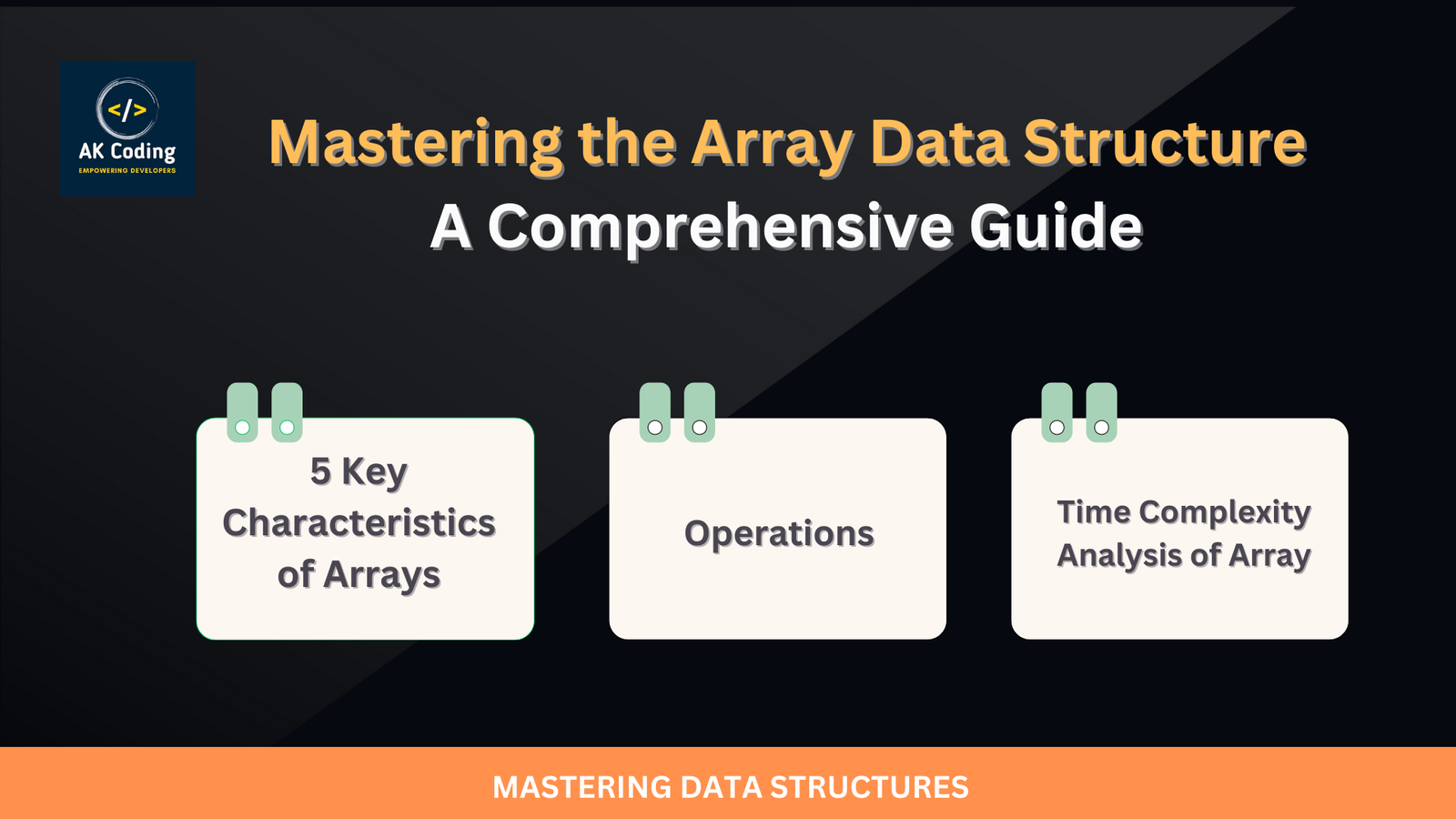
Table of Contents
Array Data Structure
Array data structure are fundamental data structures that store collections of elements of the same type in contiguous memory locations. Accessed by index, they provide efficient random access to elements and are versatile in storing homogeneous data. Array data structure offer constant-time access to individual elements and support operations such as insertion, deletion, searching, and copying. Despite their simplicity, array data structure offer powerful capabilities for organizing and managing data efficiently. They serve as the foundation for implementing other data structures such as lists, queues, and stacks, and are extensively used in algorithm design, numerical computing, string manipulation, and memory management.
