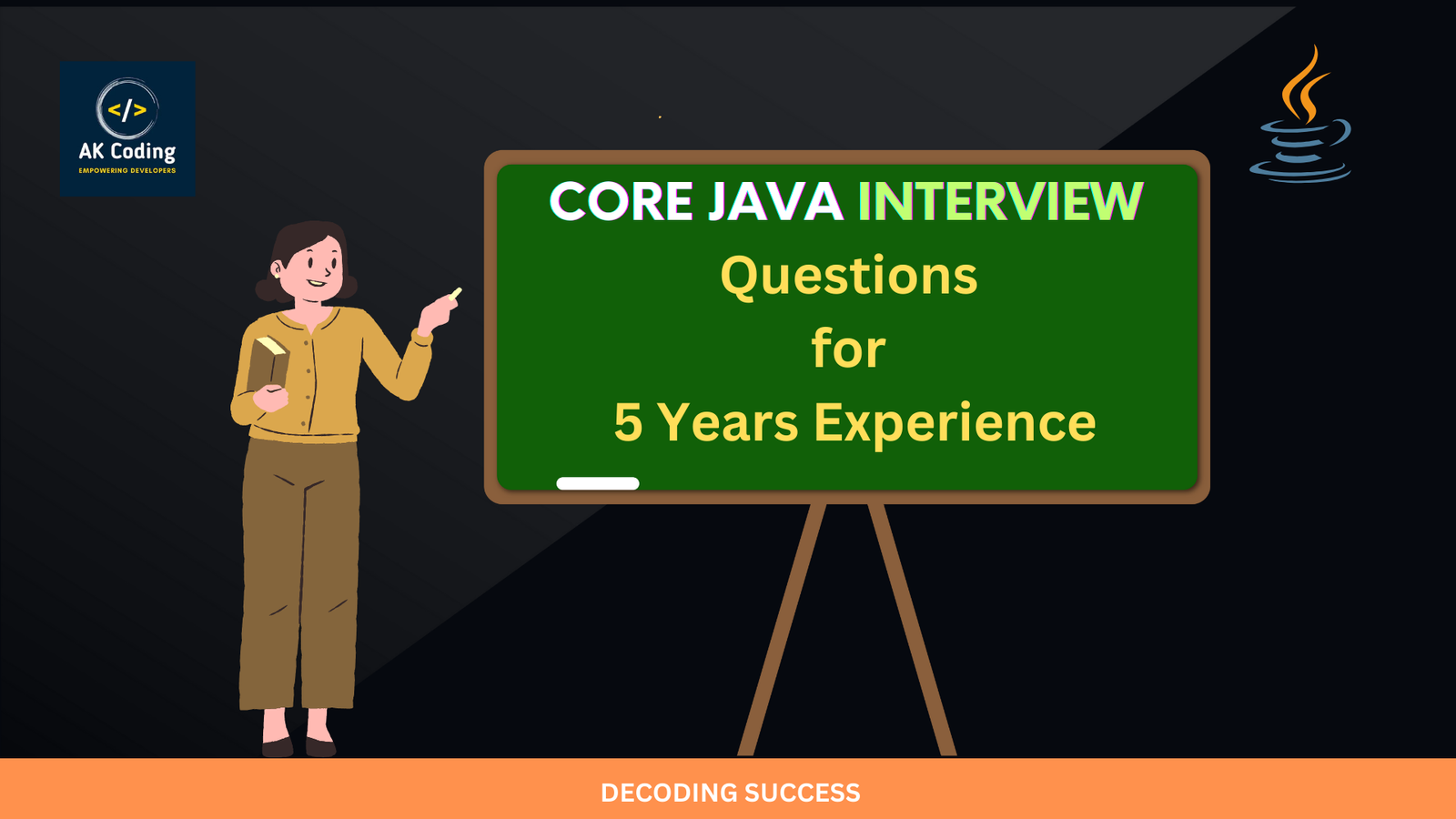
Table of Contents
Here are 20 Core Java Interview Questions for 5 Years Experience:
What is the difference between == and .equals() method in Java?
In Java, both the ==
operator and the .equals()
method are used for comparison, but they serve different purposes and operate differently:
==
Operator:
- The
==
operator is used to compare the references of two objects or variables. - When applied to primitive data types,
==
compares the actual values of the variables. - When applied to objects,
==
compares the memory addresses (references) of the objects, not their contents. - For example:
//Core Java Interview Questions for 5 Years Experience
String str1 = "hello";
String str2 = "hello";
String str3 = new String("hello");
System.out.println(str1 == str2); // true (references are the same) System.out.println(str1 == str3); // false (references are different)
//Core Java Interview Questions for 5 Years Experience
.equals()
Method:
- The
.equals()
method is used to compare the contents or values of two objects. - It is a method defined in the
Object
class and is overridden by most classes to provide meaningful comparison based on the object’s contents. - When using
.equals()
with objects, it compares the actual values stored in the objects, not their memory addresses. - For example:
//Core Java Interview Questions for 5 Years Experience
String str1 = "hello";
String str2 = "hello";
String str3 = new String("hello");
System.out.println(str1.equals(str2)); // true (contents are the same) System.out.println(str1.equals(str3)); // true (contents are the same)
//Core Java Interview Questions for 5 Years Experience
In summary, the ==
operator compares the references (memory addresses) of objects or the values of primitive data types, while the .equals()
method compares the contents (values) of objects. It is important to use the appropriate comparison method based on the desired behavior and context of the comparison.
Explain the concept of multithreading in Java and how it is achieved.
Multithreading in Java refers to the ability of a Java program to execute multiple threads concurrently. A thread is a lightweight subprocess, and multithreading allows multiple threads to execute simultaneously within a single process. Multithreading is achieved in Java through the use of the Thread
class or the Runnable
interface.
Here’s an explanation of the concept of multithreading in Java and how it is achieved:
- Thread Class:
- In Java, multithreading is primarily achieved by extending the
Thread
class or implementing theRunnable
interface. - To create a new thread using the
Thread
class, you can extend it and override therun()
method, which contains the code to be executed by the thread. - For example:
//Core Java Interview Questions for 5 Years Experience
class MyThread extends Thread { public void run() {
// Code to be executed by the thread
System.out.println("Thread running...");
}
}
public class Main {
public static void main(String[] args) {
MyThread thread = new MyThread(); thread.start();
// Start the thread
} }
//Core Java Interview Questions for 5 Years Experience
- Runnable Interface:
- Alternatively, you can implement the
Runnable
interface and pass it to aThread
object to create a new thread. - The
Runnable
interface defines a single method,run()
, which contains the code to be executed by the thread. - For example:
//Core Java Interview Questions for 5 Years Experience
class MyRunnable implements Runnable { public void run() {
// Code to be executed by the thread
System.out.println("Thread running...");
}
}
public class Main { public static void main(String[] args) {
Thread thread = new Thread(new MyRunnable()); thread.start();
// Start the thread
}
}
//Core Java Interview Questions for 5 Years Experience
- Thread Lifecycle:
- Threads in Java go through various states in their lifecycle, including new, runnable, blocked, waiting, timed waiting, and terminated.
- The
start()
method is used to transition a thread from the new state to the runnable state, where it is eligible to run. - The
run()
method contains the actual code executed by the thread. - Once the
run()
method completes execution, or if the thread is explicitly stopped using thestop()
method (deprecated), the thread enters the terminated state.
