Kotlin Interview Questions for Senior Developer. Here are the top 20 interview questions along with answers for a senior Kotlin developer
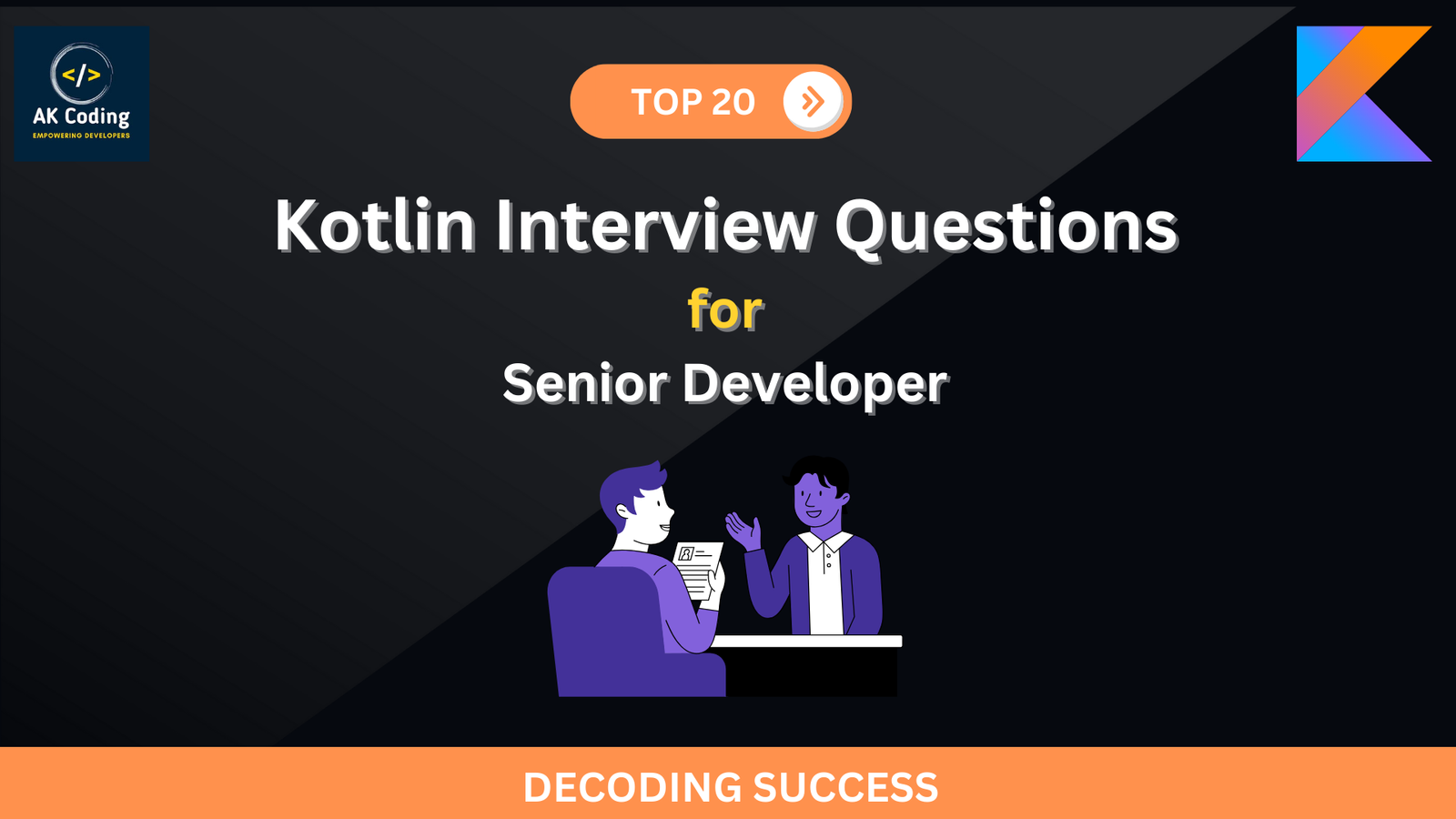
Table of Contents
Kotlin Interview Questions for Senior Developer
1. What are the key features of Kotlin?
- Concise Syntax: Kotlin reduces boilerplate code, making it more concise compared to Java. This improves code readability and developer productivity.
- Null Safety: Kotlin’s type system distinguishes between nullable and non-nullable types, preventing null pointer exceptions at compile time. This feature enhances code reliability and reduces crashes.
- Functional Programming Support: Kotlin supports functional programming paradigms with features like higher-order functions, lambda expressions, and immutable data structures. This enables writing expressive and concise code.
- Coroutines: Kotlin provides coroutines for asynchronous and concurrent programming. Coroutines simplify asynchronous code with structured concurrency and suspend functions, making it easier to write and maintain asynchronous code.
- Extension Functions: Kotlin allows adding new functionality to existing classes without modifying their source code through extension functions. This enhances code modularity and readability.
