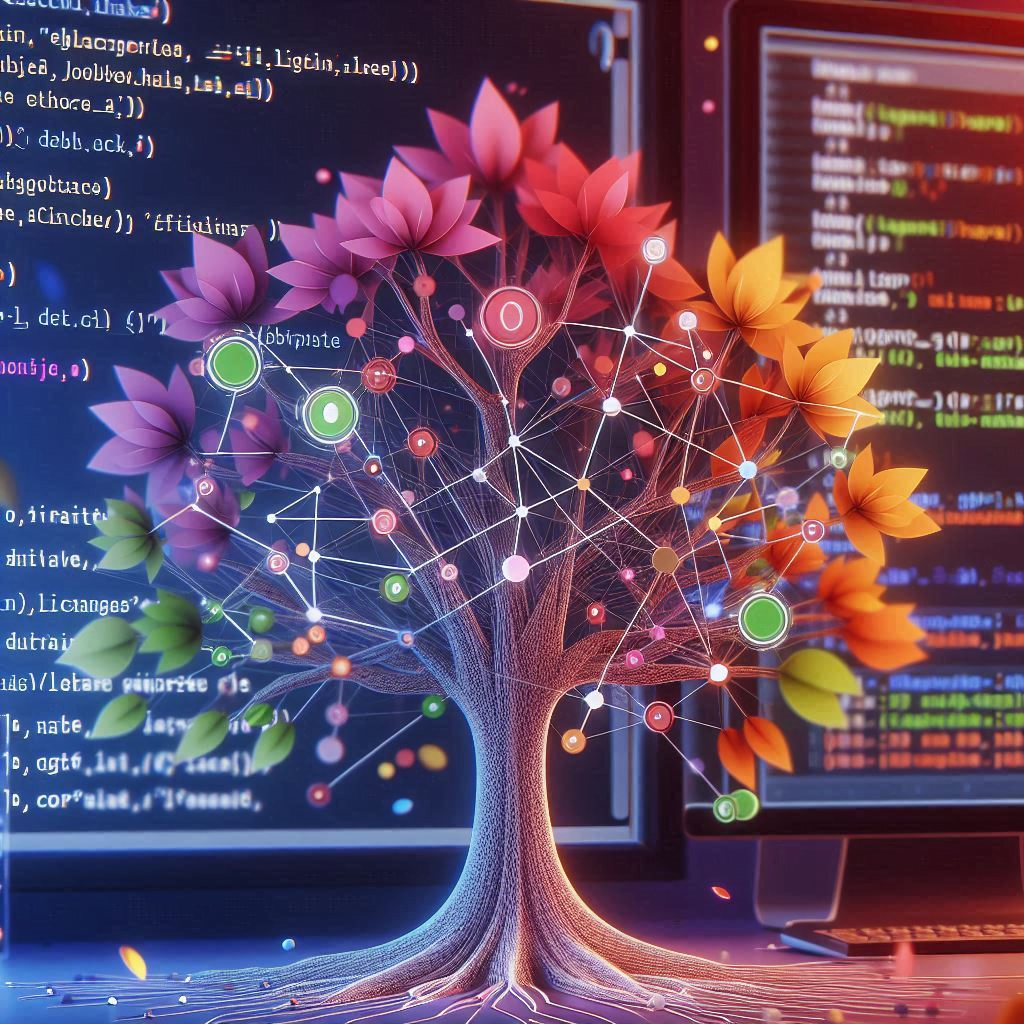
Table of Contents
1. Introduction
In the world of data structures and algorithms, Binary Search Trees (BSTs) are fundamental tools that offer efficient ways to store and retrieve ordered data. However, the performance of a standard BST can vary significantly depending on its shape and balance. This is where the concept of an Optimal Binary Search Tree (Optimal BST) comes into play.
An Optimal BST is designed to minimize the search cost by arranging nodes in such a way that frequently accessed elements are closer to the root. This optimization is crucial in scenarios where certain keys are accessed more frequently than others, such as in database indexing or file systems.
