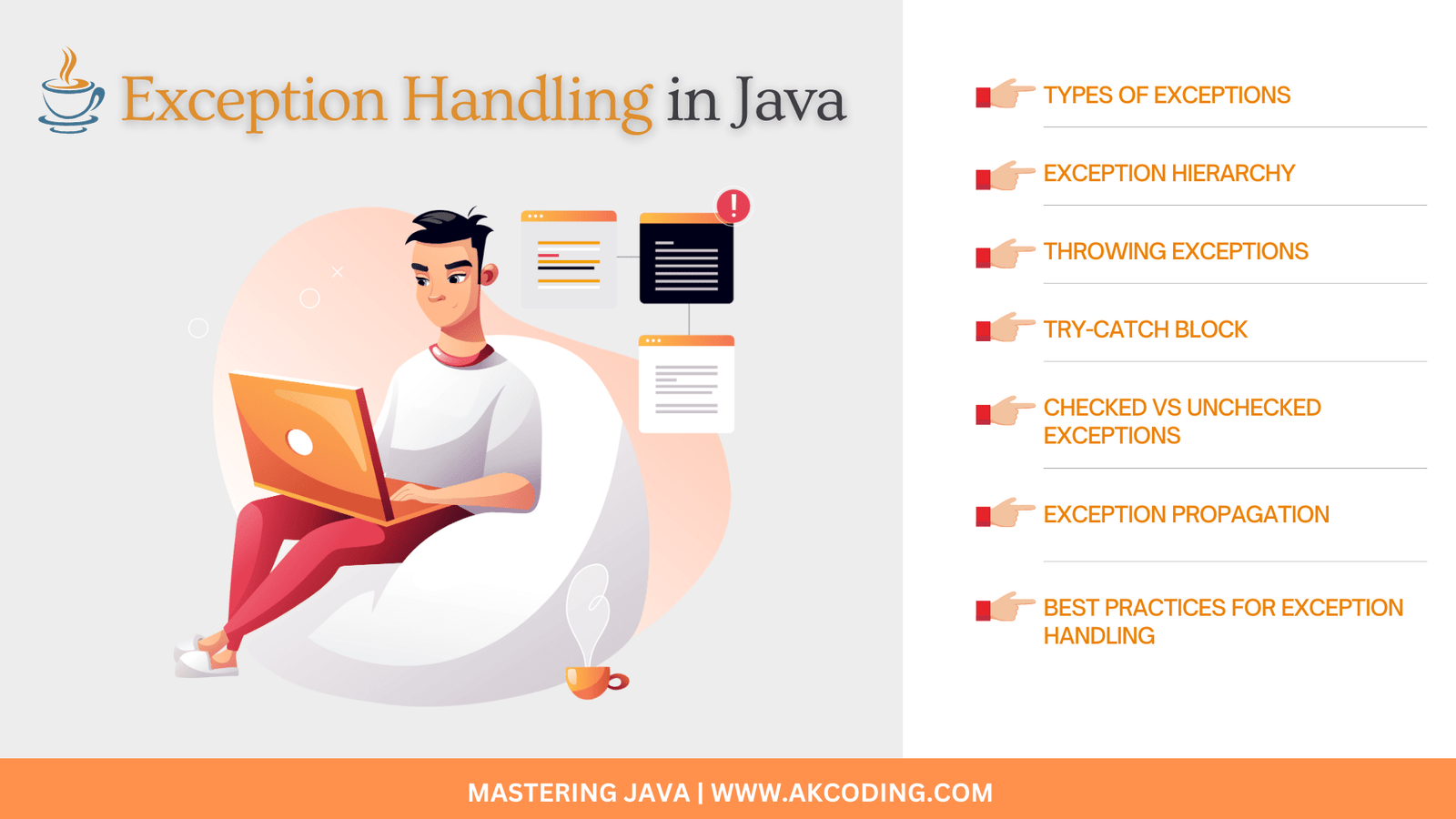
Table of Contents
Introduction to Exception Handling in Java
Exception Handling in Java is a mechanism provided by Java to deal with runtime errors and exceptional situations that may occur during program execution. These exceptions disrupt the normal flow of program execution and need to be handled appropriately to ensure program reliability and stability.
