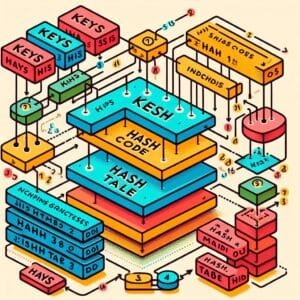
Hashing and Hash Tables in data structure
1. Introduction to Hashing
- Definition of hashing.
- The need for hashing in computer science.
- Real-world analogies of hashing (e.g., dictionaries, phonebooks).
2. Key Concepts in Hashing
- Hash Function:
- Definition and purpose.
- Properties of a good hash function (deterministic, efficient, uniform distribution, etc.).
- Hash Table:
- Data structure overview.
- How hash tables store and retrieve data efficiently.
- Keys and Values:
- Explanation of key-value pairs.
- Examples in everyday applications (e.g., username-password mappings).
3. How Hash Tables Work
- Inserting a key-value pair.
- Retrieving a value using the key.
- Deleting a key-value pair.
4. Collisions in Hashing
- Definition of collision.
- Causes of collisions.
- Collision resolution techniques:
- Chaining (Separate Chaining).
- Open Addressing:
- Linear Probing.
- Quadratic Probing.
- Double Hashing.
5. Hash Table Operations
- Time complexities for:
- Insertion.
- Search.
- Deletion.
- Best, worst, and average-case scenarios.
6. Applications of Hashing and Hash Tables
- Caching mechanisms.
- HashMaps in programming languages (e.g., Python dictionaries, Java HashMap).
- Cryptography and data security.
- Database indexing.
7. Limitations of Hash Tables
- Hash collisions.
- Inefficiency with poor hash functions.
- Memory overhead compared to other data structures.
8. Designing a Hash Function
- Guidelines for creating a good hash function.
- Examples of common hash functions (e.g., division method, multiplication method).
9. Advanced Hashing Techniques
- Perfect hashing.
- Dynamic resizing of hash tables (rehashing).
- Bloom filters (brief introduction).
10. Practical Implementation
- Code examples:
- Hash table implementation in Python, Java, or C++.
- Demonstration of collision handling.
- Sample problem and solution using a hash table.
11. Comparison with Other Data Structures
- Hash tables vs. arrays.
- Hash tables vs. trees (e.g., Binary Search Tree, AVL Tree).
12. Conclusion and Key Takeaways
- Recap of hashing concepts and benefits.
- When and why to use hash tables.
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
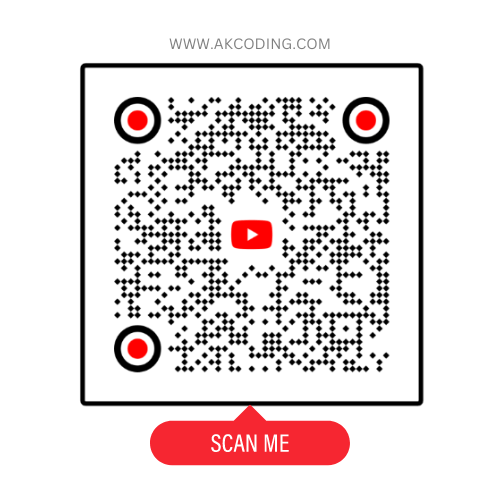