- Concurrency and Synchronization:
- Multithreading allows multiple threads to execute concurrently, which can lead to issues such as race conditions and thread interference.
- Java provides mechanisms such as synchronization (using
synchronized
keyword) and locks (usingLock
interface) to synchronize access to shared resources and ensure thread safety. - Synchronization ensures that only one thread can access a synchronized block of code or method at a time, preventing concurrent modification and ensuring consistency.
In summary, multithreading in Java allows for concurrent execution of multiple threads within a single process. It is achieved using the Thread
class or the Runnable
interface, and synchronization mechanisms are used to ensure thread safety and prevent issues such as race conditions.
What is the difference between checked and unchecked exceptions in Java? Can you provide examples of each?
In Java, exceptions are categorized into two types: checked exceptions and unchecked exceptions. Here’s the difference between them along with examples of each:
- Checked Exceptions:
- Checked exceptions are exceptions that are checked at compile-time. This means that the compiler ensures that code handles or declares these exceptions.
- Checked exceptions are subclasses of the
Exception
class, excluding subclasses ofRuntimeException
and its subclasses. - Examples of checked exceptions include:
IOException
: This exception is thrown when an input or output operation fails, such as when working with files or streams.SQLException
: This exception is thrown when an error occurs while accessing a database using JDBC (Java Database Connectivity).ClassNotFoundException
: This exception is thrown when an application tries to load a class but the class cannot be found at runtime.
//Core Java Interview Questions for 5 Years Experience
import java.io.*; public
class Main {
public static void main(String[] args) {
try {
BufferedReader reader = new BufferedReader(new FileReader("example.txt"));
String line = reader.readLine();
System.out.println(line); reader.close();
} catch (IOException e)
{
System.out.println("An error occurred while reading the file.");
e.printStackTrace();
}
}
}
//Core Java Interview Questions for 5 Years Experience
- Unchecked Exceptions:
- Unchecked exceptions, also known as runtime exceptions, are exceptions that are not checked at compile-time. These exceptions can occur at runtime, and the compiler does not enforce the handling or declaration of these exceptions.
- Unchecked exceptions are subclasses of the
RuntimeException
class and its subclasses. - Examples of unchecked exceptions include:
NullPointerException
: This exception is thrown when attempting to access or invoke a method on a null object reference.ArrayIndexOutOfBoundsException
: This exception is thrown when trying to access an array element at an index that is outside the bounds of the array.ArithmeticException
: This exception is thrown when an arithmetic operation encounters an exceptional condition, such as division by zero.
//Core Java Interview Questions for 5 Years Experience
public class Main { public static void main(String[] args) { int[] numbers = {1, 2, 3}; System.out.println(numbers[4]);
// ArrayIndexOutOfBoundsException
} }
//Core Java Interview Questions for 5 Years Experience
In summary, the main difference between checked and unchecked exceptions lies in when they are checked by the compiler. Checked exceptions are checked at compile-time and must be handled or declared, whereas unchecked exceptions are not checked at compile-time and can occur at runtime without being explicitly handled.
What are the different types of inheritance in Java? Explain them with examples.
In Java, inheritance is a mechanism where a new class (subclass or derived class) inherits properties and behaviors (fields and methods) from an existing class (superclass or base class). There are different types of inheritance in Java, including single inheritance, multilevel inheritance, hierarchical inheritance, and multiple inheritance (through interfaces). Let’s explain each type with examples:
- Single Inheritance:
- Single inheritance refers to the inheritance relationship where a subclass extends only one superclass.
- Example:
//Core Java Interview Questions for 5 Years Experience
// Superclass
class Animal {
void eat()
{ System.out.println("Animal is eating");
} }
// Subclass inheriting from Animal
class Dog extends Animal {
void bark() {
System.out.println("Dog is barking");
}
}
// Main class public class Main
{
public static void main(String[] args)
{ Dog dog = new Dog(); dog.eat();
// Inherits from Animal class dog.bark(); // Inherits from Dog class
} }
//Core Java Interview Questions for 5 Years Experience
- Multilevel Inheritance:
- Multilevel inheritance refers to the inheritance relationship where a subclass extends another subclass, creating a chain of inheritance.
- Example:
//Core Java Interview Questions for 5 Years Experience
// Superclass
class Animal {
void eat() { System.out.println("Animal is eating");
} }
// Subclass inheriting from Animal
class Dog extends Animal {
void bark() { System.out.println("Dog is barking");
} }
// Subclass inheriting from Dog
class Labrador extends Dog {
void play() { System.out.println("Labrador is playing");
} }
// Main class public
class Main {
public static void main(String[] args) { Labrador labrador = new Labrador(); labrador.eat();
// Inherits from Animal class labrador.bark();
// Inherits from Dog class labrador.play();
// Inherits from Labrador class
} }
//Core Java Interview Questions for 5 Years Experience
- Hierarchical Inheritance:
- Hierarchical inheritance refers to the inheritance relationship where multiple subclasses inherit from the same superclass.
- Example:
//Core Java Interview Questions for 5 Years Experience
// Superclass
class Animal { void eat() { System.out.println("Animal is eating");
} }
// Subclass inheriting from
Animal class Dog extends Animal { void bark() { System.out.println("Dog is barking"); } }
// Subclass inheriting from
Animal class Cat extends Animal
{ void meow() {
System.out.println("Cat is meowing");
} }
// Main class public
class Main {
public static void main(String[] args) {
Dog dog = new Dog(); dog.eat(); /
/ Inherits from Animal class dog.bark();
// Inherits from Dog class
Cat cat = new Cat(); cat.eat();
// Inherits from Animal class cat.meow();
// Inherits from Cat class
} }
//Core Java Interview Questions for 5 Years Experience
- Multiple Inheritance (Through Interfaces):
- Java does not support multiple inheritance of classes, where a subclass inherits from multiple superclasses. However, it supports multiple inheritance through interfaces, where a class can implement multiple interfaces.
- Example:
//Core Java Interview Questions for 5 Years Experience
// Interface 1
interface Walkable { void walk();
}
// Interface 2
interface Swimmable {
void swim();
}
//
Class implementing both interfaces class Duck implements Walkable, Swimmable { public void walk() { System.out.println("Duck is walking");
}
public void swim() {
System.out.println("Duck is swimming");
} }
// Main class
public class Main {
public static void main(String[] args) {
Duck duck = new Duck(); duck.walk();
// Implements
Walkable interface duck.swim();
// Implements Swimmable interface
} }
//Core Java Interview Questions for 5 Years Experience
In summary, Java supports various types of inheritance, including single, multilevel, and hierarchical inheritance. While Java does not support multiple inheritance of classes, it supports multiple inheritance through interfaces, allowing classes to implement multiple interfaces.
What is the purpose of the static keyword in Java? How is it used?
In Java, the static
keyword is used to declare members (fields and methods) that belong to the class itself rather than to instances of the class (objects). When a member is declared as static
, it is associated with the class rather than with any specific instance of the class. Here’s the purpose of the static
keyword and how it is used:
- Purpose of the
static
Keyword:
- Shared Data:
static
members are shared among all instances of the class. They are initialized only once, and their values are shared across all objects of the class. - Class-Level Behavior:
static
methods can be called directly on the class itself without the need to create an instance of the class. They are often used for utility methods or operations that do not require access to instance-specific data. - Memory Efficiency:
static
members are allocated memory only once, reducing memory consumption when multiple instances of the class are created. - Constants:
static final
variables are often used to define constants that remain constant throughout the lifetime of the program.
