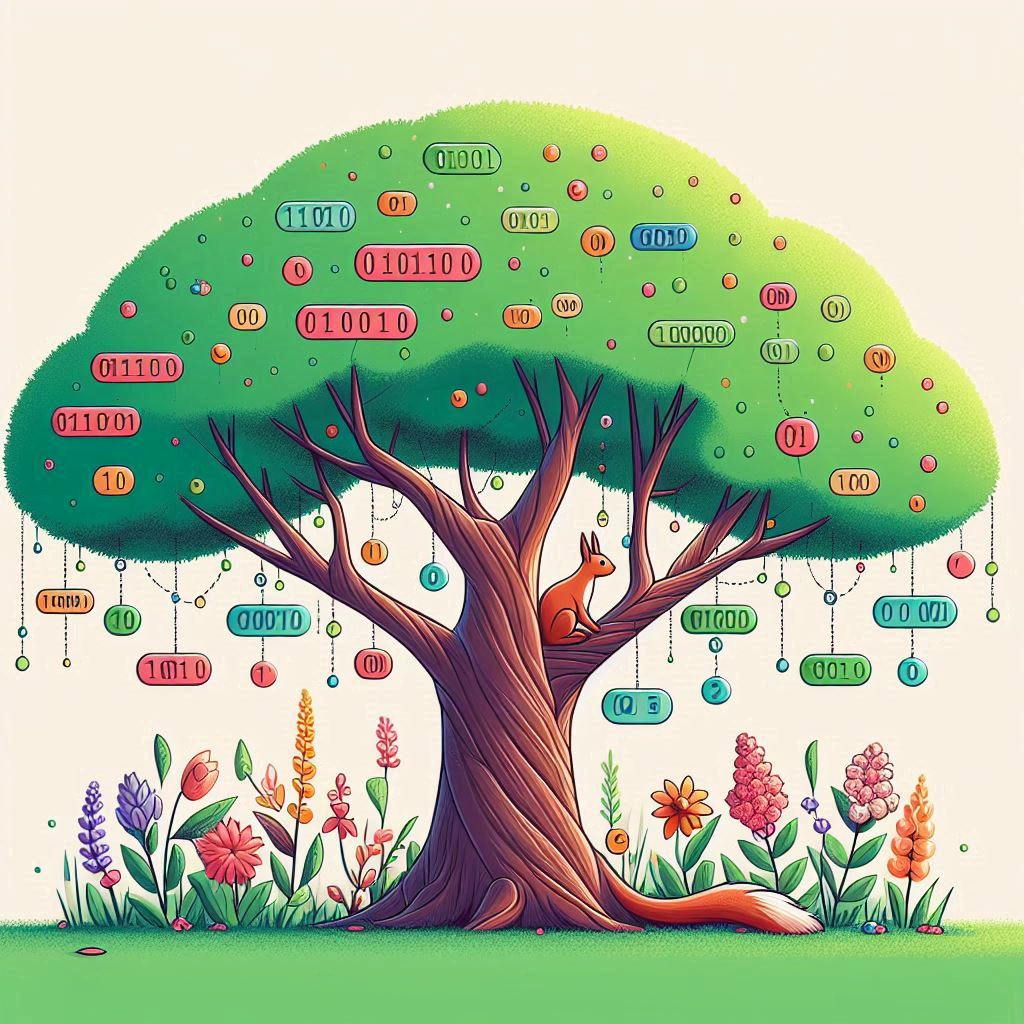
Binary Search Tree
1. Introduction
A Binary Search Tree (BST) is a fundamental data structure in computer science, widely used for its efficiency in search, insert, and delete operations. BSTs provide a systematic way of storing data that allows for quick retrieval, making them a crucial tool in many applications such as databases, search engines, and file systems.
In this blog post, we will explore what a Binary Search Tree is, understand its structure and properties, and dive into its core operations. Whether you’re a beginner looking to grasp the basics or a seasoned developer seeking to refresh your knowledge, this guide will provide you with a clear understanding of BSTs and their significance in the world of programming.