5 Key Characteristics of Array Data Structure
- Homogeneous Elements: Arrays consist of elements that are of the same data type (e.g., integers, characters, floats). This uniformity allows for efficient memory allocation and access.
- Index-based Access: Each element in an array is identified by its index or position within the array. Array indices typically start from 0 and go up to the size of the array minus one.
- Fixed Size: In most programming languages, arrays have a fixed size, meaning that the number of elements they can hold is determined at the time of declaration and cannot be changed dynamically during runtime.
- Contiguous Memory Allocation: Elements in an array are stored in adjacent memory locations, allowing for efficient memory access and traversal.
- Random Access: Arrays support constant-time access to individual elements using their indices. This allows for efficient retrieval, modification, and manipulation of array elements.
Overview of Operations on Array Data Structure
- Initialization: Arrays can be initialized with a specific size and optionally with initial values for its elements.
- Accessing Elements: Individual elements in an array can be accessed using their indices. The time complexity for accessing an element in an array is O(1).
- Insertion and Deletion: Inserting or deleting elements in an array can be less efficient compared to accessing elements, especially when performed in the middle or beginning of the array. The time complexity for insertion or deletion at a specific index is O(n), where n is the size of the array.
- Traversal: Arrays can be traversed sequentially to access and process each element in the array.
- Sorting and Searching: Arrays are commonly used in sorting and searching algorithms due to their random access property. Sorting algorithms like quicksort and mergesort, as well as searching algorithms like binary search, often operate on arrays.
Accessing Elements of Array Data Structure
Accessing elements in an array data structure involves retrieving the value stored at a specific index position within the array. In most programming languages, array data structure indexing starts from 0, meaning the first element is accessed using index 0, the second element using index 1, and so on. Here’s how you can access elements in an array data structure.
Using Square Brackets [ ] Notation:
- In many programming languages like C, C++, Java, and JavaScript, you can access array elements using square brackets notation. Example (in JavaScript):
// Declare an array
let numbers = [10, 20, 30, 40, 50];
// Access the first element (index 0)
let firstElement = numbers[0];
console.log(firstElement); // Output: 10
// Access the third element (index 2)
let thirdElement = numbers[2];
console.log(thirdElement); // Output: 30
Using Pointer Arithmetic (C and C++):
- In C and C++, arrays are internally implemented as pointers. You can use pointer arithmetic to access array elements. Example (in C):
// Declare an array
int numbers[] = {10, 20, 30, 40, 50};
// Access the first element (index 0)
int firstElement = *(numbers + 0);
printf("%d\n", firstElement); // Output: 10
// Access the third element (index 2)
int thirdElement = *(numbers + 2);
printf("%d\n", thirdElement); // Output: 30
Regardless of the programming language, accessing elements in an array data structure is a fundamental operation and is crucial for working with arrays efficiently.
Insertion and Deletion in Array Data Structure
Insertion and deletion operations in an array involve adding or removing elements from the array data structure. These operations can be performed at different positions within the array, such as at the beginning, end, or middle. However, the efficiency of these operations depends on the programming language and the specific implementation of arrays. Here’s how insertion and deletion typically work in array data structure:
Insertion
- At the End:
- To insert an element at the end of an array data structure, you need to resize the array (if necessary) and then assign the new element to the last position.
- The time complexity for inserting an element at the end of the array is usually O(1) on average, but O(n) in the worst case if resizing is required.
- At the Beginning:
- Inserting an element at the beginning of an array requires shifting all existing elements one position to the right to make space for the new element.
- The time complexity for inserting an element at the beginning of the array is O(n) because of the shifting operation.
- At a Specific Position (Middle):
- Inserting an element at a specific position (middle) of an array also involves shifting elements to make space for the new element.
