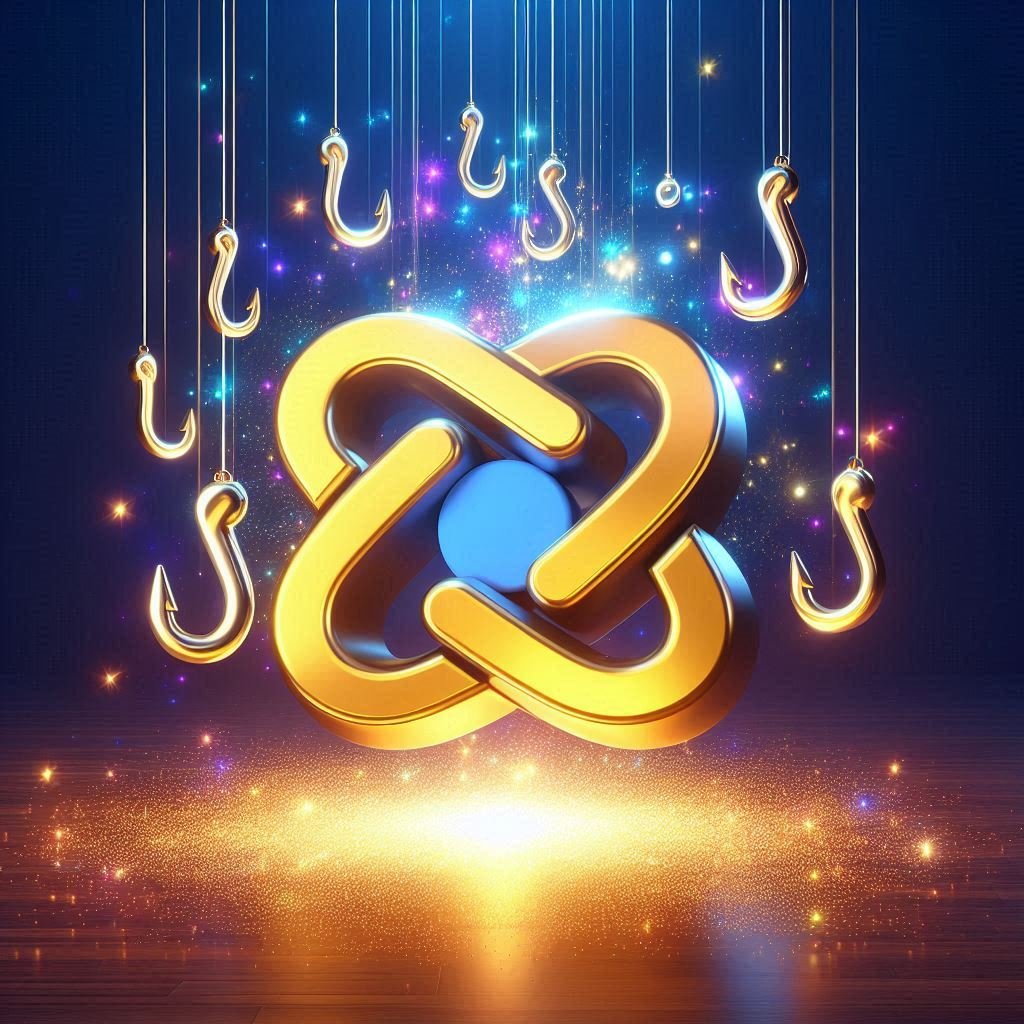
Introduction to React Hooks
React Hooks are a fundamental feature in React that revolutionized the way developers work with functional components. Introduced in React 16.8, Hooks enable developers to use state and other React features without converting functional components into class components. This innovation has led to simpler, more maintainable code and improved component reusability.
Table of Contents
Definition and Purpose of React Hooks
React Hooks are special functions that allow you to “hook into” React features like state management and lifecycle events within functional components. Before Hooks, these capabilities were exclusive to class components, requiring developers to use complex structures and lifecycle methods. Hooks bridge this gap, offering a unified approach to managing component state, side effects, and other complex behaviors.
The primary purposes of React Hooks include:
- Simplifying Component Logic: Hooks enable you to organize logic by feature, rather than lifecycle methods or classes.
- Enhancing Reusability: With custom Hooks, you can extract common logic from components, promoting code reuse and cleaner architecture.
- Reducing Boilerplate: Hooks eliminate the need for class-based syntax and lifecycle methods, resulting in less boilerplate code.
