Advanced Questions
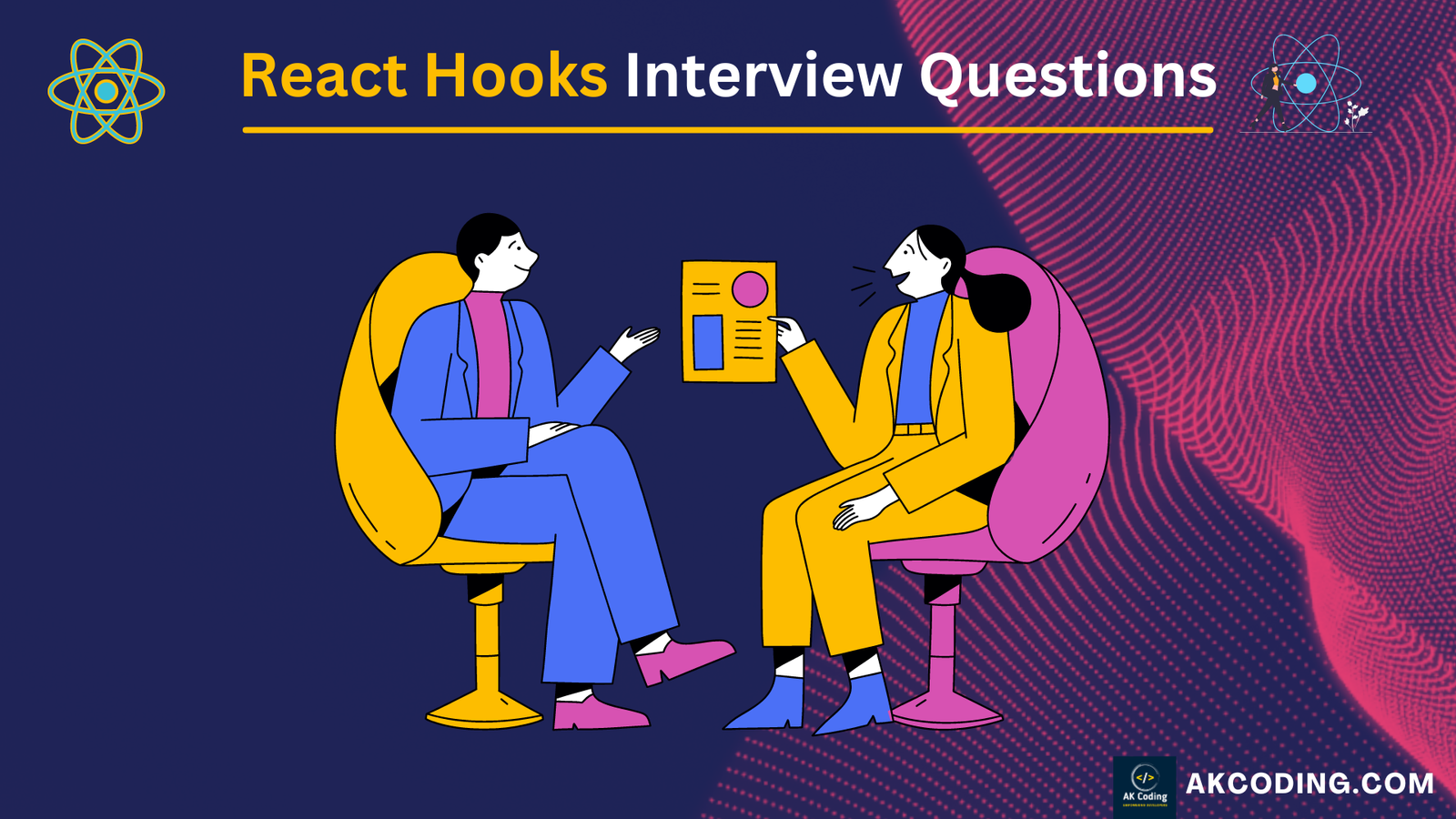
Table of Contents
React Hooks Interview Questions
Advanced React Hooks interview questions are designed to assess a candidate’s in-depth understanding of React Hooks, including how they work, best practices, and advanced concepts. Here are some advanced interview questions focused on React Hooks:
1. State Management with Hooks
- Question: Explain the difference between
useState
anduseReducer
. In what scenarios would you chooseuseReducer
overuseState
? - Answer:
useState
is ideal for simple state management with individual state variables, whileuseReducer
is useful for complex state logic and managing state transitions with actions. You’d chooseuseReducer
when dealing with multiple state variables or complex operations, like updating a shopping cart or handling multiple form fields.