Definition of Exception:
An exception in Java is an event that occurs during the execution of a program, disrupting the normal flow of control. It represents an abnormal condition or error situation that can arise at runtime, such as divide by zero, array index out of bounds, file not found, etc.
Purpose of Exception Handling:
The primary purpose of exception handling in Java is to provide a structured and controlled way to deal with errors and exceptional situations that may occur during program execution. Exception handling allows developers to:
- Identify and gracefully handle errors to prevent program crashes.
- Separate error-handling code from the main program logic, making code more readable and maintainable.
- Provide meaningful error messages and context information to aid in debugging and troubleshooting.
- Recover from exceptional conditions and continue program execution if possible.
- Implement robust and reliable software applications that can handle unforeseen errors gracefully.
Advantages of Using Exception Handling:
- Robustness: Exception handling improves the robustness of Java programs by allowing developers to anticipate and handle unexpected errors, preventing program crashes and improving application reliability.
- Maintainability: Separating error-handling code from the main program logic improves code maintainability by making it easier to understand and modify. This promotes code reuse and facilitates future enhancements.
- Debugging: Exception handling provides valuable debugging information, such as stack traces and error messages, which help developers diagnose and fix problems more efficiently during development and testing.
- Graceful Error Recovery: Exception handling enables developers to recover from exceptional conditions and take appropriate corrective actions, allowing programs to continue execution in a controlled manner even in the presence of errors.
- Enhanced User Experience: Properly handled exceptions provide meaningful error messages and feedback to users, improving the overall user experience and reducing frustration when errors occur.
In summary, exception handling in Java is a powerful mechanism for managing errors and exceptional situations, improving program reliability, maintainability, and user experience. It is an essential aspect of modern software development and a fundamental skill for Java developers.
Types of Exceptions in Java
In Java, exceptions are broadly categorized into two main types: checked exceptions and unchecked exceptions. Additionally, there is a third category known as errors.
- Checked Exceptions:
- Checked exceptions are exceptions that are checked at compile-time by the compiler. This means that the compiler ensures that these exceptions are either caught and handled or declared using the
throws
keyword in the method signature. - Examples of checked exceptions include
IOException
,SQLException
, andClassNotFoundException
. - Checked exceptions are subclasses of
Exception
but not subclasses ofRuntimeException
.
- Unchecked Exceptions:
- Unchecked exceptions are exceptions that are not checked at compile-time. These exceptions typically occur at runtime and are subclasses of
RuntimeException
. - Unchecked exceptions do not need to be declared or caught explicitly, although it’s considered a best practice to handle them when appropriate.
- Examples of unchecked exceptions include
NullPointerException
,ArrayIndexOutOfBoundsException
, andArithmeticException
.
- Errors:
- Errors represent abnormal conditions that are beyond the control of the application and typically indicate serious problems that cannot be handled programmatically.
- Errors are subclasses of
Error
and are not meant to be caught or handled by application code. - Examples of errors include
OutOfMemoryError
,StackOverflowError
, andNoClassDefFoundError
.
In summary, exceptions in Java are categorized into checked exceptions, unchecked exceptions, and errors. Checked exceptions must be caught or declared, unchecked exceptions are not required to be caught or declared, and errors indicate serious problems that typically cannot be handled by application code. Understanding these types of exceptions is essential for effective exception handling in Java programs.
Exception Handling in Java Hierarchy
In Java, exceptions are organized in a hierarchical structure, with the root of the hierarchy being the Throwable
class. The Throwable
class has two direct subclasses: Exception
and Error
. This hierarchy allows for more specific exception types to be defined and handled based on the nature of the error. Here’s an overview of the Java exception hierarchy:
- Throwable: The root class of the Java exception hierarchy. It serves as the base class for all exceptions and errors.
- Subclasses:
- Exception: Represents exceptional conditions that a well-behaved Java program should catch and handle. Subclasses of
Exception
are checked exceptions, meaning they must be caught or declared. - Error: Represents serious errors that are beyond the control of the application. Errors are typically caused by system-level failures or resource exhaustion and are not meant to be caught or handled programmatically.
- Exception: Represents exceptional conditions that a well-behaved Java program should catch and handle. Subclasses of
- Subclasses:
- Exception: The base class for all checked exceptions in Java. It is further subclassed into various categories of exceptions based on the type of error or condition.
- Subclasses (not an exhaustive list):
- IOException: Represents errors related to input-output operations, such as file handling and network communication.
