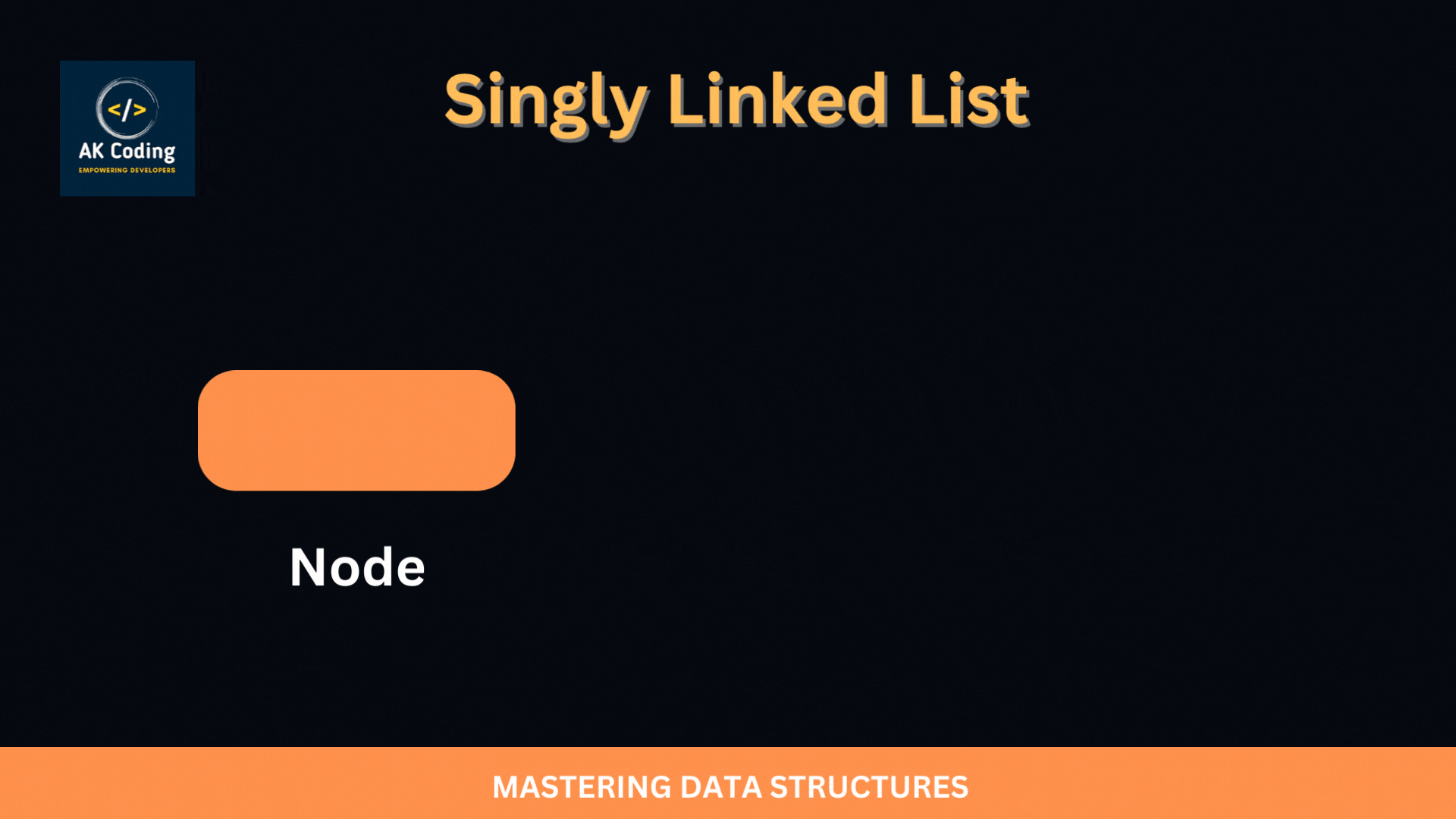
Table of Contents
Introduction of singly linked list
A Singly Linked List is a fundamental data structure in computer science, representing a collection of nodes where each node stores a data element and a reference to the next node in the sequence. Unlike arrays, linked lists do not have a fixed size, allowing for dynamic memory allocation as elements are added or removed.
