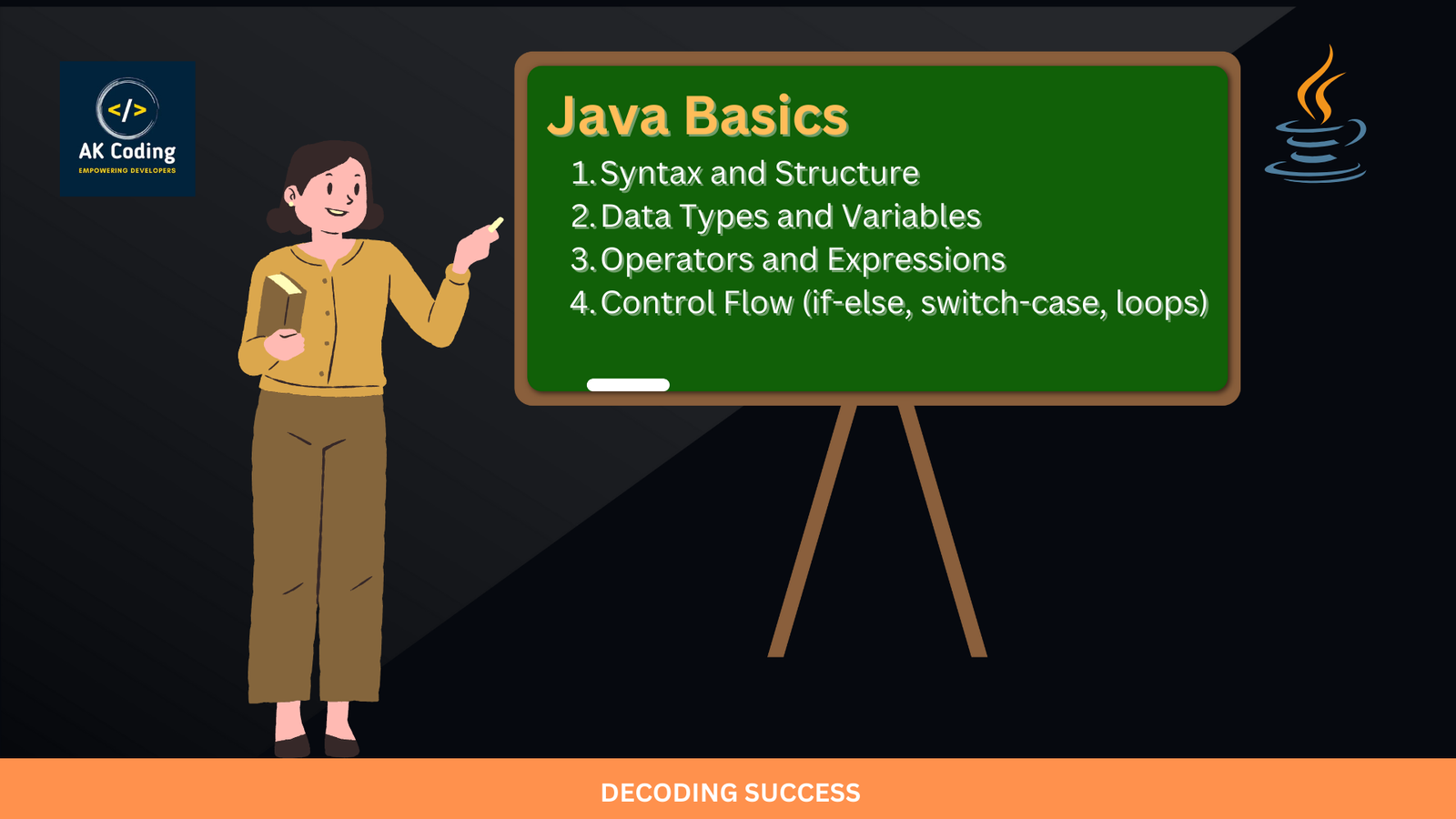
Table of Contents
Syntax and Structure : Java Basics
Java Basics
- Overview of Java:
- Java is a high-level, object-oriented programming language developed by Sun Microsystems (now owned by Oracle Corporation) in 1995.
- It is designed to be platform-independent, allowing Java programs to run on any device or operating system with a compatible Java Virtual Machine (JVM).
- Key Concepts:
- Object-Oriented Programming (OOP): Java follows the principles of OOP, including encapsulation, inheritance, and polymorphism.
- Platform Independence: Java programs are compiled into bytecode, which can run on any system with a JVM, regardless of the underlying hardware or operating system.
- Strong Typing: Java is a strongly typed language, meaning all variables must be declared with a specific data type.