- Data Classes: Kotlin’s data classes are used to represent immutable data models. They automatically generate
equals()
,hashCode()
,toString()
, andcopy()
methods based on the properties declared in the primary constructor. - Interoperability with Java: Kotlin is fully interoperable with Java, allowing seamless integration with existing Java codebases, libraries, and frameworks. This facilitates the gradual adoption of Kotlin in projects and makes it easy for Java developers to transition to Kotlin.
- Smart Casts: Kotlin’s smart casts automatically cast variables after type checks, reducing the need for explicit casting and improving code readability.
- Type Inference: Kotlin’s type inference system automatically infers the types of variables, reducing the need for explicit type declarations and making the code more concise.
- Immutable Collections: Kotlin provides immutable collections (e.g., lists, sets, maps) by default, promoting functional programming practices and reducing the risk of accidental mutation.
These features make Kotlin a modern and expressive programming language suitable for a wide range of applications, including Android development, web development, backend systems, and more.
2. How does Kotlin handle null safety?
Kotlin handles null safety through its type system, which distinguishes between nullable and non-nullable types. In Kotlin, every variable type is non-nullable by default, meaning it cannot hold a null value unless explicitly specified. To denote that a variable can hold a null value, you append a ?
to its type declaration, creating a nullable type.
Kotlin provides several operators and language constructs to work with nullable types safely:
- Safe Call Operator (?.): Allows you to access properties or call methods on a nullable object. If the object is null, the expression evaluates to null instead of throwing a NullPointerException.
val length: Int? = str?.length
- Elvis Operator (?:): Provides a default value in case a nullable expression evaluates to null.
val length: Int = str?.length ?: -1
- Safe Cast Operator (as?): Allows you to safely cast an object to a nullable type. If the cast is not possible, the result is null.
val strLength: Int? = str as? String
- Not-Null Assertion (!!): Asserts that an expression is not null. If the expression is null, it throws a NullPointerException.
val length: Int = str!!.length
Additionally, Kotlin’s compiler performs compile-time null checks to detect potential null pointer exceptions. This helps catch null-related errors early in the development process, reducing the likelihood of runtime crashes. Overall, Kotlin’s null safety features promote safer and more robust code, mitigating one of the most common sources of bugs in software development.
3. Explain higher-order functions in Kotlin.
Higher-order functions are functions that can accept other functions as parameters and/or return functions as results. In Kotlin, functions are treated as first-class citizens, meaning they can be assigned to variables, passed as arguments, and returned from other functions, just like any other type of value.
Here’s an example of a higher-order function in Kotlin:
fun operation(x: Int, y: Int, op: (Int, Int) -> Int): Int {
return op(x, y)
}
In this example, the operation
function takes two integer parameters (x
and y
) and a function parameter op
. This op
parameter is a higher-order function that takes two integers as input and returns an integer. The operation
function then calls the op
function with the provided x
and y
parameters and returns the result.
You can use this operation
function to perform various operations, such as addition, subtraction, multiplication, or any custom operation defined by the caller:
val resultAdd = operation(5, 3) { a, b -> a + b }
val resultSubtract = operation(5, 3) { a, b -> a - b }
val resultMultiply = operation(5, 3) { a, b -> a * b }
println("Result of addition: $resultAdd") // Output: Result of addition: 8
println("Result of subtraction: $resultSubtract") // Output: Result of subtraction: 2
println("Result of multiplication: $resultMultiply") // Output: Result of multiplication: 15
In this example, we pass lambda expressions as arguments to the operation
function to perform addition, subtraction, and multiplication. This demonstrates how higher-order functions enable flexibility and code reusability by allowing functions to be treated as values.
4. What are coroutines in Kotlin?
Coroutines in Kotlin are a concurrency design pattern that allow for asynchronous and non-blocking programming. They provide a way to perform long-running tasks efficiently without blocking the main thread or wasting resources by creating a large number of threads.
Coroutines are lightweight threads that can be suspended and resumed asynchronously. They are designed to be more efficient than traditional threads, as they can be multiplexed onto a smaller number of system threads. This allows coroutines to scale much better than threads, especially in scenarios with a large number of concurrent tasks.
In Kotlin, coroutines are built on top of suspending functions, which are functions that can be paused and resumed later. Coroutines can suspend execution at a particular point and yield control back to the calling code without blocking the thread. This allows other coroutines or tasks to run while waiting for an operation to complete, such as network requests or disk I/O.
Coroutines provide a structured concurrency model, allowing developers to easily manage and coordinate asynchronous tasks. They can be used to write asynchronous code in a sequential and intuitive manner, similar to synchronous code, while still benefiting from the performance and scalability advantages of asynchronous programming.
Overall, coroutines in Kotlin offer a powerful and flexible way to handle asynchronous tasks, making it easier to write efficient and responsive applications.
5. How does Kotlin support extension functions?
Kotlin supports extension functions, which allow developers to add new functionality to existing classes without modifying their source code. Extension functions are defined outside of the class they extend but can be called as if they were methods of the class.
Here’s how extension functions work in Kotlin:
- Syntax: To define an extension function, you prefix its name with the name of the class it extends and use the
.
operator before the function name. Thethis
keyword inside the function refers to the instance of the class being extended.
fun String.addExclamation(): String {
return "$this!"
