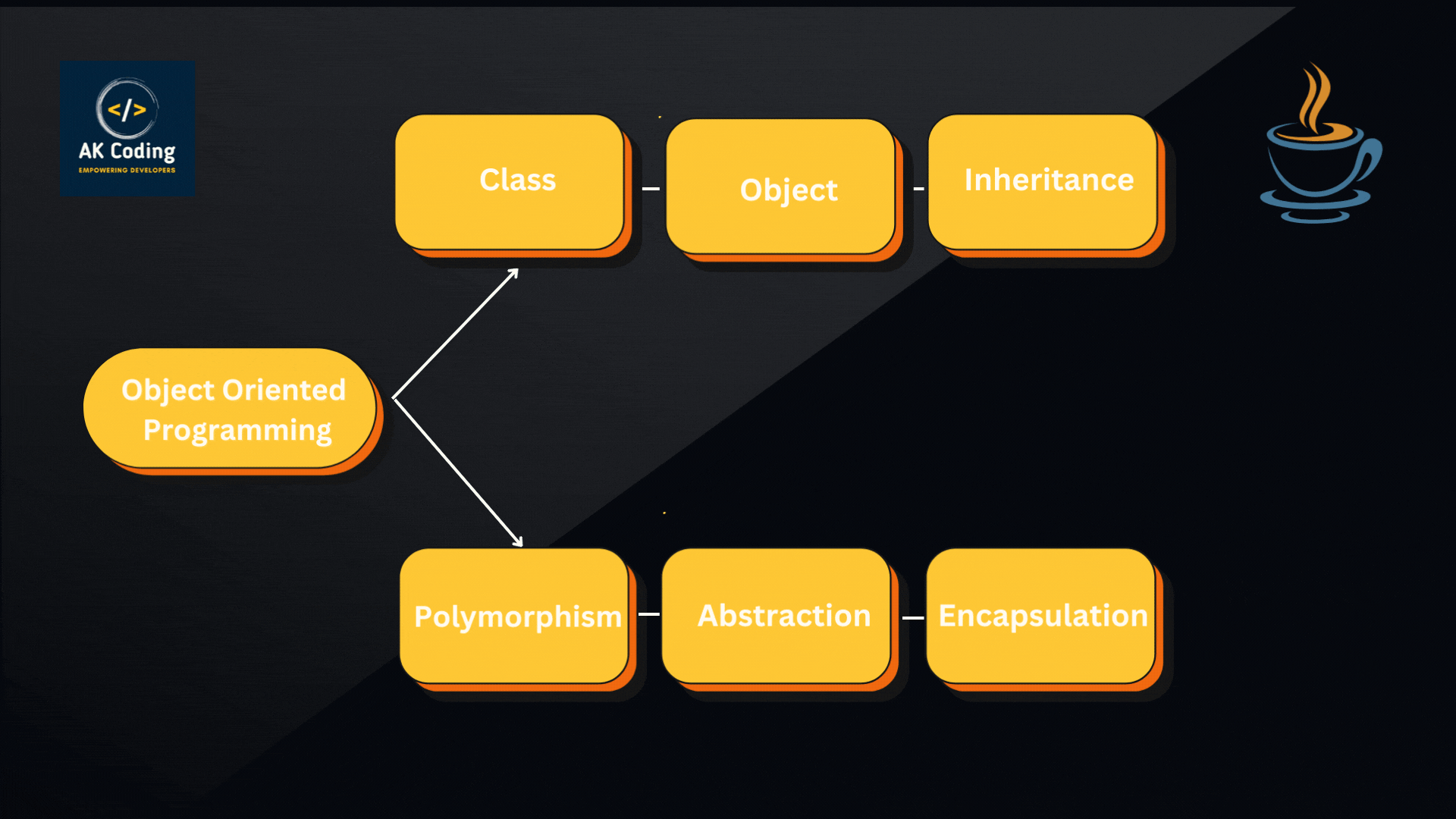
Table of Contents
Introduction to Object Oriented Programming (OOP)
Object Oriented Programming (OOP) is a programming paradigm that revolves around the concept of objects, which are instances of classes representing real-world entities. Java is one of the most popular programming languages that follows the principles of OOP extensively.
Definition and Concept of OOP
OOP is a programming paradigm based on the concept of objects, which can contain data in the form of fields (attributes or properties) and code in the form of procedures (methods or functions). Objects are instances of classes, which act as blueprints or templates for creating objects. OOP emphasizes modularity, reusability, and extensibility in software development by organizing code into objects and classes.