- Garbage Collection: Java automatically manages memory allocation and deallocation through garbage collection, reducing the risk of memory leaks and memory-related bugs.
- Basic Syntax:
- Case Sensitivity: Java is case-sensitive, meaning variable names, method names, and keywords must be typed exactly as they are declared.
- Semicolons: Statements in Java end with a semicolon (;).
- Comments: Java supports single-line comments (
//
) and multi-line comments (/* */
) for documenting code. - Whitespace: Whitespace characters like spaces, tabs, and line breaks are ignored by the compiler but can improve code readability.
- Java Structure:
- Classes: Java programs are organized into classes, which serve as blueprints for creating objects. Each class typically represents a real-world entity or concept and contains data (fields) and behavior (methods).
- Methods: Methods are functions defined within classes to perform specific tasks. They can accept input parameters, execute code, and return values.
- Main Method: Every Java application must contain a
main()
method, which serves as the entry point for the program. It is where the program starts execution. - Packages: Packages are used to organize related classes and provide a namespace to prevent naming conflicts. They are declared at the beginning of Java source files using the
package
keyword. - Import Statements: Import statements are used to access classes and packages from other Java files or external libraries. They are declared at the beginning of Java source files using the
import
keyword.
- Example: Hello World Program:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
- In this example, we define a class named
HelloWorld
with amain()
method. Themain()
method prints “Hello, World!” to the console using theSystem.out.println()
method.
Understanding the basics of Java syntax and structure is essential for writing and understanding Java programs. As you progress, you’ll explore more advanced concepts and techniques to build robust and efficient Java applications.
Java Data Types and Variables
In Java, data types specify the type of data that can be stored in a variable. Variables are used to store values that can be manipulated and accessed in a program. Here are the main data types and variables in Java:
- Primitive Data Types:
- Java has eight primitive data types, which represent basic types of data. They are:
- byte: 8-bit integer value (-128 to 127)
- short: 16-bit integer value (-32,768 to 32,767)
- int: 32-bit integer value (-2^31 to 2^31-1)
- long: 64-bit integer value (-2^63 to 2^63-1)
- float: 32-bit floating point value
- double: 64-bit floating point value
- boolean: Represents true or false values
- char: 16-bit Unicode character (0 to 65,535)
- Variables:
- Variables are named storage locations in memory used to store data.
- Each variable has a data type that determines the type of data it can store.
- Variables must be declared before they can be used, specifying their data type and name.
- Variable Declaration:
- Syntax:
data_type variable_name;
- Example:
java int age; double salary; boolean isEmployed;
- Variable Initialization:
- Variables can be initialized with an initial value at the time of declaration or later in the program.
- Syntax:
data_type variable_name = initial_value;
- Example:
java int age = 30; double salary = 50000.50; boolean isEmployed = true;
- Variable Naming Rules:
- Variable names must start with a letter, underscore (_), or dollar sign ($) and can be followed by letters, digits, underscores, or dollar signs.
- Variable names are case-sensitive.
- Variable names cannot be Java keywords or reserved words.
- Constants:
- Constants are variables whose values cannot be changed once initialized.
- Use the
final
keyword to declare constants. - Example:
java final double PI = 3.14159; final int DAYS_IN_WEEK = 7;
Understanding data types and variables is fundamental in Java programming, as they are used extensively to store and manipulate data in programs. Proper usage of data types and variables ensures efficient memory utilization and maintains code readability and clarity.
Java Operators and Expressions
In Java, operators are symbols that perform operations on operands, such as variables, literals, or expressions. Expressions are combinations of operators and operands that produce a value. Here are the main types of operators and expressions in Java:
- Arithmetic Operators:
- Arithmetic operators perform mathematical operations on numeric operands.
- Addition (
+
), subtraction (-
), multiplication (*
), division (/
), and modulus (%
) are common arithmetic operators.
- Assignment Operators:
- Assignment operators are used to assign values to variables.
- The most basic assignment operator is
=
. For example:int x = 10;
- Comparison Operators:
- Comparison operators compare two values and return a boolean result (
true
orfalse
). - Common comparison operators include equality (
==
), inequality (!=
), greater than (>
), less than (<
), greater than or equal to (>=
), and less than or equal to (<=
).
- Logical Operators:
- Logical operators perform logical operations on boolean values.
- Common logical operators include logical AND (
&&
), logical OR (||
), and logical NOT (!
).
- Increment and Decrement Operators:
- Increment (
++
) and decrement (--
) operators are used to increase or decrease the value of a variable by 1. - They can be used as prefix or postfix operators, affecting the order of evaluation.