In this blog post, we will delve into the concept of an Optimal BST, exploring its definition, the algorithm used to construct it, and its practical applications. We’ll cover the following:
- Definition and Concept: Understand what makes a BST optimal and how it differs from a regular BST.
- Algorithm and Approach: Learn about the dynamic programming approach used to create an Optimal BST and see a step-by-step illustration.
- Applications and Benefits: Explore the real-world advantages of using an Optimal BST and where it is most beneficial.
- Limitations and Considerations: Examine the constraints and trade-offs involved in implementing an Optimal BST.
- Comparison with Other Structures: Compare Optimal BSTs with other balanced tree structures to understand their relative advantages and use cases.
By the end of this post, you’ll have a clear understanding of how to design an Optimal Binary Search Tree and why it can be a valuable asset in optimizing data retrieval processes. Let’s dive in!
2. Understanding Binary Search Trees
Binary Search Trees (BSTs) are a specialized type of binary tree that maintain a sorted order of elements, which facilitates efficient searching, insertion, and deletion operations. To understand Optimal Binary Search Trees, it’s crucial first to grasp the fundamental concepts of BSTs. Here’s a detailed breakdown:
Definition of a Binary Search Tree
A Binary Search Tree is a binary tree with the following properties:
- Each node has at most two children: A left child and a right child.
- Node ordering: For any given node:
- The value of all nodes in the left subtree is less than the value of the node.
- The value of all nodes in the right subtree is greater than the value of the node.
These properties ensure that the BST can perform operations like search, insert, and delete efficiently.
Key Properties of BSTs
- Inorder Traversal: An inorder traversal of a BST visits nodes in ascending order of their values. This property is used to retrieve data in a sorted sequence.
- Height: The height of a BST affects its performance. The height is the number of edges on the longest path from the root to a leaf. A balanced BST has a height of O(log n), where n is the number of nodes, leading to efficient operations.
- Search Time Complexity: The average time complexity for search operations in a balanced BST is O(log n), but it can degrade to O(n) in the worst case if the tree becomes unbalanced.
Basic Operations
- Insertion: To insert a node, start at the root and compare the value with the current node. Move left if the value is less and right if greater, until you find the correct spot to place the new node.
- Deletion: To delete a node, locate the node and consider three scenarios:
- Node with no children: Simply remove it.
- Node with one child: Replace the node with its child.
- Node with two children: Find the in-order successor (smallest value in the right subtree) or predecessor (largest value in the left subtree) to replace the node and then delete the successor or predecessor.
- Search: Start at the root and compare the target value with the current node. Move left or right based on comparison until the value is found or a leaf node is reached.
Limitations of Standard BSTs
- Unbalanced Trees: If nodes are inserted in a sorted order, the BST can become unbalanced, resembling a linked list, which leads to poor performance (O(n) time complexity for operations).
- Performance Issues: The efficiency of search, insert, and delete operations heavily depends on the height of the tree.
In summary, while BSTs provide a structured way to manage and access ordered data, their performance can be compromised if they are not balanced. This is why optimization strategies, such as those used in Optimal Binary Search Trees, are important to ensure efficient operation in real-world applications.
3. What is an Optimal Binary Search Tree?
An Optimal Binary Search Tree (Optimal BST) is a specialized form of a Binary Search Tree designed to minimize the total search cost based on the frequency of access for each key. The goal of constructing an Optimal BST is to achieve the most efficient search performance for a given set of keys with known access frequencies.
Definition and Concept
An Optimal BST is defined by the following criteria:
- Minimizing Search Cost: The primary objective is to minimize the weighted path length or total search cost, which is the sum of the products of the access frequencies of each key and their respective depths in the tree. Depth refers to the distance of a node from the root, where the root node has a depth of 0.