Key Principles of OOP
- Encapsulation: Encapsulation refers to bundling data and methods that operate on the data within a single unit, called a class. It hides the internal state of objects from external access and ensures that the object’s state is accessed and modified only through well-defined interfaces (methods).
- Inheritance: Inheritance is a mechanism by which a class can inherit properties and behaviors (methods) from another class, called the superclass or parent class. The class that inherits from the superclass is called the subclass or child class. Inheritance promotes code reuse and allows for the creation of hierarchical relationships among classes.
- Polymorphism: Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables the same method to behave differently based on the type of object it is invoked on. Polymorphism is achieved through method overriding (runtime polymorphism) and method overloading (compile-time polymorphism).
Benefits of OOP
- Modularity: OOP promotes modular design by breaking down complex systems into smaller, manageable units (objects and classes). Each class encapsulates a specific set of functionalities, making the codebase easier to understand, maintain, and reuse.
- Reusability: OOP facilitates code reuse through inheritance and composition. Classes and objects can be reused in different parts of the program or in other programs, leading to faster development, fewer errors, and increased productivity.
- Extensibility: OOP allows for easy extension and modification of existing code without affecting the rest of the program. New classes can be created by extending existing classes (inheritance) or by composing existing classes (composition), enabling flexibility and adaptability in software design.
- Encapsulation and Data Hiding: Encapsulation hides the internal state of objects from external access, providing better control over data access and modification. This enhances security, reduces the risk of unintended side effects, and promotes a clear separation of concerns in the codebase.
- Polymorphism and Flexibility: Polymorphism enables code to be more flexible and adaptable to different types of objects and scenarios. It allows for the implementation of generic algorithms and behaviors that can operate on objects of various types, promoting code reuse and simplifying complex systems.
Overall, OOP in Java offers a powerful and flexible approach to software development, allowing developers to build modular, reusable, and maintainable codebases that can evolve and adapt to changing requirements over time.
Classes and Objects in Java
In Java, a class is a blueprint or template for creating objects. It defines the structure and behavior of objects of a particular type. Objects are instances of classes that encapsulate data (attributes or properties) and behavior (methods or functions) related to that type.
Definition and Purpose of Classes and Objects
A class in Java serves as a blueprint that defines the properties and behaviors common to all objects of that class. It encapsulates data and functionality into a single unit, promoting modularity, reusability, and extensibility in software development. Classes abstract the details of implementation, allowing for a clear separation of concerns and promoting a more organized and maintainable codebase.
Objects, on the other hand, are instances of classes created at runtime. They represent specific entities or instances of the class, with their own unique state (data) and behavior (methods). Objects allow us to model real-world entities or concepts in our Java programs, enabling us to interact with and manipulate data in a structured and object oriented manner.
The purpose of classes and objects in Java is to facilitate object oriented programming (OOP), a powerful paradigm for organizing and managing complex software systems. By defining classes and creating objects, developers can model real-world entities, implement reusable components, and build modular, maintainable, and scalable software solutions. Classes and objects enable abstraction, encapsulation, inheritance, and polymorphism, the core principles of OOP, which promote code reusability, flexibility, and ease of maintenance.
Class Declaration in Java
In Java, a class declaration serves as a blueprint for creating objects. It defines the structure and behavior of objects of that particular type. The syntax for declaring a class in Java is as follows:
[access modifier] class ClassName {
// Fields (attributes or properties)
[access modifier] [data type] fieldName1;
[access modifier] [data type] fieldName2;
// ...
// Constructors
[access modifier] ClassName(parameters) {
// Constructor body
}
// Methods (functions)
[access modifier] [return type] methodName1(parameters) {
// Method body
}
[access modifier] [return type] methodName2(parameters) {
// Method body
}
// ...
}
Here’s a breakdown of the components of a class declaration in Java:
- Access Modifier: It specifies the visibility of the class to other classes. It can be one of the following:
public
,protected
,default
(no modifier), orprivate
. - Class Name: It is the name of the class, following the naming conventions in Java.
- Fields: These are variables declared within the class to represent its state or data. They define the attributes or properties of objects created from the class.
- Constructors: Constructors are special methods used for initializing objects. They have the same name as the class and do not have a return type. They are invoked when an object of the class is created using the
new
keyword. - Methods: These are functions defined within the class to perform operations or provide functionality. They define the behavior of objects created from the class.
