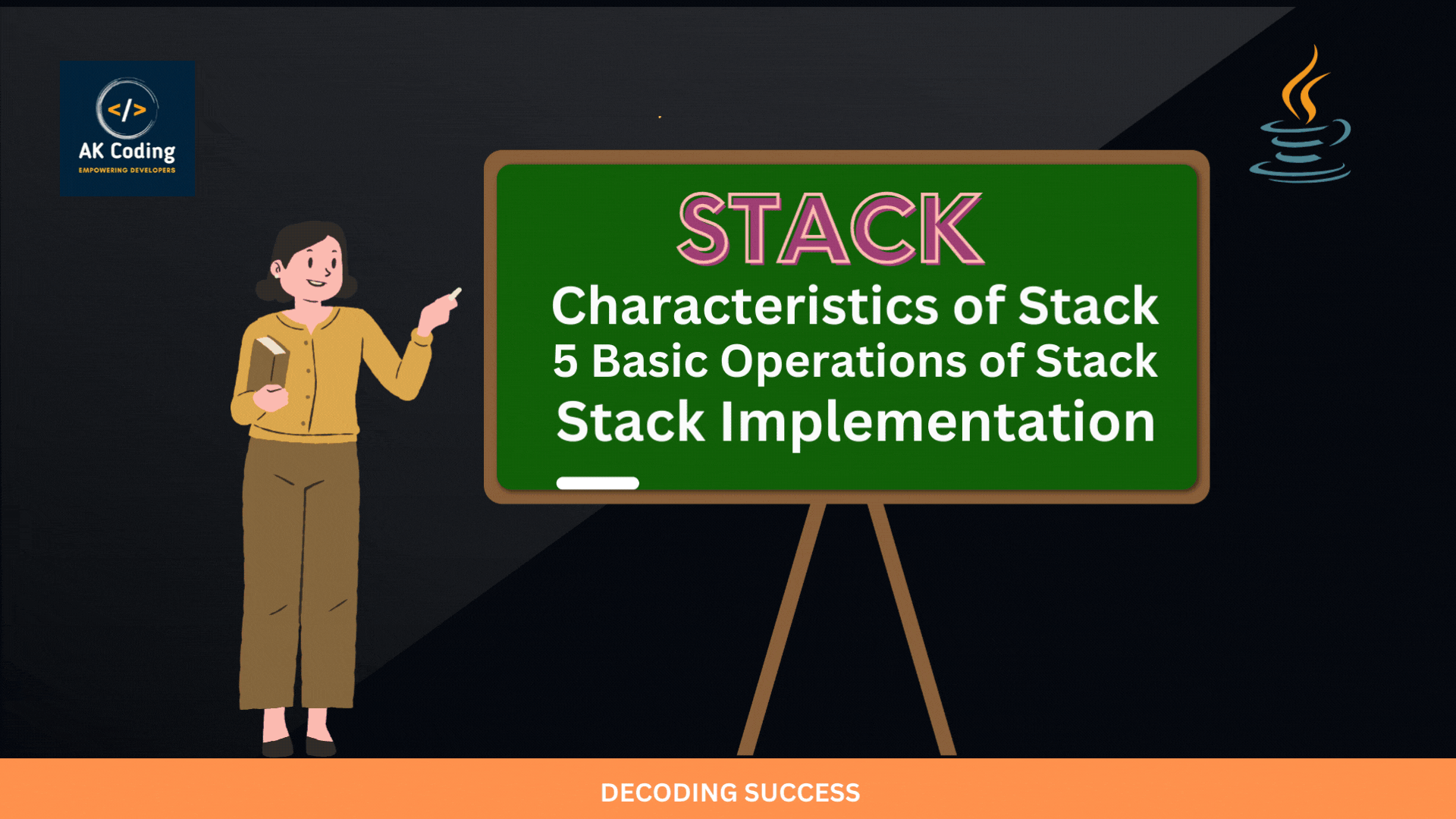
Table of Contents
Introduction to Stack in data structure
Introduction
Stack in data structure are fundamental data structures widely used in computer science and programming. They follow the Last In, First Out (LIFO) principle, where the last element added to the stack is the first one to be removed. This characteristic makes stacks suitable for various applications where elements need to be accessed and processed in a specific order.