- Access Frequency: In an Optimal BST, each key is associated with an access frequency, representing how often the key is accessed. This frequency helps determine the optimal arrangement of the keys within the tree.
- Cost Function: The cost of a BST is computed based on the depth of each node and its access frequency. For example, if a key is accessed frequently and is placed closer to the root, the overall cost is reduced. Conversely, infrequently accessed keys should be placed further from the root to minimize their impact on the total cost.
How It Differs from a Regular BST
- Standard BST: In a standard BST, the tree is structured based on key ordering but does not take access frequencies into account. As a result, the tree might not be optimized for search efficiency if the keys are not evenly distributed or if access patterns vary significantly.
- Optimal BST: An Optimal BST considers the access frequencies and arranges the keys in such a way that the most frequently accessed keys are closer to the root, thereby reducing the average search time and overall cost. This optimization is achieved through a careful construction process using dynamic programming.
Key Criteria for Optimization
- Minimizing Average Search Time: By placing frequently accessed keys nearer to the root, the Optimal BST reduces the average time required to access each key.
- Balancing Cost vs. Structure: While the goal is to minimize search cost, the Optimal BST also aims to balance the tree structure to avoid excessive height or skewed configurations.
Optimal BST Algorithm
The construction of an Optimal BST typically involves dynamic programming techniques to evaluate different tree configurations and select the one with the minimum total search cost. This process involves:
- Frequency Calculation: Determining the access frequencies for each key.
- Cost Matrix Construction: Computing the cost of various subtree configurations.
- Optimal Tree Construction: Using the cost matrix to build the tree with the minimum cost.
In summary, an Optimal Binary Search Tree is a refined version of a BST that is specifically designed to optimize search performance by considering key access frequencies. By minimizing the total search cost, it provides significant advantages in applications where efficient data retrieval is crucial.
4. Optimal Binary Search Tree Algorithm
The Optimal Binary Search Tree (Optimal BST) algorithm is a dynamic programming approach used to construct a binary search tree that minimizes the total search cost based on the access frequencies of the keys. Here’s a step-by-step explanation of how the algorithm works:
1. Problem Formulation
Given:
- A set of keys, each associated with a probability or frequency of access.
- A set of costs for unsuccessful searches (if applicable).
Objective:
- Construct a binary search tree that minimizes the expected search cost.
2. Dynamic Programming Approach
The algorithm uses dynamic programming to determine the optimal tree structure. It involves the following steps:
- Define Subproblems:
- Let
cost[i][j]
represent the minimum cost of constructing an Optimal BST from keysi
toj
.
- Initialize Base Cases:
- For a single key
k
, the cost is just the probability of accessing that key:
[
\text{cost}[i][i] = \text{frequency}[i]
]
- Compute Costs for Subtrees:
- For each possible subtree size, compute the cost of the subtree and its optimal root. The cost is derived from the sum of the subtree costs plus the cost of the root node:
[
\text{cost}[i][j] = \min_{i \leq r \leq j} \left( \text{cost}[i][r-1] + \text{cost}[r+1][j] + \text{sum_freq}[i][j] \right)
]
wheresum_freq[i][j]
is the sum of frequencies from keyi
toj
.
- Iterate Over All Subtrees:
- Iterate through all possible lengths of subtrees and possible roots for each subtree length. Update the cost matrix with the minimum cost for each subtree.
- Compute Final Result:
- The final result, which is the minimum cost for the entire set of keys, is found in
cost[0][n-1]
, wheren
is the total number of keys.
Algorithm Steps
- Calculate the Frequency Sum Matrix:
- Create a matrix
sum_freq[i][j]
where each entry is the sum of access frequencies from keyi
toj
.
- Initialize the Cost Matrix:
- Initialize the diagonal entries of the cost matrix for single keys.
- Fill the Cost Matrix:
- For each subtree length from 2 to
n
, and for each possible starting indexi
, calculate the cost for all possible root nodesr
and updatecost[i][j]
.
