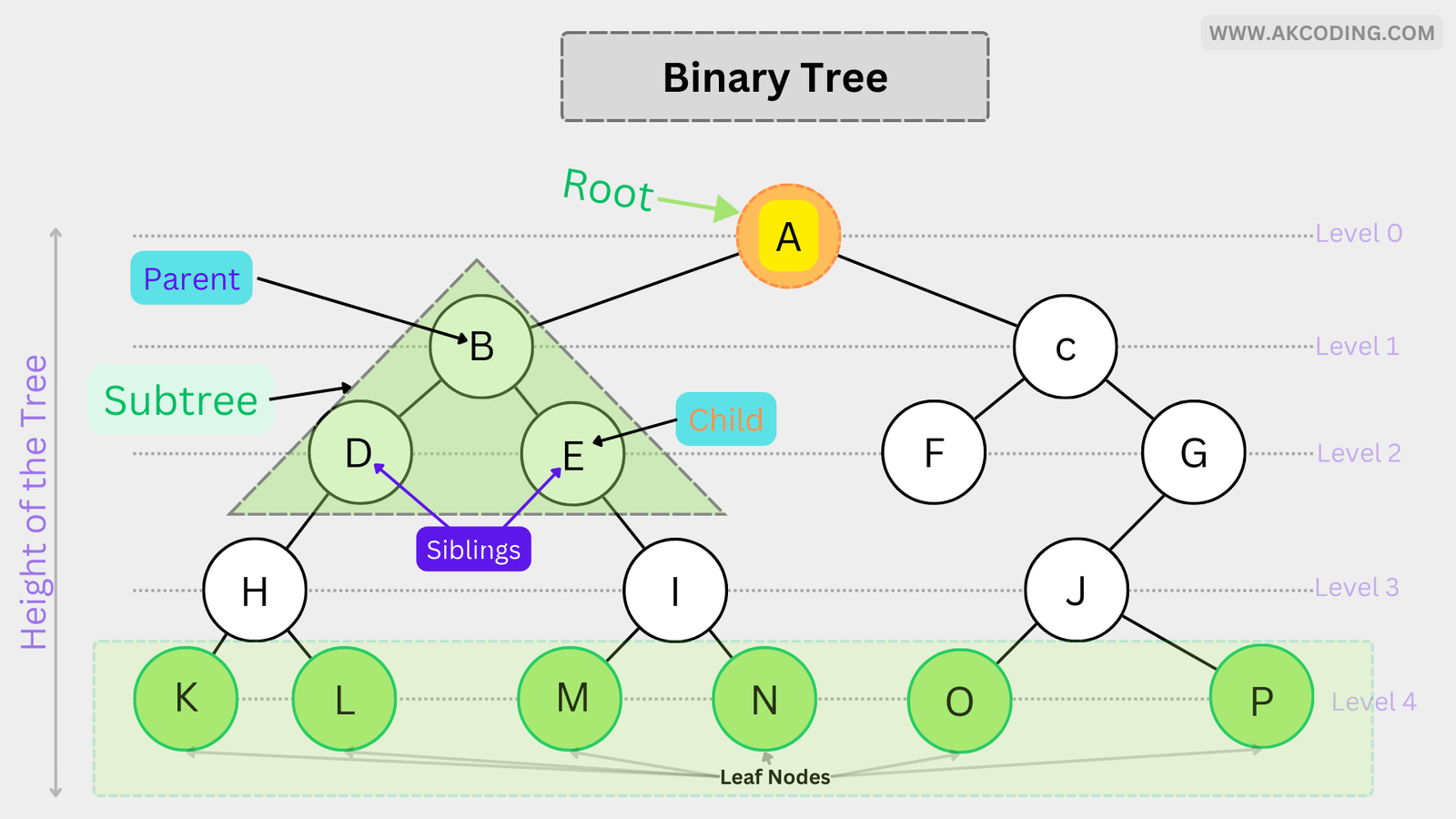
Table of Contents
Introduction
Introduction
A binary tree is a fundamental data structure used in computer science and programming. It is a hierarchical structure with a unique design that allows for efficient data storage and retrieval. Binary trees are often used in algorithms for their flexibility, balance, and various traversal methods.
