Introduction
Embarking on a journey into the realm of coding interviews can be both exciting and challenging. Whether you’re a seasoned developer looking to refine your skills or a coding enthusiast preparing for your first interview, this comprehensive guide will equip you with the knowledge and strategies needed to tackle coding interview questions with confidence.
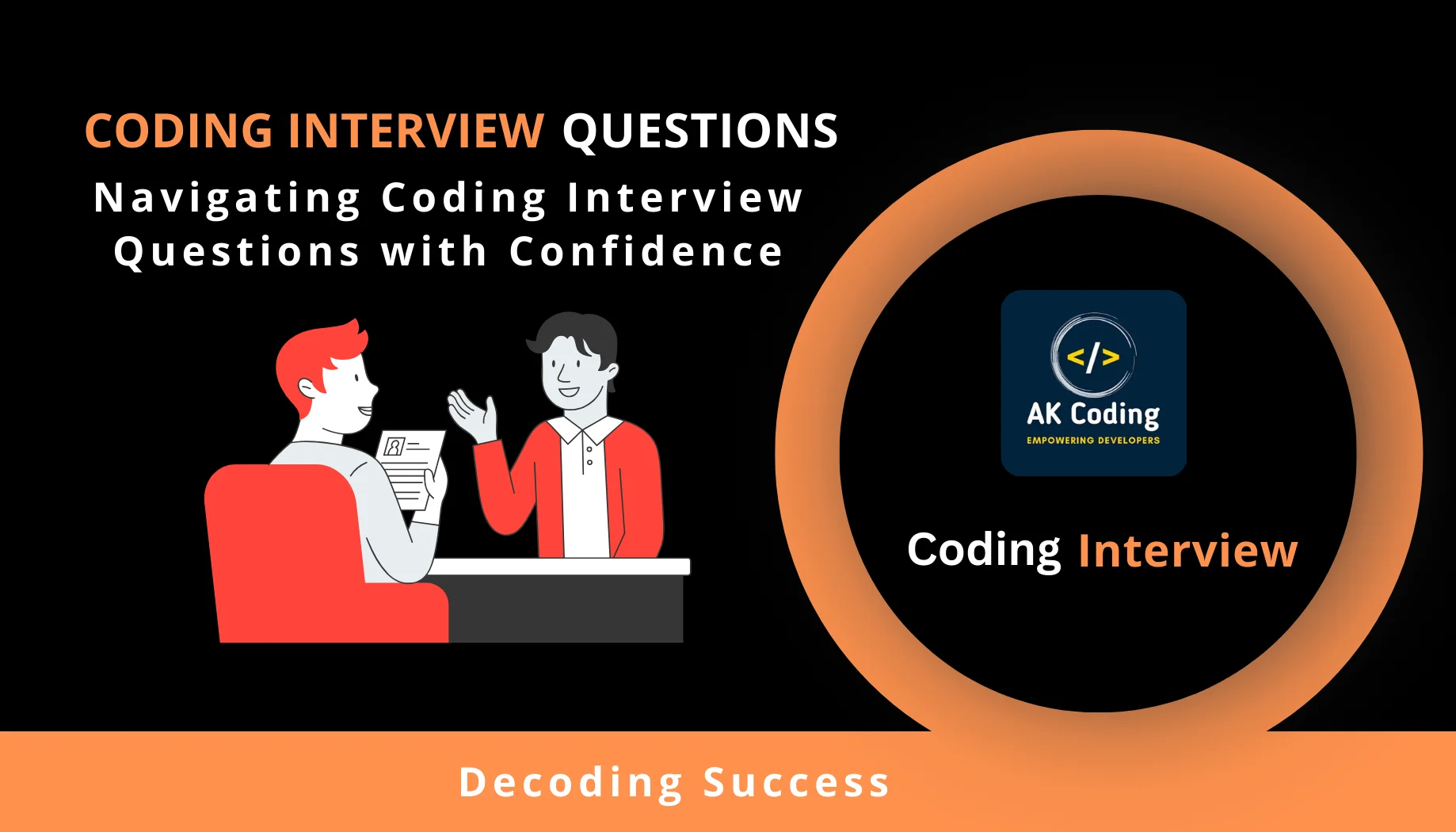
Table of Contents
6 Key sections for coding interview:
1. Understanding the Landscape:
- Navigating through the types of coding interviews.