- The time complexity for this operation is O(n) because, on average, half of the elements need to be shifted.
Deletion
- At the End:
- Deleting an element from the end of an array data structure involves simply decrementing the array’s size, effectively removing the last element.
- The time complexity for deleting an element from the end of the array is O(1).
- At the Beginning:
- Deleting an element from the beginning of an array requires shifting all remaining elements one position to the left to fill the gap.
- The time complexity for this operation is O(n) because of the shifting operation.
- At a Specific Position (Middle):
- Deleting an element from a specific position (middle) of an array also involves shifting elements to fill the gap left by the deleted element.
- The time complexity for this operation is O(n) because, on average, half of the elements need to be shifted.
It’s important to note that in languages like Python, arrays are implemented as lists, which are dynamic arrays. Insertion and deletion operations may have different efficiencies depending on the implementation and resizing strategies used by the language or library.
Searching in Array Data Structure
Searching in an array involves finding the position of a specific element within the array. There are several methods for searching in an array, each with its own characteristics and efficiency. The two most common methods are linear search and binary search:
Linear Search
- Linear search, also known as sequential search, involves iterating through each element in the array from the beginning until the target element is found or the end of the array is reached.
- Linear search is straightforward to implement and is suitable for unordered arrays or when the array size is small.
- The time complexity of linear search is O(n), where n is the number of elements in the array. In the worst-case scenario, the target element may be at the end of the array or not present, requiring iterating through all elements. Example (in Python):
def linear_search(arr, target):
for i in range(len(arr)):
if arr[i] == target:
return i # Return index if target found
return -1 # Return -1 if target not found
# Example usage:
numbers = [10, 20, 30, 40, 50]
target = 30
index = linear_search(numbers, target)
print("Index of", target, ":", index)
Binary Search
- Binary search is a more efficient searching algorithm that works on sorted arrays. It repeatedly divides the search interval in half until the target element is found or the interval becomes empty.
- Binary search has a time complexity of O(log n), where n is the number of elements in the array. It is significantly faster than linear search for large arrays.
- Binary search requires the array to be sorted beforehand, which may add an additional overhead. Example (in Python):
def binary_search(arr, target):
low, high = 0, len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid # Return index if target found
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1 # Return -1 if target not found
# Example usage:
numbers = [10, 20, 30, 40, 50]
target = 30
index = binary_search(numbers, target)
print("Index of", target, ":", index)
It’s important to choose the appropriate search algorithm based on factors such as the size of the array, whether it’s sorted or not, and the expected frequency of searches. Binary search is ideal for large sorted arrays, while linear search may be suitable for small or unsorted arrays.
Time Complexity Analysis of Array Data Structure
The time complexity analysis of array operations depends on the specific operation being performed. Here’s a breakdown of the time complexity for common array operations:
Accessing an Element (Random Access)
- Time Complexity: O(1)
- Explanation: Accessing an element in an array by index involves simple pointer arithmetic, which allows for constant-time access to any element in the array. Regardless of the size of the array, the time taken to access an element remains constant.
Insertion and Deletion:
- At the End:
- Time Complexity: O(1) amortized
- Explanation: Inserting or deleting an element at the end of an array typically requires constant time. However, if the array needs to be resized to accommodate the new element or to reclaim memory after deletion, the operation may have an amortized time complexity of O(1), as resizing occurs infrequently.
- At the Beginning or at a Specific Position (Middle):
- Time Complexity: O(n)
- Explanation: Inserting or deleting an element at the beginning or at a specific position in the array requires shifting all subsequent elements, which takes linear time proportional to the number of elements in the array (n).
Searching
- Linear Search:
- Time Complexity: O(n)
- Explanation: In the worst-case scenario, linear search may need to iterate through all elements of the array to find the target element. Therefore, its time complexity is linear and proportional to the size of the array (n).
- Binary Search (for Sorted Arrays):
- Time Complexity: O(log n)
- Explanation: Binary search repeatedly divides the search interval in half, reducing the search space by half with each comparison. This results in a logarithmic time complexity, where the time taken is proportional to the logarithm of the size of the array (n).