Definition
A binary tree is a type of tree data structure where each node has at most two children, referred to as the left child and the right child. This structure is rooted, meaning it has a single topmost node called the root, from which all other nodes descend. The edges connecting the nodes represent the relationships within the tree, creating a branching pattern.
Common Uses of Binary Trees
Binary trees are versatile and can be used for a wide range of applications. Here are some common uses:
- Searching and Sorting Algorithms:
- Binary trees form the basis for binary search trees (BSTs), which support efficient operations like search, insertion, and deletion.
- Insertion sort, quicksort, and other sorting algorithms utilize binary trees for their internal operations.
- Hierarchical Data Structures:
- Binary trees can represent hierarchical relationships, such as organizational structures, family trees, or decision trees.
- In computer graphics, they are used to represent scene graphs or spatial hierarchies.
- Expression Trees:
- Binary trees can be used to represent arithmetic expressions, with nodes representing operators and operands. This structure allows for the evaluation of expressions in a specific order (infix, prefix, or postfix).
- Tree Traversals:
- Binary trees support various traversal methods, enabling operations like in-order, pre-order, post-order, and level-order traversals. These methods are crucial in applications like syntax tree traversal, parsing, and file system navigation.
- Binary Heaps and Priority Queues:
- Binary trees serve as the underlying structure for binary heaps, which are used in priority queues and algorithms like heapsort.
- Decision Trees and Machine Learning:
- In machine learning, binary trees form the basis for decision trees and random forests, used for classification and regression tasks.
- Binary trees are employed in rule-based systems for decision-making.
Binary trees are a crucial data structure in computer science, with various applications in algorithms, data processing, and machine learning. Their simple yet versatile structure allows for efficient operations and provides a solid foundation for more complex tree-based algorithms and systems.
Basic Structure
Binary trees are hierarchical data structures in which each node has at most two children, typically referred to as the “left child” and “right child.” Here’s an overview of the basic structure of a binary tree:
1. Node
- A node is a fundamental building block of a binary tree. It contains:
- Data: The value or information stored in the node.
- Left Child: A reference to the left child node.
- Right Child: A reference to the right child node.
2. Root Node
- The root node is the topmost node in a binary tree. It is the starting point for traversals and is typically where insertions begin.
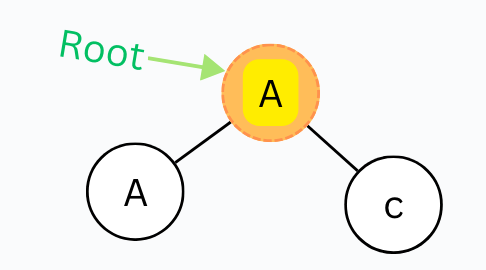
3. Parent and Child Relationships
- In a binary tree, each node can have zero, one, or two children.
- Parent Node: A node that has one or more child nodes.
- Child Node: A node that is a direct descendant of another node. Each child node has a unique parent.
4. Leaf Nodes
- Leaf nodes (also known as terminal nodes) are nodes without any children. They represent the end points of a tree structure.