- SQLException: Represents errors related to database access and manipulation.
- RuntimeException: Represents unchecked exceptions that occur at runtime and are not required to be caught or declared. Subclasses of
RuntimeException
include common runtime exceptions likeNullPointerException
,ArrayIndexOutOfBoundsException
, andArithmeticException
.
- Subclasses (not an exhaustive list):
- Error: The base class for serious errors that typically indicate problems with the Java Virtual Machine (JVM) or the underlying system. Errors are not meant to be caught or handled programmatically by application code.
- Subclasses (not an exhaustive list):
- OutOfMemoryError: Indicates that the Java Virtual Machine (JVM) has run out of memory and cannot allocate more memory for new objects.
- StackOverflowError: Indicates that the call stack has exceeded its maximum limit, usually due to excessive recursion.
- NoClassDefFoundError: Indicates that the Java Virtual Machine (JVM) cannot find the definition of a class at runtime, typically due to missing dependencies or incorrect classpath settings.
- Subclasses (not an exhaustive list):
Understanding the Java exception hierarchy is essential for effective exception handling and error management in Java programs. It allows developers to catch and handle specific types of exceptions appropriately based on their nature and severity.
Exception Handling in Java Hierarchy Diagram
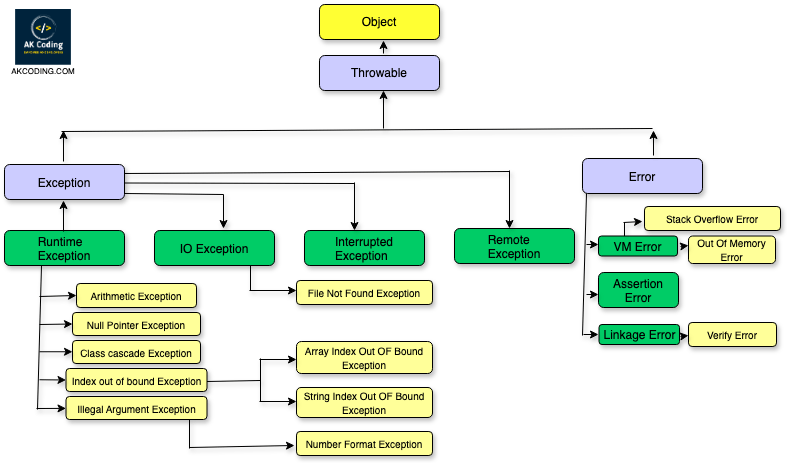
try-catch Block
The try-catch
block is a fundamental construct in Java used for exception handling. It allows you to enclose a block of code that might throw exceptions within a try
block, and then catch and handle those exceptions in one or more catch
blocks.
Here’s the basic syntax of a try-catch
block in Java:
//Exception Handling in Java
try {
// Code that may throw an exception
} catch (ExceptionType1 e1) {
// Exception handling code for ExceptionType1
} catch (ExceptionType2 e2) {
// Exception handling code for ExceptionType2
} finally {
// Optional finally block for cleanup code
}
//Exception Handling in Java
- The
try
block encloses the code that might throw exceptions. If an exception occurs within this block, control is transferred to the appropriatecatch
block. - Each
catch
block specifies the type of exception it can handle. If the type of exception thrown matches the type specified in acatch
block, the corresponding block is executed. - The
finally
block is optional and is used to specify cleanup code that should always be executed, regardless of whether an exception occurred or not. Thefinally
block executes after thetry
block or after thecatch
block (if an exception was caught).
Here’s an example of using a try-catch
block in Java:
//Exception Handling in Java
public class TryCatchExample {
public static void main(String[] args) {
try {
int result = divide(10, 0); // This will throw an ArithmeticException
System.out.println("Result: " + result); // This line will not be executed
} catch (ArithmeticException e) {
System.out.println("An arithmetic exception occurred: " + e.getMessage());
} finally {
System.out.println("Cleanup code executed"); // This will always be executed
}
}
public static int divide(int dividend, int divisor) {
return dividend / divisor; // This may throw an ArithmeticException
}
}
//Exception Handling in Java
In this example:
- The
divide
method divides two numbers, and it may throw anArithmeticException
if the divisor is zero. - The
divide
method is called within atry
block in themain
method. - If an
ArithmeticException
occurs during the execution of thetry
block, control is transferred to the correspondingcatch
block, where the exception is handled. - The
finally
block contains cleanup code that is executed regardless of whether an exception occurred or not.