- Usage of the
static
Keyword:
- Static Fields (Class Variables): Use the
static
keyword to declare fields that are shared among all instances of the class. These fields are accessed using the class name, followed by the dot operator (ClassName.fieldName
).
//Core Java Interview Questions for 5 Years Experience
class MyClass {
static int count = 0;
// Static field
}
//Core Java Interview Questions for 5 Years Experience
- Static Methods (Class Methods): Use the
static
keyword to declare methods that can be invoked directly on the class without the need for an instance. Static methods cannot access instance variables directly (unless they are passed as parameters), but they can access other static members.
//Core Java Interview Questions for 5 Years Experience
class MyClass { static void printMessage() {
// Static method System.out.println("Hello, World!");
} }
//Core Java Interview Questions for 5 Years Experience
- Static Initialization Blocks: Use static initialization blocks to initialize static variables or perform any one-time initialization tasks for the class. Static initialization blocks are executed once when the class is loaded into memory.
//Core Java Interview Questions for 5 Years Experience
class MyClass {
static {
// Static initialization block
// Initialization code goes here
} }
//Core Java Interview Questions for 5 Years Experience
class MyClass {
static {
// Static initialization block
// Initialization code goes here
} }
//Core Java Interview Questions for 5 Years Experience
- Static Nested Classes: Use the
static
keyword to define static nested classes, which are nested classes that do not have access to instance members of the enclosing class. Static nested classes are often used for grouping related utility classes or helper functions.
//Core Java Interview Questions for 5 Years Experience
class OuterClass {
static class NestedClass
{
// Static nested class // Class members go here
} }
//Core Java Interview Questions for 5 Years Experience
In summary, the static
keyword in Java is used to declare members that are associated with the class itself rather than with instances of the class. It is commonly used for defining shared data, class-level behavior, constants, and utility methods. Understanding the purpose and usage of the static
keyword is essential for writing efficient and maintainable Java code.
Explain the use of the final keyword in Java. When and where would you use it?
In Java, the final
keyword is used to define constants, restrict inheritance, and prevent method overriding. It can be applied to variables, methods, and classes, providing various benefits such as immutability, code safety, and design clarity. Here’s a detailed explanation of the use of the final
keyword and scenarios where it would be appropriate to use:
- Final Variables:
- When applied to variables, the
final
keyword indicates that the variable’s value cannot be changed once initialized. It effectively makes the variable constant. - Example:
//Core Java Interview Questions for 5 Years Experience
final int MAX_SIZE = 100;
//Core Java Interview Questions for 5 Years Experience
- Use
final
variables to define constants that are not expected to change throughout the program’s execution. Constants provide readability, maintainability, and prevent accidental modifications to important values.
- Final Methods:
- When applied to methods, the
final
keyword indicates that the method cannot be overridden by subclasses. It enforces the method’s behavior to remain unchanged. - Example:
//Core Java Interview Questions for 5 Years Experience
class Parent {
final void display() { System.out.println("Displaying from Parent class");
} }
class Child extends Parent {
// Error: Cannot override final method
// void display() { ... }
}
//Core Java Interview Questions for 5 Years Experience
- Use
final
methods to prevent subclasses from altering the behavior of critical methods, ensuring consistency and stability in the class hierarchy.
- Final Classes:
- When applied to classes, the
final
keyword indicates that the class cannot be subclassed. It prevents inheritance and ensures that the class’s implementation remains unchanged. - Example:
//Core Java Interview Questions for 5 Years Experience
final class MyFinalClass {
// Class members go here
}
// Error: Cannot subclass final class // class Subclass extends MyFinalClass { ... }
//Core Java Interview Questions for 5 Years Experience
- Use
final
classes to prevent extension and inheritance, particularly for utility classes, immutable classes, or classes with sensitive implementations that should not be modified or extended.
- Final Parameters:
- When applied to method parameters, the
final
keyword indicates that the parameter’s value cannot be modified within the method body. - Example:
//Core Java Interview Questions for 5 Years Experience
void process(final int value) {
// Error: Cannot modify final parameter
// value = 10;
System.out.println("Value: " + value);
}
//Core Java Interview Questions for 5 Years Experience
- Use
final
parameters to enforce immutability and prevent accidental modification of method arguments, enhancing code clarity and preventing unintended side effects.
In summary, the final
keyword in Java is used to define constants, prevent method overriding, restrict inheritance, and enforce immutability. It enhances code safety, readability, and maintainability by clearly expressing the intent and constraints of variables, methods, and classes. Use final
appropriately in scenarios where immutability, stability, and consistency are desired.
What are the differences between abstract classes and interfaces in Java? When would you use each?
Abstract classes and interfaces are both used to define contracts for classes in Java, but they have distinct differences in their implementation and usage. Here are the key differences between abstract classes and interfaces, along with scenarios for when to use each:
- Definition:
- Abstract Class:
- An abstract class is a class that cannot be instantiated and may contain abstract methods, concrete methods, and fields.
- Abstract methods are declared without implementation and must be overridden by subclasses.
- Interface:
- An interface is a reference type in Java that contains only abstract methods, constants (static final fields), and default methods (with implementation).
- Interfaces cannot contain fields other than constants and cannot be instantiated on their own.
- Multiple Inheritance:
- Abstract Class:
- A Java class can extend only one abstract class (single inheritance).
- Interface:
- A Java class can implement multiple interfaces (multiple inheritance).
- Constructor:
- Abstract Class:
- An abstract class can have constructors, which are invoked when a subclass is instantiated.
- Interface:
- Interfaces cannot have constructors because they cannot be instantiated directly.
- Method Implementation:
- Abstract Class:
- An abstract class can have both abstract methods (without implementation) and concrete methods (with implementation).
- Subclasses can inherit concrete methods from the abstract class.
- Interface:
- All methods in an interface are implicitly abstract and have no method bodies.
- Default methods introduced in Java 8 provide a way to define method implementations in interfaces.
- Usage:
- Abstract Class:
- Use an abstract class when you want to provide a common base implementation for subclasses and when you want to define fields or concrete methods that subclasses can inherit.
- Abstract classes are useful for creating a hierarchy of related classes with shared behavior.
- Interface:
- Use an interface when you want to define a contract for classes that are not related by inheritance but share common behavior.
- Interfaces are useful for defining APIs (Application Programming Interfaces) and for achieving loose coupling between components in a system.
- Interfaces are also useful for achieving multiple inheritance in Java when a class needs to inherit behavior from multiple sources.
- Evolution:
- Abstract Class:
- Adding new methods to an abstract class may break existing subclasses that have not implemented the new methods.
- Interface:
- Adding new methods to an interface does not break existing classes that implement the interface, as they are not required to implement the new methods (unless default methods are used).
In summary, abstract classes and interfaces serve different purposes in Java. Use abstract classes when you want to provide a common base implementation for related subclasses and use interfaces when you want to define a contract for unrelated classes or achieve multiple inheritance. Choose the appropriate mechanism based on the specific requirements and design considerations of your application.