}
- Usage: Once an extension function is defined, you can call it on instances of the extended class as if it were a regular method of the class.
val greeting = "Hello"
val excitedGreeting = greeting.addExclamation()
println(excitedGreeting) // Output: Hello!
- Scope: Extension functions are resolved statically based on the declared type of the variable or expression they are called on. They do not actually modify the original class or affect its inheritance hierarchy.
- Nullable Receivers: Extension functions can be defined for nullable types as well. However, they must check for nullability inside the function body if they access properties or methods of the receiver object.
fun String?.safeLength(): Int {
return this?.length ?: 0
}
val str: String? = "Hello"
val length = str.safeLength()
println(length) // Output: 5
- Scoping Functions: Kotlin also provides scoping functions like
run
,with
,let
,also
, andapply
, which can be used to further extend the capabilities of objects in a concise and expressive manner.
Extension functions are a powerful feature of Kotlin that promotes code modularity, reusability, and readability. They allow developers to enhance existing classes with additional functionality without the need to modify their source code, making it easier to work with third-party libraries and framework classes.
6. What is the significance of data classes in Kotlin?
Data classes in Kotlin are specifically designed for representing data in a concise and immutable way. They offer several advantages that make them significant:
- Automatic Generation of Methods: Data classes automatically generate
equals()
,hashCode()
,toString()
,componentN()
functions, and acopy()
function based on the properties declared in the primary constructor. This eliminates the need for boilerplate code, making data manipulation more convenient and less error-prone. - Readability and Maintainability: By providing a concise way to define immutable data models, data classes improve code readability and maintainability. They clearly express the intent of the code and reduce verbosity, making it easier for developers to understand and work with data structures.
- Immutability: Properties declared in data classes are immutable by default, meaning their values cannot be changed after initialization. This promotes a functional programming style and helps prevent unintended side effects, leading to safer and more predictable code.
- Destructuring Declarations: Data classes support destructuring declarations, allowing objects to be easily deconstructed into their component properties. This feature simplifies pattern matching and makes it easier to work with data in Kotlin.
- Interoperability: Data classes seamlessly integrate with other Kotlin language features, such as type inference, extension functions, and higher-order functions. They also support interoperability with Java, making it easy to work with Java libraries and frameworks in Kotlin projects.
Overall, data classes play a crucial role in Kotlin development by providing a convenient and efficient way to define and manipulate immutable data structures. They help improve code quality, reduce boilerplate, and enhance developer productivity.
7. How does Kotlin ensure type safety?
Kotlin ensures type safety through its static type system, which performs compile-time checks to detect and prevent type-related errors. Here are several ways Kotlin ensures type safety:
- Nullable Types: Kotlin’s type system distinguishes between nullable and non-nullable types. By default, variables are non-nullable, meaning they cannot hold null values unless explicitly specified. This prevents null pointer exceptions at runtime and ensures that variables are only assigned values compatible with their declared types.
- Null Safety Operators: Kotlin provides operators like
?.
,?:
, and!!
to safely handle nullable types. These operators allow developers to safely access properties and methods of nullable objects, provide default values for null expressions, or assert non-nullability when necessary. - Smart Casts: Kotlin’s smart casts automatically cast variables after type checks, eliminating the need for explicit casting in most cases. This ensures that variables are used safely and consistently without risking ClassCastExceptions.
- Type Inference: Kotlin’s type inference system automatically infers the types of variables based on their initialization values. This reduces the need for explicit type declarations, making code more concise and readable while still ensuring type safety at compile-time.
- Sealed Classes: Sealed classes in Kotlin represent restricted class hierarchies, where all subclasses must be defined within the same file. This ensures that exhaustive when expressions are used to handle instances of sealed classes, improving type safety and preventing unexpected behavior.
- Extension Functions: Kotlin’s extension functions allow developers to extend existing classes with new functionality without modifying their source code. This promotes code modularity and reuse while preserving type safety, as extension functions are resolved statically based on the declared type of the receiver object.
Overall, Kotlin’s type system is designed to catch type-related errors early in the development process, at compile-time rather than runtime. By enforcing type safety at compile-time and providing tools to handle nullable types safely, Kotlin helps developers write more robust and reliable code.
8. What is tail recursion optimization in Kotlin?
Tail recursion optimization (TRO) is a compiler optimization technique used in Kotlin (as well as in many other programming languages) to optimize tail-recursive functions.
In a tail-recursive function, the recursive call is the last operation performed within the function, and its result is immediately returned. This allows the compiler to optimize the function by reusing the current stack frame for the next recursive call, rather than creating a new stack frame for each recursive call.
By eliminating unnecessary stack frame creation, tail recursion optimization reduces the risk of stack overflow errors and improves the efficiency of recursive algorithms.
In Kotlin, you can mark a function as tail-recursive using the tailrec
modifier. This tells the compiler to apply tail recursion optimization to the function, if possible. If the function doesn’t meet the criteria for tail recursion (i.e., if the recursive call isn’t in the tail position), the compiler will generate a compile-time error.