The structure of a Singly Linked List consists of nodes connected in a linear sequence, with each node containing data and a pointer/reference to the next node. This organization enables efficient insertion and deletion operations, as well as traversal through the list.
Singly Linked Lists are versatile and find applications in various scenarios, including implementing stacks, queues, and dynamic memory allocation. Understanding the concepts and operations of Singly Linked Lists is essential for any programmer, as they form the basis for more complex data structures and algorithms. In this tutorial, we will explore the fundamentals of Singly Linked Lists, covering their structure, basic operations, and practical applications.
Node Structure

The node structure of a Singly Linked List consists of two main components: data and a reference to the next node in the sequence. Here’s an example of a typical node structure for a Singly Linked List in Java.
public class Node {
// Data stored in the node
private int data;
// Reference to the next node in the sequence
private Node next;
// Constructor to initialize node with data
public Node(int data) {
this.data = data;
this.next = null; // Initially, the next node reference is null
}
// Getters and setters for data and next reference
public int getData() {
return data;
}
public void setData(int data) {
this.data = data;
}
public Node getNext() {
return next;
}
public void setNext(Node next) {
this.next = next;
}
}
In this node structure:
data
represents the value stored in the node.next
is a reference to the next node in the sequence. It is initialized tonull
if the node is the last node in the list.- The constructor initializes a node with the provided data and sets the next reference to
null
. - Getters and setters are provided to access and modify the data and next reference of the node.
This node structure forms the building blocks of a Singly Linked List, allowing for the creation of a linear sequence of nodes where each node contains data and a reference to the next node in the list.
Basic Operations
Insertion
Inserting a node at the beginning of the list.
To insert a node at the beginning of a Singly Linked List, you need to follow these steps:
- Create a new node with the data to be inserted.
- Set the
next
reference of the new node to point to the current head of the list. - Update the head of the list to point to the newly inserted node.
Here’s the Java code to insert a node at the beginning of a Singly Linked List:
public class SinglyLinkedList {
private Node head; // Head of the linked list
// Node structure
private static class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
// Method to insert a node at the beginning of the list
public void insertAtBeginning(int data) {
Node newNode = new Node(data); // Create a new node with the given data
newNode.next = head; // Set the next reference of the new node to the current head
head = newNode; // Update the head to point to the new node
}
// Method to display the linked list
public void display() {
Node current = head; // Start from the head of the list
while (current != null) {
System.out.print(current.data + " "); // Print the data of the current node
current = current.next; // Move to the next node
}
System.out.println(); // Print a newline after displaying all nodes
}
public static void main(String[] args) {
SinglyLinkedList list = new SinglyLinkedList();
list.insertAtBeginning(3);
list.insertAtBeginning(5);
list.insertAtBeginning(7);
list.display(); // Output: 7 5 3
}
}
In this code:
- The
insertAtBeginning
method creates a new node with the given data, sets itsnext
reference to the current head of the list, and updates the head to point to the new node. - The
display
method is used to print the elements of the linked list for demonstration purposes. - In the
main
method, we create a new instance of theSinglyLinkedList
class, insert nodes at the beginning of the list, and display the list to verify the insertion.
Inserting a node at the end of the list.
To insert a node at the end of a Singly Linked List, you need to follow these steps:
- Create a new node with the data to be inserted.
- Traverse the list until you reach the last node (i.e., the node with
next
reference pointing tonull
). - Set the
next
reference of the last node to point to the newly created node.
Here’s the Java code to insert a node at the end of a Singly Linked List:
public class SinglyLinkedList {
private Node head; // Head of the linked list
// Node structure
private static class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
// Method to insert a node at the end of the list
public void insertAtEnd(int data) {
Node newNode = new Node(data); // Create a new node with the given data
if (head == null) { // If the list is empty, set the new node as the head
head = newNode;
return;
}
Node current = head;
while (current.next != null) {
current = current.next; // Traverse the list until the last node
}
current.next = newNode; // Set the next reference of the last node to the new node
}
// Method to display the linked list
public void display() {
Node current = head; // Start from the head of the list
while (current != null) {
System.out.print(current.data + " "); // Print the data of the current node
current = current.next; // Move to the next node
}
System.out.println(); // Print a newline after displaying all nodes
}
public static void main(String[] args) {
SinglyLinkedList list = new SinglyLinkedList();
list.insertAtEnd(3);
list.insertAtEnd(5);
list.insertAtEnd(7);
list.display(); // Output: 3 5 7
}
}
In this code:
- The
insertAtEnd
method creates a new node with the given data and inserts it at the end of the list by traversing the list until the last node and updating itsnext
reference. - The
display
method is used to print the elements of the linked list for demonstration purposes. - In the
main
method, we create a new instance of theSinglyLinkedList
class, insert nodes at the end of the list, and display the list to verify the insertion.