Example:
public class Person {
// Fields
private String name;
private int age;
// Constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Methods
public void displayInfo() {
System.out.println("Name: " + name);
System.out.println("Age: " + age);
}
}
In this example, the Person
class has two fields (name
and age
), a constructor that initializes these fields, and a method displayInfo()
to display information about a person.
Object Instantiation in Java
In Java, object instantiation refers to the process of creating an instance of a class, or in simpler terms, creating an object. Object instantiation involves allocating memory for the object and initializing its fields using the constructor defined in the class. The syntax for instantiating an object in Java is as follows:
ClassName objectName = new ClassName(arguments);
Here’s a breakdown of the components of object instantiation in Java:
- ClassName: It is the name of the class for which you want to create an object.
- objectName: It is the name given to the object being created. This name is used to reference the object in the program.
- new: It is the keyword used to allocate memory for the object and create the instance.
- arguments: These are the arguments passed to the constructor of the class, if any, to initialize the object’s state.
Example:
public class Main {
public static void main(String[] args) {
// Instantiating an object of the Person class
Person person1 = new Person("John", 30);
// Using the object to call methods or access fields
person1.displayInfo();
}
}
In this example, an object of the Person
class is instantiated using the new
keyword and assigned to the variable person1
. The constructor of the Person
class is called with arguments "John"
and 30
to initialize the name
and age
fields of the object. Finally, the displayInfo()
method of the Person
class is called using the person1
object to display information about the person.
Class Members: Fields and Methods
In Java, classes can contain two types of members: fields (attributes or properties) and methods (functions). These members define the state and behavior of objects created from the class. Here’s an explanation of fields and methods in Java:
Fields:
Fields are variables declared within a class to represent its state or data. They define the attributes or properties of objects created from the class. Fields can have different access modifiers (public, private, protected, or default) to control their visibility and accessibility from other classes.
Example:
public class Person {
// Fields
private String name;
private int age;
}
In this example, the Person
class has two fields: name
and age
, which represent the name and age of a person, respectively.
Methods:
Methods are functions defined within a class to perform operations or provide functionality. They define the behavior of objects created from the class. Methods can have different access modifiers and return types. They can also accept parameters to perform specific tasks.
Example:
public class Person {
// Fields
private String name;
private int age;
// Constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Method to display information about the person
public void displayInfo() {
System.out.println("Name: " + name);
System.out.println("Age: " + age);
}
}
In this example, the Person
class has a constructor to initialize the name
and age
fields, and a method displayInfo()
to display information about a person.
Fields and methods collectively define the structure and behavior of a class in Java. They encapsulate data and functionality within a single unit, promoting modularity, reusability, and extensibility in software development.
Constructors and Initialization in Java
In Java, constructors are special methods used for initializing objects. They have the same name as the class and do not have a return type, not even void
. Constructors are invoked when an object of the class is created using the new
keyword. They are responsible for initializing the state of the object, typically by setting the initial values of its fields.
Here’s an overview of constructors and initialization in Java:
Constructors:
- Constructors are used to initialize the state of objects when they are created.
- They have the same name as the class and do not have a return type.
- If a class does not explicitly define any constructors, Java provides a default constructor with no parameters.
- You can define multiple constructors in a class with different parameter lists (overloading).
- Constructors can call other constructors using
this()
orsuper()
.
Example:
public class Person {
private String name;
private int age;
// Constructor with parameters
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Default constructor
public Person() {
// Default values
this.name = "Unknown";
this.age = 0;
}
}
Initialization:
- Initialization refers to the process of setting initial values to fields or variables.
- Fields of a class can be initialized using constructors, initializer blocks, or directly at the point of declaration.
- Initializer blocks are used to initialize fields with complex expressions or calculations.
Example:
public class Person {
private String name;
private int age;
// Constructor with parameters
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Initializer block
{
// Initialization with default values
this.name = "Unknown";
this.age = 0;
}
}
In summary, constructors and initialization play a crucial role in object creation and initialization in Java. They ensure that objects are properly initialized with the required state, enabling them to perform their intended tasks effectively.
Access Modifiers and Encapsulation
In Java, access modifiers are keywords used to control the visibility and accessibility of classes, fields, methods, and constructors. They specify the level of access that other classes or code within the same package can have to the members of a class. There are four types of access modifiers in Java:
- public: Members declared as public are accessible from any other class or package. They have the widest scope of visibility.