- Extract the Optimal BST Structure:
- Use the computed cost matrix to reconstruct the Optimal BST structure if needed.
Example Illustration
Consider a simple example with keys and their frequencies:
- Keys:
[10, 20, 30]
- Frequencies:
[4, 2, 6]
- Compute
sum_freq
:
sum_freq[0][1] = 6
sum_freq[1][2] = 8
sum_freq[0][2] = 12
- Initialize and fill the
cost
matrix:
- For single keys:
cost[0][0] = 4
,cost[1][1] = 2
,cost[2][2] = 6
- For pairs and triples, compute the minimum cost and update the matrix.
- The result in
cost[0][2]
will give the minimum cost for the entire set of keys.
Complexity
- Time Complexity: O(n^3), where
n
is the number of keys. This is due to the triple nested loops in the dynamic programming approach. - Space Complexity: O(n^2) for storing the cost and frequency matrices.
In summary, the Optimal BST algorithm efficiently constructs a binary search tree that minimizes the total search cost using dynamic programming techniques. By considering the access frequencies and possible subtree configurations, it ensures the most cost-effective arrangement of keys.
Dynamic Programming Approach for Optimal Binary Search Tree
The dynamic programming approach for constructing an Optimal Binary Search Tree (Optimal BST) is a method to efficiently determine the most cost-effective arrangement of keys in a binary search tree based on their access frequencies. Here’s a detailed look at how dynamic programming is applied to solve this problem:
1. Problem Breakdown
The problem involves finding a binary search tree structure that minimizes the total cost of searches, where the cost is calculated based on the depth of each node and its access frequency. The steps include:
- Defining Subproblems:
- We need to find the minimum cost of a BST for any given subarray of keys.
- Using Optimal Substructure:
- The cost of the optimal tree for a subarray can be derived from the costs of smaller subarrays.
2. Dynamic Programming Table
To solve the problem, we use a 2D table cost[i][j]
where cost[i][j]
represents the minimum cost of an optimal BST that contains keys from index i
to j
.
- Base Case:
- For a single key, the cost is simply the frequency of that key:
[
\text{cost}[i][i] = \text{frequency}[i]
] - Recursive Relation:
- For a subarray from
i
toj
, choose each keyr
as the root and compute the cost:
[
\text{cost}[i][j] = \min_{i \leq r \leq j} \left( \text{cost}[i][r-1] + \text{cost}[r+1][j] + \text{sum_freq}[i][j] \right)
]
wheresum_freq[i][j]
is the sum of frequencies from keyi
toj
.
3. Steps to Implement
- Initialize the Cost and Frequency Sum Matrices:
- Create matrices
cost
andsum_freq
where:cost[i][i] = frequency[i]
(base case for single key)sum_freq[i][j]
is the sum of frequencies fromi
toj
.
- Compute Frequency Sums:
- Compute
sum_freq
for all subarrays[i][j]
:
[
\text{sum_freq}[i][j] = \text{frequency}[i] + \text{frequency}[i+1] + \cdots + \text{frequency}[j]
]
- Fill the Cost Matrix:
- For increasing lengths of subarrays, compute the cost for all possible roots:
- For each length from 2 to
n
, and for each subarray starting indexi
: - Compute
cost[i][j]
by choosing each possible rootr
within the subarray and calculating the minimum cost.
- For each length from 2 to
- Extract the Optimal Cost:
- The final minimum cost for the entire array of keys will be found in
cost[0][n-1]
, wheren
is the total number of keys.
4. Example
Consider three keys with frequencies:
- Keys:
[10, 20, 30]
- Frequencies:
[4, 2, 6]
- Initialize Tables:
cost[0][0] = 4
,cost[1][1] = 2
,cost[2][2] = 6
- Compute
sum_freq
values:sum_freq[0][1] = 6
sum_freq[1][2] = 8
sum_freq[0][2] = 12
- Compute Costs for Subarrays:
- For subarrays of length 2 and 3, calculate the minimum cost by considering each key as the root and using the previously computed costs.