Inserting a node at a specific position in the list.
To insert a node at a specific position in a Singly Linked List, you need to follow these steps:
- Create a new node with the data to be inserted.
- Traverse the list until you reach the node at the desired position (index).
- Update the
next
reference of the new node to point to the node at the current position. - Update the
next
reference of the previous node (at position – 1) to point to the new node.
Here’s the Java code to insert a node at a specific position in a Singly Linked List:
public class SinglyLinkedList {
private Node head; // Head of the linked list
// Node structure
private static class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
// Method to insert a node at a specific position in the list
public void insertAtPosition(int data, int position) {
if (position < 0) {
System.out.println("Invalid position");
return;
}
Node newNode = new Node(data); // Create a new node with the given data
if (position == 0) { // If position is 0, insert at the beginning of the list
newNode.next = head;
head = newNode;
return;
}
Node current = head;
int currentPosition = 0;
while (currentPosition < position - 1 && current != null) {
current = current.next; // Traverse the list until the node before the desired position
currentPosition++;
}
if (current == null) {
System.out.println("Invalid position");
return;
}
newNode.next = current.next; // Set the next reference of the new node to the node at the current position
current.next = newNode; // Set the next reference of the previous node to the new node
}
// Method to display the linked list
public void display() {
Node current = head; // Start from the head of the list
while (current != null) {
System.out.print(current.data + " "); // Print the data of the current node
current = current.next; // Move to the next node
}
System.out.println(); // Print a newline after displaying all nodes
}
public static void main(String[] args) {
SinglyLinkedList list = new SinglyLinkedList();
list.insertAtPosition(3, 0);
list.insertAtPosition(5, 1);
list.insertAtPosition(7, 1);
list.display(); // Output: 3 7 5
}
}
In this code:
- The
insertAtPosition
method inserts a new node with the given data at the specified position in the list by traversing the list until the node before the desired position and updating thenext
references accordingly. - The
display
method is used to print the elements of the linked list for demonstration purposes. - In the
main
method, we create a new instance of theSinglyLinkedList
class, insert nodes at specific positions in the list, and display the list to verify the insertion.
Deletion
Deleting the first node in the list.
To delete the first node in a Singly Linked List, you need to follow these steps:
- Check if the list is empty. If it is, there’s nothing to delete.
- Update the
head
reference to point to the next node of the current head. - Optionally, if needed, you may also release the memory occupied by the deleted node.
Here’s the Java code to delete the first node in a Singly Linked List:
public class SinglyLinkedList {
private Node head; // Head of the linked list
// Node structure
private static class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
// Method to delete the first node in the list
public void deleteFirstNode() {
if (head == null) {
System.out.println("List is empty. Nothing to delete.");
return;
}
head = head.next; // Update the head to point to the next node
}
// Method to display the linked list
public void display() {
Node current = head; // Start from the head of the list
while (current != null) {
System.out.print(current.data + " "); // Print the data of the current node
current = current.next; // Move to the next node
}
System.out.println(); // Print a newline after displaying all nodes
}
public static void main(String[] args) {
SinglyLinkedList list = new SinglyLinkedList();
list.deleteFirstNode(); // Output: List is empty. Nothing to delete.
// Insert nodes for demonstration
list.head = new Node(3);
list.head.next = new Node(5);
list.head.next.next = new Node(7);
list.display(); // Output: 3 5 7
list.deleteFirstNode();
list.display(); // Output: 5 7
}
}
In this code:
- The
deleteFirstNode
method deletes the first node in the list by updating thehead
reference to point to the next node. - If the list is empty, a message is printed indicating that there’s nothing to delete.
- The
display
method is used to print the elements of the linked list for demonstration purposes. - In the
main
method, we create a new instance of theSinglyLinkedList
class, insert nodes into the list, delete the first node, and display the updated list to verify the deletion.
