Introduction
Navigation is a fundamental part of any web application. Whether you’re building a simple website or a complex web app, users need a seamless way to move between pages. In traditional React applications, navigation is often handled using React Router, but in Next.js, routing is built-in and incredibly easy to use.
In this tutorial, you’ll learn how to navigate between pages in Next.js, covering various techniques such as client-side navigation, programmatic navigation, dynamic routing, and link prefetching. By the end, you’ll have a solid understanding of how navigation works in Next.js and how to optimize it for performance.
1. Understanding Next.js Routing
Next.js uses a file-based routing system, which means that the structure of your pages/
directory defines your application’s routes. Here’s an example:
/pages
├── index.js // Homepage (/)
├── about.js // About page (/about)
├── contact.js // Contact page (/contact)
Each file inside pages/
automatically becomes a route. This makes routing in Next.js intuitive and easy to manage.
2. Navigating Between Pages Using <Link />
The recommended way to navigate between pages in Next.js is by using the built-in <Link />
component from next/link
. This provides client-side navigation, ensuring a smooth user experience without a full page reload.
Example:
import Link from 'next/link';
export default function HomePage() {
return (
<div>
<h1>Welcome to My Website</h1>
<nav>
<ul>
<li><Link href="/about">About Us</Link></li>
<li><Link href="/contact">Contact</Link></li>
</ul>
</nav>
</div>
);
}
Why Use <Link />
?
- Prevents full-page reloads, improving performance.
- Prefetches pages automatically for faster transitions.
- Works with both static and dynamic routes.
3. Programmatic Navigation in Next.js
Sometimes, you need to navigate between pages dynamically based on user actions. Next.js provides the useRouter
hook from next/router
for this purpose.
Example:
import { useRouter } from 'next/router';
export default function Dashboard() {
const router = useRouter();
const goToProfile = () => {
router.push('/profile');
};
return (
<div>
<h1>Dashboard</h1>
<button onClick={goToProfile}>Go to Profile</button>
</div>
);
}
router.push()
vs. router.replace()
router.push('/profile')
→ Adds a new entry in the browser history.router.replace('/profile')
→ Replaces the current entry, preventing users from going back.
4. Navigating to Dynamic Pages
Dynamic routes allow you to create parameterized URLs (e.g., /product/[id]
). You can navigate dynamically using useRouter
.
Example:
import { useRouter } from 'next/router';
export default function Products() {
const router = useRouter();
const viewProduct = (id) => {
router.push(`/product/${id}`);
};
return (
<div>
<h1>Product List</h1>
<button onClick={() => viewProduct(1)}>View Product 1</button>
<button onClick={() => viewProduct(2)}>View Product 2</button>
</div>
);
}
5. Navigating with Query Parameters
If you need to pass query parameters while navigating, you can do so with router.push()
.
Example:
const handleSearch = (query) => {
router.push({
pathname: '/search',
query: { q: query },
});
};
In the destination page (search.js
), you can access the query parameters using:
const { query } = useRouter();
console.log(query.q); // Output: user input query
6. Using next/link
with Custom Styles
You can style links dynamically using the className
or style
prop.
<Link href="/about">
<a style={{ color: 'blue', fontWeight: 'bold' }}>About Us</a>
</Link>
Alternatively, use CSS modules:
import styles from './Nav.module.css';
<Link href="/about">
<a className={styles.link}>About</a>
</Link>
7. Prefetching for Faster Navigation
By default, Next.js prefetches linked pages in the background, making navigation almost instant.
If you want to disable prefetching, you can do:
<Link href="/about" prefetch={false}>About</Link>
8. Navigating with Scroll Behavior
By default, Next.js scrolls to the top when navigating between pages. To preserve scroll position, use:
router.push('/about', undefined, { scroll: false });
Conclusion
Navigating between pages in Next.js is fast, simple, and efficient. Using <Link />
ensures client-side transitions, while useRouter
allows for dynamic and programmatic navigation. With features like automatic prefetching, dynamic routes, and query parameters, Next.js makes routing a breeze.
Now that you’ve mastered navigation in Next.js, try implementing it in your project and see the performance benefits for yourself! 🚀
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
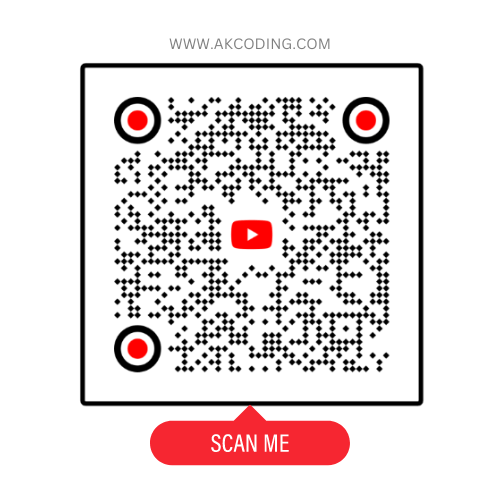