How does Java handle memory management and garbage collection? Explain the concept of garbage collection in Java.
Java manages memory dynamically using a combination of automatic memory management and garbage collection. Garbage collection is the process by which Java identifies and removes objects that are no longer in use, reclaiming their memory and making it available for future allocations. Here’s an explanation of how Java handles memory management and garbage collection:
- Automatic Memory Management:
- In Java, memory allocation and deallocation are handled automatically by the Java Virtual Machine (JVM).
- When you create an object using the
new
keyword, memory is allocated for the object on the heap. - The JVM automatically deallocates memory for objects that are no longer referenced or reachable by the application.
- Garbage Collection Process:
- Garbage collection is the process of reclaiming memory occupied by objects that are no longer in use or reachable by the application.
- The Java garbage collector runs periodically in the background to identify and remove unreferenced objects.
- The garbage collector uses the concept of reachability to determine which objects are eligible for garbage collection. An object is considered reachable if it can be accessed directly or indirectly from the application’s root references (e.g., local variables, static variables, method parameters, etc.).
- Objects that are not reachable from any root reference are considered garbage and are eligible for collection.
- Garbage Collection Algorithms:
- Java provides different garbage collection algorithms, each with its own strengths and weaknesses, catering to different application requirements and performance goals.
- Some common garbage collection algorithms in Java include:
- Mark and Sweep: This algorithm marks reachable objects and sweeps away unreachable objects.
- Generational Garbage Collection: This algorithm divides the heap into multiple generations (young generation, old generation) and applies different garbage collection strategies to each generation.
- Parallel Garbage Collection: This algorithm uses multiple threads to perform garbage collection concurrently, reducing pause times and improving throughput.
- G1 Garbage Collector: Introduced in Java 7, the Garbage-First (G1) garbage collector is designed to provide predictable garbage collection pause times and efficient heap utilization.
- Tuning Garbage Collection:
- Garbage collection behavior can be tuned and configured using JVM command-line options and garbage collection settings.
- Parameters such as heap size, garbage collection algorithm, and garbage collection pause time goals can be adjusted based on the application’s memory usage patterns and performance requirements.
In summary, Java handles memory management and garbage collection automatically, relieving developers from the burden of manual memory management. Garbage collection in Java is a sophisticated process that identifies and removes unreferenced objects, reclaiming memory and preventing memory leaks. By periodically running the garbage collector and employing various garbage collection algorithms, Java ensures efficient memory utilization and helps maintain application performance and stability.
What is the difference between ArrayList and LinkedList in Java? When would you use each?
ArrayList
and LinkedList
are both implementations of the List
interface in Java, but they have different underlying data structures and performance characteristics. Here’s a comparison of ArrayList
and LinkedList
along with scenarios for when to use each:
- Underlying Data Structure:
ArrayList
: Internally backed by a dynamic array, which allows for fast random access to elements using index-based operations (get(int index)
andset(int index, E element)
).LinkedList
: Implemented as a doubly linked list, where each element is stored as a node containing a reference to the previous and next elements. Random access is slower compared toArrayList
, as it requires traversing the list from the beginning or end.
- Insertion and Deletion Performance:
ArrayList
: Insertion and deletion operations (add(E element)
andremove(int index)
) at the end of the list are efficient, as they require resizing the underlying array only occasionally (amortized constant time). However, insertion and deletion operations at the beginning or middle of the list may be inefficient, as they require shifting elements to accommodate the change (linear time complexity).LinkedList
: Insertion and deletion operations are efficient for both ends of the list (head and tail) because they involve updating references of adjacent nodes (constant time complexity). However, insertion and deletion operations in the middle of the list may be slower compared toArrayList
, as they require traversing the list to locate the insertion or deletion point (linear time complexity).
- Memory Overhead:
ArrayList
: Consumes less memory per element compared toLinkedList
, as it only stores the elements and maintains an internal array.LinkedList
: Consumes more memory per element compared toArrayList
, as it stores additional memory overhead for maintaining node references.
- Random Access vs. Sequential Access:
ArrayList
: Suitable for scenarios that require frequent random access to elements, such as retrieving elements by index or iterating over elements sequentially.LinkedList
: Suitable for scenarios that require frequent insertion and deletion operations at both ends of the list, such as implementing a queue or deque (double-ended queue).
- Performance Considerations:
ArrayList
: Preferred when the application requires frequent random access and modification of elements, or when memory efficiency is a concern.LinkedList
: Preferred when the application requires frequent insertion and deletion operations at both ends of the list, or when the list size is expected to change dynamically and frequently.
In summary, ArrayList
and LinkedList
have different performance characteristics and usage scenarios. Use ArrayList
when the application requires frequent random access to elements or when memory efficiency is important. Use LinkedList
when the application requires frequent insertion and deletion operations at both ends of the list or when the list size is expected to change dynamically.
Explain the concept of serialization and deserialization in Java. How can objects be serialized?
Serialization in Java refers to the process of converting objects into a stream of bytes, which can be persisted to a file, transmitted over a network, or stored in a database. Deserialization, on the other hand, is the process of reconstructing objects from the serialized byte stream. Serialization is commonly used for data persistence, communication between distributed systems, and caching.
Here’s an explanation of serialization and deserialization in Java:
- Serialization:
- Serialization allows objects to be converted into a byte stream, which can be written to a file or transmitted over a network.
- In Java, the
java.io.Serializable
interface is used to mark classes as serializable. Classes that implement this interface indicate that their instances can be serialized. - The serialization process involves converting the state of an object (its fields and their values) into a sequence of bytes. This byte stream contains the object’s data along with metadata to identify the object’s class and version.
- The
java.io.ObjectOutputStream
class is used to serialize objects in Java. This class provides methods for writing objects to an output stream. - Example of serialization:
//Core Java Interview Questions for 5 Years Experience
import java.io.*; public class SerializationDemo {
public static void main(String[] args) {
try {
// Create an object to serialize
MyClass obj = new MyClass();
// Serialize the object
FileOutputStream fileOut = new FileOutputStream("object.ser"); ObjectOutputStream out = new ObjectOutputStream(fileOut); out.writeObject(obj); out.close(); fileOut.close(); System.out.println("Object serialized successfully."); } catch (IOException e) { e.printStackTrace(); } } }
//Core Java Interview Questions for 5 Years Experience
- Deserialization:
- Deserialization is the process of reconstructing objects from a byte stream, restoring their state to the state they were in when serialized.
- In Java, the
java.io.ObjectInputStream
class is used to deserialize objects. This class provides methods for reading objects from an input stream. - During deserialization, the byte stream is read and converted back into objects, using the metadata stored in the stream to identify the object’s class and version.
- Example of deserialization:
//Core Java Interview Questions for 5 Years Experience
import java.io.*; public class DeserializationDemo { public static void main(String[] args) { try { // Deserialize the object
FileInputStream fileIn = new FileInputStream("object.ser"); ObjectInputStream in = new ObjectInputStream(fileIn); MyClass obj = (MyClass) in.readObject(); in.close(); fileIn.close(); System.out.println("Object deserialized successfully."); } catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
} }
//Core Java Interview Questions for 5 Years Experience
- Transient Fields:
- Fields marked as
transient
are not serialized by default. This can be useful for excluding sensitive or non-serializable data from the serialization process. - Example of transient field:
//Core Java Interview Questions for 5 Years Experience
import java.io.*; class MyClass implements Serializable
{
private transient String sensitiveData;
// Marked as transient
// Other fields and methods
}
//Core Java Interview Questions for 5 Years Experience
Serialization and deserialization in Java provide a powerful mechanism for persisting object data and transferring objects between different parts of an application or across a network. However, it’s essential to handle serialization and deserialization carefully, considering factors such as backward compatibility, security, and performance.