2. What is a Binary Search Tree?
A Binary Search Tree (BST) is a specialized type of binary tree that organizes data in a hierarchical structure. Each node in a BST contains three elements: a value, a reference to the left child, and a reference to the right child.
Key Characteristics of a Binary Search Tree:
- Node Structure:
- Value: Each node holds a unique value.
- Left Child: A pointer/reference to the left child node.
- Right Child: A pointer/reference to the right child node.
- Binary Tree Properties:
- A BST is a binary tree, meaning each node has at most two children.
- The left child node’s value is always less than the parent node’s value.
- The right child node’s value is always greater than or equal to the parent node’s value.
- Recursive Definition:
- The left and right subtrees of a node are also binary search trees.
Visual Representation:
Consider the following example:
15
/ \
10 20
/ \ / \
8 12 17 25
- The root node is 15.
- The left subtree contains values less than 15 (10, 8, 12).
- The right subtree contains values greater than or equal to 15 (20, 17, 25).
Why Use a Binary Search Tree?
BSTs are incredibly efficient for operations such as searching, inserting, and deleting elements. They are designed to keep the data sorted and allow for quick lookup, which is why they are commonly used in various applications where fast data retrieval is essential.
Core Operations:
- Search: Quickly locate a value in the tree by comparing it to the root and traversing left or right as needed.
- Insertion: Add new elements while maintaining the BST properties.
- Deletion: Remove elements while ensuring the tree remains a BST.
The structure and properties of BSTs make them a powerful tool for managing ordered data, and they serve as the foundation for more advanced data structures like AVL Trees and Red-Black Trees.
3. Basic Operations on a Binary Search Tree
Binary Search Trees (BSTs) are designed to perform various operations efficiently. The three most fundamental operations are searching, insertion, and deletion. Understanding these operations is crucial for working with BSTs.
1. Searching in a Binary Search Tree
The search operation in a BST is highly efficient due to the tree’s ordered structure. The process involves comparing the target value with the root and recursively traversing the tree.
Algorithm:
- Start at the root node.
- Compare the target value with the current node’s value.
- If they are equal, the target value is found.
- If the target value is less than the current node’s value, move to the left child.
- If the target value is greater than the current node’s value, move to the right child.
- Repeat the process until the target value is found or the subtree becomes empty (target value is not in the tree).
Time Complexity: O(log n) on average, where n is the number of nodes in the tree. In the worst case (if the tree becomes unbalanced), the complexity can degrade to O(n).
2. Insertion in a Binary Search Tree
Inserting a new value into a BST involves finding the correct location in the tree while maintaining the BST properties.
Algorithm:
- Start at the root node.
- Compare the value to be inserted with the current node’s value.
- If the value is less, move to the left child.
- If the value is greater, move to the right child.
- Continue this process until you reach a null (empty) child.
- Insert the new node at this position.
Example:
To insert the value 13 into the following BST:
15
/ \
10 20
/ \ / \
8 12 17 25
- Compare 13 with 15 (go left), then with 10 (go right), then with 12 (go right).
- Insert 13 as the right child of 12.
Time Complexity: O(log n) on average. In the worst case (unbalanced tree), it can be O(n).
3. Deletion in a Binary Search Tree
The deletion operation is more complex because it involves three cases based on the node to be deleted:
Case 1: Deleting a Leaf Node (Node with No Children)
- Simply remove the node.
Case 2: Deleting a Node with One Child
- Replace the node with its only child.
Case 3: Deleting a Node with Two Children
- Find the node’s in-order successor (the smallest node in the right subtree).
- Replace the node’s value with the in-order successor’s value.
- Delete the in-order successor (which will now be in one of the simpler cases).
Example:
To delete 15 from the BST:
15
/ \
10 20
/ \ / \
8 12 17 25
- Find 15’s in-order successor (17).
- Replace 15 with 17.
- Delete 17 from its original position.
Time Complexity: O(log n) on average. In the worst case, it can be O(n).
4. Traversal in a Binary Search Tree
Traversal refers to the process of visiting all nodes in the tree. There are several ways to traverse a BST:
- In-Order Traversal (Left, Root, Right): Visits nodes in ascending order.