Copying an Array
- Time Complexity: O(n)
- Explanation: Copying an entire array requires iterating through all elements of the array and copying each element individually. As a result, the time complexity of array copying is linear and proportional to the size of the array (n).
Overall, arrays offer efficient random access to elements (O(1)), but operations such as insertion, deletion, and searching may have linear or logarithmic time complexity depending on the specific operation and algorithm used. It’s important to consider these complexities when choosing data structures and algorithms for different programming tasks.
Applications and Use Cases of Array Data Structure
Arrays are fundamental data structures with numerous applications across various domains. Here are some common applications and use cases of arrays:
- Storing and Accessing Data: Arrays are widely used for storing collections of data elements of the same type. They provide efficient random access to individual elements using their indices.
- Matrices and Multidimensional Arrays: Arrays are used to represent matrices and multidimensional data structures. They are essential for tasks such as image processing, numerical simulations, and representing tabular data.
- Buffers and Memory Allocation: Arrays are used to allocate memory buffers for storing data in applications such as file I/O, network communication, and graphics processing. For example, arrays are used to represent image pixels in computer graphics.
- String Manipulation: Arrays of characters are used to represent and manipulate strings in programming languages. Many string-related operations, such as concatenation, substring extraction, and character manipulation, are performed using arrays.
- Collections and Lists: Arrays serve as the underlying data structure for implementing other collection types such as lists, queues, and stacks. For example, dynamic arrays (e.g., ArrayList in Java) are resizable arrays that dynamically adjust their size as elements are added or removed.
- Sorting and Searching Algorithms: Arrays are used extensively in sorting and searching algorithms due to their random access property. Sorting algorithms like quicksort, mergesort, and heapsort operate directly on arrays for efficient data processing.
- Caches and Memory Management: Arrays are used in cache memory management to store frequently accessed data elements for faster retrieval. They are also used in memory management algorithms for allocating and deallocating memory blocks.
- Lookup Tables and Dictionaries: Arrays are used to implement lookup tables and dictionaries for mapping keys to values. For example, hash tables and associative arrays use arrays as the underlying data structure for efficient key-value storage and retrieval.
- Sparse Arrays and Bitmaps: Arrays can be used to represent sparse data structures and bitmaps efficiently. Sparse arrays store only non-zero elements, while bitmaps represent sets or binary data using compact arrays of bits.
- Parallel and Distributed Computing: Arrays are used in parallel and distributed computing environments for data parallelism and distributed data storage. Parallel arrays allow for efficient parallel processing of data elements across multiple processors or computing nodes.
These are just a few examples of the many applications and use cases of arrays. Arrays are versatile data structures that play a fundamental role in computer science and software development, enabling efficient storage, manipulation, and processing of data in various applications and domains.
Limitations of Array Data Structure
- Fixed Size: Arrays have a fixed size once allocated, meaning their size cannot be changed dynamically at runtime. This limitation makes it challenging to accommodate varying amounts of data or dynamically growing collections.
- Contiguous Memory Allocation: Arrays require contiguous memory allocation, which can be challenging to obtain for large arrays or in memory-constrained environments. This limitation can lead to memory fragmentation and inefficient memory usage.
- Inefficient Insertion and Deletion: Insertion and deletion operations at arbitrary positions in an array can be inefficient, especially for large arrays. Adding or removing elements may require shifting all subsequent elements, resulting in a time complexity of O(n).
- Homogeneous Data Type: Arrays typically store elements of the same data type. This limitation restricts their flexibility in handling heterogeneous data and may require additional data transformations or storage mechanisms.
- Sparse Data Representation: Arrays are not suitable for representing sparse data structures where most elements are empty or contain default values. Using arrays for sparse data may result in inefficient memory usage and decreased performance.
- Wasted Space: Arrays may allocate more memory than necessary, especially if the array size is larger than the actual number of elements stored. This can result in wasted space and increased memory overhead.
- Lack of Built-in Operations: Arrays in some programming languages lack built-in operations for common tasks such as sorting, searching, and resizing. Developers may need to implement these operations manually or use libraries and frameworks to overcome this limitation.
