Have you ever wondered why some programming languages are lightning-fast while others seem to lag behind? If you’ve worked with loops, especially nested ones, you’ve likely noticed this difference. Today, we’re diving into how different languages handle 1 billion iterations of nested loops and why C/C++ dominate in speed while Python takes its time.
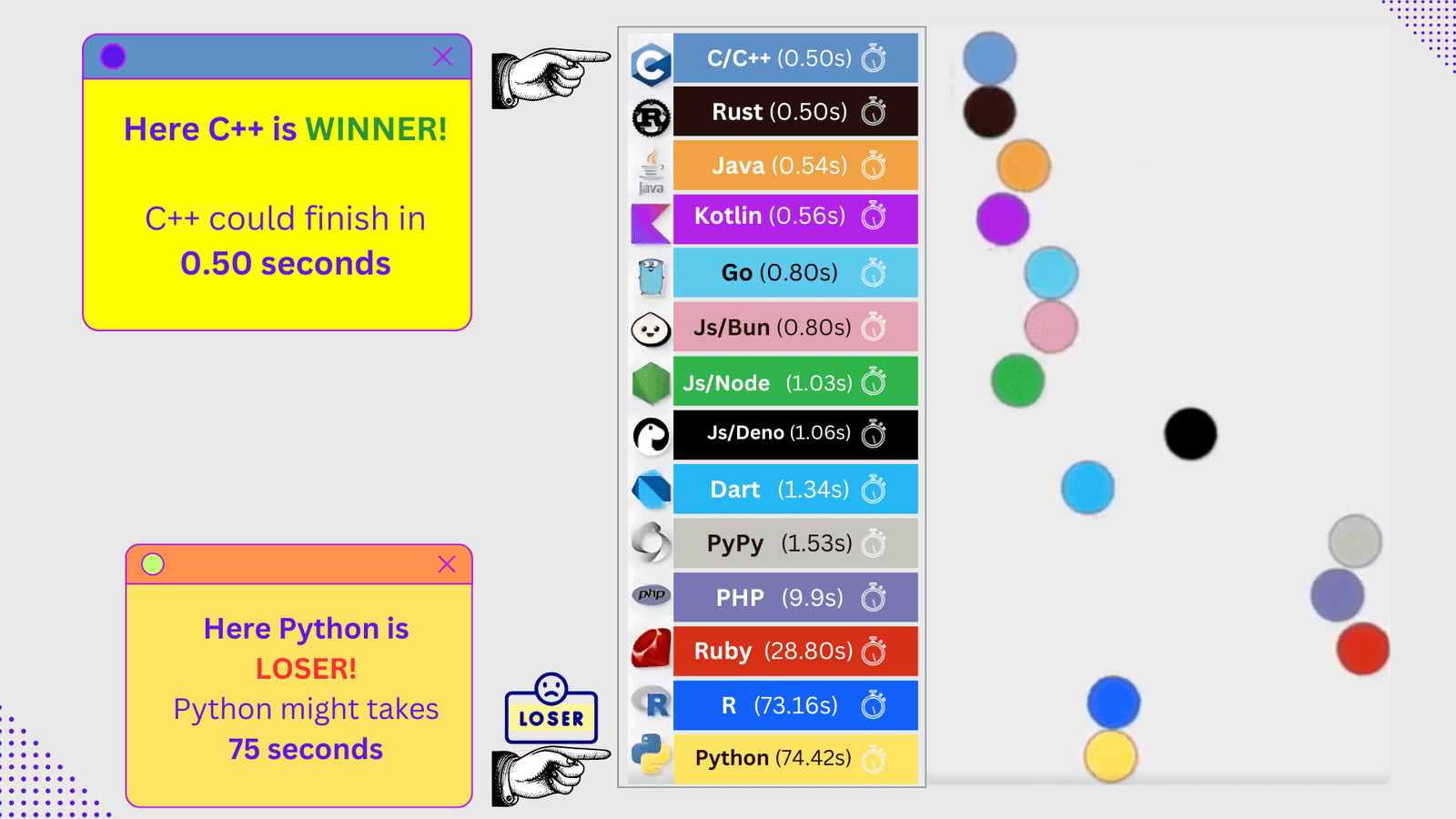
1 Billion Nested Loop Iterations in Different Languages
Table of Contents
Setting the Stage: The Task
Imagine you have two nested loops:
for i = 0 to 999,999:
for j = 0 to 999:
# Perform a simple operation
This structure results in 1 billion (1,000,000 x 1,000) iterations. We’ll compare the performance of this task in different languages:
Why C and C++ Are Fast
C and C++ are known for their blazing speed, and there are a few reasons why:
1. Compiled to Machine Code
C and C++ are compiled languages. When you write a program in C or C++, the compiler translates it into machine code, which is directly executed by the CPU. This eliminates the overhead of interpretation at runtime.