Here’s an example of a tail-recursive function in Kotlin:
tailrec fun factorial(n: Int, accumulator: Int = 1): Int {
return if (n == 0) accumulator else factorial(n - 1, n * accumulator)
}
In this example, the factorial
function is tail-recursive because the recursive call factorial(n - 1, n * accumulator)
is the last operation performed in the function, and its result is immediately returned. As a result, the Kotlin compiler can optimize this function for tail recursion, improving its performance and avoiding stack overflow errors for large values of n
.
9. How does Kotlin support functional programming?
Kotlin provides robust support for functional programming paradigms, offering several features that facilitate functional-style programming:
- Higher-order functions: Kotlin treats functions as first-class citizens, allowing them to be passed as arguments to other functions and returned as values from functions. This enables the use of higher-order functions, which can accept other functions as parameters or return functions as results.
- Lambda expressions: Kotlin supports concise syntax for defining anonymous functions, known as lambda expressions. Lambda expressions allow for the creation of inline function literals, making it easier to work with higher-order functions and functional constructs.
- Immutable data structures: Kotlin encourages immutability by providing immutable collections (e.g., lists, sets, maps) by default. Immutable data structures facilitate functional programming practices by ensuring that data remains unchanged after creation, leading to safer and more predictable code.
- Extension functions: Kotlin’s extension functions enable the addition of new functionality to existing classes without modifying their source code. This promotes code modularity and reusability, supporting functional programming principles.
- Sequences and streams: Kotlin provides
Sequence
andStream
API for lazily evaluated collections. Sequences and streams enable efficient processing of large datasets by allowing operations to be performed on elements only when necessary, promoting functional-style data manipulation. - Pattern matching: Kotlin offers pattern matching capabilities through the
when
expression, which allows for matching values against a set of patterns and executing corresponding code blocks. Pattern matching enhances the expressiveness and readability of Kotlin code, facilitating functional programming patterns. - Inline functions: Kotlin supports inline functions, which allow function bodies to be copied directly into the call site at compile time. Inline functions can improve performance and facilitate functional programming constructs such as higher-order functions and lambda expressions.
- Null safety: Kotlin’s null safety features, including nullable and non-nullable types, promote functional programming practices by providing a type system that prevents null pointer exceptions at compile time. This encourages the use of safe and predictable code patterns, enhancing the functional programming experience in Kotlin.
Overall, Kotlin’s support for functional programming enables developers to write expressive, concise, and maintainable code by leveraging functional programming paradigms and constructs. These features make Kotlin well-suited for developing functional-style applications and libraries.
10. What are sealed classes in Kotlin?
Sealed classes in Kotlin are used to represent restricted class hierarchies where the set of subclasses is known and limited. Unlike regular classes, which can be subclassed freely, sealed classes restrict the inheritance hierarchy to a predefined set of subclasses, all of which must be declared within the same file as the sealed class itself.
The primary purpose of sealed classes is to enable exhaustive pattern matching, especially in combination with the when
expression. Since the compiler knows all possible subclasses of a sealed class, it can enforce exhaustive checks when using a when
expression, ensuring that all possible cases are covered.
Here’s an example of a sealed class in Kotlin:
sealed class Result
class Success(val data: String) : Result()
class Error(val message: String) : Result()
In this example, Result
is a sealed class with two subclasses: Success
and Error
. Both subclasses are declared within the same file as the sealed class itself. This ensures that no other subclasses can be added from external sources, making the class hierarchy sealed.
Sealed classes are particularly useful for representing restricted states or outcomes in applications. For example, the Result
class above could be used to represent the result of a computation, where Success
represents a successful result containing data, and Error
represents a failure with an error message.
Overall, sealed classes in Kotlin provide a powerful tool for defining restricted class hierarchies and enabling exhaustive pattern matching, making code more robust and easier to reason about.
11. Explain the use of the let
function in Kotlin.
The let
function in Kotlin is a scoping function that allows you to execute a block of code on a nullable object safely. It is particularly useful when you need to perform operations on an object that may be null, without resorting to nested null checks or the use of the safe call operator (?.
).
Here’s how the let
function works:
- Syntax: The
let
function is invoked on an object and takes a lambda function as an argument. Inside the lambda, the object is referred to using theit
keyword. - Usage with Nullable Objects: If the object on which
let
is called is not null, the lambda function is executed with the non-null object as its argument. If the object is null, the lambda is not executed. - Return Value: The
let
function returns the result of the lambda function, which allows you to chain function calls or perform further operations on the result.
Here’s an example to illustrate the usage of the let
function:
val name: String? = "John"
val upperCaseName = name?.let {
println("Original name: $it")
it.toUpperCase()
}
println("Upper case name: $upperCaseName")
In this example:
- The
let
function is called on the nullablename
variable. - Inside the lambda, the non-null value of
name
is referred to asit
. - If
name
is not null, the lambda prints the original name and returns its uppercase version. - The result of the
let
function (the uppercase name) is assigned to theupperCaseName
variable. - The uppercase name is printed outside the
let
function.