Definition of Stack
A stack is a linear data structure that consists of a collection of elements arranged in a sequential order. The key operations on a stack are:
- Push: Adds an element to the top of the stack.
- Pop: Removes and returns the element from the top of the stack.
- Peek (Top): Returns the element at the top of the stack without removing it.
- IsEmpty: Checks if the stack is empty.
- IsFull: Checks if the stack is full (applicable for fixed-size stacks).
Characteristics of Stack
- LIFO Principle: Stacks operate based on the Last In, First Out (LIFO) principle, where the most recently added element is the first one to be removed.
- Single Access Point: Stacks have a single access point, typically referred to as the top of the stack, where all push and pop operations occur.
- Dynamic Size: Stacks can dynamically grow and shrink as elements are added or removed, depending on the implementation.
- Efficient Operations: Push, pop, and peek operations on stacks typically have a time complexity of O(1), making them efficient for certain applications.
- Limited Access: Stacks support limited access to elements. In most cases, only the top element of the stack is accessible for modification or removal.
Applications of Stack
Stacks find applications in various domains and scenarios, including:
- Function Call Stack: Stacks are used in programming languages to manage function calls and maintain the execution context. Each function call creates a stack frame, allowing for nested function calls and proper execution order.
- Expression Evaluation: Stacks are utilized in evaluating arithmetic expressions, postfix expressions, and infix-to-postfix conversions. They help in maintaining the order of operations and resolving parentheses.
- Undo/Redo Functionality: Stacks are employed in implementing undo and redo functionality in text editors, graphic design software, and other applications where users need to revert or redo their actions.
- Backtracking Algorithms: Stacks play a crucial role in backtracking algorithms, such as depth-first search (DFS), maze solving, and pathfinding algorithms. They facilitate the exploration of paths and the backtracking process.
- Memory Management: Stacks are used in memory management systems to allocate and deallocate memory dynamically. They help in managing function call frames, local variables, and dynamic memory allocation.
In summary, stacks are versatile data structures with numerous applications in computer science, software development, and various other fields. Understanding their definition, characteristics, and applications is essential for mastering their usage and leveraging their efficiency in solving real-world problems.
5 Basic Operations of Stack
The basic operations of a stack are fundamental to its functionality and usability. Here are the main operations performed on a stack:
- Push: The push operation adds an element to the top of the stack. It increases the size of the stack by one and places the new element at the top position. After a push operation, the newly added element becomes the top of the stack.
- Pop: The pop operation removes the element from the top of the stack. It decreases the size of the stack by one and returns the removed element. After a pop operation, the element immediately below the removed element becomes the new top of the stack.
- Peek (Top): The peek operation returns the element at the top of the stack without removing it. It allows you to access the top element of the stack without modifying the stack’s contents.
- IsEmpty: The isEmpty operation checks whether the stack is empty or not. It returns true if the stack contains no elements (i.e., its size is zero), and false otherwise.
- IsFull: The isFull operation checks whether the stack is full or not. It is applicable only for fixed-size stacks with a maximum capacity. It returns true if the stack is full (i.e., its size is equal to its maximum capacity), and false otherwise.
These operations collectively provide the essential functionality required to manipulate and manage elements within a stack. They form the foundation for implementing various algorithms, data structures, and applications that rely on stack-based processing. Proper implementation and utilization of these operations ensure the efficient and effective use of stacks in solving real-world problems across diverse domains.
Stack Implementation
A. Array-based Implementation
Array-based stack implementation involves using an array to store the elements of the stack. Here’s a step-by-step guide to implementing a stack using arrays in C:
- Define Constants: If you’re working with a fixed-size stack, define constants for the maximum size of the stack.
