Java 8 Interview Questions for 10 Years Experience, the interview questions will likely delve deeper into Java 8 features, advanced concepts, design patterns, and best practices. Here are some Java 8 interview questions tailored for a candidate with significant experience
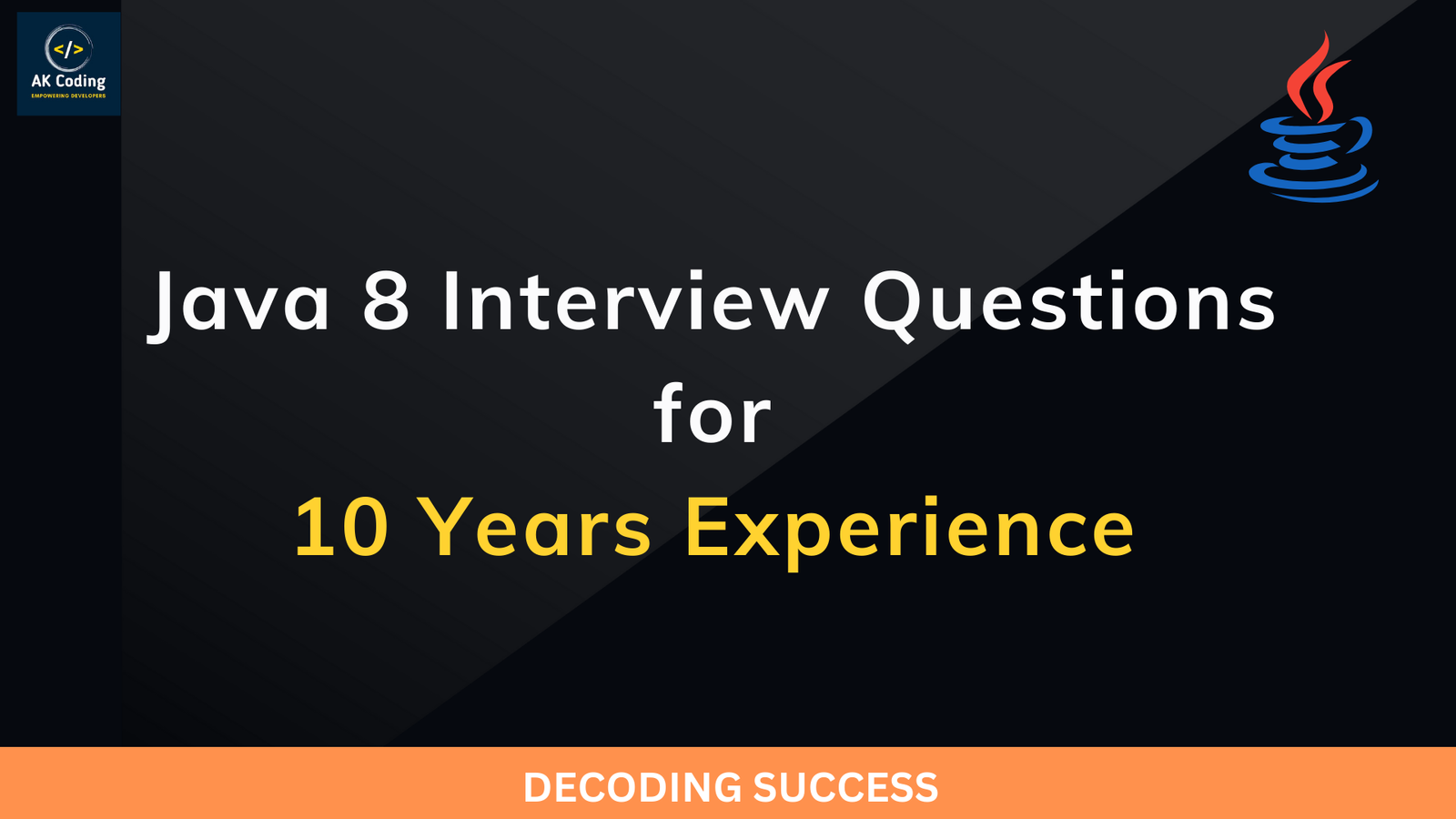
Java 8 Interview Questions for 10 Years Experience
Table of Contents
1. What are the main features introduced in Java 8?
Java 8 introduced several significant features and enhancements to the Java programming language and platform. Some of the main features include:
- Lambda Expressions: Lambda expressions allow the concise representation of anonymous functions, enabling functional programming paradigms in Java. They provide a way to pass behavior as an argument to methods, making code more expressive and readable.