- protected: Members declared as protected are accessible within the same package and by subclasses (whether they are in the same package or different package).
- default (no modifier): If no access modifier is specified, the member has default access. Members with default access are accessible only within the same package.
- private: Members declared as private are accessible only within the same class. They have the narrowest scope of visibility.
Encapsulation is the mechanism of wrapping the data (fields) and methods (functions) into a single unit called a class. It is achieved by declaring the fields as private and providing public methods (getters and setters) to access and modify the data. Encapsulation helps in achieving data hiding, abstraction, and protection of data from unauthorized access or modification.
Here’s an example demonstrating access modifiers and encapsulation in Java:
public class Person {
private String name; // private field
private int age; // private field
// Constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Getter method for name
public String getName() {
return name;
}
// Setter method for name
public void setName(String name) {
this.name = name;
}
// Getter method for age
public int getAge() {
return age;
}
// Setter method for age
public void setAge(int age) {
this.age = age;
}
}
In this example, the name
and age
fields are declared as private to encapsulate the data. Public getter and setter methods are provided to access and modify these fields. This ensures that the data is accessed and modified through controlled methods, maintaining encapsulation and enforcing data integrity. Access modifiers (public
and private
) control the visibility of the fields and methods, allowing only authorized access from other classes or packages.
Inheritance and Polymorphism in Java
In Java, inheritance and polymorphism are key concepts of object oriented programming (OOP) that allow for code reuse, flexibility, and abstraction. Here’s an overview of each concept:
Inheritance:
- Inheritance is a mechanism by which a class (subclass or child class) can inherit properties and behaviors (fields and methods) from another class (superclass or parent class).
- The subclass extends the superclass, thereby inheriting its members.
- Inheritance promotes code reuse and enables the creation of hierarchical relationships among classes.
- Subclasses can override inherited methods to provide specialized implementations or extend functionality.
- Java supports single inheritance (a class can inherit from only one superclass) and multiple inheritance through interfaces (a class can implement multiple interfaces).
Example:
// Superclass
public class Animal {
public void sound() {
System.out.println("Animal makes a sound");
}
}
// Subclass inheriting from Animal
public class Dog extends Animal {
// Method overriding
@Override
public void sound() {
System.out.println("Dog barks");
}
}
Polymorphism:
- Polymorphism allows objects of different classes to be treated as objects of a common superclass.
- It enables the same method to behave differently based on the type of object it is invoked on.
- Polymorphism can be achieved through method overriding (runtime polymorphism) and method overloading (compile-time polymorphism).
- Runtime polymorphism occurs when a method is overridden in a subclass, and the method to be executed is determined at runtime based on the type of object.
- Compile-time polymorphism occurs when multiple methods with the same name but different parameter lists are defined in a class, and the appropriate method is selected at compile time based on the method signature.
Example:
// Superclass
public class Animal {
public void sound() {
System.out.println("Animal makes a sound");
}
}
// Subclass overriding the sound() method
public class Dog extends Animal {
@Override
public void sound() {
System.out.println("Dog barks");
}
}
// Polymorphic behavior
public class Main {
public static void main(String[] args) {
Animal animal1 = new Animal();
Animal animal2 = new Dog(); // Polymorphic reference
animal1.sound(); // Output: "Animal makes a sound"
animal2.sound(); // Output: "Dog barks"
}
}
In this example, animal2
is a polymorphic reference to the Animal
class that actually refers to an object of type Dog
. The sound()
method behaves differently based on the type of object it is invoked on, demonstrating runtime polymorphism.
Extending Classes and Superclasses
In Java, extending classes and superclasses are fundamental concepts of inheritance, which is a key aspect of object oriented programming (OOP). Here’s an overview:
Extending Classes:
- Extending a class allows you to create a new class (subclass or child class) that inherits properties and behaviors (fields and methods) from an existing class (superclass or parent class).
- The
extends
keyword is used to indicate that a class is a subclass of another class. - Subclasses can add new fields and methods, override inherited methods, and inherit constructors from the superclass.
- Subclasses can also access non-private members of the superclass using the
super
keyword.
Example:
// Superclass
public class Animal {
public void sound() {
System.out.println("Animal makes a sound");
}
}
// Subclass extending Animal
public class Dog extends Animal {
// Additional method in subclass
public void wagTail() {
System.out.println("Dog wags its tail");
}
// Method overriding
@Override
public void sound() {
System.out.println("Dog barks");
}
}
Superclasses:
- A superclass is a class that is extended by one or more subclasses.