- Define Stack Structure: Create a structure to represent the stack. This structure should contain the array to store elements, a variable to keep track of the top of the stack, and possibly other metadata such as the maximum size of the stack.
- Initialize Stack: Initialize the stack by allocating memory for the array and setting the top of the stack to -1 (indicating an empty stack).
- Push Operation: Implement the push operation to add elements to the stack. This involves incrementing the top of the stack and storing the new element at the top position.
- Pop Operation: Implement the pop operation to remove elements from the stack. This involves returning the element at the top of the stack, decrementing the top of the stack, and optionally freeing memory if needed.
- Peek Operation: Implement the peek operation to retrieve the element at the top of the stack without removing it. This involves returning the element at the current top position.
- IsEmpty Operation: Implement the isEmpty operation to check if the stack is empty. This involves checking if the top of the stack is -1.
- IsFull Operation (Optional): If you’re working with a fixed-size stack, implement the isFull operation to check if the stack is full. This involves checking if the top of the stack is equal to the maximum size of the stack minus one.
- Handling Overflow and Underflow
- Cleanup: Free any dynamically allocated memory and perform any necessary cleanup operations when the stack is no longer needed.
Here’s a sample implementation of an array-based stack in C:
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100 // Maximum size of the stack
// Stack structure
typedef struct {
int arr[MAX_SIZE];
int top;
} Stack;
// Initialize stack
void initStack(Stack *stack) {
stack->top = -1; // Empty stack
}
// Push operation
void push(Stack *stack, int value) {
if (stack->top == MAX_SIZE - 1) {
printf("Stack Overflow\n");
return;
}
stack->arr[++stack->top] = value;
}
// Pop operation
int pop(Stack *stack) {
if (stack->top == -1) {
printf("Stack Underflow\n");
exit(1);
}
return stack->arr[stack->top--];
}
// Peek operation
int peek(Stack *stack) {
if (stack->top == -1) {
printf("Stack is empty\n");
exit(1);
}
return stack->arr[stack->top];
}
// IsEmpty operation
int isEmpty(Stack *stack) {
return stack->top == -1;
}
// Main function for testing
int main() {
Stack stack;
initStack(&stack);
push(&stack, 10);
push(&stack, 20);
push(&stack, 30);
printf("Peek: %d\n", peek(&stack));
while (!isEmpty(&stack)) {
printf("Popped: %d\n", pop(&stack));
}
return 0;
}
In this implementation, we define a stack structure containing an array arr
to store elements and a variable top
to keep track of the top of the stack. We then implement the basic stack operations – push, pop, peek, and isEmpty – as described above. Finally, we test the stack operations in the main
function.
B. Linked List-based Implementation
Implementing a stack using a linked list involves creating a linked list where each node stores an element of the stack. Here’s a step-by-step guide to implementing a linked list-based stack in C:
- Define Node Structure: Create a structure to represent a node in the linked list. Each node should contain a data field to store the element and a pointer field to point to the next node.
- Define Stack Structure: Create a structure to represent the stack. This structure should contain a pointer to the top node of the linked list.
- Push Operation: Implement the push operation to add elements to the stack. This involves creating a new node, setting its data field to the new element, and updating pointers to maintain the stack’s structure.
- Pop Operation: Implement the pop operation to remove elements from the stack. This involves removing the top node from the linked list, returning its data field, and updating pointers accordingly.
- Peek Operation: Implement the peek operation to retrieve the element at the top of the stack without removing it. This involves returning the data field of the top node.
- IsEmpty Operation: Implement the isEmpty operation to check if the stack is empty. This involves checking if the top pointer is NULL.
- Cleanup: Free any dynamically allocated memory and perform any necessary cleanup operations when the stack is no longer needed.