- Final Result:
- The value in
cost[0][2]
represents the minimum cost for the entire set of keys.
5. Complexity
- Time Complexity: O(n^3) due to the nested loops required to fill the
cost
matrix. - Space Complexity: O(n^2) for the
cost
andsum_freq
matrices.
The dynamic programming approach efficiently solves the Optimal BST problem by breaking it into manageable subproblems and using previously computed results to build up the solution. This approach ensures that the overall cost of the BST is minimized based on the access frequencies of the keys.
Applications and Benefits of Optimal Binary Search Trees (Optimal BSTs)
Applications of Optimal Binary Search Trees
- Database Indexing:
- Optimal BSTs are used in database systems to construct indices that minimize the cost of lookups. Efficient indexing helps in quick retrieval of data, improving query performance.
- Compiler Design:
- In compilers, Optimal BSTs can be used to implement symbol tables, where symbols are organized in a way that minimizes access times during compilation.
- Memory Management:
- Optimal BSTs can be applied in memory management systems where data access patterns are known. By organizing data in an optimal way, access times can be minimized.
- Information Retrieval Systems:
- Search engines and other information retrieval systems use Optimal BSTs to store and retrieve documents efficiently based on query frequencies and access patterns.
- Decision-Making Systems:
- Systems that involve decision-making based on probability distributions can use Optimal BSTs to minimize the average decision cost by organizing decisions in an optimal structure.
- Network Routing:
- Optimal BSTs can be used in network routing algorithms where nodes represent network addresses and access frequencies represent the likelihood of data transfer, optimizing routing paths.
Benefits of Optimal Binary Search Trees
- Minimized Search Cost:
- The primary benefit of Optimal BSTs is the minimization of search cost. By organizing the keys based on their access frequencies, the expected search time is reduced, leading to faster data retrieval.
- Efficient Memory Usage:
- Optimal BSTs ensure that frequently accessed keys are located closer to the root, reducing the overall depth of the tree and, consequently, memory access times.
- Improved Performance:
- With an optimal structure, the performance of operations such as search, insertion, and deletion is improved compared to non-optimal or randomly structured trees.
- Cost-Effective Operations:
- For systems with predictable access patterns, Optimal BSTs provide a cost-effective solution by reducing the number of operations required to access or update data.
- Scalability:
- Optimal BSTs can handle large datasets efficiently. As the size of the dataset grows, the tree structure ensures that search times remain optimal, supporting scalable applications.
- Balanced Structure:
- Although not always perfectly balanced, the Optimal BST provides a balanced structure that avoids the worst-case scenarios of unbalanced trees, ensuring more consistent performance.
- Predictable Access Times:
- By considering access frequencies, Optimal BSTs offer predictable and reliable access times, which is crucial for systems requiring consistent performance.
- Enhanced System Design:
- Using Optimal BSTs in system design helps in creating more efficient and user-friendly systems by reducing latency and improving the overall responsiveness of the system.
Optimal Binary Search Trees offer significant advantages in various applications where access frequencies are known and can be leveraged to minimize search costs. Their benefits in terms of performance, memory efficiency, and scalability make them a valuable tool in designing efficient data structures and systems. By optimizing the arrangement of keys, Optimal BSTs ensure that data retrieval is both fast and cost-effective, enhancing the overall efficiency of computational systems.
Limitations and Considerations of Optimal Binary Search Trees
While Optimal Binary Search Trees (Optimal BSTs) offer numerous benefits, they also come with certain limitations and considerations that need to be addressed. Here’s a comprehensive look at these aspects:
1. Complexity of Construction
- High Computational Cost:
- Constructing an Optimal BST involves dynamic programming, which can be computationally expensive, especially for large datasets. The time complexity of O(n^3) can be prohibitive for very large numbers of keys.