- It provides common behavior and characteristics that are shared among its subclasses.
- Superclasses can define fields and methods that are inherited by their subclasses.
- They can also have constructors that are invoked when objects of the subclass are created.
Example:
// Superclass
public class Animal {
private String name;
// Constructor
public Animal(String name) {
this.name = name;
}
// Getter method
public String getName() {
return name;
}
}
Using super
Keyword:
- The
super
keyword is used to refer to the superclass members (fields, methods, constructors) within the subclass. - It can be used to invoke the superclass constructor, access superclass fields or methods, and call superclass methods that are overridden in the subclass.
Example:
// Subclass using super keyword
public class Dog extends Animal {
private String breed;
// Constructor
public Dog(String name, String breed) {
super(name); // Invoke superclass constructor
this.breed = breed;
}
// Method overriding
@Override
public String getName() {
return "Dog name: " + super.getName(); // Access superclass method
}
}
Inheritance and superclass-subclass relationships allow for code reuse, abstraction, and modularity in Java programming. They enable the creation of hierarchies of classes with shared characteristics and behaviors, promoting cleaner and more maintainable code.
Method Overriding and Overloading in Java
Method overriding and method overloading are two important concepts in Java that allow you to define multiple methods with the same name but different behaviors. Here’s an overview of each concept:
Method Overriding:
- Method overriding is a feature that allows a subclass to provide a specific implementation of a method that is already defined in its superclass.
- The method signature (name, return type, and parameters) must be the same in both the superclass and subclass for method overriding to occur.
- The
@Override
annotation is used to indicate that a method is intended to override a method from the superclass. While not strictly required, it helps to prevent accidental method signature changes that could prevent the overriding from occurring. - Method overriding enables polymorphism, where the appropriate method implementation is determined at runtime based on the type of object.
Example:
// Superclass
public class Animal {
public void sound() {
System.out.println("Animal makes a sound");
}
}
// Subclass overriding the sound() method
public class Dog extends Animal {
@Override
public void sound() {
System.out.println("Dog barks");
}
}
Method Overloading:
- Method overloading is a feature that allows a class to have multiple methods with the same name but different parameter lists.
- The methods must have different parameter types, number of parameters, or both.
- Method overloading enables you to create methods that perform similar tasks but with different input arguments.
- The compiler resolves the method invocation based on the number and types of arguments provided.
Example:
public class Calculator {
// Method overloading
public int add(int a, int b) {
return a + b;
}
// Overloaded method with different parameter types
public double add(double a, double b) {
return a + b;
}
}
In summary, method overriding allows a subclass to provide a specific implementation of a method defined in its superclass, while method overloading allows a class to define multiple methods with the same name but different parameter lists. Both concepts are essential for achieving polymorphism and code flexibility in Java programming.
Polymorphism and Dynamic Binding
Polymorphism and dynamic binding are two closely related concepts in object oriented programming (OOP) that enable flexibility, code reusability, and runtime behavior determination. Here’s an overview of each concept:
Polymorphism:
- Polymorphism is the ability of an object to take on different forms or behaviors based on the context in which it is used.
- In Java, polymorphism is achieved through method overriding, where a subclass can provide a specific implementation of a method that is already defined in its superclass.
- Polymorphism allows for a single interface (method name) to represent different behaviors across different subclasses.
- It enables flexibility and extensibility in the code, allowing for easier maintenance and scalability.
Example:
// Superclass
public class Animal {
public void sound() {
System.out.println("Animal makes a sound");
}
}
// Subclass overriding the sound() method
public class Dog extends Animal {
@Override
public void sound() {
System.out.println("Dog barks");
}
}
Dynamic Binding:
- Dynamic binding, also known as late binding or runtime polymorphism, is the process of associating a method call with the actual implementation of the method at runtime.
- In Java, method calls are resolved dynamically based on the type of the object at runtime. This allows for the selection of the appropriate method implementation based on the actual type of the object, rather than the reference type.
- Dynamic binding enables polymorphic behavior, where the method to be executed is determined dynamically based on the actual type of the object.
Example:
Animal animal = new Dog(); // Polymorphic reference
animal.sound(); // Dynamic binding: calls Dog's sound() method
In this example, the sound()
method call is resolved dynamically at runtime based on the actual type of the object (Dog
), rather than the reference type (Animal
). This demonstrates dynamic binding and polymorphic behavior in action.