Here’s a sample implementation of a linked list-based stack in C:
#include <stdio.h>
#include <stdlib.h>
// Node structure
typedef struct Node {
int data;
struct Node *next;
} Node;
// Stack structure
typedef struct {
Node *top;
} Stack;
// Initialize stack
void initStack(Stack *stack) {
stack->top = NULL; // Empty stack
}
// Push operation
void push(Stack *stack, int value) {
Node *newNode = (Node *)malloc(sizeof(Node));
if (newNode == NULL) {
printf("Memory allocation failed\n");
exit(1);
}
newNode->data = value;
newNode->next = stack->top;
stack->top = newNode;
}
// Pop operation
int pop(Stack *stack) {
if (stack->top == NULL) {
printf("Stack Underflow\n");
exit(1);
}
Node *temp = stack->top;
int value = temp->data;
stack->top = temp->next;
free(temp);
return value;
}
// Peek operation
int peek(Stack *stack) {
if (stack->top == NULL) {
printf("Stack is empty\n");
exit(1);
}
return stack->top->data;
}
// IsEmpty operation
int isEmpty(Stack *stack) {
return stack->top == NULL;
}
// Main function for testing
int main() {
Stack stack;
initStack(&stack);
push(&stack, 10);
push(&stack, 20);
push(&stack, 30);
printf("Peek: %d\n", peek(&stack));
while (!isEmpty(&stack)) {
printf("Popped: %d\n", pop(&stack));
}
return 0;
}
In this implementation, we define a node structure to represent a node in the linked list and a stack structure containing a pointer to the top node of the linked list. We then implement the basic stack operations – push, pop, peek, and isEmpty – as described above. Finally, we test the stack operations in the main
function.
Time Complexity Analysis of Stack
Time complexity analysis of stack operations helps us understand the efficiency of various implementations. Here’s a breakdown of the time complexity for stack operations in both array-based and linked list-based implementations:
Array-based Implementation:
- Push Operation:
- Time Complexity: O(1)
- Explanation: In the array-based implementation, pushing an element onto the stack involves inserting the element at the top position of the array. Since arrays provide direct access to elements based on indices, adding an element to the top of the stack takes constant time.
- Pop Operation:
- Time Complexity: O(1)
- Explanation: Popping an element from the stack in an array-based implementation entails removing the element from the top position of the array. Since arrays allow direct access to elements, removing an element from the top of the stack also takes constant time.
- Peek Operation:
- Time Complexity: O(1)
- Explanation: The peek operation involves accessing the element at the top of the stack without removing it. Since arrays provide direct access to elements based on indices, accessing the top element of the stack also takes constant time.
- IsEmpty Operation:
- Time Complexity: O(1)
- Explanation: The isEmpty operation simply checks whether the top index of the stack is -1 (indicating an empty stack). This operation involves a single comparison and therefore takes constant time.
- IsFull Operation (Optional for Fixed-size Stacks):
- Time Complexity: O(1)
- Explanation: For fixed-size stacks, the isFull operation checks whether the top index of the stack is equal to the maximum size of the stack minus one. This operation involves a single comparison and therefore takes constant time.
Linked List-based Implementation:
- Push Operation:
- Time Complexity: O(1)
- Explanation: In the linked list-based implementation, pushing an element onto the stack involves creating a new node and updating pointers to insert the node at the beginning of the linked list. Since linked lists allow constant-time insertion at the beginning, pushing an element onto the stack takes constant time.
- Pop Operation:
- Time Complexity: O(1)
- Explanation: Popping an element from the stack in a linked list-based implementation entails removing the first node from the linked list and updating pointers accordingly. Since linked lists allow constant-time removal from the beginning, popping an element from the stack also takes constant time.
- Peek Operation:
- Time Complexity: O(1)
- Explanation: The peek operation involves accessing the data field of the first node in the linked list without removing it. Since linked lists allow constant-time access to the first node, accessing the top element of the stack also takes constant time.
- IsEmpty Operation:
- Time Complexity: O(1)
- Explanation: The isEmpty operation simply checks whether the top pointer of the stack is NULL (indicating an empty stack). This operation involves a single comparison and therefore takes constant time.