How Hooks Change Functional Component Behavior
Hooks change the behavior of functional components by allowing them to maintain internal state and handle side effects, features traditionally associated with class components. With Hooks, functional components can now:
- Use State: The
useState
hook enables functional components to have internal state, just like class components. This makes it easier to create interactive and dynamic components. - Handle Side Effects: The
useEffect
hook allows functional components to manage side effects, such as fetching data or subscribing to events. This effectively replaces lifecycle methods likecomponentDidMount
andcomponentDidUpdate
. - Access Context: The
useContext
hook provides a straightforward way to consume context, reducing the need for prop drilling and making state sharing across components simpler.
These changes have made functional components the preferred approach for many React developers, as they are generally more concise and easier to understand.
Common Use Cases for React Hooks
React Hooks are versatile and can be used in a variety of scenarios. Some common use cases include:
- Managing Local State: Using
useState
to handle local component state, such as form inputs or toggles. - Performing Side Effects: Employing
useEffect
to fetch data from APIs, set up event listeners, or update the document title. - Complex State Management: Using
useReducer
for more complex state logic, resembling a Redux-like approach. - Creating Custom Hooks: Building reusable logic with custom Hooks to encapsulate behaviors like form validation, data fetching, or authentication.
- Accessing Context: Using
useContext
to consume context values without passing props through multiple layers.
These use cases demonstrate the flexibility and power of React Hooks, allowing developers to build robust, maintainable React applications without the complexities of class components.
Types of React Hooks
Basic Hooks
- useState
- The
useState
hook in React allows functional components to manage state. It initializes a state variable with a default value and provides a function to update that state.useState
is crucial for making React components dynamic and responsive to user input or other changes. Here’s an example:
const [count, setCount] = useState(0);
- In this example,
count
holds the current state value, andsetCount
is used to update it.useState
makes it easy to manage component-level state without needing class components.
- useEffect
useEffect
is a React Hook that allows you to perform side effects in functional components. It’s used for tasks like fetching data, directly interacting with the DOM, or setting up subscriptions. The hook runs after the component renders and can be configured to run on every render, only on specific state or prop changes, or once on component mount and unmount. By usinguseEffect
, you can manage side effects efficiently within React’s functional components, ensuring clean and predictable behavior.
- useContext
- The
useContext
hook in React allows components to access and consume values from a context without the need to pass props manually through every level of the component tree. It simplifies the process of sharing global data (like themes, user authentication, or settings) between deeply nested components. By usinguseContext
, you can retrieve the context value directly in any functional component, making state management easier and more efficient in large applications.
const value = useContext(MyContext);
Additional Built-in Hooks
- useReducer
- The
useReducer
hook in React is used for managing more complex state logic compared touseState
. It is particularly helpful when the state transitions involve multiple actions or when the state depends on previous values.useReducer
takes in a reducer function and an initial state, returning the current state and a dispatch function. It’s ideal for scenarios like managing form inputs or implementing complex state logic similar to Redux. - Example:
- The
const [state, dispatch] = useReducer(reducer, initialState);
This provides a more structured way of handling state updates through action dispatching.
- useRef
- The
useRef
hook in React is used to create a mutable reference that persists across renders without causing re-renders when updated. It can be used to directly access and manipulate DOM elements or to store any mutable value that you want to keep between renders without triggering a re-render.- For example, it is commonly used for:
- Accessing DOM elements (
ref.current
gives you the reference). - Storing values like timers, previous states, or external libraries that need to persist across renders.
- The
- useCallback
- The
useCallback
hook in React is used to memoize functions, preventing them from being recreated on every render. This is particularly useful when passing functions as props to child components, as it helps optimize performance by reducing unnecessary re-renders.useCallback
returns a memoized version of the function that only changes if the dependencies change, making it ideal for performance tuning in complex React applications.
- The
- useMemo
useMemo
is a React hook used to optimize performance by memoizing the result of expensive calculations. It allows you to recompute a value only when its dependencies change, preventing unnecessary recalculations on every render. This is particularly useful for optimizing rendering of large components or when running complex computations.- Example:
- const memoizedValue = useMemo(() => expensiveFunction(data), [data]);
- In this example,
useMemo
ensures thatexpensiveFunction
is only recalculated whendata
changes, reducing performance overhead.
- useLayoutEffect
useLayoutEffect
is a React hook that is similar touseEffect
, but it fires synchronously after all DOM mutations are completed. This means it runs before the browser repaints, allowing you to make DOM updates and measurements before the user sees the changes. It is typically used for tasks that require immediate DOM manipulations, such as measuring element sizes or synchronizing UI layout updates. However, it should be used cautiously, as it can block visual updates if overused. In most cases,useEffect
is preferred for non-urgent side effects.