In summary, polymorphism and dynamic binding are essential concepts in OOP that allow for flexible, extensible, and maintainable code by enabling different behaviors to be associated with objects based on their runtime types.
Abstract Classes and Interfaces in Java
Abstract classes and interfaces are two important concepts in Java that enable abstraction, code reusability, and flexibility. Here’s an overview of each:
Abstract Classes:
- An abstract class is a class that cannot be instantiated directly and is meant to be subclassed.
- It may contain abstract methods (methods without a body) as well as concrete methods (methods with a body).
- Abstract methods are meant to be implemented by subclasses, while concrete methods may provide default behavior.
- Abstract classes can have constructors, fields, and methods like any other class.
- Subclasses of an abstract class must either implement all abstract methods or be declared abstract themselves.
Example:
public abstract class Shape {
protected int x;
protected int y;
public Shape(int x, int y) {
this.x = x;
this.y = y;
}
// Abstract method
public abstract void draw();
// Concrete method
public void moveTo(int newX, int newY) {
this.x = newX;
this.y = newY;
}
}
Interfaces:
- An interface is a reference type in Java that can contain only abstract methods, default methods, static methods, and constant fields.
- It provides a contract for classes to implement, defining a set of methods that must be implemented by implementing classes.
- Interfaces support multiple inheritance, allowing a class to implement multiple interfaces.
- Classes implement interfaces using the
implements
keyword, and must provide implementations for all methods defined in the interface. - Interfaces are used to achieve abstraction, decoupling, and code reusability.
Example:
public interface Drawable {
void draw(); // Abstract method
default void resize(int width, int height) {
// Default method implementation
System.out.println("Resizing...");
}
}
Differences:
- Abstract classes can have constructors, fields, and methods (both abstract and concrete), while interfaces cannot have constructors or fields (except for constant fields).
- A class can extend only one abstract class, but it can implement multiple interfaces.
- Abstract classes can have access modifiers (public, protected, private), while interface methods are implicitly public and abstract (except for default and static methods, which can have access modifiers).
- Abstract classes are used to provide a common base implementation for subclasses, while interfaces are used to define a contract for classes to implement.
In summary, abstract classes and interfaces are both used to achieve abstraction and define contracts for classes, but they differ in their implementation details and usage scenarios. Abstract classes are used when a common base implementation is needed, while interfaces are used to define behavior without providing any implementation.
Composition vs. Inheritance in Java
Composition and inheritance are two fundamental concepts in object oriented programming (OOP) that facilitate code reuse and promote modularity. Here’s an overview of each:
Composition:
- Composition is a design principle where a class contains references to other classes as members, known as composition relationships.
- It enables building complex objects by combining simpler objects, forming a “has-a” relationship.
- In composition, one class encapsulates another class, providing access to its functionality through its methods.
- Composition promotes code reuse, flexibility, and modularity by allowing for the creation of objects with distinct behaviors and responsibilities.
Example:
public class Engine {
public void start() {
System.out.println("Engine started");
}
}
public class Car {
private Engine engine;
public Car() {
this.engine = new Engine(); // Composition relationship
}
public void startCar() {
engine.start(); // Delegate functionality to Engine object
}
}
Inheritance:
- Inheritance is a mechanism where a class (subclass or child class) inherits properties and behaviors (fields and methods) from another class (superclass or parent class).
- It forms an “is-a” relationship, where a subclass is a specialization of its superclass.
- Inheritance promotes code reuse by allowing subclasses to reuse the functionality of the superclass and extend or modify it as needed.
- It enables polymorphism, where objects of different classes can be treated interchangeably if they share a common superclass.
Example:
public class Animal {
public void sound() {
System.out.println("Animal makes a sound");
}
}
public class Dog extends Animal {
@Override
public void sound() {
System.out.println("Dog barks");
}
}
Comparison:
- Composition favors object composition over class inheritance, promoting flexibility and reducing coupling between classes.
- Inheritance creates a tight coupling between classes, as subclasses are dependent on the implementation details of their superclasses.
- Composition allows for more flexible and modular designs, as objects can be easily replaced or extended without impacting other parts of the system.
- Inheritance is suitable for creating class hierarchies where subclasses share common behavior and functionality with their superclasses.
In summary, composition and inheritance are both important techniques for achieving code reuse and promoting modularity in OOP. While inheritance establishes an “is-a” relationship between classes, composition establishes a “has-a” relationship, enabling more flexible and modular designs. The choice between composition and inheritance depends on the specific requirements and design goals of the application.