Deleting the last node in the list.
To delete the last node in a Singly Linked List, you need to follow these steps:
- Check if the list is empty. If it is, there’s nothing to delete.
- If the list has only one node (i.e., the head node), set the
head
reference tonull
. - Traverse the list until you reach the second-to-last node.
- Update the
next
reference of the second-to-last node tonull
, effectively removing the last node from the list. - Optionally, if needed, you may also release the memory occupied by the deleted node.
Here’s the Java code to delete the last node in a Singly Linked List:
public class SinglyLinkedList {
private Node head; // Head of the linked list
// Node structure
private static class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
// Method to delete the last node in the list
public void deleteLastNode() {
if (head == null) {
System.out.println("List is empty. Nothing to delete.");
return;
}
if (head.next == null) { // If there's only one node in the list
head = null; // Set the head to null, indicating an empty list
return;
}
Node current = head;
while (current.next.next != null) {
current = current.next; // Traverse the list until the second-to-last node
}
current.next = null; // Set the next reference of the second-to-last node to null
}
// Method to display the linked list
public void display() {
Node current = head; // Start from the head of the list
while (current != null) {
System.out.print(current.data + " "); // Print the data of the current node
current = current.next; // Move to the next node
}
System.out.println(); // Print a newline after displaying all nodes
}
public static void main(String[] args) {
SinglyLinkedList list = new SinglyLinkedList();
list.deleteLastNode(); // Output: List is empty. Nothing to delete.
// Insert nodes for demonstration
list.head = new Node(3);
list.head.next = new Node(5);
list.head.next.next = new Node(7);
list.display(); // Output: 3 5 7
list.deleteLastNode();
list.display(); // Output: 3 5
}
}
In this code:
- The
deleteLastNode
method deletes the last node in the list by traversing the list until the second-to-last node and setting itsnext
reference tonull
. - If the list is empty or has only one node, appropriate messages are printed indicating that there’s nothing to delete or the list is now empty.
- The
display
method is used to print the elements of the linked list for demonstration purposes. - In the
main
method, we create a new instance of theSinglyLinkedList
class, insert nodes into the list, delete the last node, and display the updated list to verify the deletion.
Deleting a node at a specific position in the list
To delete a node at a specific position in a Singly Linked List, you need to follow these steps:
- Check if the list is empty. If it is, there’s nothing to delete.
- Handle the special case of deleting the head node (position 0).
- Traverse the list until you reach the node just before the node to be deleted (position – 1).
- Update the
next
reference of the previous node to skip over the node to be deleted and point directly to its next node. - Optionally, if needed, you may also release the memory occupied by the deleted node.
Here’s the Java code to delete a node at a specific position in a Singly Linked List:
public class SinglyLinkedList {
private Node head; // Head of the linked list
// Node structure
private static class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
// Method to delete a node at a specific position in the list
public void deleteAtPosition(int position) {
if (head == null) {
System.out.println("List is empty. Nothing to delete.");
return;
}
if (position == 0) { // If deleting the head node
head = head.next; // Update the head to the next node
return;
}
Node current = head;
int currentPosition = 0;
while (currentPosition < position - 1 && current != null) {
current = current.next; // Traverse the list until the node just before the desired position
currentPosition++;
}
if (current == null || current.next == null) {
System.out.println("Invalid position.");
return;
}
current.next = current.next.next; // Skip over the node to be deleted
}
// Method to display the linked list
public void display() {
Node current = head; // Start from the head of the list
while (current != null) {
System.out.print(current.data + " "); // Print the data of the current node
current = current.next; // Move to the next node
}
System.out.println(); // Print a newline after displaying all nodes
}
public static void main(String[] args) {
SinglyLinkedList list = new SinglyLinkedList();
list.deleteAtPosition(1); // Output: List is empty. Nothing to delete.
// Insert nodes for demonstration
list.head = new Node(3);
list.head.next = new Node(5);
list.head.next.next = new Node(7);
list.display(); // Output: 3 5 7
list.deleteAtPosition(1); // Delete node at position 1 (value 5)
list.display(); // Output: 3 7
}
}
In this code:
- The
deleteAtPosition
method deletes the node at the specified position in the list by traversing the list until the node just before the desired position and updating itsnext
reference to skip over the node to be deleted. - If the list is empty or the specified position is invalid, appropriate messages are printed.
