues have distinct characteristics and suitability for different scenarios. Circular queues optimize space utilization, simplify implementation, and offer better performance for certain operations, making them suitable for real-time systems and fixed-size buffer applications. On the other hand, linear queues may suffer from wasted space and require additional logic for handling wraparound or resizing, but they are commonly used in various applications due to their simplicity and flexibility.
Read other awesome articles in Medium.com