- Stream API: The Stream API provides a fluent and declarative way to process collections of objects in a functional style. Streams enable operations like filtering, mapping, and reducing elements, making it easier to write concise and efficient code for data processing tasks.
- Functional Interfaces: Java 8 introduced the
@FunctionalInterface
annotation to define functional interfaces, interfaces with a single abstract method. Functional interfaces facilitate the use of lambda expressions and method references, enabling functional programming patterns. - Default Methods: Default methods allow interfaces to have method implementations, enabling backward compatibility without breaking existing implementations. This feature facilitates the addition of new methods to interfaces in evolving APIs.
- Method References: Method references provide a shorthand syntax for referring to methods or constructors using the
::
operator. They simplify code by allowing developers to reuse existing method implementations as lambda expressions. - Optional: The
Optional
class provides a container object to represent an optional value or absence of a value. It encourages better handling of null values and reduces the risk ofNullPointerExceptions
by forcing developers to explicitly handle the absence of a value. - Date and Time API: The Date and Time API (
java.time
) introduced in Java 8 provides comprehensive and thread-safe classes for date and time manipulation. It addresses the limitations and design flaws of the legacyjava.util.Date
andjava.util.Calendar
classes. - CompletableFuture: The CompletableFuture class facilitates asynchronous programming by representing a future result of an asynchronous computation. It supports a wide range of asynchronous operations and enables composition of asynchronous tasks using fluent APIs.
These features introduced in Java 8 revolutionized the way Java developers write code, promoting functional programming principles, improving code readability and maintainability, and enhancing support for concurrent and asynchronous programming.
2. Explain lambda expressions in Java 8 and provide an example of their usage
Lambda expressions in Java 8 provide a concise way to represent anonymous functions, enabling functional programming paradigms within the language. A lambda expression consists of a list of parameters, an arrow token ->
, and a body. They are particularly useful for implementing single-method interfaces (functional interfaces) and for passing behavior as arguments to methods. Lambda expressions help in reducing boilerplate code and making the codebase more readable and maintainable.
Here’s a basic syntax of a lambda expression:
//Java 8 Interview Questions for 10 Years Experience
(parameters) -> expression
And here’s how you might use a lambda expression to represent a simple function that doubles an integer:
//Java 8 Interview Questions for 10 Years Experience
// Lambda expression to double a number
Function<Integer, Integer> doubleNumber = (num) -> num * 2;
// Using the lambda expression
int result = doubleNumber.apply(5); // Result will be 10
//Java 8 Interview Questions for 10 Years Experience
In this example:
(num)
specifies the parameter of the lambda expression.->
denotes the arrow token, separating the parameters from the body.num * 2
is the expression that defines the behavior of the lambda. It takes the inputnum
and returns its double.
Lambda expressions are commonly used in conjunction with functional interfaces, which are interfaces with only one abstract method. In this case, the lambda expression can be assigned to a reference of the functional interface type, as shown in the example above with Function<Integer, Integer>
.
3. What is the Stream API in Java 8? How does it differ from collections?
The Stream API in Java 8 provides a powerful and flexible way to process collections of data in a functional style. Unlike traditional collections, which represent data structures holding elements, streams represent a sequence of elements that can be processed sequentially or in parallel. Streams support functional-style operations such as filtering, mapping, reducing, and aggregating elements, enabling concise and expressive code for data processing tasks.
Here are some key differences between the Stream API and collections:
- Mutability: Collections are mutable data structures that hold elements and support operations like adding, removing, and modifying elements. In contrast, streams are immutable sequences of elements that cannot be modified. Instead, stream operations produce new streams as output, leaving the original stream unchanged.
- Eager vs. Lazy Evaluation: Collections perform eager evaluation, meaning that operations are executed immediately when invoked. In contrast, streams support lazy evaluation, where operations are deferred until the terminal operation is called. This allows for more efficient processing, as only the necessary elements are processed at each stage.
- Parallelism: Collections do not inherently support parallel processing, requiring explicit parallelization using techniques like multithreading. Streams, on the other hand, support parallel processing out of the box, allowing for easy parallelization of operations across multiple threads using the
parallel()
method. - Intermediate and Terminal Operations: Collections offer a set of methods for data manipulation, such as
add
,remove
, andget
. Streams provide two types of operations: intermediate operations, which transform the elements of the stream and return a new stream, and terminal operations, which produce a result or side effect and terminate the stream.