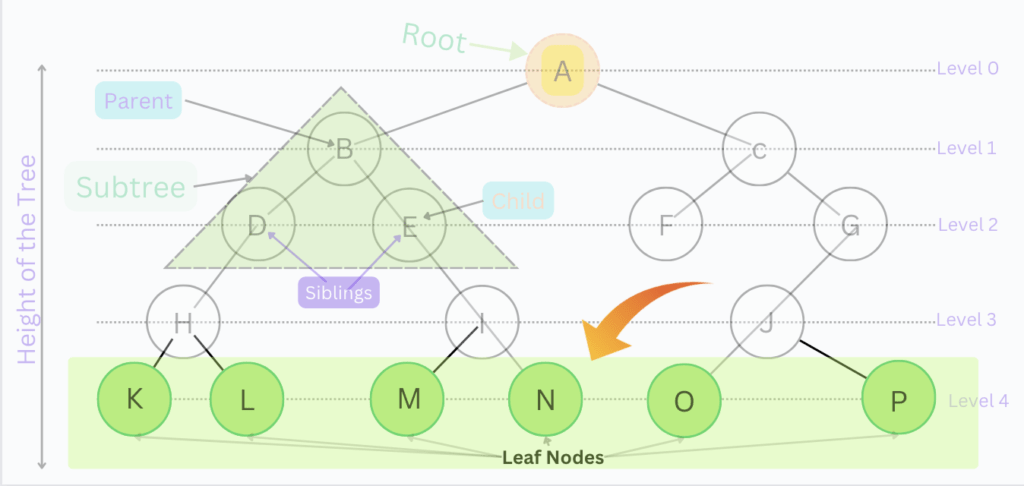
5. Height and Depth
- Height: The height of a node is the length of the longest path from the node to any leaf. The height of a binary tree is the height of the root node.
- Depth: The depth of a node is the length of the path from the root node to that node.
6. Balanced and Unbalanced Trees
- A binary tree is balanced when the height difference between the left and right subtrees of any node is at most one.
- A binary tree is unbalanced when this condition is not met, potentially leading to longer search times or inefficient operations.
7. Subtrees
- A subtree is a tree formed from a node and all its descendants.
- Left Subtree: The subtree rooted at the left child of a node.
- Right Subtree: The subtree rooted at the right child of a node.
Types of Binary Tree
Binary trees are versatile data structures with different variations designed for specific purposes or characteristics. Here’s an overview of the common types of binary trees:
1. Full Binary Tree
- A full binary tree is a binary tree where every node has either zero or two children. It does not contain nodes with only one child.
2. Complete Binary Tree
- A complete binary tree is a binary tree in which all levels, except possibly the last, are completely filled. The nodes in the last level are filled from left to right, with no gaps between them.
3. Perfect Binary Tree
- A perfect binary tree is a binary tree where all interior nodes have two children, and all leaf nodes are at the same level. It is a complete binary tree with all levels fully filled.
4. Balanced Binary Tree
- A balanced binary tree is a binary tree where the height difference between the left and right subtrees for any node is at most one. This ensures that the tree maintains a balanced structure, allowing for efficient operations.
5. Degenerate (Skewed) Binary Tree
- A degenerate binary tree, also known as a skewed binary tree, is a binary tree where each node has only one child. This type of tree resembles a linked list, resulting in longer search times and inefficient operations.
6. Binary Search Tree (BST)
- A binary search tree (BST) is a binary tree where the nodes are arranged in a specific order:
- For each node, the left subtree contains only nodes with values less than the node’s value.
- The right subtree contains only nodes with values greater than or equal to the node’s value.
- This structure allows for efficient search, insertion, and deletion operations.
7. AVL Tree
- An AVL tree is a type of balanced binary search tree where the height difference between the left and right subtrees is at most one for every node. This balance is maintained through rotations during insertion and deletion operations.
8. Red-Black Tree
- A red-black tree is a balanced binary search tree where each node has a color attribute (red or black) to ensure the tree remains balanced. The tree maintains specific properties to balance itself through rotations and color changes during insertions and deletions.
9. Binary Heap
- A binary heap is a binary tree where the nodes maintain a specific order:
- Min-Heap: Each parent node’s value is less than or equal to its children’s values.
- Max-Heap: Each parent node’s value is greater than or equal to its children’s values.
- Binary heaps are used in priority queues and algorithms like heapsort.
10. Splay Tree
- A splay tree is a self-balancing binary search tree that performs a “splay” operation to move recently accessed nodes closer to the root. This helps to optimize frequently accessed nodes over time.