- The
display
method is used to print the elements of the linked list for demonstration purposes. - In the
main
method, we create a new instance of theSinglyLinkedList
class, insert nodes into the list, delete a node at a specific position, and display the updated list to verify the deletion.
Traversal
Traversing a Singly Linked List involves visiting each node in the list, starting from the head node, and iterating through the list until the last node (where the next
reference is null
). During traversal, you typically perform some operation on each node, such as printing its data or processing it in some way.
Here’s how you can perform traversal on a Singly Linked List in Java:
public class SinglyLinkedList {
private Node head; // Head of the linked list
// Node structure
private static class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
// Method to traverse and display the linked list
public void traverse() {
Node current = head; // Start from the head of the list
while (current != null) {
// Perform some operation on the current node (e.g., print its data)
System.out.print(current.data + " ");
// Move to the next node
current = current.next;
}
// Print a newline after displaying all nodes
System.out.println();
}
public static void main(String[] args) {
SinglyLinkedList list = new SinglyLinkedList();
// Insert nodes for demonstration
list.head = new Node(3);
list.head.next = new Node(5);
list.head.next.next = new Node(7);
// Traverse and display the linked list
list.traverse(); // Output: 3 5 7
}
}
In this code:
- The
traverse
method traverses the linked list by starting from the head node and iterating through each node until thenext
reference isnull
. - Within the loop, you can perform any operation on each node, such as printing its data, processing it, or accessing its properties.
- The
main
method creates an instance of theSinglyLinkedList
class, inserts nodes into the list, and then traverses and displays the linked list to verify the traversal operation.
Searching
Searching in a Singly Linked List involves traversing the list to find a node that matches a given value or satisfies a specific condition. Here’s how you can implement a search operation in a Singly Linked List in Java:
public class SinglyLinkedList {
private Node head; // Head of the linked list
// Node structure
private static class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
// Method to search for a value in the linked list
public boolean search(int value) {
Node current = head; // Start from the head of the list
while (current != null) {
// If the current node's data matches the value, return true
if (current.data == value) {
return true;
}
// Move to the next node
current = current.next;
}
// If the value is not found, return false
return false;
}
public static void main(String[] args) {
SinglyLinkedList list = new SinglyLinkedList();
// Insert nodes for demonstration
list.head = new Node(3);
list.head.next = new Node(5);
list.head.next.next = new Node(7);
// Search for values in the linked list
System.out.println("Search for 5: " + list.search(5)); // Output: true
System.out.println("Search for 10: " + list.search(10)); // Output: false
}
}
In this code:
- The
search
method searches for a given value in the linked list by traversing the list from the head node. - Within the loop, if the current node’s data matches the value being searched for, the method returns
true
. - If the value is not found after traversing the entire list, the method returns
false
. - In the
main
method, we create an instance of theSinglyLinkedList
class, insert nodes into the list, and then search for specific values in the linked list to verify the search operation.
Length of the List
To get the length of a Singly Linked List, you need to traverse the list and count the number of nodes until you reach the end of the list. Here’s how you can implement a method to get the length of a Singly Linked List in Java:
public class SinglyLinkedList {
private Node head; // Head of the linked list
// Node structure
private static class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
// Method to get the length of the linked list
public int getLength() {
int length = 0; // Initialize length to 0
Node current = head; // Start from the head of the list
while (current != null) {
length++; // Increment length for each node
current = current.next; // Move to the next node
}
return length;
}
public static void main(String[] args) {
SinglyLinkedList list = new SinglyLinkedList();
// Insert nodes for demonstration
list.head = new Node(3);
list.head.next = new Node(5);
list.head.next.next = new Node(7);
// Get the length of the linked list
int length = list.getLength();
System.out.println("Length of the linked list: " + length); // Output: Length of the linked list: 3
}
}
In this code:
- The
getLength
method traverses the linked list and counts the number of nodes until the end of the list is reached. - The
length
variable is initialized to 0 and incremented for each node encountered during traversal. - Once the traversal is complete, the method returns the calculated length of the linked list.
- In the
main
method, we create an instance of theSinglyLinkedList
class, insert nodes into the list, and then get the length of the linked list to verify the operation.