2. Handling Side Effects
- Question: What are dependency arrays in
useEffect
, and why are they important? How would you handle an effect that depends on dynamic data? - Answer: Dependency arrays control when the
useEffect
function runs, based on the variables listed. If the array is empty, the effect runs once on mount. If it contains variables, the effect re-runs when those variables change. To handle dynamic data, ensure the dependencies are correctly listed to avoid unintended re-renders or infinite loops.
3. Performance Optimization
- Question: How do
useMemo
anduseCallback
help optimize React applications? What are the risks of overusing them? - Answer:
useMemo
memoizes computed values to avoid recalculations, whileuseCallback
memoizes functions to prevent re-renders when passing callbacks to child components. Overuse can lead to complex code and memory overhead. Use these hooks only when needed to optimize performance.
4. Custom Hooks
- Question: Describe a scenario where you would create a custom Hook. What are the benefits of custom Hooks in a React application?
- Answer: A custom Hook is useful for encapsulating common logic, such as form validation or data fetching. Custom Hooks promote code reuse and separation of concerns. For example, a custom Hook for fetching data abstracts the fetching logic, allowing reuse across multiple components.
5. Context and Global State
- Question: What is the role of
useContext
in React? How can you avoid “prop drilling” using context? - Answer:
useContext
allows functional components to consume values from a React context without passing props through multiple levels (prop drilling). By providing a context at a higher level in the component tree, you can share state across components without passing props down through each level.
6. Managing Component Lifecycle
- Question: Explain the differences between
useEffect
anduseLayoutEffect
. When would you useuseLayoutEffect
instead ofuseEffect
? - Answer:
useEffect
runs after the component is painted to the screen, whileuseLayoutEffect
runs before painting, affecting the layout. UseuseLayoutEffect
when changes must happen before rendering, such as DOM measurements or critical layout manipulations.
7. Managing Refs and Imperative Handles
- Question: How can
useRef
be used to manage DOM elements in functional components? Explain the use ofuseImperativeHandle
in React. - Answer:
useRef
provides a reference to DOM elements or values that persist across renders, useful for focus management or accessing DOM attributes.useImperativeHandle
allows you to expose imperative methods to parent components, facilitating controlled components or specialized behaviors.
8. What are the key differences between React vs Vue js
Here’s a comparison of React vs Vue.js in tabular format:
Feature | React | Vue.js |
---|---|---|
Type | JavaScript Library | JavaScript Framework |
Created By | Evan You | |
Initial Release | 2013 | 2014 |
Architecture | Component-based, focuses on the view layer | Component-based, but also provides features like Vuex for state management |
Learning Curve | Steeper, especially with JSX and extensive ecosystem | Easier, simpler syntax and structure |
Ecosystem | Large, with numerous third-party libraries and tools | Smaller, but with a cohesive ecosystem |
Data Binding | One-way data binding | Two-way data binding |
State Management | Requires additional libraries like Redux or Context API | Built-in state management with Vuex |
Performance | High, especially with the Virtual DOM | Comparable performance, also uses Virtual DOM |
Community Size | Larger, more widespread in enterprise applications | Growing, popular among small to medium projects |
Flexibility | Highly flexible, allows for integration with other libraries or frameworks | Flexible, but more opinionated in structure |
Integration | Often integrated with other tools for routing, state management, etc. | Comes with built-in solutions for routing, state management, etc. |
Use Cases | Best for complex, large-scale applications | Ideal for small to medium-sized applications |
Popularity | Higher, more widely used in the industry | Increasing in popularity, especially in the Asian market |
Community Support | Extensive, with vast resources available | Strong, but with fewer resources compared to React |
These advanced questions cover a range of topics, from state management to performance optimization and lifecycle handling, providing a comprehensive assessment of a candidate’s expertise with React Hooks.
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
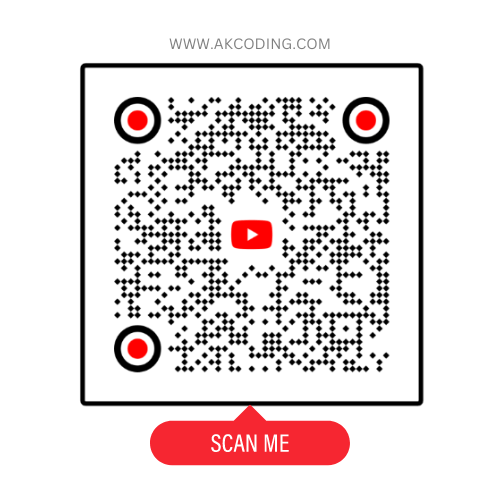