- Bitwise Operators:
- Bitwise operators perform operations on individual bits of integer operands.
- Common bitwise operators include bitwise AND (
&
), bitwise OR (|
), bitwise XOR (^
), left shift (<<
), and right shift (>>
).
- Ternary Operator:
- The ternary operator (
?:
) is a conditional operator that evaluates a boolean expression and returns one of two values based on the result. - Syntax:
condition ? value1 : value2
- Operator Precedence and Associativity:
- Operators in Java have precedence and associativity rules that determine the order of evaluation in expressions.
- Parentheses can be used to override the default precedence and explicitly specify the order of evaluation.
- Compound Expressions:
- Compound expressions combine multiple operators and operands to form complex expressions.
- Proper understanding of operator precedence and associativity is crucial for correctly evaluating compound expressions.
Understanding Java operators and expressions is essential for writing effective and efficient Java code. They are fundamental building blocks used extensively in programming to perform calculations, make decisions, and control program flow.
Java Control Flow
Control flow in Java refers to the order in which statements are executed in a program. It allows programmers to control the flow of execution based on certain conditions and make decisions about which code blocks to execute. Here are the main control flow structures in Java:
- Conditional Statements:
- Conditional statements allow the execution of different code blocks based on specified conditions.
- The
if
,else if
, andelse
statements are used for conditional branching. - Example:
java int x = 10; if (x > 0) { System.out.println("Positive number"); } else if (x < 0) { System.out.println("Negative number"); } else { System.out.println("Zero"); }
- Switch Statement:
- The switch statement allows for multi-way branching based on the value of an expression.
- It compares the value of the expression to various case values and executes the code block corresponding to the matching case.
- Example:
java int dayOfWeek = 3; switch (dayOfWeek) { case 1: System.out.println("Monday"); break; case 2: System.out.println("Tuesday"); break; // other cases... default: System.out.println("Invalid day"); }
- Loops:
- Loops are used to repeatedly execute a block of code until a certain condition is met.
- Java supports three types of loops:
for
,while
, anddo-while
. - Example of a
for
loop:
java for (int i = 0; i < 5; i++) { System.out.println(i); }
- Example of a
while
loop:
java int i = 0; while (i < 5) { System.out.println(i); i++; }
- Example of a
do-while
loop:
java int i = 0; do { System.out.println(i); i++; } while (i < 5);
- Break and Continue Statements:
- The
break
statement is used to exit a loop prematurely. - The
continue
statement is used to skip the current iteration of a loop and continue with the next iteration. - Example:
java for (int i = 0; i < 10; i++) { if (i == 5) { break; // exit loop when i equals 5 } if (i % 2 == 0) { continue; // skip even numbers } System.out.println(i); }
Control flow structures in Java provide the ability to make decisions, repeat tasks, and control the flow of execution in a program. By using conditional statements, loops, and branching constructs effectively, programmers can write code that performs complex operations and responds dynamically to changing conditions.
Conclusions
In conclusion, mastering the fundamentals of Java lays a strong foundation for building robust and scalable software applications. By understanding key concepts such as data types, variables, operators, control flow structures, and object-oriented principles, developers can write clean, efficient, and maintainable code. Here are some key takeaways from Java Basics:
- Platform Independence: Java’s platform independence allows programs to run on any device or operating system with a compatible Java Virtual Machine (JVM), making it a versatile choice for cross-platform development.
- Object-Oriented Programming (OOP): Java’s support for OOP principles such as encapsulation, inheritance, and polymorphism promotes code reusability, modularity, and flexibility in software design.
- Data Types and Variables: Java offers a wide range of data types for representing different kinds of data, along with variables to store and manipulate values dynamically.
- Operators and Expressions: Java provides various operators for performing arithmetic, logical, comparison, and bitwise operations, allowing developers to manipulate data and control program flow efficiently.
- Control Flow Structures: Control flow structures like conditional statements, loops, and branching constructs enable programmers to make decisions, repeat tasks, and control the flow of execution in their programs.
- Best Practices: Following best practices such as proper naming conventions, code readability, error handling, and documentation enhances code quality and maintainability.
- Continuous Learning: Java is a vast and evolving language, and continuous learning is essential to stay updated with new features, tools, and best practices in Java programming.
By applying the principles and techniques learned in Java Basics, developers can embark on a journey to explore more advanced topics, frameworks, and technologies in Java development. With its widespread adoption in various industries and strong community support, Java continues to be a leading programming language for building diverse and innovative software solutions.
FAQs
- What is Java and Why Should I Learn It?