Conclusion
Object oriented programming (OOP) in Java offers a powerful and flexible approach to software development, enabling developers to create modular, reusable, and maintainable code. Here are some key conclusions about OOP in Java:
- Encapsulation: Encapsulation allows the bundling of data (fields) and methods (functions) into a single unit (class), promoting data hiding and abstraction. This helps in reducing complexity and improving code readability and maintainability.
- Inheritance: Inheritance enables the creation of hierarchies of classes, where subclasses inherit properties and behaviors from their superclasses. It promotes code reuse, extensibility, and polymorphism, allowing for the definition of common interfaces and shared functionality.
- Polymorphism: Polymorphism allows objects of different classes to be treated interchangeably through a common interface, enabling flexibility and dynamic behavior. Method overriding and method overloading are two key mechanisms that enable polymorphic behavior in Java.
- Abstraction: Abstraction allows developers to focus on essential details while hiding unnecessary complexity. It enables the creation of abstract data types, interfaces, and classes, providing a clear and concise representation of concepts and entities in the problem domain.
- Modularity: OOP promotes modularity by organizing code into reusable components (classes and objects) that can be independently developed, tested, and maintained. This facilitates code organization, collaboration, and scalability in large software projects.
- Composition vs. Inheritance: While both composition and inheritance are techniques for achieving code reuse, composition favors object composition over class inheritance, promoting flexibility and reducing coupling between classes. Choosing between composition and inheritance depends on the specific requirements and design goals of the application.
- Design Patterns: Design patterns provide proven solutions to common design problems in software development. They leverage the principles of OOP to address recurring challenges, such as object creation, structural organization, and behavior encapsulation. Understanding and applying design patterns can enhance code quality, maintainability, and extensibility.
In conclusion, OOP in Java offers a rich set of features and principles that enable developers to build robust, scalable, and maintainable software solutions. By embracing encapsulation, inheritance, polymorphism, abstraction, modularity, and design patterns, Java developers can create elegant and efficient code that meets the evolving needs of modern software development.
FAQs
What is Object Oriented Programming (OOP)?
OOP is a programming paradigm that organizes software design around objects and data, rather than actions and logic. It emphasizes concepts like encapsulation, inheritance, polymorphism, and abstraction.
What are the four main principles of OOP?
The four main principles of OOP are:
Encapsulation: Bundling data and methods that operate on the data into a single unit (class).
Inheritance: Creating new classes by extending existing classes, inheriting their properties and behaviors.
Polymorphism: The ability of objects to take on different forms or behaviors based on the context in which they are used.
Abstraction: Hiding the implementation details of an object and exposing only the essential features.
What is a class in Java?
A class in Java is a blueprint or template for creating objects. It defines the structure and behavior of objects of a particular type.
What is an object in Java?
An object in Java is an instance of a class. It represents a specific entity or instance of the class, encapsulating its state (data) and behavior (methods).
What is encapsulation and why is it important?
Encapsulation is the bundling of data (fields) and methods (functions) that operate on the data into a single unit (class). It helps in hiding the internal state of an object and protecting it from external interference. Encapsulation promotes code modularity, reusability, and maintainability.
What is inheritance and how does it work in Java?
Inheritance is a mechanism in which a class (subclass or child class) inherits properties and behaviors (fields and methods) from another class (superclass or parent class). Subclasses extend the functionality of their superclasses, promoting code reuse and enabling hierarchical relationships between classes.
What is polymorphism and how is it achieved in Java?
Polymorphism is the ability of objects to take on different forms or behaviors based on the context in which they are used. In Java, polymorphism is achieved through method overriding (runtime polymorphism) and method overloading (compile-time polymorphism).
What is abstraction and why is it important in OOP?
Abstraction is the process of hiding the implementation details of an object and exposing only the essential features. It allows for simplification and generalization of complex systems, enabling easier understanding, maintenance, and extension of software code.
What is the difference between an abstract class and an interface?
An abstract class is a class that cannot be instantiated directly and may contain abstract methods (methods without a body) as well as concrete methods. Interfaces, on the other hand, define a contract for classes to implement and can only contain abstract methods, default methods, static methods, and constant fields. A class can extend only one abstract class, but it can implement multiple interfaces.
How do you achieve multiple inheritance in Java?