In summary, both array-based and linked list-based implementations of stacks offer constant-time complexity for basic stack operations such as push, pop, peek, and isEmpty. However, the choice between these implementations may depend on factors such as memory usage, dynamic resizing requirements, and specific application constraints.
Common Use Cases and Applications
Stacks find numerous applications in computer science, software engineering, and various other fields due to their versatility and simplicity. Some common use cases and applications of stacks include:
- Function Call Stack: Stacks are extensively used in programming languages to manage function calls and maintain the execution context. Each function call creates a stack frame, which contains information such as local variables, return addresses, and function parameters. When a function is called, its stack frame is pushed onto the call stack, and when the function returns, its stack frame is popped from the stack.
- Expression Evaluation: Stacks are employed in evaluating arithmetic expressions, postfix expressions, and infix-to-postfix conversions. In infix-to-postfix conversion, a stack is used to store operators temporarily and ensure the correct order of operations. Similarly, in postfix expression evaluation, a stack is used to store operands and intermediate results while processing the expression.
- Backtracking Algorithms: Stacks play a crucial role in backtracking algorithms, such as depth-first search (DFS) and backtracking-based problem-solving techniques. In DFS, a stack is used to keep track of the nodes to be explored, allowing for efficient traversal of graphs and trees. Backtracking algorithms, such as the N-queens problem and maze solving, utilize stacks to store partial solutions and backtrack when necessary.
- Undo/Redo Functionality: Stacks are utilized in implementing undo and redo functionality in text editors, graphic design software, and other applications where users need to revert or redo their actions. Each user action, such as typing, deleting, or formatting, is recorded as a command object and pushed onto the undo stack. When the user requests an undo operation, the command objects are popped from the undo stack and executed in reverse order.
- Expression Parsing and Syntax Analysis: Stacks are used in lexical analysis, parsing, and syntax analysis phases of compilers and interpreters. In these phases, stacks are employed to implement algorithms such as recursive descent parsing, operator precedence parsing, and LR parsing. Stacks help in maintaining the parsing state, tracking nested structures, and enforcing syntactic rules.
- Memory Management: Stacks are involved in memory management systems to allocate and deallocate memory dynamically. Stacks are used to manage function call frames, local variables, and dynamic memory allocation. In languages like C and C++, local variables and function parameters are typically stored on the stack, while heap-allocated memory is managed separately.
- Algorithmic Problems: Stacks are used to solve various algorithmic problems and puzzles, including tower of Hanoi, parentheses matching, histogram area calculation, and postfix expression evaluation. Stacks provide a natural and efficient way to handle problems that involve nested structures, recursive processing, or last-in, first-out (LIFO) order of elements.
In summary, stacks are indispensable data structures with a wide range of applications in programming, software development, algorithm design, and beyond. Understanding the principles of stacks and their applications is essential for mastering problem-solving skills and building efficient and elegant solutions to real-world problems.