2. Minimal Abstraction
C and C++ provide low-level control over hardware. They don’t have the layers of abstraction found in high-level languages, meaning fewer instructions for the CPU to process.
3. Optimized Memory Management
Memory allocation and deallocation in C and C++ are handled manually or efficiently through features like stack allocation, which makes loop iterations faster.
4. Optimizing Compilers
Modern C/C++ compilers (like GCC and Clang) optimize the code during compilation. For instance, they might unroll loops or eliminate redundant calculations.
Example Code in C++:
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 1000000; i++) {
for (int j = 0; j < 1000; j++) {
// Simple operation
}
}
return 0;
}
Why Python is Slow
Python, while being one of the most popular and versatile programming languages, lags in performance for such tasks. Here’s why:
1. Interpreted Language
Python is an interpreted language, meaning the code is executed line-by-line by the Python interpreter at runtime. This adds significant overhead compared to compiled languages.
2. Dynamic Typing
In Python, variables are dynamically typed. Each operation involves type checking, which slows down execution.
3. Higher Abstraction
Python prioritizes developer productivity and readability over low-level performance. This abstraction means more instructions are processed per iteration.
4. Global Interpreter Lock (GIL)
Python’s GIL ensures only one thread executes Python bytecode at a time, even on multi-core systems. While this doesn’t directly affect single-threaded loops, it highlights Python’s limitations in CPU-bound tasks.
Example Code in Python:
for i in range(1000000):
for j in range(1000):
# Simple operation
Benchmark Results
Here’s a rough comparison of execution times for 1 billion iterations:
Language | Execution Time (approx.) |
---|---|
C | ~0.5 seconds |
C++ | ~0.5 seconds |
Python | ~74 seconds |
The exact times may vary depending on the hardware and compiler/interpreter, but the trend is clear: C and C++ are orders of magnitude faster.
Optimizing Python
If you must use Python, here are some tips to improve performance:
- Use NumPy: NumPy’s vectorized operations leverage C under the hood, making them much faster than Python loops.
- JIT Compilation: Use tools like PyPy or Numba to compile Python code to machine code at runtime.
- Avoid Nested Loops: Refactor your code to minimize nested loops or move performance-critical sections to C/C++ using extensions.
Conclusion
When raw performance is key, especially for compute-intensive tasks like nested loops, C and C++ reign supreme. Python, with its ease of use and flexibility, may not compete in speed, but it’s still a great choice for most applications. Knowing the strengths and limitations of each language helps you make the right choice for your specific needs.
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
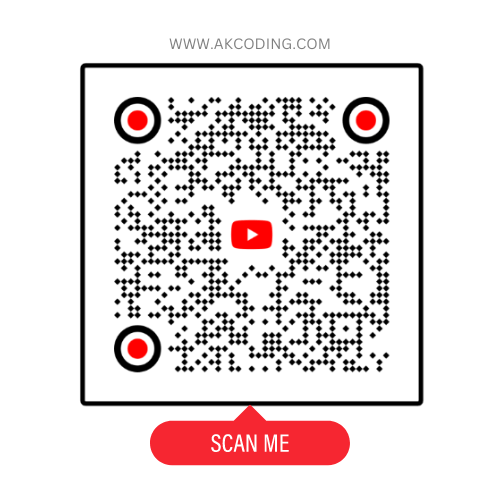