What is the purpose of the transient keyword in Java?
In Java, the transient
keyword is used to indicate that a field should not be serialized when the object containing it is serialized. When an object is serialized, all of its non-transient fields are converted into a byte stream, but transient fields are excluded from this process. This can be useful for excluding sensitive or non-serializable data from the serialization process, or for fields that are derived transiently and do not need to be persisted.
The main purpose of the transient
keyword in Java is to provide control over the serialization process, allowing developers to specify which fields should not be included in the serialized form of an object.
Here are some common use cases for the transient
keyword:
- Excluding Sensitive Data: Fields containing sensitive information, such as passwords, encryption keys, or temporary data, can be marked as transient to prevent them from being persisted in the serialized object. This enhances security by ensuring that sensitive information does not get stored in a potentially insecure location.
- Excluding Non-Serializable Data: Fields that reference non-serializable objects or resources, such as file handles, database connections, or network sockets, can be marked as transient to prevent serialization errors. Since these objects cannot be serialized, marking them as transient ensures that they are not included in the serialized object.
- Optimizing Serialization: Fields that are derived transiently or can be recomputed easily may not need to be serialized. By marking these fields as transient, developers can optimize the serialization process by excluding unnecessary data, reducing the size of the serialized object and improving performance.
Here’s an example of using the transient
keyword in Java:import java.io.Serializable; class MyClass implements Serializable { private transient String sensitiveData; // Marked as transient private int id; private String name; // Constructor, getters, setters }
In this example, the sensitiveData
field is marked as transient to ensure that it is not included in the serialized form of the MyClass
objects. Only the id
and name
fields will be serialized when instances of MyClass
are serialized. This helps protect sensitive information and optimize the serialization process.
Explain the concept of polymorphism in Java with examples.
Polymorphism in Java is the ability of a single entity, such as a method or class, to take on multiple forms. In Java, polymorphism is achieved through method overriding and method overloading, as well as through inheritance and interface implementations. Polymorphism allows objects of different classes to be treated as objects of a common superclass or interface, providing flexibility and extensibility in object-oriented programming. Here’s an explanation of polymorphism in Java with examples:
- Method Overriding:
- Method overriding occurs when a subclass provides a specific implementation of a method that is already defined in its superclass.
- The overridden method in the subclass has the same signature (name, return type, and parameters) as the method in the superclass.
- At runtime, the JVM determines which version of the method to invoke based on the actual type of the object.
- Example:
//Core Java Interview Questions for 5 Years Experience
class Animal { void makeSound() {
System.out.println("Animal makes a sound");
} }
class Dog extends Animal {
@Override void makeSound() {
System.out.println("Dog barks");
} }
public class Main {
public static void main(String[] args) {
Animal animal = new Dog();
// Upcasting animal.makeSound();
// Output: Dog barks
} }
//Core Java Interview Questions for 5 Years Experience
- Method Overloading:
- Method overloading occurs when multiple methods with the same name but different parameter lists are defined within the same class.
- Overloaded methods have different signatures, allowing them to accept different types or numbers of parameters.
- The appropriate version of the method to invoke is determined by the number and types of arguments passed during method invocation.
- Example:
//Core Java Interview Questions for 5 Years Experience
class Calculator {
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
} }
public class Main {
public static void main(String[] args) {
Calculator calc = new Calculator();
System.out.println(calc.add(5, 3));
// Output: 8 System.out.println(calc.add(2.5, 3.5));
// Output: 6.0
} }
//Core Java Interview Questions for 5 Years Experience
- Polymorphism with Inheritance:
- Polymorphism allows objects of subclasses to be treated as objects of their superclass.
- This allows for more generic and flexible code, where methods or classes can operate on objects of a common superclass without needing to know the specific subclass.
- Example:
//Core Java Interview Questions for 5 Years Experience
class Shape {
void draw() {
System.out.println("Drawing a shape");
} }
class Circle extends Shape {
@Override void draw() {
System.out.println("Drawing a circle");
} }
public class Main {
public static void main(String[] args) {
Shape shape = new Circle();
// Upcasting shape.draw();
// Output: Drawing a circle
} }
//Core Java Interview Questions for 5 Years Experience
- Polymorphism with Interfaces:
- Interfaces in Java can be used to achieve polymorphism by allowing objects of different classes to be treated uniformly based on their common interface.
- Classes that implement the same interface can be used interchangeably, allowing for flexibility in code design and implementation.
- Example:
//Core Java Interview Questions for 5 Years Experience
interface Printable {
void print();
}
class Document implements Printable {
@Override public void print() {
System.out.println("Printing a document");
} }
class Image implements Printable {
@Override public void print() {
System.out.println("Printing an image");
} }
public class Main {
public static void main(String[] args) {
Printable printable1 = new Document();
Printable printable2 = new Image(); printable1.print();
// Output: Printing a document printable2.print();
// Output: Printing an image
} }
//Core Java Interview Questions for 5 Years Experience
In summary, polymorphism in Java allows for code reuse, flexibility, and extensibility by enabling objects of different classes to be treated uniformly based on their common superclass or interface. This concept is essential in object-oriented programming and promotes the development of modular, maintainable, and scalable software systems.
What are the different types of design patterns in Java? Can you provide examples of some commonly used design patterns?
Design patterns are reusable solutions to common software design problems that have been identified and documented by experienced software developers. In Java, design patterns are categorized into three main types based on their purpose: creational, structural, and behavioral patterns. Here’s an overview of each type along with examples of commonly used design patterns in Java:
- Creational Patterns:
- Creational patterns focus on object creation mechanisms, abstracting the instantiation process to make the system more flexible and decoupled from the specific classes being instantiated.
- Examples of creational patterns include:
- Singleton Pattern: Ensures that a class has only one instance and provides a global point of access to that instance.
- Factory Method Pattern: Defines an interface for creating objects but allows subclasses to alter the type of objects that will be created.
- Abstract Factory Pattern: Provides an interface for creating families of related or dependent objects without specifying their concrete classes.
- Structural Patterns:
- Structural patterns deal with object composition and provide ways to compose objects into larger structures while keeping these structures flexible and efficient.
- Examples of structural patterns include:
- Adapter Pattern: Allows incompatible interfaces to work together by providing a wrapper (adapter) that converts the interface of one class into another interface expected by the client.
- Decorator Pattern: Adds new functionalities to objects dynamically by wrapping them with decorator objects, without altering their structure.
- Facade Pattern: Provides a unified interface to a set of interfaces in a subsystem, simplifying complex systems by providing a higher-level interface.
- Behavioral Patterns:
- Behavioral patterns focus on communication between objects, defining how objects interact and collaborate with each other to accomplish complex tasks.
- Examples of behavioral patterns include:
- Observer Pattern: Defines a one-to-many dependency between objects, so that when one object changes state, all its dependents are notified and updated automatically.