Overall, the Stream API in Java 8 offers a higher-level abstraction for data processing compared to traditional collections, promoting functional programming paradigms and enabling more expressive and efficient code for data manipulation tasks.
4. How do you handle null values in Java 8?
In Java 8, there are several approaches for handling null values effectively:
- Optional Class: The
Optional
class introduced in Java 8 provides a container object that may or may not contain a non-null value. It encourages a more explicit handling of null values, reducing the risk ofNullPointerExceptions
. Developers can use methods likeisPresent()
,get()
,orElse()
,orElseGet()
, andorElseThrow()
to work withOptional
objects safely. - Null Checks: Traditional null checks can still be used to handle null values. However, care must be taken to ensure proper null checks are performed before accessing potentially null references to avoid
NullPointerExceptions
. - Null Object Pattern: Instead of using null values, consider using the null object pattern, where a special “null” object is used to represent absence of a value. This approach can help avoid the need for explicit null checks and improve code clarity.
- JavaBean Convention: When working with JavaBeans, adhere to the convention of providing appropriate default values or setter methods that validate input parameters to prevent setting null values where not allowed.
Overall, choosing the appropriate approach depends on the specific requirements and design considerations of the application. Using Optional
and avoiding null values where possible can lead to more robust and readable code, while traditional null checks and null object pattern may be suitable in certain contexts.
5. Explain the Date and Time API introduced in Java 8.
The Date and Time API introduced in Java 8, located in the java.time
package, provides a comprehensive set of classes for representing dates, times, durations, and intervals, while addressing the limitations and design flaws of the legacy java.util.Date
and java.util.Calendar
classes. Here are some key features of the Date and Time API:
- Immutable Classes: The Date and Time API introduces immutable classes such as
LocalDate
,LocalTime
, andLocalDateTime
to represent date, time, and date-time values respectively. These classes are thread-safe and provide methods for performing various operations like addition, subtraction, comparison, and formatting. - Separation of Concerns: The API separates the representation of date, time, and time zone information, making it easier to work with different aspects of date and time separately. This helps in avoiding common pitfalls related to time zone handling and daylight saving time changes.
- Support for Time Zones: The API provides classes like
ZoneId
andZoneOffset
to represent time zone information and handle time zone conversions. It also includes classes likeZonedDateTime
andOffsetDateTime
to represent date-time values with time zone information. - Parsing and Formatting: The API offers built-in support for parsing and formatting date-time strings using classes like
DateTimeFormatter
, which provides a flexible and customizable way to parse and format date-time values according to different patterns and locales. - Arithmetic Operations: The API provides methods for performing arithmetic operations on date-time values, such as adding or subtracting durations or periods, calculating the difference between two date-time values, and adjusting date-time values based on different criteria like day-of-week or month.
Overall, the Date and Time API in Java 8 simplifies date and time manipulation, improves code readability and maintainability, and provides better support for internationalization and time zone handling compared to the legacy date and time classes.
6. What are default and static methods in interfaces, and how do they impact the design of APIs?
Default and static methods were introduced in Java 8 to enhance the functionality of interfaces without breaking backward compatibility. Here’s an explanation of each and how they impact API design:
- Default Methods:
- Default methods allow interfaces to provide method implementations, enabling backward compatibility by allowing existing interfaces to evolve without breaking implementing classes.
- They are declared using the
default
keyword and can be overridden by implementing classes. Interfaces can have multiple default methods. - Default methods enable the addition of new methods to interfaces without forcing implementing classes to implement them, providing flexibility and allowing for incremental changes to interfaces over time.
- Static Methods:
- Static methods in interfaces allow the declaration of utility methods that are associated with the interface but do not require an instance of the implementing class.
- They are declared using the
static
keyword and cannot be overridden by implementing classes. Interfaces can have multiple static methods. - Static methods provide a way to organize utility methods related to the interface’s functionality, improving code organization and readability.
Impact on API Design:
- Default and static methods impact API design by allowing interfaces to evolve over time without breaking existing implementations. They provide a mechanism for adding new functionality to interfaces without requiring all implementing classes to implement the new methods.
- Default methods are particularly useful for adding new methods to existing interfaces without affecting the existing codebase, enabling backward compatibility and facilitating API evolution.
- Static methods provide a way to organize utility methods related to the interface’s functionality, promoting code organization and encapsulation of related functionalities within the interface itself.