The try-catch
block is an essential mechanism for handling exceptions in Java, allowing you to gracefully handle errors and ensure proper resource management in your applications.
Throwing Exceptions
Throwing exceptions in Java is a way to explicitly signal that an error condition has occurred during program execution. This can be done using the throw
keyword followed by an instance of a subclass of Throwable
, such as Exception
or Error
.
Here’s the basic syntax for throwing an exception:
//Exception Handling in Java
throw throwableInstance;
Where throwableInstance
is an instance of a subclass of Throwable
.
Here’s an example demonstrating how to throw an exception in Java:
//Exception Handling in Java
public class CustomExceptionExample {
public static void main(String[] args) {
try {
// Simulate an error condition
int result = divide(10, 0); // This will throw an ArithmeticException
System.out.println("Result: " + result); // This line will not be executed
} catch (ArithmeticException e) {
// Handle the exception
System.out.println("An arithmetic exception occurred: " + e.getMessage());
}
}
public static int divide(int dividend, int divisor) {
if (divisor == 0) {
// Throw an ArithmeticException if the divisor is zero
throw new ArithmeticException("Divisor cannot be zero");
}
return dividend / divisor;
}
}
//Exception Handling in Java
In this example:
- The
divide
method divides two numbers but first checks if the divisor is zero. - If the divisor is zero, it throws an
ArithmeticException
using thethrow
keyword along with a new instance ofArithmeticException
. - In the
main
method, thedivide
method is called within atry
block. - If an
ArithmeticException
is thrown within thedivide
method, it is caught and handled in the correspondingcatch
block.
Throwing exceptions allows you to signal error conditions and propagate them up the call stack, where they can be caught and handled appropriately. This helps in building robust and reliable Java applications by gracefully handling unexpected situations and providing feedback to users or developers about the nature of errors.
Checked vs Unchecked Exceptions
Here’s a comparison of checked and unchecked exceptions
Aspect | Checked Exceptions | Unchecked Exceptions |
---|---|---|
Syntax | Must be declared or caught | Not required to be declared or caught |
Subclass of | Subclass of Exception but not RuntimeException | Subclass of RuntimeException |
Examples | IOException , SQLException | NullPointerException , ArrayIndexOutOfBoundsException |
Checked by Compiler | Yes | No |
Mandatory Handling | Yes | No |
Compile-Time Exception | Yes | No |
Intent | Typically represents recoverable conditions that should be handled | Typically represents programming errors or conditions that cannot be recovered from |
Common Causes | I/O errors, database errors, network errors | Null pointer dereference, array access out of bounds, arithmetic errors |
Forces Handling | Yes | No |
In summary, checked exceptions must be declared or caught, are checked by the compiler, and typically represent recoverable conditions. Unchecked exceptions are not required to be declared or caught, are not checked by the compiler, and typically represent programming errors or unrecoverable conditions.
Exception Propagation
Exception propagation in Java refers to the mechanism by which an exception, once thrown within a method, can propagate upwards through the call stack until it is caught and handled or until it reaches the top-level caller (such as the JVM), resulting in program termination if not handled.
Here’s how exception propagation works in Java:
- Exception Thrown: When an exceptional condition occurs within a method, either due to an error condition or explicitly using the
throw
statement, an exception object representing the error is created and thrown. - Propagation Up the Call Stack: The exception propagates up the call stack to the caller of the method where the exception occurred. If the exception is not caught within the current method, it is passed to the caller method.
- Passing Through Methods: If the exception is not caught in the caller method, it continues to propagate up the call stack, passing through each method in the chain until it reaches a method that catches it or until it reaches the top-level caller (such as the JVM).
- Handling or Program Termination: If the exception is caught and handled by any method in the call stack, the program continues execution from the point where the exception was caught. If the exception is not caught and handled by any method in the call stack, it reaches the top-level caller (such as the JVM), resulting in program termination and the generation of an error message or stack trace.
Exception propagation allows for the separation of error handling code from the code that detects errors, enabling a more modular and flexible approach to error management in Java programs. By allowing exceptions to propagate up the call stack, Java provides a mechanism for centralized exception handling at higher levels of the application, where errors can be logged, reported, or handled in an appropriate manner.