The use of the let
function in this example ensures that the code block is only executed if name
is not null, preventing null pointer exceptions. It allows for concise and safe handling of nullable objects, improving code readability and reducing the need for explicit null checks.
12. How does Kotlin handle default arguments in functions?
In Kotlin, you can specify default values for function parameters, allowing you to call functions with fewer arguments if desired. When a default value is provided for a parameter, it becomes optional, and you can omit it when calling the function. If the parameter is not provided, the default value will be used.
Here’s how Kotlin handles default arguments in functions:
- Syntax: To specify a default value for a function parameter, you simply provide the value after the parameter’s type in the function declaration.
fun greet(name: String = "World") {
println("Hello, $name!")
}
- Usage: When calling a function with default arguments, you can omit any arguments for which default values are provided.
greet() // Output: Hello, World!
greet("John") // Output: Hello, John!
- Positional vs. Named Arguments: When calling a function with default arguments, you can provide arguments positionally or by name.
greet(name = "Jane") // Output: Hello, Jane!
- Mixing Default and Non-Default Arguments: If a function has both parameters with default values and parameters without default values, you can still call the function with just the non-default arguments.
fun greet(name: String, greeting: String = "Hello") {
println("$greeting, $name!")
}
greet("Alice") // Output: Hello, Alice!
greet("Bob", "Hi") // Output: Hi, Bob!
Kotlin’s support for default arguments simplifies function calls and reduces the need for overloaded functions or multiple function definitions with different arities. It enhances code readability and maintainability by providing a concise way to define functions with optional parameters.
13. Discuss the usage of the apply
function in Kotlin.
The apply
function in Kotlin is a scoping function that allows you to apply a series of operations to an object within a lambda expression. It is particularly useful for initializing objects or configuring their properties in a concise and readable manner.
Here’s how the apply
function works:
- Syntax: The
apply
function is invoked on an object and takes a lambda function as its argument. Inside the lambda, the object is referred to using thethis
keyword. - Usage: Inside the lambda, you can access and modify the properties of the object using the
this
keyword. Any modifications made to the object within the lambda are applied to the original object. - Return Value: The
apply
function returns the receiver object (i.e., the object on which it was called). This allows you to chain function calls or perform further operations on the modified object.
Here’s an example to illustrate the usage of the apply
function:
class Person {
var name: String = ""
var age: Int = 0
}
val person = Person().apply {
name = "John"
age = 30
}
println("Name: ${person.name}, Age: ${person.age}")
In this example:
- The
apply
function is called on a newly createdPerson
object. - Inside the lambda, the properties
name
andage
of thePerson
object are initialized. - The modifications made within the lambda are applied to the original
Person
object. - The
apply
function returns the modifiedPerson
object, which is then assigned to theperson
variable. - Finally, the properties of the
person
object are printed to the console.
The apply
function is commonly used when initializing objects or configuring their properties, as it allows for a fluent and concise syntax. It promotes code readability and maintainability by providing a clear and expressive way to perform operations on objects. Additionally, the apply
function can help reduce the amount of repetitive code, especially when initializing multiple properties of an object.
14. How do you ensure thread safety in Kotlin applications?
Ensuring thread safety in Kotlin applications involves adopting various techniques and strategies to prevent data races, race conditions, and other concurrency-related issues. Here are some common approaches to ensure thread safety:
- Immutable Data: Prefer immutable data structures and objects whenever possible. Immutable objects cannot be modified after creation, eliminating the risk of concurrent modification and thread interference.
- Synchronization: Use synchronization mechanisms such as synchronized blocks or locks to ensure that critical sections of code are executed by only one thread at a time. This prevents multiple threads from accessing shared resources concurrently and ensures data consistency.
- Atomic Operations: Utilize atomic operations and thread-safe data structures from the
java.util.concurrent
package, such asAtomicInteger
,AtomicBoolean
, orConcurrentHashMap
, for performing atomic operations and managing shared state. - Volatile Keyword: Use the
volatile
keyword to ensure visibility of changes to shared variables across threads. When a variable is declared asvolatile
, its value is always read directly from main memory, ensuring that changes made by one thread are immediately visible to other threads. - Thread Confinement: Limit the scope of shared mutable state by confining it to a single thread or a specific execution context. This reduces the risk of concurrent access and simplifies synchronization requirements.
- Immutable Singleton Pattern: Implement singletons as immutable objects to ensure that they remain thread-safe without the need for synchronization. This can be achieved by using lazy initialization with the
by lazy
delegate. - Concurrency Utilities: Use Kotlin’s built-in concurrency utilities, such as coroutines, to simplify asynchronous programming and manage concurrency in a structured and safe manner. Coroutines provide lightweight threads that can be executed cooperatively, avoiding the overhead of traditional threads.
- Testing: Thoroughly test concurrent code using techniques such as stress testing, race condition detection, and property-based testing to identify and address potential thread safety issues before deployment.
- Code Reviews and Static Analysis: Conduct code reviews and use static analysis tools to identify potential concurrency-related bugs, such as data races or incorrect synchronization, early in the development process.