- Strategy Pattern: Defines a family of algorithms, encapsulates each algorithm, and makes them interchangeable. Clients can choose which algorithm to use dynamically.
- Command Pattern: Encapsulates a request as an object, allowing clients to parameterize and queue requests, as well as support undoable operations.
These are just a few examples of the many design patterns available in Java. Each design pattern addresses a specific problem and provides a proven solution that can be adapted and applied to various software design scenarios. By understanding and using design patterns effectively, developers can improve code maintainability, flexibility, and scalability, leading to better software quality and productivity.
How does exception handling work in Java? Explain the try-catch-finally block.
Exception handling in Java is a mechanism that allows developers to manage and respond to runtime errors, known as exceptions, in a controlled and graceful manner. Exceptions are objects representing abnormal conditions that occur during the execution of a program, such as division by zero, array index out of bounds, or file not found.
The try-catch-finally
block is a fundamental construct in Java for handling exceptions. It allows developers to write code that may potentially throw exceptions and handle those exceptions gracefully. Here’s an explanation of how the try-catch-finally
block works in Java:
try
Block:
- The
try
block encloses the code that may potentially throw exceptions. It contains the statements that need to be monitored for exceptions. - When an exception occurs within the
try
block, the execution of the block is terminated, and the control is transferred to the nearestcatch
block (if available).
catch
Block:
- The
catch
block follows thetry
block and provides a mechanism to catch and handle specific types of exceptions. - Each
catch
block specifies the type of exception it can handle using thecatch
keyword followed by the exception type in parentheses. - When an exception of the specified type occurs within the
try
block, the control is transferred to the correspondingcatch
block, and the exception is caught and handled within that block.
finally
Block:
- The
finally
block, if present, follows thecatch
block (or thetry
block if nocatch
block is present) and is used to execute cleanup code regardless of whether an exception occurred or not. - The
finally
block is guaranteed to execute, even if an exception is thrown or caught. It ensures that resources are released and cleanup operations are performed, such as closing files or releasing locks. - The
finally
block is optional and can be omitted if cleanup operations are not required.
Here’s an example of using the try-catch-finally
block in Java:
//Core Java Interview Questions for 5 Years Experience
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
FileInputStream fileInputStream = null;
try {
// Open a file for reading
fileInputStream = new FileInputStream("example.txt");
// Perform read operations
// ...
} catch (FileNotFoundException e) {
// Handle file not found exception System.err.println("File not found: " + e.getMessage());
} catch (IOException e) {
// Handle IO exception System.err.println("IO exception: " + e.getMessage());
} finally {
// Close the file stream in the finally block to ensure it is always closed
if (fileInputStream != null) { try {
fileInputStream.close();
}
catch (IOException e) {
System.err.println("Error closing file: " + e.getMessage());
} } }
} }
//Core Java Interview Questions for 5 Years Experience
In this example:
- The
try
block attempts to open a file for reading. - If the file is not found (
FileNotFoundException
), the control is transferred to the correspondingcatch
block. - If an IO exception occurs during the read operation (
IOException
), the control is transferred to the correspondingcatch
block. - The
finally
block ensures that the file stream is closed, regardless of whether an exception occurred or not.
Overall, the try-catch-finally
block provides a structured and robust approach to handle exceptions in Java, ensuring that errors are gracefully handled and resources are properly managed.
What are lambda expressions in Java? How are they used, and what are their advantages?
Lambda expressions, introduced in Java 8, are a powerful feature that allows developers to treat functionality as a method argument, or to create concise and expressive anonymous functions. Lambda expressions enable functional programming capabilities in Java by providing a way to express instances of single-method interfaces (functional interfaces) more compactly.
Here’s an explanation of lambda expressions in Java, how they are used, and their advantages:
- Syntax of Lambda Expressions:
- Lambda expressions consist of parameters, an arrow (
->
), and a body. - The parameters represent the input arguments to the method.
- The arrow separates the parameters from the body of the lambda expression.
- The body represents the implementation of the method. Syntax:
(parameters) -> expression
or (parameters) -> { statements; }
- Using Lambda Expressions:
- Lambda expressions are typically used in contexts where functional interfaces are expected, such as in the implementation of interfaces with a single abstract method (SAM types).
- They can be used to replace anonymous inner classes with more concise and readable code.
- Lambda expressions can be passed as arguments to methods, assigned to variables, or used as return values from methods.
- Advantages of Lambda Expressions:
- Conciseness: Lambda expressions allow for more concise and readable code compared to anonymous inner classes, reducing boilerplate code and improving code clarity.
- Flexibility: They enable developers to express behavior directly inline with the code, making it easier to write functional-style code.
- Readability: Lambda expressions can make code more readable by expressing the intention of the code more clearly, especially when dealing with higher-order functions and functional programming constructs.
- Functional Programming: Lambda expressions facilitate functional programming paradigms in Java, allowing developers to use functional interfaces, streams, and other functional programming features more effectively.
Here’s an example demonstrating the use of lambda expressions in Java:
//Core Java Interview Questions for 5 Years Experience
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<String> names = new ArrayList<>();
names.add("Alice"); names.add("Bob");
names.add("Charlie");
// Using lambda expression to iterate and print each element of the list
names.forEach(name -> System.out.println(name));
// Using lambda expression to filter elements based on a condition
names.removeIf(name -> name.startsWith("A"));
// Using lambda expression as a method reference
names.forEach(System.out::println);
} }
//Core Java Interview Questions for 5 Years Experience
In this example:
- The
forEach
method of theList
interface is used with a lambda expression to iterate over each element of the list and print it. - The
removeIf
method is used with a lambda expression to remove elements from the list that start with the letter “A”. - A lambda expression is used as a method reference to print each element of the modified list.
Lambda expressions provide a concise and expressive way to represent functionality in Java, enabling developers to write more efficient, readable, and maintainable code. They are a fundamental feature of modern Java programming and are widely used in functional programming, concurrent programming, and stream processing.
What is the difference between HashSet and TreeSet in Java? When would you use each?
HashSet
and TreeSet
are both implementations of the Set
interface in Java, but they have different underlying data structures and performance characteristics. Here’s a comparison of HashSet
and TreeSet
along with scenarios for when to use each:
- Underlying Data Structure:
HashSet
: Implemented using a hash table or hash map data structure, which provides constant-time performance for basic operations such as add, remove, and contains.TreeSet
: Implemented using a self-balancing binary search tree (Red-Black Tree), which maintains elements in sorted order according to their natural ordering or a custom comparator. The tree structure provides logarithmic-time performance for basic operations.
- Ordering:
HashSet
: Does not guarantee any specific order of elements. The iteration order of elements may change over time, depending on the internal implementation and the hash codes of elements.TreeSet
: Maintains elements in sorted order (ascending order by default), allowing for efficient retrieval of elements in sorted sequence. The iteration order of elements is based on the natural ordering of elements or a custom comparator provided during TreeSet creation.
- Performance:
HashSet
: Provides constant-time performance (O(1)) for basic operations such as add, remove, and contains, on average. However, the performance may degrade to linear-time (O(n)) in the worst case scenario due to hash collisions.TreeSet
: Provides logarithmic-time performance (O(log n)) for basic operations such as add, remove, and contains, as the elements are stored in a balanced binary search tree. The performance remains consistent even in the worst-case scenario.