These are the primary types of binary trees, each serving different purposes in data structures, algorithms, and computational processes. Understanding their characteristics and applications is key to utilizing them effectively in various scenarios.
Traversals
Tree traversal refers to the process of visiting each node in a tree data structure in a systematic manner. The purpose of traversal is to access and process each node in the tree, which is essential for various operations such as searching, updating, and performing specific computations on the tree data.
Types of Tree Traversal
There are several methods of tree traversal, each serving different purposes and producing different orders of node visits:
- Depth-First Traversal (DFT):
- In-order Traversal:
- Process: Left subtree, root node, right subtree.
- Application: Retrieves nodes in ascending order for binary search trees.
- Pre-order Traversal:
- Process: Root node, left subtree, right subtree.
- Application: Creates a copy of the tree, used in prefix notation.
- Post-order Traversal:
- Process: Left subtree, right subtree, root node.
- Application: Deletes or processes nodes from bottom-up, used in postfix notation.
- Breadth-First Traversal (BFT):
- Level-order Traversal:
- Process: Visits nodes level by level, starting from the root.
- Application: Shortest path in unweighted graphs, level-wise processing.
Detailed Descriptions
- In-order Traversal:
- Description: Visits the left subtree first, then the root node, and finally the right subtree.
- Example Sequence: For a binary search tree, it visits nodes in non-decreasing order.
- Use Case: Sorting elements, evaluating expressions in expression trees.
- Pre-order Traversal:
- Description: Visits the root node first, then the left subtree, and finally the right subtree.
- Example Sequence: Useful for creating a prefix expression of the tree’s contents.
- Use Case: Replicating trees, evaluating prefix expressions, syntax trees in compilers.
- Post-order Traversal:
- Description: Visits the left subtree first, then the right subtree, and finally the root node.
- Example Sequence: Processes nodes after visiting their children.
- Use Case: Deleting trees, evaluating postfix expressions, bottom-up processing.
- Level-order Traversal:
- Description: Visits nodes level by level from top to bottom and left to right within each level.
- Example Sequence: Visits the root, then all children of the root, then all grandchildren, and so on.
- Use Case: Finding the shortest path, network broadcast, breadth-first search algorithms.
Importance of Tree Traversal
- Data Processing: Enables accessing and processing each node in a tree for various operations like searching, updating, and computing.
- Tree Algorithms: Essential for implementing many tree-based algorithms and applications.
- Hierarchical Data: Efficiently handles hierarchical data structures, making it crucial in databases, file systems, and more.
Tree traversal is fundamental in computer science for working with hierarchical data structures, ensuring that nodes are visited and processed in a desired order based on specific requirements and applications.
Binary Search Tree (BST)
A Binary Search Tree (BST) is a type of binary tree that maintains a specific order property, which makes searching for an element efficient. In a BST, each node has a maximum of two children, referred to as the left child and the right child. The tree is structured such that for any given node:
- The value of all nodes in the left subtree is less than the value of the current node.
- The value of all nodes in the right subtree is greater than the value of the current node.
This property makes it straightforward to perform operations such as insertion, deletion, and searching, as the tree’s structure ensures a sorted sequence of elements.
Properties of a Binary Search Tree
- Binary Tree Structure:
- Each node in a BST has at most two children.
- The left child contains values less than its parent node.
- The right child contains values greater than its parent node.
- Ordering Property:
- For any node ( N ):
- All values in the left subtree of ( N ) are less than the value of ( N ).
- All values in the right subtree of ( N ) are greater than the value of ( N ).
- This property must hold true for every node in the tree.
- Unique Values:
- Typically, BSTs do not contain duplicate values. Each value in the tree must be unique to maintain the integrity of the ordering property.
- Recursive Definition:
- A BST can be recursively defined. Each node is itself a BST, where:
- The left subtree is a BST.
- The right subtree is a BST.
