If you are seeking clarity on virtual DOM in react js and differences between virtual DOM and real DOM then this blog post give you clear ficture of react virtual DOM, diffing algorithom like Reconciliation and Fiber engin.
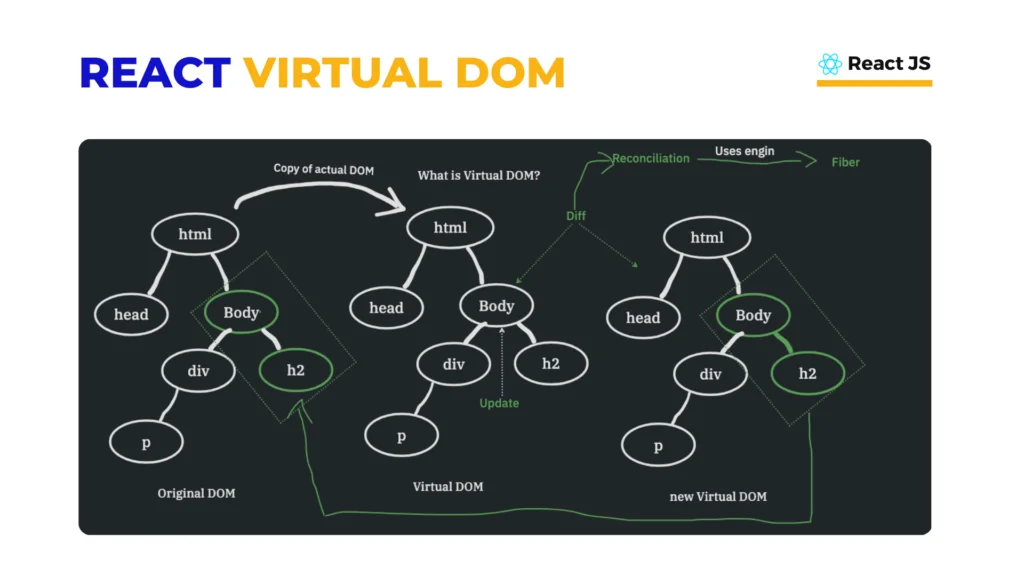
Table of Contents
What is DOM ?
The acronym for Document Object Model is DOM. The language used on websites is HTML. It offers numerous unique tags for the web page structure, such as the ability to link multiple pages together. A document object with a tree structure represents the structure of a web page. The document object’s structure can be altered by the JavaScript programming language to add dynamic behavior to web pages.
The programming interface for documents with a tree structure on web pages is called the DOM. The term “DOM Tree” refers to the document tree.
Visual Diagram of DOM
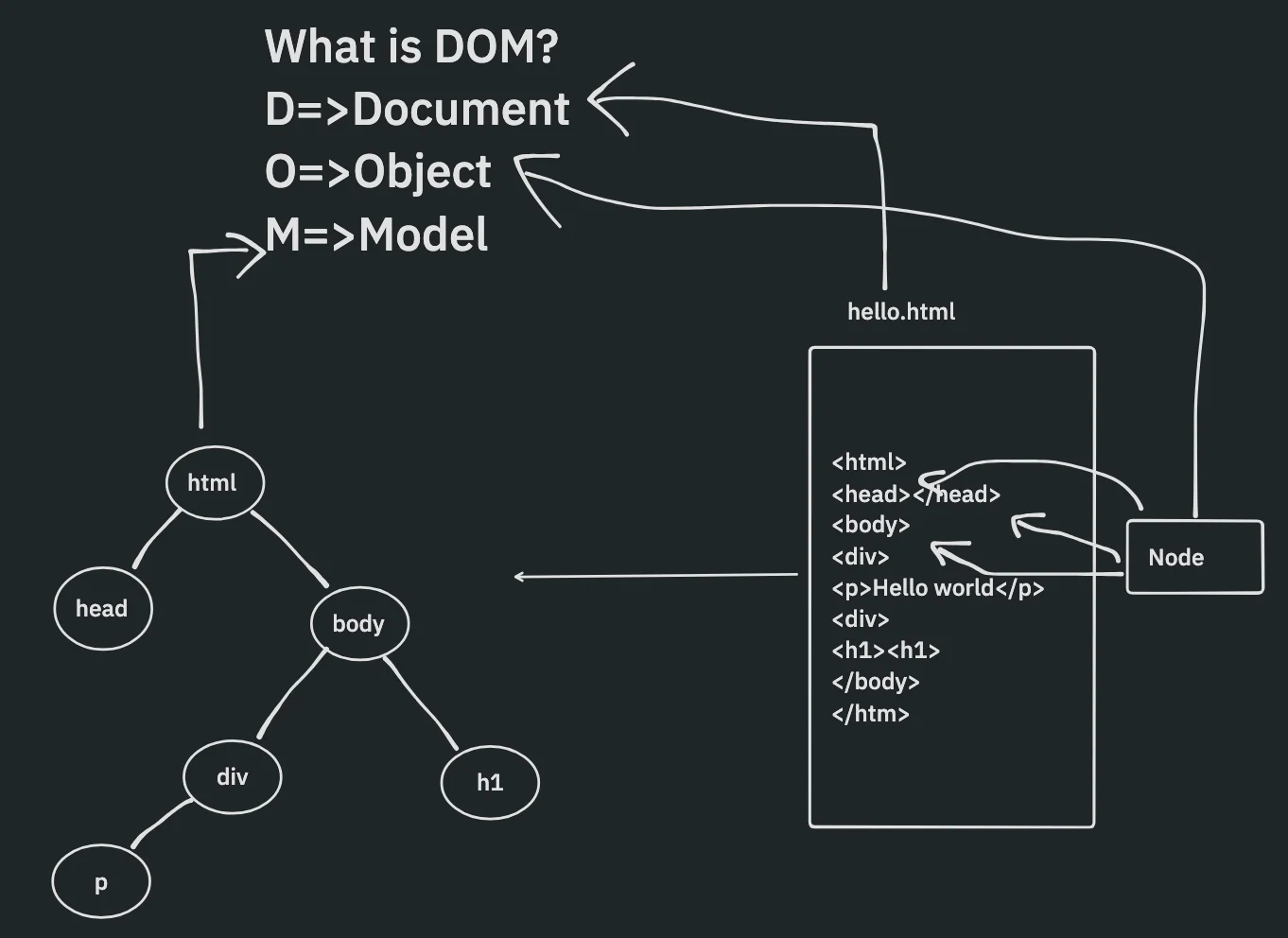
DOM Manipulation
Now, in case we do need to modify the paragraph’s content (the <p> tag), we will require the capability to locate the <p> tag within the document tree and subsequently provide a new text value to it. All of these tasks require the use of the JavaScript DOM APIs.

