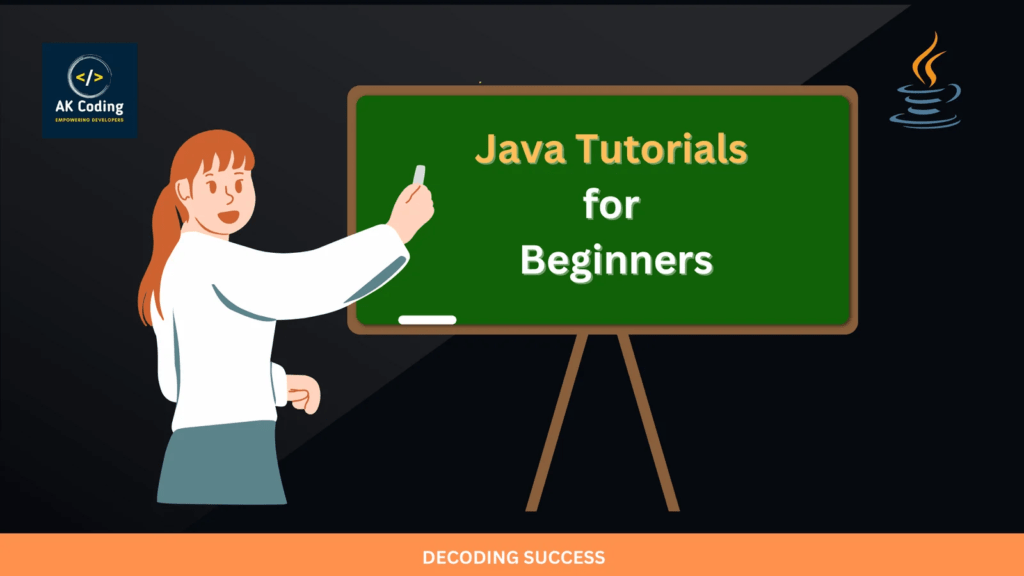
Certainly! Here’s an outline for a comprehensive Java tutorial:
Table of Contents
A Comprehensive Road map to Java Tutorial
1. Introduction to Java
2. Java Basics
- Syntax and Structure
- Data Types and Variables
- Operators and Expressions
- Control Flow (if-else, switch-case, loops)
3. Object-Oriented Programming in Java
4. Exception Handling
5. Collections Framework
- Introduction to Collections
- List, Set, Map Interfaces
- ArrayList, LinkedList, HashSet, HashMap Implementations
- Iterators and Iterable Interface
6. Generics
- Introduction to Generics
- Generic Classes and Methods
- Type Erasure
7. Input/Output (I/O) Streams
- File Handling
- Byte Streams and Character Streams
- Serialization and Deserialization
8. Multithreading
- Introduction to Threads
- Thread Lifecycle
- Synchronization
- Thread Pools and Executors
9. Java 8 Features
- Lambda Expressions
- Stream API
- Optional Class
- Date and Time API
10. JDBC (Java Database Connectivity)
- Introduction to JDBC
- Connecting to Databases
- Executing SQL Queries
- Handling Result Sets
11. GUI Development with Swing
- Introduction to Swing
- Components and Containers
- Event Handling
12. JavaFX
- Introduction to JavaFX
- UI Controls and Layouts
- Event Handling in JavaFX
13. Web Development with Java
- Introduction to Servlets
- JavaServer Pages (JSP)
- Java Persistence API (JPA)
14. Testing in Java
- Introduction to JUnit
- Writing and Running Test Cases
- Mockito for Mocking Dependencies
15. Best Practices and Coding Standards
- Naming Conventions
- Code Formatting
- Exception Handling Strategies
16. Advanced Topics
- Reflection
- Annotations
- Design Patterns
17. Java Development Tools
- Integrated Development Environments (IDEs)
- Build Tools (Maven, Gradle)
- Version Control Systems (Git)
18. Java Interview Preparation
- Common Interview Questions
- Practice Exercises and Challenges
19. Conclusion
- Recap of Key Concepts
- Future Trends in Java Development
This outline covers a comprehensive range of Java topics, suitable for beginners as well as intermediate learners. Each topic can be expanded into detailed tutorials, exercises, and practical examples to provide a thorough understanding of Java programming.
For Other Awesome Article visit AKCODING.COM and read articles in Medium