Stack Comparison with Other Data Structures
Here’s a comparison between stacks and other data structures:
Feature | Stack | Queue | Linked List | Array |
---|---|---|---|---|
Definition | A linear data structure following the Last In, First Out (LIFO) principle. | A linear data structure following the First In, First Out (FIFO) principle. | A linear data structure consisting of a sequence of elements connected by pointers. | A collection of elements stored in contiguous memory locations. |
Basic Operations | Push (add element), Pop (remove element), Peek (get top element), IsEmpty | Enqueue (add element), Dequeue (remove element), Peek (get front element), IsEmpty | Insert (add element at any position), Delete (remove element at any position), Search, Insertion at the beginning/end, Deletion at the beginning/end | Insert (add element at any position), Delete (remove element at any position), Access elements by index, Resize |
Data Organization | Elements are stored in a single column, with access limited to the topmost element. | Elements are stored in a single column, with access limited to both ends (front and rear). | Elements are stored in individual nodes connected by pointers, allowing for flexible insertion and deletion. | Elements are stored in contiguous memory locations, with access based on indices. |
Memory Allocation | Dynamic memory allocation for each element added to the stack. | Dynamic memory allocation for each element added to the queue. | Dynamic memory allocation for each node added to the linked list. | Static memory allocation for a fixed-size array or dynamic memory allocation for a resizable array. |
Efficiency | Efficient for operations such as push, pop, and peek (O(1) time complexity). | Efficient for operations such as enqueue, dequeue, and peek (O(1) time complexity). | Efficient for insertion and deletion at any position (O(1) time complexity with pointers). | Efficient for random access (O(1) time complexity) and resizing (O(n) time complexity). |
Use Cases | Function call stack, expression evaluation, backtracking algorithms, undo/redo functionality. | Process scheduling, buffer management, breadth-first search (BFS) algorithms. | Dynamic data storage, memory management, implementation of other data structures. | Dynamic arrays, lists with fixed size or known maximum capacity, implementation of matrices. |
This comparison highlights the key differences and similarities between stacks and other commonly used data structures, including queues, linked lists, and arrays. Understanding these differences is crucial for choosing the appropriate data structure based on specific requirements and performance considerations.
Best Practices and Considerations of Stack
When working with stacks, there are several best practices and considerations to keep in mind to ensure efficient and effective usage. Here are some important ones:
- Choose the Right Implementation: Depending on your specific requirements and constraints, choose between array-based and linked list-based implementations of stacks. Consider factors such as memory usage, dynamic resizing needs, and time complexity of operations.
- Limit the Scope of Access: Stacks typically provide limited access to elements, with operations restricted to the top of the stack. Avoid exposing internal implementation details or providing direct access to stack elements beyond the top.
- Handle Stack Overflow and Underflow: Implement mechanisms to handle stack overflow (e.g., in array-based implementations) and stack underflow (e.g., in pop operations). Handle edge cases gracefully and provide appropriate error handling mechanisms.
- Manage Memory Efficiently: Be mindful of memory allocation and deallocation when working with dynamic memory structures (e.g., linked list-based stacks). Free memory resources promptly when elements are removed from the stack to avoid memory leaks.
- Consider Thread Safety: If your application involves concurrent access to stacks from multiple threads, ensure thread safety by implementing appropriate synchronization mechanisms (e.g., mutexes, locks) to prevent race conditions and data corruption.
- Optimize for Performance: Optimize stack operations for performance by minimizing unnecessary memory allocations, reducing the number of function calls, and avoiding redundant operations. Profile your code and identify bottlenecks to optimize critical sections.
- Use Stacks for Appropriate Use Cases: Understand the strengths and limitations of stacks and use them for suitable use cases where their LIFO (Last In, First Out) behavior and limited access provide the desired functionality and efficiency.
- Document and Maintain Code: Document your stack implementation thoroughly, including the rationale behind design decisions, data structures used, and time complexities of operations. Maintain clean, well-structured code with meaningful variable names and clear comments to aid readability and maintainability.
- Test Extensively: Test your stack implementation rigorously with various input scenarios, including edge cases, boundary conditions, and stress testing. Verify correctness, performance, and robustness to ensure reliable behavior in real-world usage scenarios.
- Consider Alternative Data Structures: Evaluate alternative data structures (e.g., queues, linked lists, arrays) and choose the most suitable one based on specific requirements, performance considerations, and trade-offs. Don’t hesitate to explore alternative approaches if stacks prove inadequate for your needs.
By adhering to these best practices and considerations, you can harness the power of stacks effectively in your applications, ensuring robustness, performance, and scalability.
Real-world Examples and Case Study of Stack
Stacks have numerous real-world applications across various domains. Here are some examples and case studies showcasing the practical use of stacks:
- Web Browser History:
- Web browsers use stacks to implement the backward and forward navigation functionality. Each time a user visits a webpage, the URL is pushed onto a stack. When the user clicks the back button, the browser pops the previous URL from the stack to navigate to the previous page. Similarly, the forward button navigates forward by popping URLs from a separate stack.
