Java Interview Questions on Multithreading and Concurrency. Here are some advanced interview questions on multithreading and concurrency suitable for candidates with 10 years of Java experience
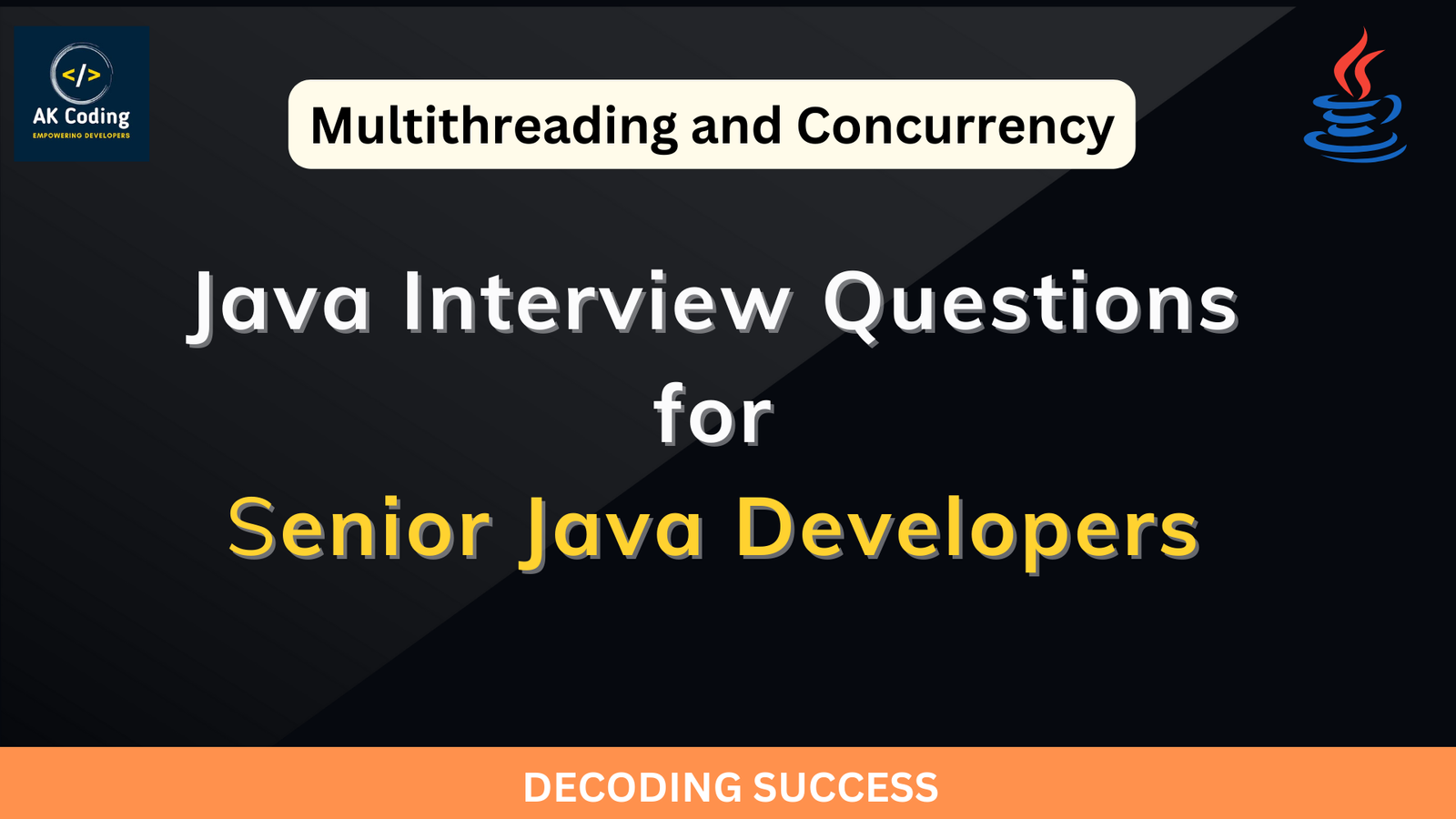
Java Interview Questions
Table of Contents
1. Can you explain the difference between parallelism and concurrency in the context of multithreading?
In the context of multithreading, parallelism and concurrency are related but distinct concepts.
Concurrency refers to the ability of a system to execute multiple tasks concurrently, allowing tasks to make progress in overlapping time periods. Concurrency does not necessarily imply parallel execution; it can be achieved even on a single processor through context switching among threads. Concurrency is essential for improving responsiveness and resource utilization in applications by enabling tasks to execute independently and concurrently.
Parallelism, on the other hand, involves the simultaneous execution of multiple tasks, ideally on multiple processors or cores, to improve performance and efficiency. It requires true simultaneous execution of tasks, typically achieved through hardware support or distributed computing environments. Parallelism is beneficial for tasks that can be divided into smaller independent units of work that can be executed simultaneously, resulting in faster execution times and increased throughput.