- Memory Usage:
- The dynamic programming approach requires substantial memory to store the
cost
andsum_freq
matrices. This can be a limitation in environments with limited resources.
2. Static Nature
- Non-Dynamic:
- Optimal BSTs are typically constructed based on static access frequencies. They are not well-suited for environments where access patterns change frequently, as recalculating the optimal tree for every change can be inefficient.
- Rebalancing Issues:
- If the access patterns or key set changes over time, maintaining an optimal BST requires rebalancing or reconstructing the tree, which can be costly in terms of both time and resources.
3. Applicability
- Limited Use Cases:
- Optimal BSTs are most effective when access frequencies are known and do not change frequently. In scenarios with unpredictable or highly dynamic access patterns, other data structures may be more appropriate.
- Overhead for Small Datasets:
- For smaller datasets, the overhead of constructing and maintaining an Optimal BST might outweigh its benefits, especially if the performance gain is marginal.
4. Implementation Complexity
- Algorithmic Complexity:
- The algorithm to compute the Optimal BST can be complex to implement and understand. It requires a good grasp of dynamic programming techniques and the ability to manage multiple dimensions of data.
- Code Maintenance:
- Maintaining and debugging code that involves dynamic programming for Optimal BSTs can be challenging, particularly for developers who are not familiar with this approach.
5. Trade-Offs
- Space vs. Time Trade-Off:
- Optimal BSTs provide time efficiency in search operations at the cost of increased space complexity. This trade-off may not be ideal for all applications, especially those with strict space constraints.
- Fixed Structure:
- The structure of an Optimal BST is fixed once constructed, and it may not adapt well to changes in access patterns. For applications requiring adaptive structures, more flexible data structures might be necessary.
6. Performance in Practice
- Real-World Performance:
- In practice, the performance benefits of Optimal BSTs may be less pronounced than theoretical calculations suggest, especially if real-world access patterns are not as uniform as assumed.
- Complexity of Real Data:
- Real-world data often involves complex and unpredictable access patterns that do not always fit neatly into the assumptions required for Optimal BST construction.
Optimal Binary Search Trees provide valuable benefits in specific scenarios where access frequencies are known and stable. However, their limitations, including computational complexity, static nature, and implementation challenges, must be carefully considered. When applying Optimal BSTs, it’s crucial to evaluate whether their advantages outweigh the limitations for your particular use case. For dynamic environments or large datasets, alternative data structures or strategies may be more suitable.
Comparison of Optimal Binary Search Trees with Other Tree Structures
When comparing Optimal Binary Search Trees (Optimal BSTs) with other tree structures, it’s important to understand their unique characteristics, advantages, and limitations. Here’s a detailed comparison:
1. Binary Search Tree (BST)
- Structure:
- BST: A Binary Search Tree has nodes arranged such that for any given node, all left descendants have smaller values and all right descendants have larger values.
- Optimal BST: An Optimal BST is a special case of BST where the arrangement of nodes minimizes the total search cost based on known access frequencies.
- Construction:
- BST: Can be constructed in O(n) time with no specific balancing strategy.
- Optimal BST: Constructed using dynamic programming with a time complexity of O(n^3), aiming to minimize the search cost.
- Performance:
- BST: Performance depends on the tree’s balance; can degrade to O(n) in the worst case if the tree becomes unbalanced.
- Optimal BST: Provides optimal search time for a given set of access frequencies but requires more effort to maintain.
- Use Cases:
- BST: Useful in scenarios where average-case performance is acceptable and dynamic insertions and deletions are frequent.
- Optimal BST: Best used when access patterns are static and known in advance, optimizing search time.
2. AVL Tree
- Structure:
- AVL Tree: A self-balancing binary search tree where the difference in heights between left and right subtrees (balance factor) is at most one.
- Construction and Maintenance:
- AVL Tree: Balancing is maintained with rotations during insertions and deletions, ensuring O(log n) height.
- Performance:
- AVL Tree: Guarantees O(log n) time complexity for search, insertion, and deletion operations.