Reversing the List
To reverse a Singly Linked List, you need to change the direction of pointers in each node. Here’s how you can implement the reversal of a Singly Linked List in Java:
public class SinglyLinkedList {
private Node head; // Head of the linked list
// Node structure
private static class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
// Method to reverse the linked list
public void reverse() {
Node prev = null; // Previous node
Node current = head; // Current node
Node next = null; // Next node
while (current != null) {
next = current.next; // Store the next node
current.next = prev; // Change the next of current node, reverse the pointer
prev = current; // Move prev to current
current = next; // Move current to next
}
head = prev; // Update the head to the last node, which is now the first node after reversal
}
// Method to display the linked list
public void display() {
Node current = head; // Start from the head of the list
while (current != null) {
System.out.print(current.data + " "); // Print the data of the current node
current = current.next; // Move to the next node
}
System.out.println(); // Print a newline after displaying all nodes
}
public static void main(String[] args) {
SinglyLinkedList list = new SinglyLinkedList();
// Insert nodes for demonstration
list.head = new Node(3);
list.head.next = new Node(5);
list.head.next.next = new Node(7);
System.out.println("Original linked list:");
list.display(); // Output: 3 5 7
// Reverse the linked list
list.reverse();
System.out.println("Reversed linked list:");
list.display(); // Output: 7 5 3
}
}
In this code:
- The
reverse
method iterates through the linked list and reverses the direction of pointers in each node. - Three pointers (
prev
,current
, andnext
) are used to keep track of the previous, current, and next nodes during the reversal process. - Once the reversal is complete, the
head
pointer is updated to point to the last node, which becomes the new head of the reversed list. - In the
main
method, we create an instance of theSinglyLinkedList
class, insert nodes into the list, reverse the list, and then display both the original and reversed linked lists to verify the operation.
Real-world applications where Singly Linked Lists are used
Singly Linked Lists are used in various real-world applications due to their simplicity and efficiency in certain scenarios. Some common applications include:
- Memory Management: Singly Linked Lists are commonly used in memory management systems of operating systems. In dynamic memory allocation, Singly Linked Lists are used to maintain free memory blocks, allowing efficient allocation and deallocation of memory.
- File Systems: Singly Linked Lists are used in file systems to maintain the list of files or directories in a directory. Each node in the list represents a file or directory, with the
next
pointer pointing to the next file or directory in the directory. - Symbol Tables: Singly Linked Lists are used in symbol tables, which are data structures used by compilers and interpreters to store information about variables, functions, and other identifiers in a program. Each node in the list represents an entry in the symbol table.
- Undo Functionality: Singly Linked Lists are used in applications that require undo functionality, such as text editors or drawing programs. Each action performed by the user can be stored as a node in the list, allowing the user to undo previous actions by traversing the list.
- Queue Implementation: Singly Linked Lists are used to implement queues, particularly in applications where dynamic resizing is required. In a queue, each node represents an element in the queue, with the
next
pointer pointing to the next element in the queue. - Music Playlist: Singly Linked Lists can be used to implement music playlists in music player applications. Each node in the list represents a song, with the
next
pointer pointing to the next song in the playlist. - Navigation Systems: Singly Linked Lists are used in navigation systems to represent routes or paths. Each node in the list represents a waypoint or destination, with the
next
pointer pointing to the next waypoint in the route.
These are just a few examples of real-world applications where Singly Linked Lists are used. They are versatile data structures that can be adapted to various scenarios where efficient insertion, deletion, and traversal of elements are required.
Advantages and disadvantages of using Singly Linked Lists in specific scenarios
Singly Linked Lists offer several advantages and disadvantages in specific scenarios:
Advantages:
- Dynamic Size: Singly Linked Lists can dynamically grow and shrink in size, allowing for efficient memory utilization in scenarios where the size of the data structure is not fixed.
- Efficient Insertion and Deletion: Insertion and deletion operations at the beginning of a Singly Linked List are efficient, requiring only a constant time complexity of O(1), regardless of the size of the list.
- Memory Efficiency: Singly Linked Lists use memory more efficiently compared to arrays or other contiguous data structures, as they allocate memory for each node only when needed.