- Java is a popular programming language known for its platform independence, robustness, and versatility. Learning Java opens up numerous career opportunities in software development, as it is widely used for building web applications, mobile apps, enterprise systems, and more.
- What are the Key Concepts of Java Basics?
- Java basics cover fundamental concepts such as data types, variables, operators, control flow structures (conditional statements, loops), and object-oriented programming principles (encapsulation, inheritance, polymorphism). Understanding these concepts is essential for writing Java code.
- How Do I Get Started with Java Programming?
- To get started with Java programming, you’ll need to install the Java Development Kit (JDK) on your computer. Then, you can use an Integrated Development Environment (IDE) like IntelliJ IDEA, Eclipse, or NetBeans to write and run Java code. There are also numerous online tutorials and resources available for beginners to learn Java programming.
- What Are the Main Data Types and Variables in Java?
- Java supports various data types, including primitive data types (int, double, boolean, etc.) and reference data types (objects, arrays, etc.). Variables are named storage locations used to store data, and each variable has a data type that determines the type of data it can hold.
- How Can I Practice and Improve My Java Skills?
- Practice is key to improving your Java skills. You can practice by writing small programs, solving coding challenges on platforms like LeetCode or HackerRank, contributing to open-source projects, and building your own projects. Additionally, reading books, taking online courses, and participating in coding communities can help you stay motivated and learn from others.
Java data types interview questions
Here are some common interview questions related to Java data types:
- What are the primitive data types in Java?
- Java has eight primitive data types: byte, short, int, long, float, double, char, and boolean.
- What is autoboxing and unboxing in Java?
- Autoboxing is the automatic conversion of primitive data types into their corresponding wrapper classes (e.g., int to Integer) and unboxing is the reverse process. This allows primitive data types to be used in collections and other contexts that require objects.
- What is the difference between primitive and reference data types in Java?
- Primitive data types store simple values directly in memory, while reference data types store references (memory addresses) to objects. Primitive data types are predefined by the language, whereas reference data types are defined by classes and can represent complex data structures.
- What is the size of each primitive data type in Java?
- The size of primitive data types varies depending on the platform and JVM implementation. However, in most cases, the sizes are as follows:
- byte: 1 byte
- short: 2 bytes
- int: 4 bytes
- long: 8 bytes
- float: 4 bytes
- double: 8 bytes
- char: 2 bytes
- boolean: JVM-dependent (typically 1 byte)
- What is the difference between int and Integer in Java?
- int is a primitive data type representing a 32-bit signed integer value, while Integer is a wrapper class for the int primitive type. Integer provides methods for converting between int values and objects, and allows int values to be used in contexts that require objects (e.g., collections).
- What is the difference between float and double in Java?
- float is a single-precision 32-bit floating-point data type, while double is a double-precision 64-bit floating-point data type. Double provides greater precision and a larger range of values compared to float, but requires more memory.
- What is the default value of each primitive data type in Java?
- The default value of each primitive data type is as follows:
- byte, short, int, long: 0
- float, double: 0.0
- char: ‘\u0000’ (null character)
- boolean: false
- What is the difference between char and String in Java?
- char is a primitive data type representing a single 16-bit Unicode character, while String is a reference data type representing a sequence of characters. Strings are immutable (cannot be changed once created), whereas char values can be modified.
- Can you explain the concept of signed and unsigned data types in Java?
- Unlike some other programming languages, Java does not have explicit support for unsigned data types. All integer types (byte, short, int, long) in Java are signed, meaning they can represent both positive and negative values. However, bitwise operations can be used to achieve similar effects to unsigned arithmetic.
- What are the limitations of using primitive data types in Java?
- Primitive data types have limitations such as fixed size, lack of methods, and inability to represent null values. Additionally, they cannot be used in contexts that require objects, such as collections and generics. To overcome these limitations, wrapper classes and reference data types can be used.
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
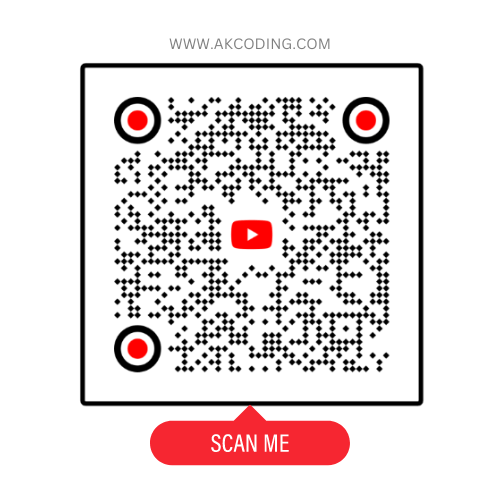