By applying these techniques and best practices, Kotlin developers can ensure that their applications are robust, reliable, and free from concurrency-related issues, even in multi-threaded environments.
15. Explain the concept of delegates in Kotlin.
In Kotlin, delegates are a powerful design pattern that allows you to delegate the implementation of certain functionalities to another object, known as the delegate. This pattern promotes code reuse, modularity, and separation of concerns by enabling composition over inheritance.
Delegates are commonly used to:
- Implement Property Delegation: Kotlin supports property delegation, where the implementation of getter and setter methods for a property is delegated to another object. This is achieved using the
by
keyword followed by the delegate object.
class Example {
var property: String by SomeDelegate()
}
- Implement Interfaces: Delegates can be used to implement interfaces by forwarding method calls to another object that provides the actual implementation of the interface methods.
interface SomeInterface {
fun someMethod()
}
class Example : SomeInterface by SomeDelegate()
- Extend Functionality: Delegates can extend the functionality of a class by providing additional methods or behavior without modifying its implementation directly.
class Example {
val delegate = SomeDelegate()
fun additionalMethod() {
// Additional functionality
}
}
- Lazy Initialization: Kotlin provides a built-in delegate called
lazy
for lazy initialization of properties. The value of the property is computed only upon first access and cached for subsequent accesses.
val lazyValue: String by lazy {
// Compute the value lazily
"Lazy initialized value"
}
Delegates enable flexible and composable code design by allowing you to separate concerns and encapsulate behavior in separate objects. They promote code reuse and maintainability by providing a clean and modular way to extend and customize the behavior of classes and properties. Overall, delegates are a powerful feature in Kotlin that enhances the expressiveness and flexibility of the language.
16. What are the advantages of using Kotlin over Java?
Using Kotlin over Java offers several advantages, including:
- Concise Syntax: Kotlin’s concise syntax reduces boilerplate code, leading to more readable and expressive code. Features like type inference, data classes, and extension functions allow developers to write code with fewer lines, improving productivity.
- Null Safety: Kotlin’s type system distinguishes between nullable and non-nullable types, reducing the risk of null pointer exceptions at compile time. Nullable types help enforce null safety, leading to more robust and predictable code.
- Interoperability with Java: Kotlin is fully interoperable with Java, allowing seamless integration with existing Java codebases, libraries, and frameworks. This enables gradual adoption of Kotlin in projects and makes it easy for Java developers to transition to Kotlin.
- Functional Programming Support: Kotlin provides first-class support for functional programming paradigms, including higher-order functions, lambda expressions, and immutable data structures. This promotes cleaner, more declarative code and facilitates concurrency and asynchronous programming.
- Coroutines: Kotlin’s built-in coroutines simplify asynchronous and concurrent programming by providing lightweight threads that can be suspended and resumed efficiently. Coroutines enable asynchronous code to be written in a sequential, imperative style, reducing callback hell and improving code readability.
- Extension Functions: Kotlin allows adding new functionality to existing classes without modifying their source code through extension functions. This promotes code modularity and reusability, enhancing the flexibility of Kotlin codebases.
- Tooling Support: Kotlin is supported by a rich set of development tools, including IDE plugins for IntelliJ IDEA, Android Studio, and Visual Studio Code. These tools provide features such as code completion, refactoring, and debugging support, making Kotlin development efficient and enjoyable.
- Safety and Nullability: Kotlin’s type system helps prevent common sources of bugs, such as null pointer exceptions and type errors, at compile time. This leads to more reliable and stable applications, reducing the time spent debugging and fixing runtime errors.
- Community and Ecosystem: Kotlin has a growing and vibrant community of developers and contributors, with an active ecosystem of libraries, frameworks, and resources. This community support ensures access to a wealth of tools and resources for Kotlin development.
Overall, Kotlin offers a modern, expressive, and pragmatic programming language that addresses many of the pain points of Java development, making it an attractive choice for building a wide range of applications, from Android apps to backend systems and beyond.
17. How do you handle exceptions in Kotlin?
In Kotlin, exceptions are handled using try-catch blocks, similar to Java. However, Kotlin provides some additional features and syntax enhancements for working with exceptions. Here’s how exceptions are handled in Kotlin:
- try-catch Blocks: Kotlin supports the traditional try-catch-finally blocks for handling exceptions. You can use them to wrap code that may throw an exception and handle the exception in the catch block.
try {
// Code that may throw an exception
val result = 10 / 0
} catch (e: ArithmeticException) {
// Handle the exception
println("Divide by zero error: ${e.message}")
} finally {
// Code to be executed regardless of whether an exception occurred or not
println("Finally block")
}
- Multiple Catch Blocks: Kotlin allows you to use multiple catch blocks to handle different types of exceptions in a single try-catch statement.
try {
// Code that may throw an exception
val result = someMethod()
} catch (e: IOException) {
// Handle IOException
println("IO Exception: ${e.message}")
} catch (e: IllegalArgumentException) {
// Handle IllegalArgumentException
println("Invalid argument: ${e.message}")
}
- Checked vs Unchecked Exceptions: Kotlin does not distinguish between checked and unchecked exceptions like Java. All exceptions in Kotlin are unchecked, meaning you’re not required to catch or declare them in the function signature.