- Versatility: Singly Linked Lists can be used to implement various data structures and algorithms, such as stacks, queues, symbol tables, and more, due to their flexibility and simplicity.
- Ease of Implementation: Singly Linked Lists are relatively easy to implement and understand, making them suitable for educational purposes and for scenarios where simplicity is preferred.
Disadvantages:
- No Random Access: Unlike arrays, Singly Linked Lists do not support random access to elements. Accessing an element at a specific index requires traversing the list from the beginning, resulting in a time complexity of O(n), where n is the number of elements in the list.
- Extra Memory Overhead: Singly Linked Lists require additional memory overhead for storing the
next
pointer in each node, which can lead to higher memory usage compared to contiguous data structures like arrays. - Traversal Overhead: Traversing a Singly Linked List from beginning to end can be slower compared to arrays, especially for large lists, due to the need to follow pointers for each node.
- Lack of Backward Traversal: Singly Linked Lists do not support backward traversal, meaning that accessing the previous node of a given node requires traversing the list from the beginning, resulting in inefficiency in scenarios where backward traversal is required.
- Fragmentation: Singly Linked Lists can suffer from memory fragmentation, especially in scenarios where nodes are frequently allocated and deallocated, leading to inefficient memory usage over time.
In summary, Singly Linked Lists offer advantages such as dynamic size, efficient insertion and deletion, and versatility, but they also have limitations such as lack of random access, extra memory overhead, and traversal overhead, which should be considered when choosing them for specific scenarios.
Conclusion
In conclusion, Singly Linked Lists are fundamental data structures that offer dynamic size, efficient insertion and deletion operations, and versatility in various scenarios. They are commonly used in memory management systems, file systems, symbol tables, and other applications where dynamic resizing and efficient manipulation of data are required.
Despite their advantages, Singly Linked Lists have limitations such as lack of random access, extra memory overhead, and traversal overhead, which can impact their performance in certain scenarios. Additionally, Singly Linked Lists do not support backward traversal, which may be a disadvantage in scenarios where backward traversal is required.
Overall, Singly Linked Lists are valuable tools in the arsenal of data structures, providing a balance between memory efficiency, simplicity, and flexibility. Understanding their strengths and weaknesses is crucial for making informed decisions when choosing data structures for specific applications.
FAQs
What is a Singly Linked List?
A Singly Linked List is a linear data structure consisting of nodes, where each node contains a data element and a pointer/reference to the next node in the sequence. The last node points to null, indicating the end of the list.
How do you insert a node at the beginning of a Singly Linked List?
To insert a node at the beginning of a Singly Linked List, create a new node with the desired data, set its next pointer to the current head of the list, and update the head to point to the new node.
What is the time complexity for searching an element in a Singly Linked List?
The time complexity for searching an element in a Singly Linked List is O(n), where n is the number of nodes in the list. This is because you may need to traverse the entire list to find the desired element.
How do you delete a node from a Singly Linked List?
To delete a node from a Singly Linked List, locate the node to be deleted and adjust the next pointer of the preceding node to skip over the node to be deleted, effectively removing it from the list. Optionally, you may also release the memory occupied by the deleted node.
What are the advantages and disadvantages of using Singly Linked Lists?
Singly Linked Lists offer dynamic size, efficient insertion and deletion at the beginning of the list, and versatility in various scenarios. However, they lack random access, have extra memory overhead, and may suffer from traversal overhead. Understanding these trade-offs is essential when choosing Singly Linked Lists for specific applications.
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
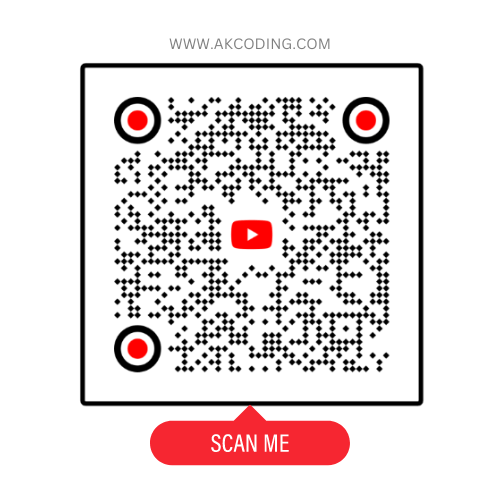