- Pre-Order Traversal (Root, Left, Right): Useful for copying the tree.
- Post-Order Traversal (Left, Right, Root): Useful for deleting the tree.
Traversal is an important operation for various tasks such as printing the tree or performing certain algorithms on the tree’s structure.
The basic operations on a Binary Search Tree are foundational to its use in efficient data management. Whether searching for, inserting, or deleting nodes, understanding these operations is key to leveraging the power of BSTs in your programming tasks.
4. Properties of a Binary Search Tree
A Binary Search Tree (BST) is a specialized binary tree with specific properties that enable efficient searching, insertion, and deletion of elements. Understanding these properties is essential for working with BSTs effectively.
1. Binary Tree Structure
A BST is a type of binary tree, meaning each node can have at most two children: a left child and a right child. This binary structure is fundamental to the organization and functionality of the BST.
2. Node Order Property
The primary property that distinguishes a BST from other binary trees is the node order property:
- Left Subtree: All nodes in the left subtree of a node have values less than the node’s value.
- Right Subtree: All nodes in the right subtree of a node have values greater than the node’s value.
- Recursion: This property holds true for each node in the tree, meaning it applies recursively to every subtree within the BST.
Example:
Consider the following BST:
15
/ \
10 20
/ \ / \
8 12 17 25
- The left subtree of 15 contains values less than 15 (10, 8, 12).
- The right subtree of 15 contains values greater than 15 (20, 17, 25).
- This pattern holds true for each subtree in the tree.
3. Uniqueness of Values
In a standard BST, all values are unique. This uniqueness ensures that each element is stored at a distinct node in the tree, preventing ambiguity during operations like searching or inserting.
4. In-Order Traversal Yields Sorted Sequence
One of the most important properties of a BST is that an in-order traversal (left, root, right) of the tree yields the values in sorted order. This property makes BSTs particularly useful for tasks that require sorted data.
Example:
In-order traversal of the above tree would produce the sequence: 8, 10, 12, 15, 17, 20, 25.
5. Depth and Height
- Depth of a node refers to the number of edges from the root to the node.
- Height of a node refers to the number of edges on the longest path from the node to a leaf.
In a balanced BST, the height is kept as low as possible, typically O(log n), where n is the number of nodes. This ensures that operations remain efficient.
6. Balanced vs. Unbalanced BST
The efficiency of BST operations depends on the tree’s balance:
- Balanced BST: A BST is considered balanced if the depth of the left and right subtrees of every node differs by at most one. Balanced trees maintain O(log n) time complexity for search, insertion, and deletion operations.
- Unbalanced BST: If a BST becomes unbalanced, with nodes skewed to one side, the time complexity for operations can degrade to O(n), where n is the number of nodes.
Example of Unbalanced BST:
10
\
20
\
30
In this case, the BST behaves more like a linked list, leading to inefficient operations.
The properties of a Binary Search Tree are designed to facilitate efficient data management and operations. By maintaining the node order property, ensuring uniqueness, and keeping the tree balanced, BSTs provide a powerful data structure for a wide range of applications. Understanding these properties is key to using BSTs effectively in your programming projects.
5. Common Applications of Binary Search Trees
Binary Search Trees (BSTs) are versatile data structures used in various applications due to their efficiency in performing search, insertion, and deletion operations. Below are some of the most common applications of BSTs:
1. Searching and Data Retrieval
One of the primary uses of BSTs is in searching and retrieving data efficiently. Because of the node order property, BSTs allow for quick lookups, making them ideal for applications where frequent search operations are required.
- Example: Implementing a phonebook application where names (or phone numbers) can be quickly searched.
2. Dynamic Sorting
BSTs maintain elements in a sorted order naturally due to their structure. This makes them suitable for dynamic data sets where elements are frequently added or removed, and the data must remain sorted.
- Example: Implementing a priority queue where elements are inserted and retrieved based on their priority level.