Overall, default and static methods in interfaces enhance the flexibility, extensibility, and maintainability of APIs, allowing for smoother evolution and improved code organization. However, care should be taken to use them judiciously and in accordance with good API design principles to ensure clarity and consistency in the API surface.
7. How does CompletableFuture facilitate asynchronous programming in Java 8?
CompletableFuture
in Java 8 facilitates asynchronous programming by providing a powerful and flexible way to represent and compose asynchronous computations, enabling developers to write non-blocking and efficient code. Here’s how CompletableFuture
facilitates asynchronous programming:
- Asynchronous Computation:
CompletableFuture
represents a future result of an asynchronous computation that may be completed at some point in the future. It allows tasks to execute asynchronously in separate threads, freeing up the calling thread to perform other operations while waiting for the result. - Composition:
CompletableFuture
supports fluent APIs for composing multiple asynchronous tasks sequentially or in parallel. Tasks can be chained together using methods likethenApply
,thenCompose
, andthenCombine
, allowing for the creation of complex asynchronous workflows. - Exception Handling:
CompletableFuture
provides methods for handling exceptions thrown during asynchronous computation, such asexceptionally
andhandle
. This allows developers to handle errors gracefully and propagate exceptions through the asynchronous pipeline. - Timeouts and Cancellation:
CompletableFuture
supports timeout mechanisms and cancellation of asynchronous tasks using methods likecompleteOnTimeout
andcancel
. This enables developers to control the execution of asynchronous tasks and prevent them from running indefinitely. - Integration with CompletionStage:
CompletableFuture
implements theCompletionStage
interface, allowing for interoperability with other asynchronous APIs in Java 8, such asCompletionStage
methods provided byCompletableFuture
andCompletionStage
methods from other asynchronous libraries.
Overall, CompletableFuture
simplifies asynchronous programming in Java by providing a high-level abstraction for representing and composing asynchronous computations, enabling developers to write non-blocking and efficient code with ease. It promotes a more functional and declarative style of asynchronous programming, making it easier to reason about and maintain asynchronous codebases.
8. What are some advantages of using parallel streams in Java 8?
Using parallel streams in Java 8 offers several advantages for processing collections of data in parallel, particularly when dealing with large datasets or computationally intensive operations. Some advantages include:
- Improved Performance: Parallel streams leverage multicore processors by automatically distributing the workload across multiple threads, leading to faster execution times compared to sequential streams. This can result in significant performance gains, especially for CPU-bound tasks.
- Simplified Parallelism: Parallel streams abstract away the complexity of multithreading and parallelism, making it easy for developers to parallelize data processing tasks without explicitly managing threads or synchronization. This promotes cleaner and more concise code, reducing the likelihood of errors and improving productivity.
- Scalability: Parallel streams dynamically adjust the degree of parallelism based on the available resources and the characteristics of the underlying hardware. This allows applications to scale efficiently with the number of available cores, maximizing resource utilization and throughput.
- Transparent Implementation: The API for parallel streams is built on top of the Stream API, providing a seamless transition from sequential to parallel processing. Developers can switch between sequential and parallel streams with minimal changes to their code, allowing for easy experimentation and optimization.
- Encourages Functional Programming: Parallel streams promote functional programming paradigms by providing a declarative and composable API for data processing. This encourages developers to write code in a more functional style, focusing on data transformations and operations rather than low-level implementation details.
Overall, using parallel streams in Java 8 offers a straightforward and efficient way to leverage parallelism for data processing tasks, resulting in improved performance, scalability, and productivity. However, it’s important to consider the characteristics of the workload and the underlying hardware to ensure optimal performance and resource utilization.
9. Explain the concept of method references in Java 8.
Method references in Java 8 provide a concise way to refer to methods or constructors without invoking them explicitly. They are particularly useful when passing methods as arguments to other methods or when using lambda expressions. Method references help reduce boilerplate code and make code more readable and expressive.
There are four types of method references:
- Reference to a Static Method: Syntax:
ContainingClass::staticMethodName
. Example:Math::max
. - Reference to an Instance Method of a Particular Object: Syntax:
objectReference::instanceMethodName
. Example:System.out::println
. - Reference to an Instance Method of an Arbitrary Object of a Particular Type: Syntax:
ContainingType::methodName
. Example:String::length
. - Reference to a Constructor: Syntax:
ClassName::new
. Example:ArrayList::new
.