- Text Editors (Undo/Redo Functionality):
- Text editors utilize stacks to implement undo and redo functionality, allowing users to revert or redo their actions. Each editing operation (e.g., typing, deleting, formatting) is recorded as a command object and pushed onto the undo stack. When the user requests an undo operation, the command objects are popped from the undo stack and executed in reverse order.
- Function Call Stack:
- Programming languages use stacks to manage function calls and maintain the execution context during program execution. Each function call creates a stack frame, which contains information such as local variables, return addresses, and function parameters. When a function is called, its stack frame is pushed onto the call stack, and when the function returns, its stack frame is popped from the stack.
- Expression Evaluation:
- Stacks are used in compilers and interpreters to evaluate arithmetic expressions, postfix expressions, and infix-to-postfix conversions. In infix-to-postfix conversion, a stack is used to store operators temporarily and ensure the correct order of operations. Similarly, in postfix expression evaluation, a stack is used to store operands and intermediate results while processing the expression.
- Backtracking Algorithms:
- Backtracking algorithms, such as depth-first search (DFS) and maze solving, utilize stacks to store partial solutions and backtrack when necessary. In DFS, a stack is used to keep track of the nodes to be explored, allowing for efficient traversal of graphs and trees. Maze-solving algorithms also use stacks to store the current path and backtrack when a dead end is encountered.
- Memory Management:
- Stacks are involved in memory management systems to allocate and deallocate memory dynamically. Stacks are used to manage function call frames, local variables, and dynamic memory allocation. In languages like C and C++, local variables and function parameters are typically stored on the stack, while heap-allocated memory is managed separately.
- Browser Tab Management:
- Web browsers use stacks to manage browser tab history and the navigation stack. When a user opens a new tab or navigates to a different webpage, the URL is pushed onto a stack. The user can then navigate backward and forward through the tab history using the browser’s navigation buttons, which pop URLs from the stack.
These examples demonstrate the versatility and practicality of stacks in various applications, ranging from web browsing and text editing to programming and algorithm design. Stacks provide an elegant and efficient solution for managing data in a Last In, First Out (LIFO) manner, making them indispensable in modern computing environments.
Conclusion
Conclusion of Stack:
In conclusion, stacks are fundamental data structures that play a crucial role in computer science, software engineering, and various other fields. They provide a simple and efficient way to manage data in a Last In, First Out (LIFO) manner, allowing for streamlined operations such as push, pop, peek, and isEmpty. Stacks find numerous real-world applications, ranging from web browsing and text editing to memory management and algorithm design. Understanding the principles of stacks and their applications is essential for mastering problem-solving skills and building efficient solutions to real-world problems.
Summary of Key Concepts:
- Stacks follow the Last In, First Out (LIFO) principle, where the most recently added element is the first one to be removed.
- Basic stack operations include push (add element), pop (remove element), peek (get top element), and isEmpty (check if stack is empty).
- Stacks can be implemented using arrays or linked lists, each with its own advantages and trade-offs.
- Common applications of stacks include function call stack, expression evaluation, backtracking algorithms, undo/redo functionality, and memory management.
- Stacks are efficient data structures for managing data in a LIFO manner, providing constant-time complexity for basic operations in most cases.
Importance of Stacks in Data Structures:
Stacks are indispensable components of data structures and algorithms due to their simplicity, efficiency, and versatility. They serve as building blocks for more complex data structures and algorithms, enabling efficient problem-solving in various domains. Understanding stacks is essential for mastering fundamental concepts in computer science and software engineering, as well as for developing robust and scalable software solutions.
Further Learning Resources:
If you’re interested in further exploring stacks and their applications, here are some recommended resources:
- “Data Structures and Algorithms in Java” by Robert Lafore – This book provides a comprehensive introduction to data structures and algorithms, including detailed explanations of stacks and their implementations in Java.