- Insight into common interview formats and structures.
- Recognizing the importance of problem-solving and algorithmic thinking.
2. Essential Data Structures:
- Exploring fundamental data structures: arrays, linked lists, stacks, and queues.
- Understanding the nuances of trees, graphs, and hash tables.
- Mastering when and how to use each data structure effectively.
3. Algorithms Demystified:
- Deep dive into sorting and searching algorithms.
- Grasping dynamic programming and greedy algorithms.
- Unraveling the complexities of recursion and backtracking.
4. System Design and Object-Oriented Principles:
- Breaking down system design questions and strategies.
- Applying object-oriented design principles effectively.
- Handling scalability and performance considerations.
5. Preparing for Behavioral Questions:
- Crafting compelling responses to common behavioral questions.
- Showcasing problem-solving skills through real-world scenarios.
- Strategies for handling stress and maintaining composure.
6. Coding Interview Best Practices:
- Practicing mock interviews and utilizing online platforms.
- Tips for effective time management during coding interviews.
- Building a robust portfolio and resume that stands out.
Common coding interview questions
Coding interviews often cover a range of topics, including data structures, algorithms, problem-solving skills, and sometimes system design. Here are some common coding interview questions that you might encounter:
1. Arrays and Strings:
- Find the missing number in an array of 1 to N.
- Implement an algorithm to rotate an array.
- Determine if a string has all unique characters.
- Check if two strings are anagrams.
2. Linked Lists:
- Reverse a linked list.
- Detect a cycle in a linked list.
- Find the intersection point of two linked lists.
3. Trees and Graphs:
- Implement depth-first search (DFS) and breadth-first search (BFS).
- Find the lowest common ancestor in a binary tree.
- Check if a binary tree is balanced.
- Perform a topological sort on a directed graph.
4. Sorting and Searching:
- Implement binary search.
- Merge two sorted arrays.
- Find the Kth largest/smallest element in an array.
5. Dynamic Programming:
- Solve the Fibonacci sequence using dynamic programming.
- Longest common subsequence.
- Knapsack problem.
6. Bit Manipulation:
- Count set bits in an integer.
- Find the single non-repeating element in an array of duplicates.
7. Design Patterns:
- Implement Singleton pattern.
- Implement a simple Observer pattern.
8. System Design:
- Design a URL shortening service.
- Design a distributed cache system.
- Design a scalable messaging system.
9. Database Queries:
- Write SQL queries to retrieve data from multiple tables.
- Optimize a given SQL query.
10. Miscellaneous:
- Implement a basic calculator.
- Determine if a number is prime.
- Implement a stack with push, pop, top, and retrieving the minimum element in constant time.
These are just a few examples, and the specific questions may vary depending on the company and the level of the position you’re interviewing for. It’s crucial to practice a variety of problems and understand the underlying concepts to perform well in coding interviews.
Advanced coding interview questions
Advanced coding interview questions often involve more complex algorithms, data structures, and problem-solving skills. Here are a few examples:
1. Hard Dynamic Programming: Longest Increasing Subsequence
- Given an unsorted array of integers, find the length of the longest increasing subsequence.
2. Graph Algorithms: Shortest Path
- Implement Dijkstra’s algorithm or Bellman-Ford algorithm to find the shortest path in a weighted graph.
3. Tree Algorithms: AVL Trees or Red-Black Trees
- Implement and balance an AVL tree or a Red-Black tree and perform operations like insertion, deletion, and searching.
4. Advanced Sorting: Merge Sort or QuickSort
- Implement an efficient sorting algorithm like Merge Sort or QuickSort and discuss its time and space complexities.
5. Bit Manipulation: Maximum XOR of Two Numbers in an Array
- Given an array of integers, find the maximum XOR of any two elements.
