Next.js has revolutionized the way developers build modern web applications, offering powerful rendering techniques that optimize performance and user experience. In this guide, we’ll explore two key rendering strategies in Next.js: Static Rendering and Dynamic Rendering. By the end, you’ll understand when to use each approach, their advantages, and practical use cases.
What is Static Rendering?
Static Rendering in Next.js refers to pre-generating HTML pages at build time. The generated static files are then served to users instantly, leading to blazing-fast page loads.
Types of Static Rendering in Next.js
- Static Site Generation (SSG) – Generates static pages at build time.
- Incremental Static Regeneration (ISR) – Updates static pages in the background without a full rebuild.
How Static Rendering Works
Next.js pre-renders the page during the build process, storing the result as a static HTML file. This means:
- No database or API requests occur on each request.
- The page loads instantly since it is served directly from the CDN.
- Ideal for SEO-friendly and content-heavy sites.
Example of Static Rendering using SSG
export async function getStaticProps() {
const response = await fetch('https://api.example.com/posts');
const posts = await response.json();
return {
props: { posts },
};
}
export default function Blog({ posts }) {
return (
<div>
<h1>Blog Posts</h1>
{posts.map(post => (
<div key={post.id}>
<h2>{post.title}</h2>
<p>{post.content}</p>
</div>
))}
</div>
);
}
Use Cases for Static Rendering
- Blogs and news websites
- Marketing and landing pages
- Documentation sites
- E-commerce product listings
What is Dynamic Rendering?
Dynamic Rendering means generating the HTML at runtime whenever a user requests a page. Unlike Static Rendering, where pages are pre-generated, dynamic pages fetch data on demand.
Types of Dynamic Rendering in Next.js
- Server-Side Rendering (SSR) – Fetches data on every request.
- Client-Side Rendering (CSR) – Fetches and renders content on the client-side after initial load.
How Dynamic Rendering Works
When a request is made, Next.js executes the necessary logic, fetches the required data, and renders the page on the server before sending it to the client.
Example of Dynamic Rendering using SSR
export async function getServerSideProps() {
const response = await fetch('https://api.example.com/user');
const user = await response.json();
return {
props: { user },
};
}
export default function Profile({ user }) {
return (
<div>
<h1>Welcome, {user.name}</h1>
<p>Email: {user.email}</p>
</div>
);
}
Use Cases for Dynamic Rendering
- Personalized dashboards (e.g., user profiles)
- Real-time data applications
- Stock market or weather updates
- E-commerce checkout pages
Static vs. Dynamic Rendering: Key Differences
Feature | Static Rendering | Dynamic Rendering |
---|---|---|
Speed | Faster (Pre-built HTML) | Slightly slower (Rendered per request) |
SEO | Excellent | Good (slightly slower indexing) |
Data Freshness | Stale if not regenerated | Always up-to-date |
Use Case | Blogs, marketing pages | User dashboards, live data |
When to Use Static vs. Dynamic Rendering
Scenario | Rendering Type |
---|---|
A blog with rarely updated content | Static Rendering (SSG) |
A dashboard displaying real-time user data | Dynamic Rendering (SSR) |
An e-commerce product page that updates hourly | Incremental Static Regeneration (ISR) |
A search results page with user input | Client-Side Rendering (CSR) |
Best Practices for Optimizing Rendering in Next.js
- Use Static Rendering (SSG) whenever possible – This ensures the fastest load times and best SEO performance.
- Leverage Incremental Static Regeneration (ISR) – Update static content without rebuilding the entire site.
- Use Server-Side Rendering (SSR) only when necessary – SSR pages increase server load and should be used sparingly.
- Minimize unnecessary API calls – Optimize backend requests to avoid slow performance.
- Use caching and CDNs – Serve static content efficiently and reduce server response times.
Conclusion
Understanding the difference between Static and Dynamic Rendering in Next.js is crucial for building high-performance applications. While SSG and ISR offer blazing-fast speeds and SEO benefits, SSR and CSR provide flexibility for dynamic, user-driven experiences. By leveraging the right rendering approach for your Next.js projects, you can improve performance, enhance user experience, and optimize resource utilization.
Want to dive deeper into Next.js? Stay tuned for more tutorials and best practices!
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
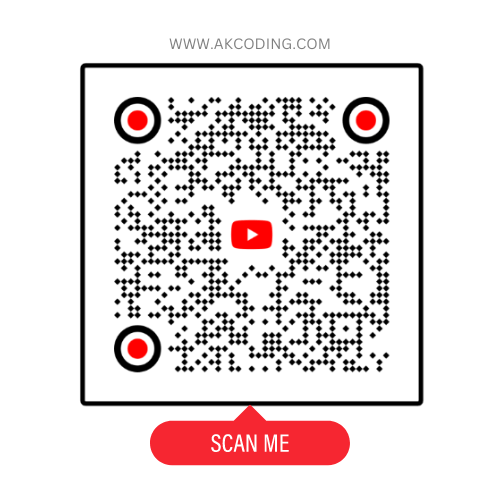