- Use Cases:
- AVL Tree: Suitable for applications requiring frequent insertions and deletions with guaranteed log-time performance.
- Comparison:
- Optimal BST: Optimal for static access patterns but not balanced. AVL Trees provide better performance in dynamic environments due to self-balancing.
3. Red-Black Tree
- Structure:
- Red-Black Tree: A self-balancing binary search tree where nodes are colored (red or black) to maintain balance and ensure O(log n) height.
- Construction and Maintenance:
- Red-Black Tree: Balancing is maintained with color flips and rotations, ensuring O(log n) height and performance.
- Performance:
- Red-Black Tree: Guarantees O(log n) time complexity for search, insertion, and deletion operations.
- Use Cases:
- Red-Black Tree: Ideal for applications requiring efficient performance with frequent modifications.
- Comparison:
- Optimal BST: Provides better search efficiency for static datasets with known access frequencies, while Red-Black Trees ensure balanced performance in dynamic scenarios.
4. B-Tree
- Structure:
- B-Tree: A self-balancing tree data structure that maintains sorted data and allows searches, insertions, and deletions in logarithmic time.
- Construction and Maintenance:
- B-Tree: Suitable for systems with large amounts of data and where nodes are stored on disk.
- Performance:
- B-Tree: Designed for efficient data retrieval with O(log n) time complexity for search, insertion, and deletion.
- Use Cases:
- B-Tree: Commonly used in databases and file systems for efficient data retrieval and storage.
- Comparison:
- Optimal BST: Best for in-memory applications with static access patterns, while B-Trees are more suited for disk-based storage systems and large datasets.
5. Trie (Prefix Tree)
- Structure:
- Trie: A tree data structure used for efficient retrieval of keys with a common prefix. It is often used in applications such as autocomplete.
- Performance:
- Trie: Provides O(m) time complexity for search, where m is the length of the key. It is particularly useful for prefix-based queries.
- Use Cases:
- Trie: Ideal for scenarios involving prefix searches and dictionary implementations.
- Comparison:
- Optimal BST: Optimized for general search scenarios with known access frequencies, while Tries are specialized for prefix-based searches.
When choosing between Optimal Binary Search Trees and other tree structures, consider the specific needs of your application. Optimal BSTs are designed to minimize search cost for static datasets with known access frequencies, whereas AVL Trees, Red-Black Trees, B-Trees, and Tries offer various benefits in terms of balancing, disk storage, and specialized searches. Understanding these differences can help you select the most suitable data structure for your use case.
Implementation of Optimal Binary Search Tree
Implementing an Optimal Binary Search Tree (Optimal BST) involves constructing a binary search tree that minimizes the total search cost based on given access frequencies for each key. This problem is typically solved using dynamic programming. Here’s a step-by-step guide to implementing an Optimal BST:
1. Problem Definition
Given a set of keys with known access frequencies, the goal is to construct a binary search tree that minimizes the expected search cost. The cost is defined by the sum of the depths of the nodes weighted by their frequencies.
2. Dynamic Programming Approach
The dynamic programming approach involves solving subproblems and using their solutions to construct the optimal BST.
Step-by-Step Implementation:
Step 1: Define Data Structures
- Input:
keys[]
: Array of keys.freq[]
: Array of access frequencies corresponding to the keys.- Output:
cost[][]
: Matrix wherecost[i][j]
represents the cost of the optimal BST for keys from indexi
toj
.root[][]
: Matrix whereroot[i][j]
stores the root index of the optimal BST for keys from indexi
toj
.
Step 2: Initialize Tables
- Cost Table:
- Initialize a 2D array
cost[][]
of sizen x n
(wheren
is the number of keys) with0
. - Root Table:
- Initialize a 2D array
root[][]
of sizen x n
with0
.