In summary, concurrency enables tasks to overlap in time, while parallelism enables tasks to execute simultaneously across multiple processing units.
2. How do you ensure thread safety in a multithreaded environment? Discuss some common techniques and mechanisms
Ensuring thread safety in a multithreaded environment is crucial to prevent data corruption and race conditions. Several common techniques and mechanisms can be employed:
- Synchronization: The use of synchronization mechanisms such as locks (synchronized blocks or explicit locks like ReentrantLock) ensures that only one thread can access a critical section of code or resource at a time, preventing concurrent modification and maintaining consistency.
- Atomic Operations: Atomic operations provided by classes like AtomicInteger and AtomicReference ensure that certain operations are performed atomically without interference from other threads, avoiding race conditions.
- Immutable Objects: Designing immutable objects that cannot be modified after creation eliminates the need for synchronization altogether, ensuring thread safety by design.
- Thread-Local Storage: Utilizing thread-local variables ensures that each thread has its own independent copy of data, avoiding contention and ensuring thread safety without synchronization.
- Concurrent Data Structures: Leveraging thread-safe data structures provided by the java.util.concurrent package, such as ConcurrentHashMap and ConcurrentLinkedQueue, allows for safe concurrent access to shared data without explicit synchronization.
By applying these techniques judiciously, developers can ensure thread safety in multithreaded environments, facilitating scalable and reliable concurrent applications.
3. Explain the difference between intrinsic locks and explicit locks in Java. When would you use one over the other?
In Java, intrinsic locks and explicit locks are both mechanisms for achieving synchronization in multithreaded environments, but they differ in their usage and features.
Intrinsic Locks:
- Intrinsic locks are also known as monitor locks and are built into the Java language.
- They are acquired implicitly using the
synchronized
keyword, which marks a block of code or a method as synchronized. - Intrinsic locks are simple to use and understand, making them suitable for basic synchronization needs.
- However, they have limited flexibility and features compared to explicit locks.
Explicit Locks:
- Explicit locks, represented by classes like
ReentrantLock
, offer more flexibility and control over locking. - They provide additional features such as the ability to specify lock acquisition order, non-blocking attempts to acquire locks, and timed lock acquisition.
- Explicit locks are suitable for complex synchronization requirements or when finer-grained locking is needed.
In summary, use intrinsic locks for simple synchronization needs where ease of use is prioritized, and opt for explicit locks when more advanced features and flexibility are required, such as in complex synchronization scenarios or performance-critical applications.
4. What is the purpose of the java.util.concurrent package? Can you give examples of classes and interfaces provided by this package?
The java.util.concurrent
package in Java provides a comprehensive set of high-level concurrency utilities and building blocks for developing multithreaded applications efficiently and effectively. Its purpose is to simplify concurrent programming by offering thread-safe data structures, synchronization primitives, and utilities for asynchronous computation and coordination.
Examples of classes and interfaces provided by the java.util.concurrent
package include:
- Executor Framework: Interfaces like
Executor
,ExecutorService
, andScheduledExecutorService
facilitate the execution of tasks asynchronously and manage thread pools efficiently. - Concurrent Data Structures: Classes like
ConcurrentHashMap
,ConcurrentSkipListMap
,ConcurrentSkipListSet
, andConcurrentLinkedQueue
provide thread-safe implementations of common data structures, enabling safe concurrent access by multiple threads without external synchronization. - Synchronization Utilities: Classes like
CountDownLatch
,CyclicBarrier
,Semaphore
, andPhaser
offer synchronization primitives for coordinating the execution of multiple threads and managing complex synchronization scenarios.
Overall, the java.util.concurrent
package simplifies concurrent programming in Java by providing a rich set of concurrency utilities that address common multithreading challenges and promote safe and efficient concurrent application development.
Thread pooling is a technique used in multithreaded applications to manage a pool of reusable threads, which are pre-initialized and kept alive for the duration of the application. Instead of creating and destroying threads for each task, thread pooling reuses existing threads from the pool, reducing the overhead associated with thread creation and termination.
The advantages of thread pooling include:
- Improved Performance: Thread pooling reduces the overhead of thread creation and termination, resulting in faster task execution and improved application responsiveness.
- Resource Management: By limiting the number of concurrent threads, thread pooling prevents resource exhaustion and contention, ensuring efficient utilization of system resources.
- Scalability: Thread pools can be dynamically sized to match the workload, allowing applications to scale gracefully with varying levels of concurrency.
6. Explain the Java Memory Model (JMM) and its significance in multithreaded programming. How does it ensure memory visibility and ordering?