- Mechanism of finding a particular node in the document tree is called Querying in the DOM.
- Adding/deleting/updating a node, in the document tree is called DOM Manipulation.
- The result of a DOM manipulation reflects on the web user interface. This process is called rendering.
DOM Manipulation is Expensive?
It is expensive to update the DOM frequently. It can cause the website to function worse and load more slowly. Rendering takes longer than querying and updating because the DOM is represented as a tree structure. If we have to go through a sizable amount of the DOM tree to locate the node to update, it could potentially be expensive.
Let’s take a look at the employee table that displays the user’s name and marital status below.
User table
The DOM manipulation will be expensive if we have to navigate this table’s document tree representation each time an update is needed.

Solution

What is Virtual DOM ?
ReactJS never makes direct modifications to the original DOM (unless a developer use case requires it). In ReactJS, an equivalent in-memory duplicate of each DOM object is generated. We refer to this copy as the Virtual DOM (VDOM).
Every element in the Virtual DOM tree is represented by a node. Whenever the state of the element changes, a new Virtual DOM tree gets constructed. The virtual DOM tree will be compared between its current and prior versions using ReactJS’s diffing method. Lastly, the diff is updated in the real DOM by the Virtual DOM using the algorithm.
Visual Representation of VDOM

Virtual DOM Key Note
- First, ReactJS creates a copy of the original DOM, calling it the Virtual DOM. Each of the nodes of the Virtual DOM represents an element.
- Next, if there is a state update of an element, a new Virtual DOM is created.
- The diffing algorithm identifies the difference of the change. In this case, a subtree from the changed node has been identified as the diff.
- Last, the ReactJS runs a batch update to update the Original DOM with these changes to keep it in sync.
Reconciliation & Fiber
Reconciliation is the process of diffing one tree with another to identify the portions that require modification and then updating the original DOM accordingly. Since version 16.0, ReactJS has been using a new reconciliation engine called Fiber.