- useImperativeHandle
useImperativeHandle
is a React hook that allows you to customize the instance value that is exposed when usingref
in functional components. It is often used withforwardRef
to provide a way for parent components to interact with child component methods or properties.
Custom Hooks
Custom Hooks in React are a powerful way to encapsulate reusable logic across components. They allow you to extract component logic into a function that can be shared among multiple components, promoting code reusability and cleaner component architecture. A custom Hook is simply a JavaScript function that starts with the word “use” and can call built-in Hooks like useState
, useEffect
, and others. This enables you to manage state, handle side effects, and more, while keeping your components focused on rendering UI. Custom Hooks can help simplify complex components and improve maintainability by organizing related logic in one place.
Best Practices for Using Hooks
Best Practices for Using Hooks
React Hooks offer a modern approach to building React applications with simpler code, enhanced reusability, and improved functionality. To maximize the benefits of Hooks while avoiding common pitfalls, it’s essential to follow best practices. Here are some key best practices for using Hooks effectively in React projects:
1. Follow Hook Rules
- Only Call Hooks at the Top Level: Hooks should be called at the top level of your component or custom Hook, not inside loops, conditions, or nested functions. This ensures consistent Hook execution order.
- Only Call Hooks from React Functions: Hooks should be used only within React functional components or custom Hooks, not in class components or non-React functions.
2. Manage State with useState
- Use Descriptive State Variables: Choose clear and meaningful names for state variables to improve code readability.
- Update State with Functional Updates: When updating state based on the previous state, use the functional form of
useState
to avoid potential issues with stale state.
const [count, setCount] = useState(0);
setCount((prevCount) => prevCount + 1);
3. Handle Side Effects with useEffect
- Specify Dependency Arrays: Always include a dependency array with
useEffect
to control when the effect runs. This prevents unintended side effects and infinite loops. - Cleanup Effects: Use cleanup functions within
useEffect
to manage resource allocation and avoid memory leaks, especially when setting up event listeners or timers.
useEffect(() => {
const interval = setInterval(() => {
console.log("Interval running");
}, 1000);
return () => clearInterval(interval);
}, []);
4. Avoid Excessive Re-renders
- Memoize Functions with
useCallback
: When passing functions as props, useuseCallback
to avoid unnecessary re-renders. - Memoize Values with
useMemo
: UseuseMemo
to memoize complex calculations or derived state to improve performance.
const memoizedValue = useMemo(() => complexCalculation(), [dependency]);
const memoizedCallback = useCallback(() => someFunction(), [dependency]);
5. Use Context and Custom Hooks Wisely
- Minimize Context Usage: While
useContext
is useful, avoid overusing it, as excessive context can lead to tightly coupled components. - Create Reusable Custom Hooks: Custom Hooks allow you to encapsulate common logic and improve code reuse. Follow the same Hook rules when creating custom Hooks.
6. Stay Consistent with Component Structure
- Separate Concerns: Keep component logic focused on a specific responsibility. Use multiple
useEffect
calls to separate different side effects. - Use Hook Linting Tools: Tools like ESLint with the “eslint-plugin-react-hooks” plugin help ensure that Hooks are used correctly.
These best practices for using React Hooks can help you write clean, efficient, and maintainable code. Following these guidelines will also reduce the risk of common bugs and improve the overall quality of your React applications.
Applications of React Hooks
Applications of React Hooks
React Hooks are a powerful feature that allows developers to use state and lifecycle-related functionalities in functional components. Since their introduction, React Hooks have transformed how React applications are built and maintained. Here are some of the most common applications of React Hooks in modern React development:
1. State Management within Functional Components
- Local State Management: Hooks like
useState
allow functional components to manage local state, providing a simpler alternative to class components. This is commonly used for interactive elements like forms, counters, and toggles. - Complex State Logic with
useReducer
: In more complex cases, such as managing a to-do list or shopping cart,useReducer
allows for Redux-like state management within functional components.
2. Handling Side Effects and Component Lifecycle
- Side Effects with
useEffect
: This hook replaces lifecycle methods in class components. It is used for handling side effects such as data fetching, subscriptions, timers, and DOM manipulations. - Layout Effects with
useLayoutEffect
: When you need to interact with the DOM before it is painted,useLayoutEffect
provides a solution, useful for scenarios like animations or manipulating layout-critical elements.
3. Reusing Logic with Custom Hooks
- Creating Reusable Logic: Custom Hooks enable you to extract common functionality into reusable components. For example, you can create custom Hooks for data fetching, form validation, or authentication.