Exception Handling Best Practices
Exception handling is a crucial aspect of Java programming, and following best practices ensures that your code is robust, maintainable, and easy to debug. Here are some best practices for exception handling in Java:
- Use Specific Exceptions: Catch specific exceptions rather than catching the general
Exception
class. This allows for more precise error handling and makes your code more readable and maintainable. - Handle Exceptions Gracefully: Handle exceptions in a way that gracefully recovers from errors or communicates the problem to the user effectively. Avoid suppressing exceptions without appropriate handling, as it may lead to unexpected behavior or silent failures.
- Resource Management with try-with-resources: When working with external resources such as files, database connections, or network sockets, use the try-with-resources statement to ensure proper resource management. This automatically closes the resources at the end of the block, even if an exception occurs.
- Avoid Empty catch Blocks: Avoid catching exceptions without performing any meaningful action. Empty catch blocks hide errors and make it difficult to diagnose problems. If you don’t know how to handle an exception, consider logging it or rethrowing it.
- Logging Exceptions: Use logging frameworks like Log4j or java.util.logging to log exceptions and error messages. Logging provides valuable information for debugging and troubleshooting issues in production environments.
- Provide Descriptive Error Messages: When throwing exceptions or logging errors, provide descriptive error messages that clearly explain the problem and help users or developers understand what went wrong. Include relevant context information to assist in diagnosing the issue.
- Use Checked Exceptions Judiciously: Use checked exceptions for recoverable errors that the caller can reasonably be expected to handle. Avoid excessive use of checked exceptions, as it can clutter the code with unnecessary try-catch blocks.
- Custom Exception Handling: Define custom exceptions for specific error conditions in your application domain. This allows you to differentiate between different types of errors and handle them appropriately.
- Avoid Swallowing Exceptions: Avoid swallowing exceptions by catching them and not taking any action. Always handle exceptions appropriately by logging them, notifying users, or taking corrective actions as necessary.
- Document Exception Handling: Document the exception-handling strategy in your code, including the types of exceptions that can be thrown, how they are handled, and any recovery mechanisms. This makes the code more understandable and helps other developers maintain it effectively.
By following these best practices, you can ensure that your Java code handles exceptions effectively, maintains resource integrity, logs errors for debugging, and provides a better user experience. Effective exception handling is crucial for building reliable and maintainable software applications.
Exception Handling in Practice
Exception handling in practice involves implementing strategies to manage and handle exceptions effectively in real-world Java applications. Here are some key aspects to consider when dealing with exception handling in practice:
- Identify Potential Exception Scenarios: Analyze your code to identify potential scenarios where exceptions may occur. This includes input validation, external dependencies (such as database access or network requests), and other error-prone operations.
- Use Try-Catch Blocks: Enclose code that may throw exceptions within try-catch blocks. Handle exceptions gracefully by providing appropriate error messages, logging the exceptions for debugging purposes, and taking corrective actions if possible.
- Handle Specific Exceptions: Catch specific exceptions rather than catching general
Exception
classes whenever possible. This allows for more precise error handling and enables different error-handling strategies based on the type of exception. - Rethrow Exceptions: If you catch an exception but cannot handle it effectively in the current context, consider rethrowing it to propagate it up the call stack. This allows higher-level code to handle the exception appropriately.
- Use Finally Blocks for Cleanup: Use
finally
blocks to ensure that resources are released and cleanup operations are performed, regardless of whether an exception occurs or not. This is especially important when dealing with external resources like files, database connections, or network sockets. - Logging and Monitoring: Implement logging mechanisms to record exceptions and error messages. Use logging frameworks like Log4j or java.util.logging to log exceptions with relevant context information, such as timestamps, stack traces, and error details. Monitor logged exceptions to identify recurring issues and potential areas for improvement.
- Provide User-Friendly Error Messages: When handling exceptions that are visible to users, provide informative and user-friendly error messages. This helps users understand the problem and provides guidance on how to resolve it or seek further assistance.
- Define Custom Exceptions: Define custom exception classes for specific error conditions in your application domain. This allows you to encapsulate error details and provide meaningful error handling based on the context of the exception.