- Throwing Exceptions: You can throw exceptions using the
throw
keyword followed by an instance of the desired exception class.
fun divide(a: Int, b: Int): Int {
if (b == 0) {
throw ArithmeticException("Division by zero")
}
return a / b
}
- Custom Exception Classes: Kotlin allows you to define custom exception classes by subclassing the
Exception
class or any other existing exception class.
class MyCustomException(message: String) : Exception(message)
- Try Expression: Kotlin provides a try expression, which allows you to handle exceptions in a more functional style by returning a value from the try block or catching the exception and returning a default value.
val result = try {
someMethodThatMayThrowException()
} catch (e: Exception) {
// Handle the exception
null
}
Overall, Kotlin provides flexible and concise syntax for handling exceptions, making it easier to write robust and reliable code. The language encourages the use of modern exception handling techniques while maintaining compatibility with existing Java codebases.
18. What are the different visibility modifiers in Kotlin?
In Kotlin, visibility modifiers control the visibility of classes, interfaces, functions, properties, and other declarations within a codebase. Kotlin provides four visibility modifiers:
- public: Declarations with the
public
visibility modifier are visible everywhere in the codebase, both within the defining module and externally. This is the default visibility modifier if none is specified explicitly. - internal: Declarations with the
internal
visibility modifier are visible only within the same module. Module, in this context, refers to a set of Kotlin files compiled together, such as a Gradle module or Maven module. - protected: Declarations with the
protected
visibility modifier are visible within the same class or subclasses of the defining class. This modifier is not applicable to top-level declarations. - private: Declarations with the
private
visibility modifier are visible only within the same file or the same scope (e.g., class or function). Private declarations are not visible outside their defining scope.
Here’s an example demonstrating the usage of visibility modifiers in Kotlin:
// File: MyClass.kt
package mypackage
internal class InternalClass {
private val privateProperty: String = "Private Property"
protected val protectedProperty: String = "Protected Property"
val publicProperty: String = "Public Property"
private fun privateFunction() {
println("Private Function")
}
protected fun protectedFunction() {
println("Protected Function")
}
fun publicFunction() {
println("Public Function")
}
}
In this example:
InternalClass
has aninternal
visibility modifier, making it visible only within the same module.privateProperty
andprivateFunction
areprivate
, visible only withinInternalClass
.protectedProperty
andprotectedFunction
areprotected
, visible withinInternalClass
and its subclasses.publicProperty
andpublicFunction
arepublic
by default, visible everywhere.
Understanding and correctly applying visibility modifiers in Kotlin helps in managing the accessibility and encapsulation of code within a codebase, leading to more maintainable and modular software architectures.
19. Discuss the usage of the when
expression in Kotlin.
The when
expression in Kotlin is a powerful control flow construct that allows you to replace complex if-else if-else
chains or switch statements with a more concise and expressive syntax. It is used to perform conditional branching based on the value of an expression, similar to a switch statement in other languages.
The when
expression has the following syntax:
when (expression) {
value1 -> { // Case 1
// Code block to execute when expression equals value1
}
value2 -> { // Case 2
// Code block to execute when expression equals value2
}
else -> { // Default case
// Code block to execute when expression does not match any other case
}
}
Here’s how the when
expression is used:
- Simple Matching: You can use the
when
expression to match the value of an expression against multiple cases and execute the corresponding code block for the first matching case.
val x = 5
when (x) {
1 -> println("One")
2 -> println("Two")
else -> println("Other")
}
- Multiple Values: You can specify multiple values separated by commas for a single case to execute the same code block for multiple values.
val x = 5
when (x) {
1, 2, 3 -> println("Small")
in 4..6 -> println("Medium")
else -> println("Large")
}
- Range Matching: You can use range expressions to match a value against a range of values.
val age = 25
when (age) {
in 0..17 -> println("Minor")
in 18..64 -> println("Adult")
else -> println("Senior")
}
- Expression Matching: You can use arbitrary expressions in
when
conditions, including function calls and variable assignments.
val num = 10
when (val result = num % 2) {
0 -> println("Even")
else -> println("Odd")
}
- Smart Casting: In Kotlin,
when
expressions can also be used for type checking and automatic casting of variables.
fun process(obj: Any) {
when (obj) {
is String -> println(obj.toUpperCase())
is Int -> println(obj * 2)
else -> println("Unknown type")
}
}
Overall, the when
expression in Kotlin provides a flexible and concise way to perform conditional branching based on the value of an expression, making code more readable and maintainable. It is a versatile construct that supports a wide range of use cases and patterns.
20. How do you ensure code quality and maintainability in Kotlin projects?
Ensuring code quality and maintainability in Kotlin projects involves adopting various practices, tools, and techniques throughout the development process. Here are some strategies to achieve these goals:
- Code Reviews: Conduct regular code reviews to ensure adherence to coding standards, best practices, and design guidelines. Peer reviews help identify potential issues, improve code quality, and share knowledge among team members.