- Example Applications: A custom Hook for managing form state can be reused across multiple forms, reducing code duplication and promoting cleaner code structure.
4. Consuming Context Data
- Simplifying Context Consumption: The
useContext
hook allows components to easily consume context without complex prop drilling. This is useful in scenarios where state needs to be shared across components, like user authentication status or theme information. - Application in Component Hierarchies: Context can help manage global state in large component trees, like theme switching, user preferences, or global alerts.
5. Optimizing Performance
- Memoization with
useMemo
: This hook helps optimize performance by memoizing complex calculations or derived state, preventing unnecessary recalculations. - Optimizing Callbacks with
useCallback
: Memoizing functions to avoid re-renders is crucial in high-performance applications. This is often used when passing functions to child components to maintain consistency.
6. Managing Refs and Imperative Methods
- Accessing DOM Elements with
useRef
: TheuseRef
hook provides a way to access DOM elements directly, useful for focus management, animations, or manipulating the DOM. - Creating Imperative APIs with
useImperativeHandle
: This hook allows parent components to call methods on child components, useful for creating controlled components or exposing specific behaviors.
7. Building Complex React Applications
- Combining Multiple Hooks: In complex applications, combining multiple hooks within a single component can provide powerful functionalities while maintaining simplicity.
- Hooks in Component Libraries: Hooks are commonly used in component libraries to encapsulate and share functionality, making it easier to build consistent UI components.
These applications demonstrate the versatility and power of React Hooks in modern React development. Whether managing state, handling side effects, optimizing performance, or building complex applications, React Hooks offer a flexible and efficient approach to building React-based solutions.
Conclusion
Conclusion on React Hooks
React Hooks have transformed the way developers build React applications, bringing a simpler and more flexible approach to managing state, side effects, and other functionalities in functional components. They have become an essential part of modern React development, allowing for a more consistent and efficient code structure.
Summary of Key Points
- Introduction to Hooks: React Hooks enable functional components to use state and other React features without requiring class components.
- Core Hooks: The most commonly used Hooks include
useState
for state management,useEffect
for handling side effects, anduseContext
for consuming context. - Advanced Hooks: Hooks like
useReducer
,useMemo
,useCallback
, anduseRef
allow for more complex state management, memoization, and DOM manipulation. - Custom Hooks: Developers can create custom Hooks to encapsulate common logic and promote code reuse across different components.
- Best Practices: Follow Hook rules, manage state with care, handle side effects properly, and avoid excessive re-renders to ensure efficient React applications.
- Applications: React Hooks are used in a variety of applications, from simple state management to complex performance optimizations, and are crucial in building scalable and maintainable React applications.
Suggestions for Further Learning and Exploration
To deepen your understanding of React Hooks and expand your knowledge, consider exploring the following resources:
- Official React Documentation:
The React documentation provides comprehensive guides on React Hooks, including detailed explanations and examples of various Hooks. - Online Courses and Tutorials:
Platforms like Coursera, Udemy, and Pluralsight offer in-depth courses on React and React Hooks, providing practical examples and hands-on projects. - Books on React and React Hooks:
Books like “Fullstack React” and “Learning React” cover React Hooks in detail, with examples and case studies to demonstrate their usage in real-world scenarios. - Community Resources and Forums:
Engage with the React community through forums like Stack Overflow or Reddit’s r/reactjs. These platforms offer opportunities to ask questions, share experiences, and learn from other developers. - Open Source Projects:
Explore open-source React projects on GitHub to see how experienced developers use React Hooks in large-scale applications. Contributing to these projects can provide valuable learning experiences.
By exploring these resources and staying up-to-date with the latest React developments, you can continue to improve your skills and apply React Hooks effectively in your projects. This ongoing learning will help you build robust, scalable, and maintainable React applications.
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
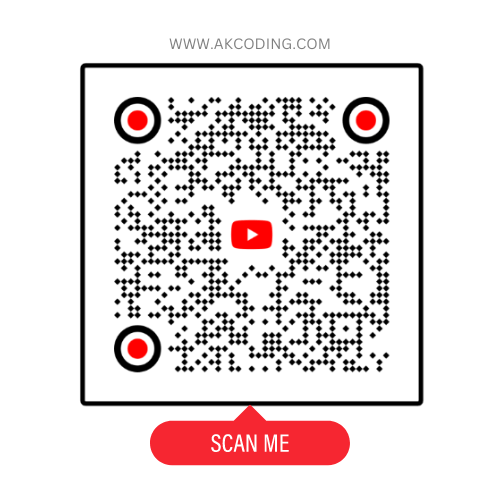