Method references can be used in conjunction with functional interfaces, allowing them to be passed as arguments to methods or assigned to variables. For example:
//Java 8 Interview Questions for 10 Years Experience
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Using a lambda expression
names.forEach(s -> System.out.println(s));
// Using a method reference
names.forEach(System.out::println);
//Java 8 Interview Questions for 10 Years Experience
In this example, System.out::println
is a method reference that refers to the println
method of the PrintStream
class. It is equivalent to the lambda expression s -> System.out.println(s)
, but with a more concise syntax. Method references provide a shorthand way to refer to methods, improving code readability and maintainability.
10. How do you ensure thread safety when working with Java 8 features such as streams and CompletableFuture?
Ensuring thread safety when working with Java 8 features such as streams and CompletableFuture
involves following best practices and using appropriate synchronization mechanisms. Here are some strategies to ensure thread safety:
- Immutable Data Structures: Prefer immutable data structures when working with streams and
CompletableFuture
. Immutable objects are inherently thread-safe because they cannot be modified after creation, eliminating the need for synchronization. - Synchronization: When working with shared mutable state, use synchronization mechanisms such as
synchronized
blocks or locks to ensure that access to shared resources is properly synchronized across multiple threads. This prevents race conditions and data corruption. - Atomic Operations: Use atomic operations provided by classes like
AtomicInteger
,AtomicLong
, andAtomicReference
to perform thread-safe updates to shared variables without explicit locking. - Concurrent Data Structures: When working with streams, use concurrent data structures provided by the
java.util.concurrent
package, such asConcurrentHashMap
,ConcurrentLinkedQueue
, andConcurrentSkipListMap
, to ensure safe concurrent access to shared collections. - CompletableFuture Composition: When composing asynchronous tasks with
CompletableFuture
, use methods likethenApply
,thenCompose
, andthenCombine
to chain asynchronous computations safely. Ensure that any side effects or shared resources accessed within the chain are properly synchronized. - Error Handling: Handle exceptions gracefully in asynchronous computations by using methods like
exceptionally
andhandle
withCompletableFuture
. Make sure that error handling code is thread-safe and does not introduce concurrency issues.
By following these guidelines and using appropriate synchronization mechanisms, you can ensure thread safety when working with Java 8 features such as streams and CompletableFuture
, enabling safe and efficient concurrent programming in your applications.
Video Tutorials
Crack DSA Interview: Google, Facebook, and Amazon!👇
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
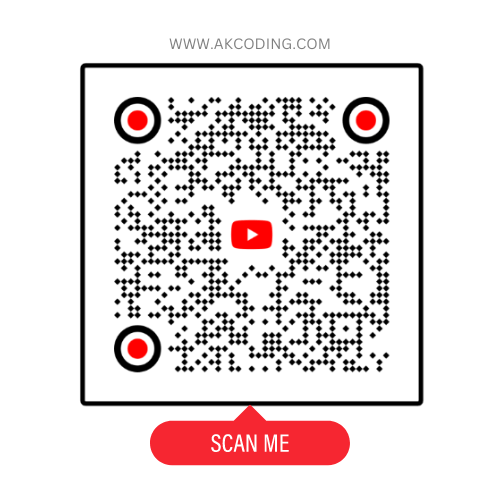
Java interview questions for freshers pdf, Top 100
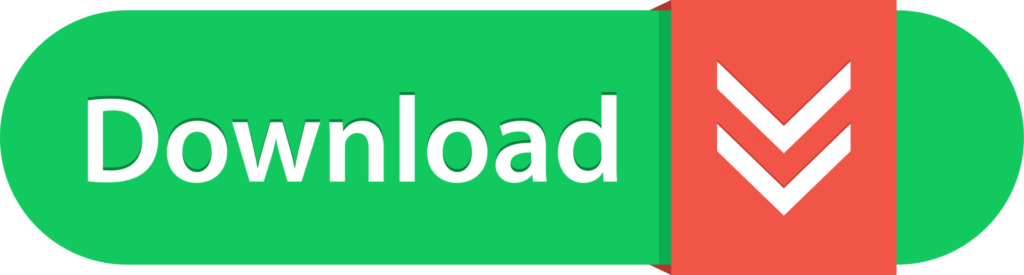
These questions cover various aspects of Java 8, including its features, advantages, usage, and concurrency considerations. Candidates with 10 years of Java experience should be prepared to discuss these topics in depth during interviews.