The Java Memory Model (JMM) defines the rules and semantics governing how threads interact through shared memory in a Java program. It ensures memory consistency, visibility, and ordering across threads by specifying the behaviors of variables, synchronization primitives, and memory operations.
JMM ensures memory visibility by guaranteeing that changes made by one thread to shared variables are visible to other threads in a predictable and consistent manner. It achieves this through concepts like happens-before relationships, memory barriers, and program order. For example, actions inside synchronized blocks have a happens-before relationship, ensuring that changes made by one thread are visible to subsequent synchronized actions by other threads.
Additionally, JMM defines the ordering of memory operations, ensuring that reads and writes to shared variables are ordered in a way that preserves program semantics. This ensures that the execution of a program produces consistent and predictable results, even in a multithreaded environment.
7. What are some common concurrency pitfalls or anti-patterns you’ve encountered in your experience, and how did you address them?
Common concurrency pitfalls include race conditions, deadlock, livelock, and thread starvation.
To address them, I’ve used techniques such as proper synchronization, deadlock detection and prevention strategies, thread pool tuning, and careful resource allocation.
8. Discuss the advantages and disadvantages of using the Fork/Join Framework for parallel programming in Java.
The Fork/Join Framework is a powerful tool for parallel programming in Java, offering several advantages and disadvantages:
Advantages:
- Ease of Use: The Fork/Join Framework simplifies parallel programming by allowing developers to express parallel algorithms in a recursive divide-and-conquer style, making it easier to write and understand parallel code.
- Automatic Work Stealing: The framework employs a work-stealing algorithm, where idle threads steal tasks from other threads’ queues, ensuring efficient load balancing and utilization of resources across multiple processors.
- Scalability: The Fork/Join Framework can scale well with the number of available processors, as it dynamically adapts to the system’s capabilities and workload, providing optimal performance for different hardware configurations.
Disadvantages:
- Limited Applicability: The Fork/Join Framework is best suited for embarrassingly parallel problems that can be decomposed into smaller independent tasks. It may not be suitable for all types of parallel algorithms or irregular computation patterns.
- Overhead: The framework incurs overhead for task decomposition, synchronization, and task-stealing, which can impact performance, especially for small, fine-grained tasks.
- Debugging Complexity: Debugging parallel programs using the Fork/Join Framework can be challenging due to the recursive nature of tasks and non-deterministic execution order, making it harder to identify and diagnose issues.
Overall, while the Fork/Join Framework offers simplicity, automatic load balancing, and scalability for parallel programming in Java, it may not be suitable for all scenarios and may introduce overhead and debugging complexities in certain cases.
9. How do you handle deadlock situations in multithreaded applications? Can you discuss some deadlock prevention and resolution strategies?
Deadlock situations in multithreaded applications occur when two or more threads are blocked indefinitely, each waiting for the other to release a resource, resulting in a stalemate. To handle deadlocks and prevent them from occurring, several strategies can be employed:
- Lock Ordering: Establish a global ordering of locks and ensure that threads always acquire locks in the same order to prevent circular dependencies and deadlock scenarios.
- Timeouts: Implement timeouts on lock acquisition attempts to prevent threads from waiting indefinitely, allowing them to break out of potential deadlock situations and recover gracefully.
- Resource Allocation Graph: Use a resource allocation graph to detect potential deadlocks by identifying cycles in the graph, then employ strategies like resource preemption or transaction rollback to resolve them.
- Avoidance: Avoid acquiring multiple locks simultaneously if possible, or use higher-level synchronization primitives like read/write locks or transactional memory to reduce the likelihood of deadlocks.
By adopting these prevention and resolution strategies, developers can mitigate the risk of deadlock occurrences and ensure the stability and reliability of multithreaded applications.
10. Can you explain the concept of thread-local storage (TLS) and its use cases in multithreaded applications?
Thread-local storage (TLS) is a mechanism in multithreaded programming that allows each thread to have its own independent copy of data, isolated from other threads. TLS provides a way to associate thread-specific data with each thread, ensuring that changes made to the data by one thread do not affect other threads. This is achieved by allocating a separate memory region for each thread to store its thread-local variables or data.
Use cases for TLS in multithreaded applications include:
- Thread-Specific State: Maintaining thread-specific state or context information, such as user sessions or request context, without the need for synchronization.
- Performance Optimization: Storing thread-specific cached data or frequently accessed variables to improve performance by reducing contention on shared resources.
- Contextual Logging: Logging thread-specific information, such as thread IDs or diagnostic data, in a thread-safe manner without synchronization overhead.
Overall, TLS provides a lightweight and efficient mechanism for managing thread-specific data in multithreaded applications, enhancing performance and concurrency.
These interview questions are designed to gauge the candidate’s depth of knowledge and practical experience in multithreading and concurrency, specifically tailored for individuals with 10 years of experience.
Java Threads Official Documentation
For Other Awsome Article visit AKCODING.COM