- This recursive nature is utilized in many BST algorithms.
- Traversal Methods:
- In-order Traversal: Visits nodes in ascending order. Left subtree → Node → Right subtree.
- Pre-order Traversal: Visits the root node first. Node → Left subtree → Right subtree.
- Post-order Traversal: Visits the root node last. Left subtree → Right subtree → Node.
- Height and Balance:
- The height of a BST is determined by the longest path from the root to a leaf.
- A BST can become unbalanced if the nodes are inserted in a particular order, leading to a height of ( O(n) ) in the worst case (e.g., inserting sorted elements).
- Balancing techniques (e.g., AVL trees, Red-Black trees) are used to maintain a balanced BST and ensure ( O(\log n) ) height.
- Search, Insertion, and Deletion:
- Search: Starts at the root and compares the target value with the current node. Moves left if the target is less, and right if greater, until the target is found or a leaf is reached.
- Time Complexity: ( O(\log n) ) on average; ( O(n) ) in the worst case.
- Insertion: Similar to search, but inserts the new value in the appropriate location when a null position is found.
- Time Complexity: ( O(\log n) ) on average; ( O(n) ) in the worst case.
- Deletion: More complex; involves three cases:
- Node to be deleted is a leaf.
- Node to be deleted has one child.
- Node to be deleted has two children (requires finding the in-order predecessor or successor).
- Time Complexity: ( O(\log n) ) on average; ( O(n) ) in the worst case.
- Balanced vs. Unbalanced BSTs:
- Balanced BSTs (e.g., AVL Trees, Red-Black Trees) maintain a balanced height to ensure efficient operations.
- Unbalanced BSTs can degrade to linear structures, causing operations to become inefficient.
Summary
A Binary Search Tree (BST) is a powerful data structure that organizes elements in a hierarchical, sorted manner, allowing for efficient search, insertion, and deletion operations. Its properties, such as the binary tree structure and ordering property, make it a fundamental structure in computer science. However, maintaining balance is crucial for ensuring optimal performance, leading to the development of balanced BSTs for better efficiency in real-world applications.
Advanced Concepts
- AVL Trees: balanced binary search trees
- Red-Black Trees: balanced binary search trees with color properties
- Splay Trees: self-balancing binary search trees
- B-Trees: multi-level balanced search trees for large datasets
Operations on Binary Trees
- Insertion
- Deletion
- Searching
- Finding the minimum and maximum values
- Checking tree balance
Applications of Binary Tree
Binary trees are fundamental data structures in computer science and have a wide range of applications across various domains. Here are some of the key applications of binary trees:
1. Binary Search Trees (BST) for Searching and Sorting
- Efficient Searching: Binary search trees allow for quick searches with a time complexity of O(log n) in a balanced tree. This makes them useful for applications that require frequent data retrieval.
- Insertion and Deletion: BSTs also enable efficient insertion and deletion of elements, ideal for dynamic datasets.
- Example: Databases often use BSTs for indexing and querying data.
2. Expression Trees for Mathematical Expressions
- Representing Expressions: Expression trees are binary trees where nodes represent operators and leaves represent operands. They are used in compilers and interpreters to parse and evaluate mathematical expressions.
- Example: An expression tree can represent an arithmetic expression like
(a + b) * c
.
3. Decision Trees for Decision-Making and Machine Learning
- Decision Structures: Decision trees are used in business and machine learning to represent decision-making processes. Each node represents a decision point, with branches leading to different outcomes.
- Applications: Decision trees are employed in AI for classification, regression, and rule-based systems. Random forests, which consist of multiple decision trees, are widely used in machine learning.
4. Binary Heaps for Priority Queues and Heapsort
- Priority Queues: Binary heaps are used to implement priority queues, where elements are ordered by priority. This is useful in scheduling and task management applications.
- Heapsort: A sorting algorithm that uses binary heaps to sort data efficiently.
- Example: Priority queues are used in operating systems for job scheduling and event handling.