- Static Structure: Arrays have a static structure, meaning their size and dimensions are fixed at compile time. This limitation makes it challenging to adapt arrays to dynamic or evolving data requirements without significant overhead or restructuring.
Despite these limitations, arrays remain a fundamental data structure with efficient random access and versatile applications. Understanding these limitations is essential for choosing the appropriate data structure for specific programming tasks and designing efficient algorithms.
Conclusion of Array Data Structure
In conclusion, the array data structure is a fundamental building block in computer science with a wide range of applications and use cases. Arrays offer efficient random access to elements, making them essential for storing and manipulating collections of data in programming languages.
Array Data Structure are versatile and can be used in various domains, including numerical computing, string manipulation, data processing, and algorithm design. They serve as the foundation for implementing other data structures such as lists, queues, and stacks, as well as for designing efficient sorting and searching algorithms.
Despite their simplicity, arrays provide powerful capabilities for organizing and managing data efficiently. They offer constant-time access to individual elements and support operations such as insertion, deletion, searching, and copying. Arrays are also used in memory management, caching, parallel computing, and distributed systems.
While Array Data Structure have many advantages, they also have limitations, such as fixed size and inefficient insertion and deletion operations at arbitrary positions. In scenarios where dynamic resizing or frequent insertions and deletions are required, other data structures like linked lists or dynamic arrays may be more suitable.
Overall, Array Data Structure remain a fundamental and indispensable data structure in computer science and software development. Understanding their properties, operations, and applications is essential for building efficient algorithms, designing scalable software systems, and solving real-world problems effectively.
Array Data Structures Video Tutorial
For More Data Structures and Algorithms
FAQs of Array Data Structure
What is Array Data Structure and its types?
An array is a fundamental data structure that stores a collection of elements of the same data type in contiguous memory locations. Each element in the array is identified by its index, which represents its position within the array. Arrays offer efficient random access to elements, making them suitable for various applications in programming.
Types of arrays include:
One-Dimensional Array: Also known as a single-dimensional array, this type of array stores elements in a linear sequence. Each element is accessed using a single index. One-dimensional arrays are the most common type of array and are used in a wide range of applications.
Multi-Dimensional Array: Multi-dimensional arrays store elements in multiple dimensions or dimensions. Examples include two-dimensional arrays (matrices), three-dimensional arrays (cubes or voxels), and higher-dimensional arrays. Multi-dimensional arrays are useful for representing structured data, such as images, matrices, and spatial data.
Dynamic Array: Dynamic arrays, also known as resizable arrays or ArrayLists in some programming languages, allow for dynamic resizing of the array size at runtime. They automatically resize themselves to accommodate additional elements as needed, making them more flexible than fixed-size arrays.
Jagged Array: A jagged array is an array of arrays, where each element of the main array can itself be an array. Unlike multi-dimensional arrays, jagged arrays do not have a fixed number of columns for each row. This flexibility allows for irregular or ragged structures, where each row may have a different number of elements.
Sparse Array: Sparse arrays are used to represent arrays where the majority of elements are empty or have default values. Instead of allocating memory for all elements, sparse arrays only store non-empty or non-default values, saving memory and improving efficiency for sparse data structures.
These types of arrays offer different capabilities and are suitable for various programming scenarios. Understanding the characteristics and use cases of each type of array is essential for efficient data organization and manipulation in programming.
What are the advantages of using arrays?
There are several advantages to using arrays in programming:
Efficient Random Access: Arrays offer constant-time access to individual elements using their index. This allows for efficient retrieval, modification, and manipulation of data elements.
Compact Data Storage: Arrays store elements in contiguous memory locations, resulting in efficient memory usage and compact data storage. This makes arrays suitable for storing large collections of data in memory.
Versatility: Arrays are versatile data structures that can store elements of the same data type. They can be used to represent a wide range of data types, including integers, floating-point numbers, characters, and custom objects.
Ease of Use: Arrays are simple and straightforward to use, with built-in support for common operations such as insertion, deletion, sorting, and searching. This makes them suitable for a wide range of programming tasks and applications.