3. Implementing Symbol Tables
In compilers and interpreters, symbol tables are used to store information about variables, functions, and other entities. BSTs can be used to implement these symbol tables, allowing for efficient insertion, lookup, and deletion of symbols.
- Example: A compiler’s symbol table where identifiers (variables, functions) are stored and retrieved based on their names.
4. Database Indexing
BSTs are often used to implement indexing in databases. Indexes are used to speed up the retrieval of records by maintaining a sorted structure that can be searched efficiently.
- Example: Indexing a database table by a specific column to quickly retrieve records based on that column’s values.
5. Range Queries
BSTs are well-suited for answering range queries, where the goal is to find all elements within a certain range. Because of the in-order traversal property, BSTs can efficiently retrieve all elements that fall within a specified range.
- Example: Finding all products within a certain price range in an e-commerce application.
6. Implementing Set and Map Data Structures
In many programming languages, set and map data structures (such as TreeSet
and TreeMap
in Java) are implemented using BSTs. These structures allow for efficient insertion, deletion, and lookup operations while maintaining sorted order.
- Example: A set data structure where elements need to be unique and sorted, such as a set of unique words in a document.
7. Autocompletion and Suggestion Engines
BSTs can be used in autocompletion and suggestion engines, where the goal is to suggest possible completions or related terms based on user input. The BST structure allows for quick retrieval of possible matches.
- Example: An autocomplete feature in a search engine where possible search queries are suggested based on the user’s input.
8. Memory Management
BSTs are used in some memory management algorithms, such as in managing free memory blocks. The sorted nature of BSTs helps in quickly finding the best-fit or first-fit memory block.
- Example: A memory allocator that uses a BST to manage and allocate free memory blocks efficiently.
9. Building Binary Heaps
Although not directly a BST, binary heaps can be seen as a specialized form of binary trees used in implementing priority queues. BSTs can be used as the basis for understanding the more specialized binary heap structure.
- Example: Implementing a priority queue for a task scheduling system.
10. Implementing File Systems
Some file systems use BST-like structures to manage directories and files. The sorted nature of BSTs allows for efficient file and directory management, especially in hierarchical file systems.
- Example: A file system’s directory structure where files and directories are stored and retrieved based on their names.
Binary Search Trees offer a flexible and efficient way to manage data in various applications. From basic search operations to complex database indexing, BSTs provide the foundational structure needed to implement a wide range of functionalities. Understanding their common applications helps in leveraging BSTs effectively in software development.
6. Variants of Binary Search Trees
Binary Search Trees (BSTs) have several variants that are designed to address specific limitations of the basic BST structure, such as balancing issues, memory usage, or operation efficiency. These variants improve upon the standard BST by ensuring better performance in scenarios where the basic BST might degrade. Below are some common variants of Binary Search Trees:
1. Balanced Binary Search Trees (BBSTs)
Balanced BSTs maintain a balance between the heights of the left and right subtrees of any node. This ensures that the tree remains roughly balanced, preventing the worst-case scenario where the tree becomes a linked list.
- Example: AVL Trees, Red-Black Trees
- Use Case: Applications requiring consistent O(log n) time complexity for insertions, deletions, and lookups, such as database indexing or file systems.
2. Self-Balancing Binary Search Trees
Self-balancing BSTs automatically re-balance themselves during insertions and deletions to ensure that the tree remains balanced. This prevents performance degradation over time.
- Example: AVL Trees, Red-Black Trees
- Use Case: When frequent insertions and deletions are expected, ensuring that the tree remains balanced for efficient operations.
3. AVL Trees
AVL Trees are a type of self-balancing BST where the heights of the two child subtrees of any node differ by at most one. If at any time the heights differ by more than one, rebalancing is performed.
- Use Case: When strict balancing is required to ensure O(log n) time complexity for operations, such as in real-time systems or applications with stringent performance requirements.
4. Red-Black Trees
Red-Black Trees are another type of self-balancing BST. In Red-Black Trees, nodes are colored red or black, and the tree maintains balance through specific rules about node colors and the paths from the root to leaves.
- Use Case: When a balance between strict balancing and performance is needed, such as in standard libraries of many programming languages (e.g.,
TreeMap
andTreeSet
in Java).