- “Introduction to Algorithms” by Thomas H. Cormen et al. – This classic textbook covers a wide range of algorithms and data structures, including stacks, with rigorous analysis and practical examples.
- Online Courses: Platforms like Coursera, edX, and Udemy offer various courses on data structures and algorithms, many of which include dedicated modules on stacks and related topics.
- Coding Practice Platforms: Websites like LeetCode, HackerRank, and CodeSignal offer a plethora of coding challenges and exercises focused on stacks and other data structures. Practicing on these platforms can help reinforce your understanding and improve your problem-solving skills.
- Open-Source Projects: Exploring open-source projects on GitHub or contributing to stack-related projects can provide hands-on experience and deeper insights into real-world applications of stacks.
By leveraging these resources and actively practicing your skills, you can deepen your understanding of stacks and become proficient in using them effectively in your projects and applications.
FAQs
Certainly! Here are some frequently asked questions (FAQ) about stack:
- What is a stack?
- A stack is a linear data structure that follows the Last In, First Out (LIFO) principle, where the last element added is the first one to be removed. It supports two primary operations: push (to add an element) and pop (to remove an element).
- How is a stack implemented?
- Stacks can be implemented using arrays or linked lists. In an array-based implementation, elements are stored in a fixed-size or dynamic array, with a pointer (top) indicating the top of the stack. In a linked list-based implementation, elements are stored in nodes, each pointing to the next node, with a pointer (top) pointing to the top node.
- What are the basic operations on a stack?
- The basic operations on a stack include:
- Push: Adds an element to the top of the stack.
- Pop: Removes and returns the element from the top of the stack.
- Peek: Returns the element at the top of the stack without removing it.
- IsEmpty: Checks if the stack is empty.
- IsFull (for array-based implementations): Checks if the stack is full.
- What are the real-world applications of stacks?
- Stacks have various real-world applications, such as managing function calls in programming languages, implementing undo/redo functionality in text editors, navigating web browser history, evaluating arithmetic expressions, and solving algorithmic problems like tower of Hanoi and maze traversal.
- What is the difference between a stack and a queue?
- While both stacks and queues are linear data structures, they differ in their principle of operation. Stacks follow the Last In, First Out (LIFO) principle, where the last element added is the first one to be removed. Queues, on the other hand, follow the First In, First Out (FIFO) principle, where the first element added is the first one to be removed.
- Can a stack be empty and full at the same time?
- No, a stack cannot be both empty and full simultaneously. If a stack is empty, it means it contains no elements, and if it is full (for array-based implementations with a fixed size), it means it has reached its maximum capacity and cannot accommodate additional elements.
- How is stack memory managed in programming languages?
- In programming languages like C and C++, stack memory is used for storing local variables, function parameters, and return addresses during function calls. The memory allocation and deallocation for stack variables are managed automatically by the compiler/runtime system.
- Are stacks thread-safe?
- Stacks themselves are not inherently thread-safe data structures. If multiple threads access a shared stack concurrently, synchronization mechanisms such as locks or mutexes must be used to ensure thread safety and prevent race conditions.
These are some of the commonly asked questions about stacks, providing a basic understanding of their concepts, operations, and applications.
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
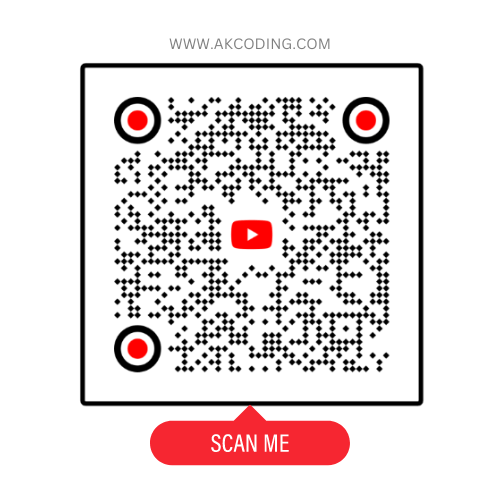