Multiple inheritance is not directly supported in Java, as it can lead to the diamond problem and other complexities. However, you can achieve a form of multiple inheritance through interfaces, where a class can implement multiple interfaces, inheriting their abstract methods.
Difference between procedural and object oriented programming with example
Procedural programming and object oriented programming (OOP) are two different paradigms used in software development. Here’s a comparison between the two with examples:
Procedural Programming:
Focus: Procedural programming focuses on creating a step-by-step procedure or algorithm to solve a problem. It revolves around functions or procedures that manipulate data.
Data and Functions: In procedural programming, data and functions are treated separately. Data is stored in variables, and functions operate on this data.
Top-down Approach: Procedural programming typically follows a top-down approach, where the main program calls various functions to accomplish a task.
Example: Consider a program to calculate the area of a rectangle in procedural programming:Procedural Programming Example (Python)
def calculate_area(length, width):
return length * width
Main Program
length = 5
width = 3
area = calculate_area(length, width)
print("Area of the rectangle:", area)
Object Oriented Programming (OOP):
Focus: Object oriented programming focuses on modeling real-world entities as objects that have data (attributes) and behavior (methods).
Objects: In OOP, objects are instances of classes. Classes define the structure and behavior of objects.
Encapsulation: OOP promotes encapsulation, where data and methods that operate on the data are bundled together within a class, providing data hiding and abstraction.
Example: Consider a program to model a rectangle using OOP principles:Object Oriented Programming Example (Python)
class Rectangle:
def init(self, length, width):
self.length = length
self.width = width
def calculate_area(self): return self.length * self.width
Main Program
rectangle = Rectangle(5, 3)
area = rectangle.calculate_area()
print("Area of the rectangle:", area)
Comparison:
Procedural programming focuses on procedures or functions that manipulate data, whereas OOP focuses on modeling real-world entities as objects with data and behavior.
In procedural programming, data and functions are treated separately, while OOP promotes encapsulation by bundling data and methods together within classes.
Procedural programming follows a top-down approach, whereas OOP promotes a bottom-up approach, where objects collaborate to achieve a task.
In summary, while both paradigms have their strengths and weaknesses, object oriented programming is often preferred for its modularity, reusability, and ease of maintenance, especially for larger and more complex software projects.
Difference between procedural and object oriented programming
Here’s a comparison between procedural and object oriented programming (OOP) in tabular format:
Feature | Procedural Programming | Object Oriented Programming (OOP) |
---|---|---|
Focus | Focuses on procedures or functions | Focuses on modeling real-world entities as objects |
Data Handling | Data and functions are treated separately | Data and methods are bundled together within classes |
Main Concepts | Emphasizes functions, variables, and procedures | Emphasizes classes, objects, encapsulation, inheritance |
Top-Down Approach | Typically follows a top-down approach | Promotes a bottom-up approach |
Example | Calculating area of a rectangle using functions | Modeling a rectangle as an object with methods |
python | python | ||
def calculate_area(length, width): | class Rectangle: | |
return length * width | def init(self, length, width): | |
self.length = length | ||
length = 5 | self.width = width | |
width = 3 | ||
area = calculate_area(length, width) | def calculate_area(self): | |
print(“Area of the rectangle:”, area) | return self.length * self.width | |
rectangle = Rectangle(5, 3) | ||
area = rectangle.calculate_area() | ||
print(“Area of the rectangle:”, area) | ||
Advantages | Simple, suitable for small-scale programs | Promotes code reusability, modularity, and scalability |
Disadvantages | Limited code reuse, difficult to maintain for large apps | Can be complex for small-scale programs |
These FAQs provide a basic understanding of OOP concepts in Java and serve as a starting point for learning more about Java programming.
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
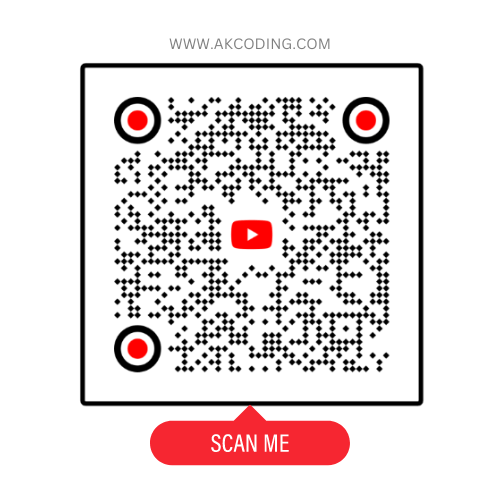