5. Splay Trees
Splay Trees are a type of self-adjusting BST. When a node is accessed, it is “splayed” (moved to the root) using tree rotations. This ensures that frequently accessed nodes are quicker to access in future operations.
- Use Case: Applications where certain elements are accessed more frequently than others, such as caches or data structures where access patterns are highly skewed.
6. Treaps
A Treap is a hybrid data structure that combines the properties of a binary search tree and a heap. Each node in a Treap has both a key and a priority, and the tree is maintained as a BST with respect to the keys and as a heap with respect to the priorities.
- Use Case: When a combination of randomization and balancing is desired, such as in randomized algorithms or priority queues with key-based search capabilities.
7. B-Trees
B-Trees are a generalization of BSTs that allow nodes to have more than two children. B-Trees are balanced multi-way search trees used in databases and file systems to efficiently manage large amounts of data.
- Use Case: Databases, file systems, and other applications where data needs to be stored and retrieved efficiently from secondary storage (e.g., disks).
8. B+ Trees
A B+ Tree is an extension of the B-Tree where all values are stored at the leaf level, and internal nodes only store keys. B+ Trees are particularly useful in database indexing and file systems.
- Use Case: Database indexing, file systems, and any application requiring efficient range queries and sequential access to data.
9. Cartesian Trees
Cartesian Trees are a type of BST where the tree is structured to satisfy the heap property concerning priorities assigned to nodes. The tree is structured as a BST with respect to node values and as a heap with respect to priorities.
- Use Case: Applications requiring efficient searching and retrieval with an additional ordering constraint based on priorities, such as priority queues.
10. Tango Trees
Tango Trees are a type of BST designed to optimize the access time for a sequence of operations by dynamically restructuring the tree based on access patterns.
- Use Case: Applications where the sequence of operations is known or can be predicted, allowing for optimizations based on that sequence, such as in online algorithms or adaptive data structures.
Understanding the various variants of Binary Search Trees allows developers to select the most appropriate data structure for their specific needs. Each variant offers unique advantages tailored to different types of applications, ensuring that operations remain efficient even as the data set grows or evolves.
7. Implementation of Binary Search Tree
Implementing a Binary Search Tree (BST) involves creating the structure that represents the tree and defining the core operations such as insertion, deletion, and search. Below is a general guide to implementing a Binary Search Tree in a common programming language like Python.
1. Defining the Node Structure
The first step in implementing a BST is to define the structure of a node, which will store the value, as well as pointers to the left and right child nodes.
class Node:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
2. Creating the Binary Search Tree Class
Next, you create a class for the BST that will manage the nodes and provide methods for inserting, deleting, and searching for nodes.
class BinarySearchTree:
def __init__(self):
self.root = None
3. Inserting a Node
The insertion operation adds a new node to the tree, maintaining the BST property. The new node is placed in the appropriate position based on its value.
def insert(self, key):
if self.root is None:
self.root = Node(key)
else:
self._insert(self.root, key)
def _insert(self, current_node, key):
if key < current_node.key:
if current_node.left is None:
current_node.left = Node(key)
else:
self._insert(current_node.left, key)
elif key > current_node.key:
if current_node.right is None:
current_node.right = Node(key)
else:
self._insert(current_node.right, key)
4. Searching for a Node
The search operation checks whether a specific value is present in the tree. It traverses the tree by comparing the target value with the current node’s key.
def search(self, key):
return self._search(self.root, key)
def _search(self, current_node, key):
if current_node is None or current_node.key == key:
return current_node
if key < current_node.key:
return self._search(current_node.left, key)
else:
return self._search(current_node.right, key)
5. Deleting a Node
The deletion operation removes a node from the tree while maintaining the BST property. Deletion can be more complex due to the need to rearrange the tree depending on whether the node has no children, one child, or two children.