Support for Iteration: Arrays support efficient iteration and traversal, allowing for easy processing of all elements in the array using loops or iterators. This makes them ideal for tasks such as data processing, filtering, and transformation.
Static Size: While it can be seen as a limitation, the static size of arrays can also be an advantage in certain scenarios where the size of the data is known in advance and does not need to change dynamically.
Efficient Sorting and Searching: Arrays are well-suited for sorting and searching algorithms due to their random access property. Sorting algorithms like quicksort and mergesort, as well as searching algorithms like binary search, often operate efficiently on arrays.
Overall, arrays offer a combination of efficiency, simplicity, and versatility, making them indispensable data structures in programming and software development.
How are arrays different from other data structures like linked lists?
Arrays and linked lists are both fundamental data structures used for storing collections of data elements, but they differ in several key aspects:
Memory Allocation:
Arrays: Arrays store elements in contiguous memory locations. This means that elements are stored one after another in memory, allowing for efficient random access using indices.
Linked Lists: Linked lists store elements as nodes, where each node contains both data and a reference (or pointer) to the next node in the sequence. Nodes may be scattered across memory, and the links between nodes facilitate traversal.
Dynamic Resizing:
Arrays: In most programming languages, arrays have a fixed size that is determined at compile time. Resizing an array typically involves creating a new, larger array and copying elements from the old array to the new one.
Linked Lists: Linked lists can dynamically resize by adding or removing nodes. This is because each node contains a reference to the next node, allowing for efficient insertion and deletion operations without the need for contiguous memory allocation.
Access Time:
Arrays: Arrays offer constant-time access to elements using their index. This provides efficient random access to any element in the array.
Linked Lists: Linked lists do not offer constant-time access to elements. Traversing a linked list requires following the links from one node to the next, which results in linear time complexity for accessing elements.
Insertion and Deletion:
Arrays: Insertion and deletion operations in arrays can be inefficient, especially for inserting or deleting elements in the middle of the array. This is because it may require shifting all subsequent elements to accommodate the change.
Linked Lists: Linked lists excel at insertion and deletion operations. Adding or removing a node from a linked list simply involves updating the links between nodes, resulting in constant-time complexity for these operations.
Memory Overhead:
Arrays: Arrays may have less memory overhead compared to linked lists since they only store the data elements themselves.
Linked Lists: Linked lists have additional memory overhead due to the pointers or references stored in each node, which are used to maintain the links between nodes.
In summary, arrays offer efficient random access but have a fixed size and may be less flexible for dynamic resizing and insertion/deletion operations. Linked lists, on the other hand, excel at dynamic resizing and insertion/deletion operations but may have slower access times due to traversal. The choice between arrays and linked lists depends on the specific requirements of the application and the trade-offs between efficiency and flexibility.
Can arrays store elements of different data types?
In most programming languages, arrays typically store elements of the same data type. This means that all elements in an array must be of the same data type, such as integers, floating-point numbers, characters, or custom objects.
For example, in Java, C, C++, and similar languages, arrays are homogeneous, meaning they can only store elements of the same data type. Attempting to store elements of different data types in the same array would result in a compilation error.
However, some programming languages, such as Python, allow for more flexibility with arrays. In Python, arrays are implemented as lists, and lists can contain elements of different data types. This is because Python lists are dynamically typed, meaning they can hold elements of any data type without restriction.
Here’s an example of a Python list containing elements of different data types:mixed_list = [1, "hello", 3.14, True]
In this example, the list mixed_list
contains elements of different types, including an integer, a string, a floating-point number, and a boolean value. Python’s dynamic typing allows for such flexibility, but it’s important to note that this behavior may not be present in all programming languages that use arrays.
What is the time complexity for accessing elements in an array?
The time complexity for accessing elements in an array is O(1), also known as constant time complexity. This means that the time taken to access an element in an array does not depend on the size of the array. Regardless of the number of elements in the array, accessing any specific element using its index can be done in constant time.
In other words, accessing elements in an array involves direct memory addressing using the index. Each element in the array is stored in contiguous memory locations, and the index is used to calculate the memory address of the desired element. As a result, accessing elements in an array is a straightforward operation with a constant time complexity.