Step 3: Compute Cost and Root Tables
Algorithm:
- Base Case:
- For a single key, the cost is simply its frequency, and it is the root of itself.
for i in range(n):
cost[i][i] = freq[i]
root[i][i] = i
- Subproblems:
- Compute costs for subtrees with more than one key.
- For each possible subtree defined by a range
[i, j]
, calculate the cost for each possible rootr
and choose the root that minimizes the cost.
for length in range(2, n+1): # length of the subtree
for i in range(n - length + 1):
j = i + length - 1
cost[i][j] = float('inf')
sum_freq = sum(freq[i:j+1])
for r in range(i, j+1):
c = (cost[i][r-1] if r > i else 0) + (cost[r+1][j] if r < j else 0) + sum_freq
if c < cost[i][j]:
cost[i][j] = c
root[i][j] = r
Step 4: Construct the Optimal BST
- Use the
root[][]
table to construct the tree recursively.
def construct_optimal_bst(root, keys, i, j):
if i > j:
return None
r = root[i][j]
node = TreeNode(keys[r])
node.left = construct_optimal_bst(root, keys, i, r-1)
node.right = construct_optimal_bst(root, keys, r+1, j)
return node
TreeNode Definition:
class TreeNode:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
Step 5: Test the Implementation
- Define test cases with various sets of keys and frequencies to verify the correctness of the implementation.
keys = [10, 12, 20]
freq = [34, 8, 50]
n = len(keys)
# Initialize tables
cost = [[0]*n for _ in range(n)]
root = [[0]*n for _ in range(n)]
# Compute cost and root tables
compute_cost_and_root(keys, freq, cost, root, n)
# Construct the Optimal BST
bst_root = construct_optimal_bst(root, keys, 0, n-1)
Implementing an Optimal Binary Search Tree involves setting up dynamic programming tables to calculate the cost of different subtrees and their optimal roots. By leveraging these calculations, you can construct a tree that minimizes the expected search cost. This approach is particularly useful for applications where access frequencies are known and static, optimizing search efficiency for large datasets.
Conclusion
The Optimal Binary Search Tree (Optimal BST) is a powerful data structure used to minimize search costs in scenarios where access frequencies for keys are known. By constructing a binary search tree that reduces the average search time, Optimal BSTs enhance the efficiency of data retrieval operations, making them invaluable for applications with predictable and static access patterns.
Key Takeaways:
- Efficient Searching: Optimal BSTs are designed to minimize the expected search cost, which is crucial for applications requiring frequent and efficient data access. By placing more frequently accessed keys closer to the root, the search operations become faster and more efficient.
- Dynamic Programming Approach: The dynamic programming approach to constructing an Optimal BST involves solving subproblems and combining their solutions to build the final tree. This method ensures that the constructed tree is indeed optimal for the given set of keys and their access frequencies.
- Implementation: Implementing an Optimal BST involves calculating the cost and root tables, which are then used to construct the tree. This process, though computationally intensive, provides a structure that significantly improves search performance.
- Applications: Optimal BSTs are particularly useful in applications with static data access patterns, such as database indexing, memory management, and other areas where search efficiency is critical.
- Limitations: While Optimal BSTs are effective for static datasets, they may not be as beneficial for dynamic datasets where access patterns frequently change. In such cases, other data structures or self-balancing trees might be more appropriate.
- Comparison with Other Structures: Compared to other tree structures, Optimal BSTs offer a focused approach to minimizing search costs but may not address other concerns such as insertion and deletion efficiency. Understanding their advantages and limitations helps in selecting the appropriate data structure based on specific application needs.
In summary, Optimal Binary Search Trees provide a structured approach to reducing search costs through careful construction based on access frequencies. While they excel in scenarios with static data, their application should be carefully considered in dynamic environments. By leveraging dynamic programming techniques, Optimal BSTs ensure efficient and effective data retrieval, contributing to overall system performance and user experience.
Read other awesome articles in Medium.com or in akcoding’s posts, you can also join our YouTube channel AK Coding