- Follow Kotlin Style Guide: Adhere to the Kotlin coding style guide to maintain consistency and readability across the codebase. Consistent code style improves collaboration, reduces cognitive overhead, and enhances maintainability.
- Unit Testing: Write comprehensive unit tests to validate the behavior of individual components and functions. Test-driven development (TDD) encourages writing tests before implementing functionality, ensuring code correctness and facilitating future changes with confidence.
- Integration Testing: Perform integration tests to verify the interaction and integration of different modules or components within the application. Integration tests help identify compatibility issues, dependencies, and integration points.
- Continuous Integration (CI): Set up a CI pipeline to automate code compilation, testing, and deployment processes. CI ensures early detection of errors, promotes code quality, and facilitates collaboration among team members.
- Static Code Analysis: Use static code analysis tools such as Kotlin Lint, Detekt, or KtLint to analyze code for potential issues, code smells, and violations of coding standards. Static analysis tools help identify and address code quality issues proactively.
- Refactoring: Continuously refactor code to improve its structure, readability, and maintainability. Refactoring eliminates code duplication, reduces complexity, and enhances code maintainability over time.
- Documentation: Document code comprehensively with meaningful comments, Javadoc/KDoc annotations, and README files. Well-documented code helps developers understand the purpose, behavior, and usage of different components, facilitating maintenance and troubleshooting.
- Dependency Management: Manage dependencies efficiently using dependency management tools like Gradle or Maven. Keep dependencies up-to-date, minimize dependency coupling, and avoid unnecessary dependencies to reduce maintenance overhead.
- Modularization: Modularize code into smaller, cohesive modules or components with well-defined responsibilities. Modular design promotes code reuse, facilitates testing, and simplifies maintenance and evolution of the codebase.
- Version Control: Use version control systems like Git to track changes, collaborate with team members, and manage code history effectively. Version control enables rollback to previous versions, code reviews, and collaboration workflows.
- Monitoring and Logging: Implement monitoring and logging mechanisms to track application performance, errors, and exceptions in production environments. Monitoring and logging help identify and resolve issues quickly, improving overall system reliability and maintainability.
By incorporating these practices into your Kotlin projects, you can ensure code quality, readability, and maintainability, leading to a more robust and sustainable codebase.
Conclusions
Conclusions of “Kotlin Interview Questions for Senior Developer”:
- Comprehensive Assessment:
- The Kotlin interview questions for senior developers cover a wide range of topics, including language features, advanced concepts, best practices, and practical scenarios.
- Depth of Knowledge:
- Senior developers are expected to demonstrate a deep understanding of Kotlin language fundamentals, such as null safety, data classes, sealed classes, and coroutines, among others.
- Problem-solving Skills:
- The interview questions are designed to assess the candidate’s problem-solving skills and ability to apply Kotlin features to real-world scenarios, including concurrency, functional programming, and Android development.
- Experience with Advanced Topics:
- Senior developers are expected to have experience with advanced Kotlin topics, such as DSLs (Domain-Specific Languages), property delegation, inline functions, and extension functions.
- Awareness of Best Practices:
- Candidates are evaluated based on their knowledge of Kotlin best practices, including code readability, maintainability, performance optimization, and idiomatic Kotlin usage.
- Adaptability and Learning Agility:
- The interview questions may also gauge the candidate’s adaptability and willingness to learn new Kotlin features and technologies, as Kotlin continues to evolve and gain popularity in various domains.
- Communication and Collaboration Skills:
- In addition to technical proficiency, senior developers are expected to demonstrate strong communication and collaboration skills, as they may need to mentor junior developers, collaborate with cross-functional teams, and communicate technical concepts effectively.
- Preparation and Continued Learning:
- Candidates are encouraged to prepare thoroughly for the interview by reviewing Kotlin language documentation, practicing coding exercises, and staying updated on recent Kotlin developments and industry trends.
In conclusion, the Kotlin interview questions for senior developers aim to assess candidates’ proficiency, problem-solving abilities, experience with advanced Kotlin topics, adherence to best practices, and readiness to contribute effectively to Kotlin-based projects and teams.
Crack DSA Interview: Google, Facebook, and Amazon!👇
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
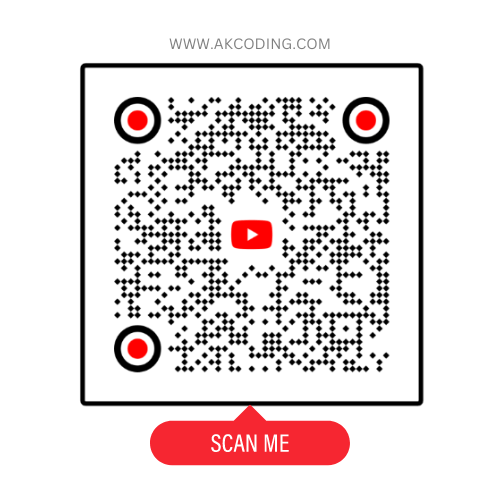