5. File Systems and Directory Structures
- Hierarchical Organization: Binary trees can represent hierarchical structures like file systems, where directories contain subdirectories and files.
- Example: Navigating a file system can be done using binary tree traversal methods.
6. Syntax Trees for Parsing and Compilers
- Parsing Code: Syntax trees are used in compilers to represent the structure of programming languages. They help in parsing code and generating intermediate representations.
- Example: Abstract syntax trees (ASTs) are used in compilers to understand the grammar of programming languages.
7. Spatial Trees for Computer Graphics and Spatial Data
- Spatial Relationships: Binary trees can represent spatial relationships in computer graphics and geographical information systems (GIS).
- Example: Quadtrees and binary space partitioning (BSP) trees are used in computer graphics to optimize rendering and collision detection.
8. Network Routing and Communication
- Efficient Routing: Binary trees can represent network topologies, enabling efficient routing and communication.
- Example: Binary trees are used in data networks for hierarchical routing and broadcast systems.
These are some of the key applications of binary trees, demonstrating their versatility and importance in computer science, algorithms, and various domains where hierarchical or efficient data operations are required.
Conclusion
Summary of Key Points
Binary trees are foundational data structures in computer science, characterized by nodes that can have at most two children—referred to as the left child and right child. They offer a flexible and efficient framework for a variety of operations, including searching, insertion, deletion, and traversal.
Here are some key points to remember about binary trees:
- Structure: A binary tree is rooted with nodes connected through parent-child relationships. The root is the topmost node, and leaves are nodes with no children.
- Types: Binary trees can be full, complete, perfect, balanced, or skewed. Specific variations like binary search trees (BSTs), AVL trees, and red-black trees offer unique properties for efficiency.
- Traversals: Binary trees support multiple traversal methods, including in-order, pre-order, post-order, and level-order, each with specific uses.
- Applications: Binary trees are used in a wide range of applications, such as data structures, algorithms, decision-making, priority queues, compilers, and file systems.
Additional Resources for Further Learning
To delve deeper into binary trees and related concepts, consider exploring the following resources:
- Books:
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein: A comprehensive book on algorithms with detailed sections on binary trees and related operations.
- “Data Structures and Algorithm Analysis” by Mark Allen Weiss: A thorough exploration of various data structures, including binary trees and their applications.
- Online Courses:
- Coursera: Offers courses on data structures and algorithms, including binary trees, from reputable institutions.
- edX: Provides similar courses on computer science fundamentals, including binary trees.
- Research Papers and Journals:
- Search for papers on binary tree applications in specific domains (e.g., machine learning, databases, computer graphics) through academic journals or databases like Google Scholar.
- Online Tutorials and Articles:
- Websites like GeeksforGeeks and Medium have a wealth of articles and tutorials on binary trees, explaining concepts in a practical, easy-to-understand manner.
These resources offer a comprehensive view of binary trees and related concepts, supporting both academic learning and practical application in real-world scenarios.
FAQs
What is binary tree
A binary tree is a hierarchical data structure where each node has at most two children, known as the left and right child. The topmost node is the root, from which all other nodes descend. Each node contains data and links to its children, if any. Binary trees are used for various operations such as insertion, deletion, and traversal (in-order, pre-order, post-order, or level-order). Variations include full, complete, perfect, balanced, and skewed trees. Applications range from binary search trees for efficient data retrieval to decision trees in machine learning and heaps for priority queues and sorting algorithms.
Threaded binary tree
A threaded binary tree is a variant of a binary tree in which “threads” replace null pointers, allowing for efficient in-order traversal without additional stack or recursion overhead. In a traditional binary tree, nodes with no children have null pointers, indicating the absence of left or right child nodes. In a threaded binary tree, these null pointers are replaced with references (or “threads”) to the next node in the in-order sequence. This threading enables traversal of the entire tree with fewer resources, offering a space-efficient way to traverse and process nodes in a specific order without external data structures.