def delete(self, key):
self.root = self._delete(self.root, key)
def _delete(self, current_node, key):
if current_node is None:
return current_node
if key < current_node.key:
current_node.left = self._delete(current_node.left, key)
elif key > current_node.key:
current_node.right = self._delete(current_node.right, key)
else:
# Node with one or no child
if current_node.left is None:
return current_node.right
elif current_node.right is None:
return current_node.left
# Node with two children: Get the inorder successor
successor = self._min_value_node(current_node.right)
current_node.key = successor.key
current_node.right = self._delete(current_node.right, successor.key)
return current_node
def _min_value_node(self, node):
current = node
while current.left is not None:
current = current.left
return current
6. Traversal Methods
Traversal methods like in-order, pre-order, and post-order are essential for various tree operations, including printing the tree’s elements in sorted order or performing other recursive operations.
def in_order_traversal(self, node, result=[]):
if node:
self.in_order_traversal(node.left, result)
result.append(node.key)
self.in_order_traversal(node.right, result)
return result
7. Complete BST Implementation Example
Here’s how you might bring all the parts together:
class Node:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, key):
if self.root is None:
self.root = Node(key)
else:
self._insert(self.root, key)
def _insert(self, current_node, key):
if key < current_node.key:
if current_node.left is None:
current_node.left = Node(key)
else:
self._insert(current_node.left, key)
elif key > current_node.key:
if current_node.right is None:
current_node.right = Node(key)
else:
self._insert(current_node.right, key)
def search(self, key):
return self._search(self.root, key)
def _search(self, current_node, key):
if current_node is None or current_node.key == key:
return current_node
if key < current_node.key:
return self._search(current_node.left, key)
else:
return self._search(current_node.right, key)
def delete(self, key):
self.root = self._delete(self.root, key)
def _delete(self, current_node, key):
if current_node is None:
return current_node
if key < current_node.key:
current_node.left = self._delete(current_node.left, key)
elif key > current_node.key:
current_node.right = self._delete(current_node.right, key)
else:
if current_node.left is None:
return current_node.right
elif current_node.right is None:
return current_node.left
successor = self._min_value_node(current_node.right)
current_node.key = successor.key
current_node.right = self._delete(current_node.right, successor.key)
return current_node
def _min_value_node(self, node):
current = node
while current.left is not None:
current = current.left
return current
def in_order_traversal(self, node, result=[]):
if node:
self.in_order_traversal(node.left, result)
result.append(node.key)
self.in_order_traversal(node.right, result)
return result
# Example usage:
bst = BinarySearchTree()
bst.insert(50)
bst.insert(30)
bst.insert(70)
bst.insert(20)
bst.insert(40)
bst.insert(60)
bst.insert(80)
print("In-order Traversal:", bst.in_order_traversal(bst.root))
print("Search for 70:", bst.search(70).key)
bst.delete(70)
print("In-order Traversal after deleting 70:", bst.in_order_traversal(bst.root))
Implementing a Binary Search Tree is an excellent exercise for understanding fundamental data structures and algorithms. This implementation covers the basic operations, but in real-world applications, you might consider additional optimizations or use self-balancing trees like AVL or Red-Black trees to ensure the tree remains balanced and operations remain efficient.
8. Common Mistakes and Pitfalls
When working with Binary Search Trees (BSTs), especially during implementation or while solving problems, it’s easy to make certain mistakes or fall into common pitfalls. Here are some of the most frequent issues encountered:
1. Ignoring the BST Property
- Mistake: A common mistake is forgetting to maintain the BST property during insertions and deletions. Each node’s left subtree should contain only nodes with values less than the node’s key, and the right subtree should contain only nodes with values greater than the node’s key.
- Consequence: Violating this property can turn your BST into a general binary tree, which loses the efficiency of BST operations like search, insert, and delete.
2. Not Handling Duplicates Properly
- Mistake: Failing to decide how to handle duplicate values when inserting nodes.
- Consequence: This can lead to an unbalanced tree or undefined behavior depending on the implementation. Typically, duplicates are either not allowed or are placed consistently either in the left or right subtree.
3. Incorrectly Implementing Deletion
- Mistake: Deletion in a BST is more complex than insertion or search, especially when the node to be deleted has two children. Incorrectly handling this scenario is a common error.