- Duplicates:
HashSet
: Does not allow duplicate elements. Adding duplicate elements to a HashSet has no effect.TreeSet
: Does not allow duplicate elements. Adding duplicate elements to a TreeSet has no effect.
- Use Cases:
HashSet
: Suitable for scenarios where fast insertion, deletion, and lookup operations are required, and the order of elements is not important. It is commonly used for implementing sets, hash-based collections, and checking for element existence.TreeSet
: Suitable for scenarios where elements need to be stored in sorted order and efficient retrieval of elements in sorted sequence is required. It is commonly used for maintaining sorted sets, implementing ordered collections, and performing range queries.
- Memory Overhead:
HashSet
: Typically consumes less memory overhead compared to TreeSet, as it does not require additional memory for maintaining sorted order or balancing the tree structure.TreeSet
: Consumes more memory overhead compared to HashSet, as it maintains elements in a sorted order using a binary search tree, which requires additional memory for node pointers and balancing operations.
In summary, HashSet
and TreeSet
have different performance characteristics and usage scenarios. Use HashSet
when fast insertion, deletion, and lookup operations are required, and the order of elements is not important. Use TreeSet
when elements need to be stored in sorted order, and efficient retrieval of elements in sorted sequence is required. Choose the appropriate implementation based on the specific requirements and performance considerations of your application.
Explain the concept of synchronization in Java. How can synchronization be achieved?
In Java, synchronization is the process of controlling access to shared resources or critical sections of code in a multi-threaded environment to prevent data races, race conditions, and other concurrency issues. Synchronization ensures that only one thread can access a shared resource at a time, thereby maintaining data consistency and avoiding unexpected behavior caused by concurrent access.
Concurrency issues can arise when multiple threads attempt to access and modify shared data simultaneously, leading to unpredictable outcomes such as data corruption, inconsistency, or deadlock. Synchronization mechanisms in Java help mitigate these issues by enforcing mutual exclusion, ensuring that critical sections of code are executed atomically by only one thread at a time.
Synchronization can be achieved in Java using the following mechanisms:
- Synchronized Methods:
- In Java, methods can be declared as synchronized to ensure that only one thread can execute them at a time.
- When a synchronized method is called, the thread acquires the intrinsic lock (also known as monitor lock or mutex) associated with the object on which the method is invoked. Other threads attempting to call synchronized methods on the same object are blocked until the lock is released.
- Example:
public synchronized void synchronizedMethod() { // Synchronized method body }
- Synchronized Blocks:
- Synchronized blocks allow finer-grained control over synchronization by specifying a block of code that should be executed atomically by only one thread at a time.
- Synchronized blocks require an explicit lock object (usually an instance of
Object
orClass
) on which the synchronization is performed. - Example:
public void someMethod() { synchronized (lockObject) { // Synchronized block body } }
- Static Synchronization:
- In addition to instance-level synchronization, Java supports synchronization at the class level using the
static
keyword. - Static synchronized methods and blocks use the class’s monitor lock for synchronization, preventing concurrent access by multiple threads to static data and static methods.
- Example:
public static synchronized void staticSynchronizedMethod() { // Static synchronized method body }
- Reentrant Synchronization:
- Java supports reentrant synchronization, which allows a thread to acquire the same lock multiple times without causing deadlock.
- Reentrant synchronization ensures that a thread holding a lock can re-enter synchronized blocks protected by the same lock without being blocked by itself.
- This behavior is useful when synchronized methods call other synchronized methods on the same object or when synchronized methods are recursive.
- Concurrency Utilities:
- Java provides high-level concurrency utilities such as
java.util.concurrent.locks
package, which offers more advanced synchronization mechanisms likeReentrantLock
,ReadWriteLock
, andSemaphore
. - These utilities provide more flexibility and control over synchronization, allowing developers to implement complex synchronization patterns and avoid common pitfalls such as deadlock and livelock.
Synchronization in Java is a fundamental concept for writing thread-safe and concurrent applications. It ensures that shared resources are accessed safely and consistently by multiple threads, preventing data corruption and maintaining application integrity. However, synchronization should be used judiciously to avoid performance bottlenecks and deadlocks, and developers should be aware of best practices and concurrency pitfalls when designing synchronized code.
What is the purpose of the volatile keyword in Java? How does it differ from synchronization?
In Java, the volatile
keyword is used to indicate that a variable’s value may be modified by multiple threads asynchronously. When a variable is declared as volatile
, it ensures that any thread reading the variable will always see the most recent value written to it by any other thread. The volatile
keyword ensures visibility of changes to the variable’s value across threads, but it does not provide atomicity or mutual exclusion like synchronization does.
Here’s a comparison of the purpose and differences between the volatile
keyword and synchronization in Java:
- Purpose of
volatile
:
volatile
ensures visibility of changes to the variable’s value across threads.- It prevents threads from caching the variable’s value locally and ensures that changes made by one thread are visible to other threads immediately.
volatile
is primarily used for variables that are shared between threads and accessed frequently, but where synchronization overhead is not necessary.
- Purpose of Synchronization:
- Synchronization ensures atomicity, mutual exclusion, and consistency of shared resources accessed by multiple threads.
- Synchronization prevents data races, race conditions, and other concurrency issues by ensuring that only one thread can access a critical section of code or shared resource at a time.
- Synchronization is used when multiple threads need to coordinate access to shared resources, maintain data consistency, or perform operations atomically.
- Differences:
- Visibility vs. Mutual Exclusion: The primary purpose of
volatile
is to ensure visibility of changes to a variable’s value across threads, while synchronization provides mutual exclusion to prevent concurrent access to shared resources. - Atomicity:
volatile
does not provide atomicity, meaning it cannot ensure that compound operations on a variable (such as incrementing a counter) are executed atomically. Synchronization mechanisms such assynchronized
blocks or locks can ensure atomicity by preventing concurrent access to critical sections of code. - Performance:
volatile
has lower overhead compared to synchronization, as it does not involve acquiring and releasing locks or entering synchronized blocks. However, synchronization mechanisms may incur higher overhead due to the cost of acquiring and releasing locks, context switching, and potential contention between threads. - Granularity:
volatile
is typically used for individual variables, while synchronization mechanisms can be applied at different levels of granularity, such as methods, blocks, or objects, allowing for more fine-grained control over concurrency.
In summary, the volatile
keyword is used to ensure visibility of changes to shared variables across threads, while synchronization mechanisms such as synchronized
blocks or locks provide mutual exclusion and ensure atomicity of operations on shared resources. Each mechanism serves a different purpose in managing concurrency in Java programs, and the choice between volatile
and synchronization depends on the specific requirements and performance considerations of the application.
How does Java support functional programming? Discuss the features introduced in Java 8 to support functional programming.
Java supports functional programming paradigm through the introduction of several features in Java 8 and later versions. Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. Here are the key features introduced in Java 8 to support functional programming:
- Lambda Expressions:
- Lambda expressions allow developers to treat functionality as a method argument, or to create concise and expressive anonymous functions.
- Lambda expressions enable functional programming capabilities in Java by providing a way to express instances of single-method interfaces (functional interfaces) more compactly.
- Lambda expressions facilitate the use of functional interfaces, higher-order functions, and functional-style programming constructs.
- Functional Interfaces:
- A functional interface is an interface that contains exactly one abstract method (SAM type), often representing a single unit of behavior.