Which of the following is the advantage of the array data structure?
1. Random Access
2.Memory Efficiency
3. Cache Friendly
4. Simplicity
5. Predictable Memory Usage
6. All of the above
What is the Disadvantage of array Data Structure
The disadvantages of the array data structure include:
Fixed Size:
Once an array is defined, its size cannot be changed (in most programming languages). This means if you underestimate the size, you might run out of space, and if you overestimate, you waste memory.
Inefficient Insertion and Deletion:
Inserting or deleting elements, especially in the middle of an array, can be slow because all subsequent elements need to be shifted. This makes insertion and deletion operations inefficient in terms of time complexity, usually taking O(n) time.
Contiguous Memory Requirement:
Arrays need contiguous blocks of memory, which can lead to memory allocation issues, especially with large arrays. Finding large contiguous memory blocks may be challenging, leading to memory fragmentation.
No Flexibility in Resizing:
Arrays cannot dynamically adjust their size during runtime, making them less flexible for applications where the size of the data set changes frequently.
Inefficient Searching in Unsorted Arrays:
Searching for an element in an unsorted array requires a linear search (O(n)) because the elements are not ordered. More efficient search methods, like binary search, cannot be used unless the array is sorted.
Homogeneous Data:
Arrays typically store elements of the same data type. If you need to store different types of data, you’ll have to use more complex data structures like objects or hashmaps.
These limitations make arrays less suitable for certain applications, particularly when dynamic resizing, frequent insertions, or deletions are required. In such cases, data structures like linked lists or dynamic arrays (like ArrayLists in Java) may be more appropriate.
Advantages of array Sata Structure
The advantages of the array data structure include:
Efficient Access (Random Access):
Arrays provide constant time complexity (O(1)) for accessing elements if you know the index. This is one of the biggest advantages of arrays because you can quickly retrieve any element without looping through the array.
Memory Efficiency:
Arrays store data in contiguous memory locations, which makes them memory-efficient for storing large amounts of data. The memory overhead is minimal compared to other data structures like linked lists, where pointers take up additional space.
Easy Iteration:
Arrays are easy to iterate over using loops, making them ideal for situations where you need to process each element sequentially. This makes them a straightforward and simple data structure to work with for many common programming tasks.
Cache Friendly:
Since arrays store elements in contiguous memory locations, they are cache-friendly. This leads to better performance when accessing elements because accessing one element often brings nearby elements into the CPU cache.
Simplicity:
Arrays are one of the simplest data structures to implement and use. Their structure and operations (like traversal, updating, etc.) are intuitive, making them ideal for beginners or for simple tasks where more complex data structures are not required.
Static Nature (Predictable Memory Usage):
In situations where you know the size of the dataset in advance, arrays can be advantageous because they have a fixed size. This predictability can make them easier to manage compared to dynamic data structures.
Multi-dimensional Support:
Arrays can easily be extended to multiple dimensions (like 2D arrays) for representing matrices, grids, or tables, which is essential in many mathematical and real-world applications.
Efficient Searching in Sorted Arrays:
If the array is sorted, efficient searching algorithms like binary search (O(log n)) can be used, making arrays a good choice for read-heavy applications where data is sorted.
Despite their simplicity and speed for access, arrays are best used when the size is known and when the number of insertions or deletions is minimal.
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
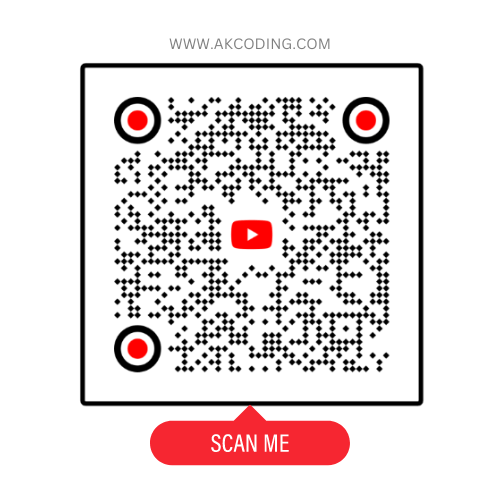