- Test Exception Handling: Write unit tests and integration tests to verify that exception handling mechanisms work as expected under different scenarios. Test edge cases, boundary conditions, and error paths to ensure robustness and reliability in exception handling.
- Review and Refactor: Regularly review and refactor exception handling code to improve clarity, maintainability, and efficiency. Eliminate redundant or unnecessary try-catch blocks, consolidate error-handling logic, and optimize error recovery strategies where possible.
By following these practices, you can effectively manage and handle exceptions in your Java applications, ensuring robustness, reliability, and a positive user experience. Effective exception handling is essential for building high-quality software that meets the expectations of users and stakeholders.
Conclusion
Java exception handling is a vital aspect of writing robust and reliable software applications. It allows developers to anticipate and gracefully handle errors and exceptional situations that may occur during program execution. By using try-catch blocks, throwing exceptions, and employing best practices, developers can ensure that their code behaves predictably and provides a better user experience.
Importance of Proper Exception Handling Practices:
Proper exception handling practices are essential for building high-quality Java applications for several reasons:
- Robustness: Effective exception handling improves the robustness of Java applications by gracefully handling errors and preventing unexpected crashes or failures.
- Reliability: Properly handled exceptions ensure that applications behave predictably under different conditions, enhancing their reliability and stability.
- User Experience: Providing informative error messages and handling exceptions gracefully improves the user experience by helping users understand and resolve issues more effectively.
- Debugging and Troubleshooting: Logging exceptions and error messages aids in debugging and troubleshooting, allowing developers to identify and fix problems more efficiently.
- Maintainability: Following best practices for exception handling, such as using specific exceptions, logging errors, and providing cleanup code in finally blocks, improves code maintainability and readability.
- Compliance: Proper exception handling practices ensure compliance with coding standards and best practices, making code reviews and audits more straightforward and ensuring consistency across the codebase.
In conclusion, proper exception handling practices are essential for building robust, reliable, and maintainable Java applications. By following best practices and handling exceptions gracefully, developers can create software that meets the expectations of users and stakeholders while maintaining high standards of quality and reliability.
FAQ of Exception Handling in Java
How to do exception handling in java
Exception handling in Java is accomplished using try-catch blocks. The code that may throw exceptions is enclosed within a try block, and potential exceptions are caught and handled in catch blocks. If an exception occurs, control is transferred to the corresponding catch block that matches the type of exception thrown. Additionally, the finally block can be used to specify cleanup code that should be executed regardless of whether an exception occurs. Proper exception handling ensures robustness and reliability in Java programs by gracefully handling errors and exceptional situations during runtime.
Exception handling in java 7
In Java 7, exception handling remained fundamentally the same as in earlier versions, but there were some enhancements and improvements introduced. One notable feature introduced in Java 7 was the “multi-catch” block, which allowed catching multiple exceptions in a single catch block. This reduced code duplication and made exception handling more concise.
Here’s an example of multi-catch block in Java 7:
In this example, the catch block catches both IOException
and SQLException
in a single block.
Another improvement in Java 7 was the introduction of the try-with-resources statement, which simplified resource management by automatically closing resources at the end of the block. This feature was particularly useful when working with external resources like files, streams, or database connections.
Here’s an example of try-with-resources statement in Java 7:
In this example, the BufferedReader
is automatically closed at the end of the try block, regardless of whether an exception occurs or not.
Overall, Java 7 introduced enhancements to exception handling, such as multi-catch blocks and try-with-resources statements, making it easier and more efficient to manage exceptions and resources in Java programs.
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
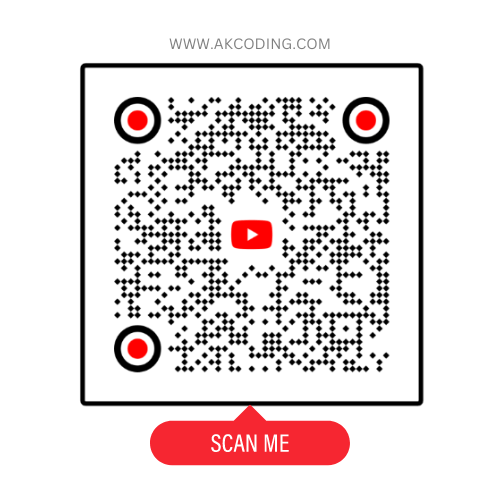