- Consequence: Mishandling the deletion process can disrupt the BST structure, leading to errors in subsequent operations.
4. Forgetting Edge Cases
- Mistake: Overlooking edge cases such as inserting into an empty tree, deleting the root node, or dealing with nodes that have only one child.
- Consequence: Failure to handle these cases can cause the program to crash or produce incorrect results.
5. Not Managing Memory Properly (in C/C++ Implementations)
- Mistake: In languages like C or C++, failing to properly manage dynamic memory allocation (e.g., not freeing memory after node deletion).
- Consequence: This can lead to memory leaks, which degrade performance over time.
6. Ignoring Tree Balancing
- Mistake: While implementing a BST, not considering the possibility of the tree becoming unbalanced, which can happen if the data is inserted in sorted order.
- Consequence: An unbalanced BST can degenerate into a linked list, resulting in O(n) time complexity for search, insertion, and deletion operations instead of O(log n).
7. Confusion Between Different Tree Types
- Mistake: Confusing BSTs with other types of trees, such as AVL trees or Red-Black trees, which are balanced by definition.
- Consequence: This can lead to incorrect assumptions about the performance and behavior of your BST, especially in terms of balancing.
8. Inefficient Traversals
- Mistake: Implementing traversal methods inefficiently, such as using additional memory unnecessarily or not taking advantage of the BST properties.
- Consequence: This can lead to slower performance, especially for large trees, and can affect the overall efficiency of the tree operations.
9. Ignoring Recursive Stack Depth
- Mistake: Overusing recursion in environments with limited stack memory, especially for deep trees.
- Consequence: This can lead to a stack overflow, crashing the program. In such cases, iterative approaches might be preferred.
10. Misunderstanding Time Complexity
- Mistake: Assuming that all BST operations are always O(log n) without considering the possibility of an unbalanced tree.
- Consequence: This misunderstanding can lead to performance bottlenecks in applications where tree operations are frequent and the tree is not balanced.
Avoiding these common mistakes and pitfalls requires a deep understanding of how BSTs work and careful consideration during implementation. Always test your implementation with various edge cases and consider the long-term implications of your design choices, such as handling duplicates and ensuring the tree remains balanced.
9. Conclusion
Binary Search Trees (BSTs) are fundamental data structures that offer efficient search, insertion, and deletion operations when implemented correctly. They are widely used in various applications, from databases to file systems, due to their ability to maintain a dynamic set of elements in a sorted order.
Understanding the basic operations, properties, and variants of BSTs is crucial for leveraging their full potential. However, one must also be mindful of common mistakes, such as ignoring the BST property or mishandling edge cases, which can lead to inefficient or incorrect implementations.
By mastering the intricacies of BSTs and applying best practices during implementation, you can harness their power to solve complex problems effectively. Whether you’re working on algorithms, building software systems, or optimizing data retrieval processes, a solid grasp of Binary Search Trees will undoubtedly enhance your skill set as a developer.
10. Additional Resources
To further enhance your understanding of Binary Search Trees (BSTs) and their applications, consider exploring the following resources:
1. Books
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein: This textbook provides an in-depth explanation of BSTs, their operations, and related algorithms, making it an excellent resource for both beginners and advanced learners.
- “Data Structures and Algorithm Analysis in C” by Mark Allen Weiss: This book covers BSTs along with other data structures, offering practical examples and code implementations.
2. Online Tutorials and Courses
- Coursera: Data Structures and Algorithms Specialization by UC San Diego & National Research University Higher School of Economics: This course covers a range of data structures, including BSTs, and provides practical coding exercises.
- GeeksforGeeks: Binary Search Tree: A comprehensive collection of articles and tutorials on BST operations, properties, and problem-solving.
3. Code Practice Platforms
- LeetCode: Practice coding problems related to BSTs on LeetCode, which offers a wide range of challenges to test your understanding and implementation skills.
- HackerRank: Another platform that provides coding problems and challenges focused on BSTs and other data structures.