- Java 8 introduced the
java.util.function
package, which includes predefined functional interfaces such asFunction
,Predicate
,Consumer
, andSupplier
. - Functional interfaces provide a foundation for lambda expressions and method references, allowing developers to write more expressive and concise code.
- Method References:
- Method references provide a shorthand syntax for referencing methods as lambda expressions.
- Method references allow developers to reuse existing methods as lambda expressions, improving code readability and maintainability.
- Method references can be classified into four types: static method references, instance method references, constructor references, and arbitrary object method references.
- Streams API:
- The Streams API introduced in Java 8 provides a fluent and declarative way to process collections of data in a functional style.
- Streams allow developers to express common operations such as filtering, mapping, reducing, and aggregating data in a concise and expressive manner.
- Streams encourage immutability, lazy evaluation, and parallelism, enabling efficient and scalable data processing operations.
- Optional:
- The
Optional
class introduced in Java 8 represents an object that may or may not contain a value. Optional
provides a functional approach to handling potentially nullable values, avoiding null pointer exceptions and promoting safer and more predictable code.Optional
encourages developers to write code that explicitly handles absent values, leading to more robust and error-resistant code.
- Default Methods:
- Java 8 introduced default methods, also known as defender methods or virtual extension methods, which allow interfaces to provide default implementations for methods.
- Default methods enable backward compatibility and extend the functionality of existing interfaces without breaking existing implementations.
- Default methods facilitate the adoption of functional programming patterns by providing utility methods and behavior to functional interfaces.
- Parallel Array Sorting:
- Java 8 introduced parallel sorting methods in the
Arrays
class, such asparallelSort()
, which leverage multi-core processors to perform sorting operations in parallel. - Parallel array sorting improves the performance of sorting large arrays by exploiting parallelism and concurrency, enhancing the scalability and efficiency of sorting algorithms.
These features introduced in Java 8 and subsequent versions significantly enhance the support for functional programming in Java, allowing developers to write more expressive, concise, and efficient code using functional programming paradigms. Functional programming enables developers to write code that is easier to reason about, test, and maintain, leading to improved productivity and software quality.
What are the best practices for writing efficient and maintainable Java code?
Writing efficient and maintainable Java code involves following a set of best practices and guidelines that promote readability, scalability, and maintainability of the codebase. Here are some key best practices for writing efficient and maintainable Java code:
- Follow Coding Standards:
- Adhere to established coding standards and style guidelines, such as those defined by the Java Code Conventions or the Google Java Style Guide.
- Consistent formatting, naming conventions, and code organization improve readability and maintainability of the codebase.
- Use Descriptive Names:
- Choose meaningful and descriptive names for variables, methods, classes, and packages that accurately convey their purpose and functionality.
- Avoid cryptic abbreviations and acronyms, and use camelCase or snake_case naming conventions for consistency.
- Write Modular and Cohesive Code:
- Break down large and complex code into smaller, modular components that have well-defined responsibilities and cohesive functionality.
- Encapsulate related functionality within classes and methods, following the single responsibility principle (SRP) and separation of concerns.
- Follow Object-Oriented Principles:
- Design classes and interfaces that adhere to object-oriented principles such as encapsulation, inheritance, and polymorphism.
- Favor composition over inheritance and strive for loose coupling between classes to promote flexibility and extensibility.
- Handle Errors and Exceptions Gracefully:
- Use proper error handling and exception management techniques to handle anticipated and unexpected errors gracefully.
- Catch exceptions at an appropriate level of abstraction, log error messages, and provide meaningful error handling and recovery mechanisms.
- Avoid Magic Numbers and Strings:
- Avoid hardcoding numeric or string literals (magic numbers and strings) directly into the code, as they can be difficult to understand and maintain.
- Define constants or enumeration types to represent these values, providing clarity and allowing for easier updates in the future.
- Optimize Performance Carefully:
- Profile the codebase to identify performance bottlenecks and optimize critical sections of code judiciously.
- Use appropriate data structures, algorithms, and optimization techniques to improve the efficiency of the code without sacrificing readability or maintainability.
- Use Immutable Objects and Thread-Safe Constructs:
- Favor immutable objects and immutable collections where possible to simplify state management and avoid concurrency issues.
- Use thread-safe data structures and synchronization mechanisms such as
synchronized
blocks,volatile
variables, and atomic classes to ensure thread safety in concurrent environments.
- Write Unit Tests:
- Practice test-driven development (TDD) and write comprehensive unit tests for all critical and non-trivial code paths.
- Automated tests help validate the correctness of the code, detect regressions, and ensure that modifications do not introduce unintended side effects.
- Document Code and Provide Documentation:
- Write clear and concise comments to document the purpose, behavior, and usage of classes, methods, and complex algorithms.
- Provide high-level and API-level documentation using tools like Javadoc to generate API documentation automatically.
- Refactor Regularly:
- Refactor the code regularly to improve its design, readability, and maintainability.
- Remove duplication, eliminate code smells, and apply design patterns and best practices to simplify complex code.
By following these best practices, developers can write efficient, maintainable, and high-quality Java code that is easy to understand, modify, and extend over time. These practices contribute to the overall robustness and reliability of the software, leading to improved productivity and satisfaction among developers and users alike.
These questions cover a range of topics and concepts in Java programming and are commonly asked during interviews for candidates with 5 years of experience in Java development.
Conclusion
In conclusion, preparing for a “Core Java Interview Questions for 5 Years Experience” requires a comprehensive understanding of fundamental concepts, advanced features, and best practices in Java programming. This collection of interview questions aims to assess candidates’ proficiency in various areas of Java development, including object-oriented programming, data structures, algorithms, concurrency, exception handling, and design patterns.
Candidates with five years of experience are expected to demonstrate not only proficiency in writing Java code but also an understanding of industry best practices, design principles, and optimization techniques. Additionally, they should be able to articulate their thought process, problem-solving skills, and real-world application of Java concepts during the interview process.
By reviewing and practicing these interview questions, candidates can refresh their knowledge, identify areas for improvement, and gain confidence in their ability to tackle challenging technical interviews. Furthermore, staying up-to-date with the latest advancements in Java, industry trends, and emerging technologies will enhance candidates’ competitiveness in the job market and contribute to their professional growth and success as Java developers.
Overall, with diligent preparation, strong technical skills, and effective communication, candidates with five years of experience in core Java can confidently approach interviews, demonstrate their expertise, and secure rewarding career opportunities in the field of software development.
Crack DSA Interview: Google, Facebook, and Amazon!👇
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
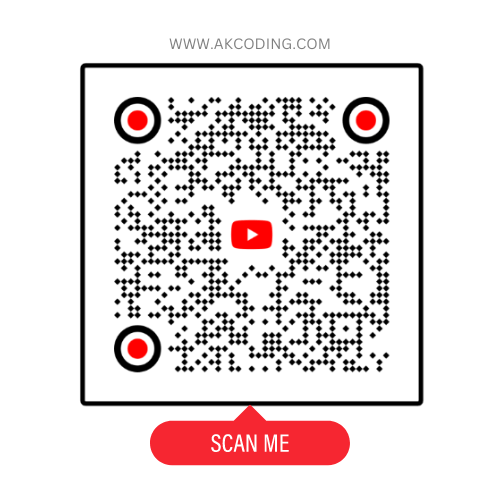