While the most common coding interview questions can vary, here is a list of questions that are frequently asked in technical interviews. Keep in mind that the specific questions might depend on the role, the company, and the interviewer’s preferences.
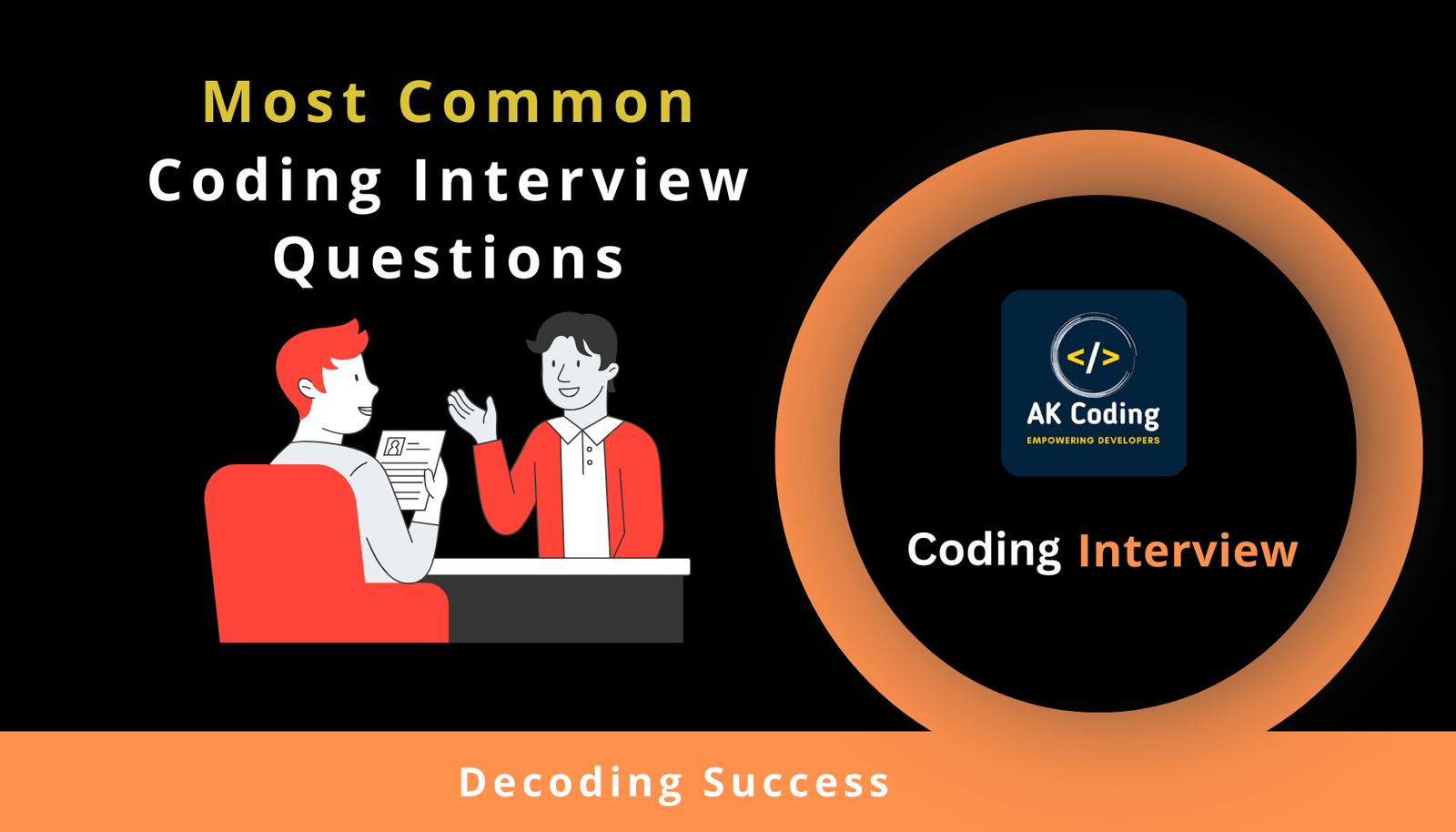
Table of Contents
Data Structures and Algorithms:
- Reverse a String:
- Write a function to reverse a string. Consider different methods such as using loops or recursion.
- Two Sum:
- Given an array of integers, find two numbers such that they add up to a specific target.
- Palindrome Check:
- Determine if a given string is a palindrome (reads the same forwards and backward).
- Linked List Operations:
- Implement basic linked list operations like insertion, deletion, and reversing.
- Binary Search:
- Implement the binary search algorithm to find an element in a sorted array.
- Depth-First Search (DFS) and Breadth-First Search (BFS):
- Traverse a graph using DFS or BFS. Solve problems like finding connected components or shortest paths.
- Merge Sort and QuickSort:
- Implement popular sorting algorithms.
- Dynamic Programming:
- Solve problems involving dynamic programming, like the Fibonacci sequence or the knapsack problem.
- Tree Traversal:
- Implement in-order, pre-order, and post-order traversal of binary trees.
Strings and Arrays:
- String Manipulation:
- Perform common string operations, such as reversing a sentence, finding the length of a string, or checking for anagrams.
- Array Rotation:
- Rotate an array to the left or right by a specified number of positions.
- Longest Substring Without Repeating Characters:
- Find the length of the longest substring without repeating characters.
System Design:
- URL Shortening Service:
- Design a URL shortening service.
- Cache Design:
- Design a cache system.
- Scalable System Design:
- Discuss how you would design a scalable and distributed system for a specific scenario.
Object-Oriented Design:
- Deck of Cards:
- Design the classes and methods for a deck of cards.
- Parking Lot System:
- Design a parking lot system with appropriate classes and functionalities.
Miscellaneous:
- Find the Missing Number:
- Given an array containing n distinct numbers taken from 0 to n, find the one that is missing from the array.
- Spiral Matrix:
- Given an m x n matrix, return all elements of the matrix in spiral order.
- Anagram Groups:
- Group anagrams from a list of strings.
- Largest Sum Subarray (Kadane’s Algorithm):
- Find the contiguous subarray with the largest sum.
- Detect Cycle in a Directed Graph:
- Determine if a directed graph contains a cycle.
These questions cover a range of topics and difficulty levels commonly encountered in coding interviews. Practice solving these problems, understand the underlying concepts, and be prepared to discuss your thought process during interviews. Additionally, adapt your preparation based on the specific requirements of the role you are interviewing for.
Other articles