6. Concurrency: Multi-threading or Parallel Programming
- Solve a problem that requires handling concurrency, such as designing a thread-safe data structure or solving a parallel computing problem.
7. Advanced Dynamic Programming: Edit Distance
- Given two strings, find the minimum number of operations required to convert one string into another (operations: insert, remove, replace).
8. Hard Search Problems: A* Algorithm
- Implement the A* algorithm for pathfinding in a grid, taking into account heuristics and cost functions.
9. Database Optimization: Indexing and Query Optimization
- Discuss strategies for optimizing database queries, indexing, and improving overall database performance.
10. System Design: Scalability and Load Balancing
- Design a scalable system that can handle a large number of users, discussing load balancing, database sharding, and other scalability concepts.
These questions are designed to test your deep understanding of algorithms, data structures, and system design. Interviewers may also focus on your ability to analyze the efficiency of your solutions and discuss trade-offs. It’s crucial to not only solve the problem but also communicate your thought process clearly. Practice and familiarity with a wide range of advanced topics will be beneficial for handling such questions effectively.
Tips for coding interviews
Certainly! Here are some tips to help you excel in coding interviews:
1. Understand the Problem
- Carefully read and understand the problem statement. Clarify any ambiguities with the interviewer before jumping into coding.
2. Ask Questions
- Don’t hesitate to ask clarifying questions. Interviewers appreciate candidates who seek additional information to ensure a complete understanding.
3. Plan and Discuss Approaches
- Take a moment to plan your approach before coding. Discuss your thought process with the interviewer to demonstrate problem-solving skills.
4. Start with Simple Examples
- Test your solution with simple examples to verify the logic before moving on to more complex cases. This helps catch potential issues early.
5. Brute Force First
- If the optimal solution isn’t immediately apparent, start with a brute-force solution. It’s better to have a working solution that you can optimize later.
6. Optimize Your Code
- Once you have a working solution, think about optimizing it. Discuss potential optimizations with the interviewer before implementing them.
7. Use Descriptive Variable Names
- Choose meaningful variable names that convey the purpose of the variable. This improves code readability.
8. Modularize Your Code
- Break down the problem into smaller, manageable functions. This not only makes your code more modular but also helps with testing and debugging.
9. Handle Edge Cases
- Consider edge cases and test your solution with them. This demonstrates a thorough understanding of the problem.
10. Practice Time Management
- Manage your time effectively. If you're stuck on a part of the problem, consider moving on and revisiting it later.
11. Learn Data Structures and Algorithms
- Be comfortable with fundamental data structures (arrays, linked lists, trees, graphs) and common algorithms (sorting, searching, dynamic programming).
12. Practice Regularly
- Regular practice on platforms like LeetCode, HackerRank, or CodeSignal is crucial. Familiarity with various problem types enhances your problem-solving skills.
13. Mock Interviews
- Conduct mock interviews with friends or use online platforms that simulate real interview scenarios. This helps in practicing communication and problem-solving under pressure.
14. Review Your Mistakes
- After solving a problem, review your solution. Understand why you made certain choices and how you can improve. Learning from mistakes is crucial for growth.
15. Stay Calm
- Keep a calm demeanor during the interview. If you get stuck, take a deep breath and approach the problem methodically.
16. Communicate Clearly
- Articulate your thought process clearly. Interviewers appreciate candidates who communicate effectively, explaining their approach and reasoning.
17. Follow-Up Questions
- Be prepared for follow-up questions that explore different aspects of the problem or ask you to optimize your solution further.
18. Stay Updated
- Stay informed about the latest technologies and industry trends. Some interviews might include questions about recent advancements.
19. Build a Portfolio
- Showcase your coding projects on platforms like GitHub. Having a portfolio of projects can make a positive impression on interviewers.
20. Learn from Feedback
- If you receive feedback from mock interviews or real interviews, use it as a learning opportunity. Continuous improvement is key.
Remember, coding interviews are not just about the correct solution but also about how you approach problems, communicate, and handle challenges. Practice consistently, learn from experiences, and you’ll become more confident and effective in coding interviews.
Web Stories
You can refer GeeksForGeeks to learn coding