4. Research Papers
- “Balanced Binary Search Trees” by Adelson-Velsky and Landis: Explore the origins and theory behind self-balancing BSTs like AVL trees, which build upon the basic concepts of BSTs.
- “Binary Search Tree and Its Variants: A Survey” by Arman Hekmatpour: This paper offers a detailed overview of various BST variants, their strengths, and their applications.
5. Open Source Projects
- GitHub Repositories: Explore open-source projects on GitHub that involve BSTs. Analyzing and contributing to such projects can provide practical experience and deeper insights into real-world applications of BSTs.
6. Video Lectures
- YouTube: Data Structures and Algorithms in Java by Michael Goodrich: A series of video lectures that covers BSTs and other data structures with detailed explanations and Java implementations.
- MIT OpenCourseWare: Introduction to Algorithms (6.006): A free course offering from MIT that includes lectures on BSTs, providing both theoretical and practical knowledge.
7. Documentation and References
- Python Documentation: Explore Python’s
collections
andbisect
modules, which provide built-in functions for managing sorted data, including binary search operations. - Java Documentation: The Java Collections Framework includes the
TreeMap
andTreeSet
classes, which internally use balanced BSTs. Reviewing these classes’ documentation can be insightful for understanding BST implementations in Java.
By diving into these resources, you can strengthen your understanding of Binary Search Trees, refine your coding skills, and stay updated with the latest advancements in data structure technology.
FAQs
1. What is a Binary Search Tree (BST)?
A Binary Search Tree (BST) is a specialized type of binary tree in which each node contains a key, and all nodes in the left subtree have keys less than the node’s key, while all nodes in the right subtree have keys greater than the node’s key. This property allows for efficient search, insertion, and deletion operations.
2. What are the common operations performed on a Binary Search Tree?
The most common operations performed on a BST include:
- Search: Finding a specific key in the tree.
- Insertion: Adding a new key to the tree while maintaining the BST property.
- Deletion: Removing a key from the tree and re-arranging nodes to maintain the BST property.
- Traversal: Visiting all nodes in a specific order (e.g., in-order, pre-order, post-order).
3. What is the difference between a Binary Tree and a Binary Search Tree?
A Binary Tree is a general tree structure where each node has at most two children, without any specific order. A Binary Search Tree, on the other hand, is a binary tree with an additional property: for any given node, all elements in the left subtree are smaller, and all elements in the right subtree are larger. This ordering in a BST enables efficient searching, insertion, and deletion.
4. What are the advantages of using a Binary Search Tree?
The primary advantages of using a BST include:
- Efficient Searching: BSTs offer average-case time complexity of O(log n) for search operations.
- Dynamic Set Management: BSTs can handle dynamic data sets where elements are frequently inserted or deleted.
- Ordered Data: BSTs maintain elements in sorted order, which is useful for in-order traversal and range queries.
5. How can a Binary Search Tree become unbalanced, and what are the consequences?
A Binary Search Tree becomes unbalanced when the tree’s structure leads to a skewed distribution of nodes, such as when nodes are inserted in a sorted or nearly sorted order. An unbalanced BST can degenerate into a linked list, causing the time complexity for search, insertion, and deletion operations to degrade to O(n) in the worst case. To avoid this, self-balancing BSTs like AVL trees or Red-Black trees are often used.
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
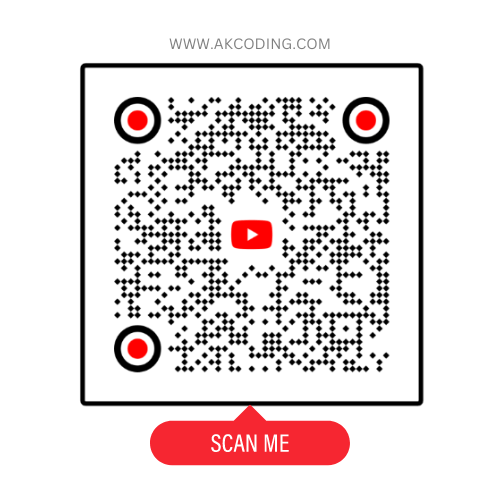