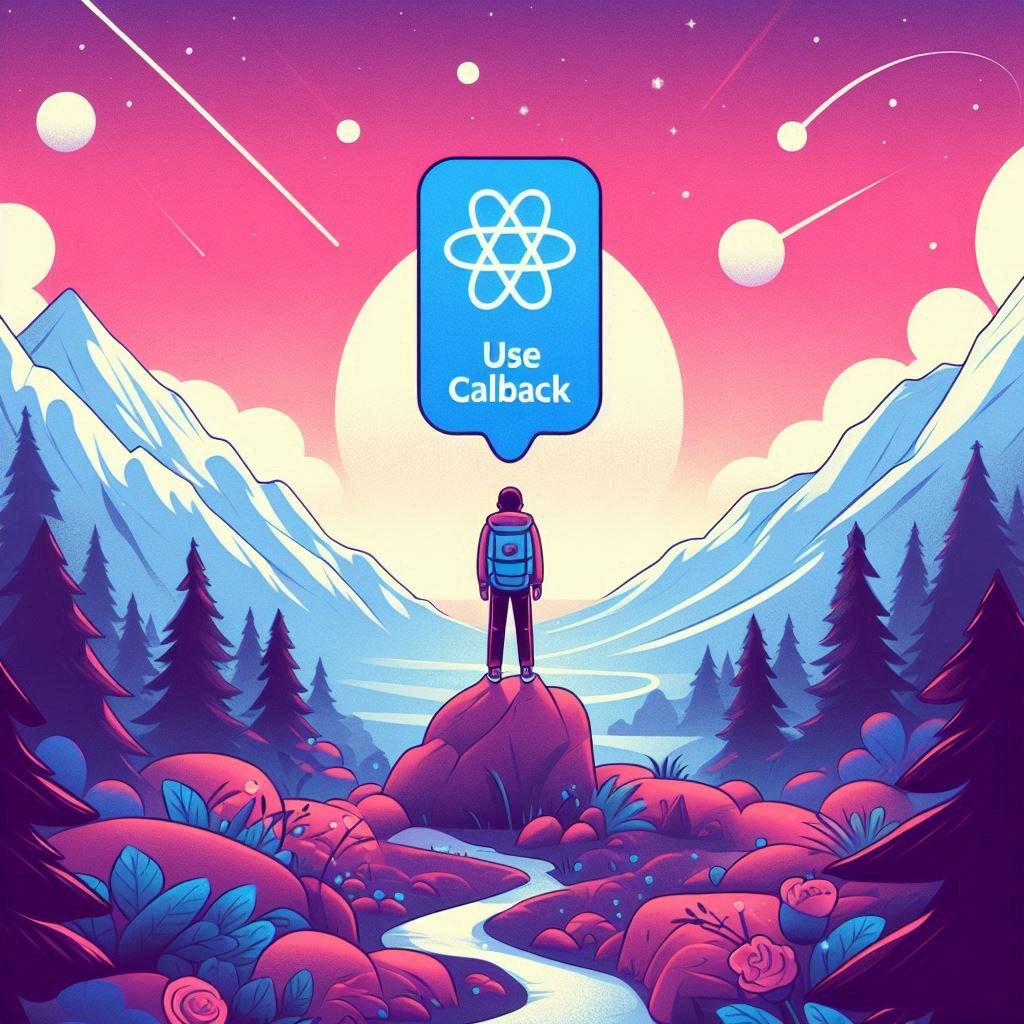
As React applications grow in complexity, optimizing performance becomes crucial. React provides several hooks to help with performance optimization, one of which is useCallback
. This blog post will explain what useCallback
is, how it works, and when you should use it in your React applications.
Table of Contents
What is useCallback
?
It
is a React hook that returns a memoized version of a callback function. This is useful when you pass callbacks to optimized child components that rely on reference equality to prevent unnecessary renders (e.g., components wrapped in React.memo
).
Syntax
It takes two arguments:
- The callback function you want to memoize.
- An array of dependencies that determines when the callback should be recomputed.
Here’s the basic syntax:
const memoizedCallback = useCallback(() => {
doSomething(a, b);
}, [a, b]);
In this example, doSomething
is only recomputed if a
or b
changes.
When to Use It?
You should consider using useCallback
in the following scenarios:
- Passing Callbacks to Child Components: If you pass a callback function to a child component that relies on reference equality (e.g., wrapped in
React.memo
), usinguseCallback
can prevent unnecessary re-renders. - Event Handlers: When defining event handlers inside functional components, using
useCallback
ensures that the same function instance is used unless dependencies change, improving performance.
Example: Optimizing Child Component Renders
Let’s look at an example where useCallback
is used to optimize a component that receives a callback as a prop.
import React, { useState, useCallback } from 'react';
const ChildComponent = React.memo(({ onClick }) => {
console.log('Child component rendered');
return <button onClick={onClick}>Click me</button>;
});
function ParentComponent() {
const [count, setCount] = useState(0);
const handleClick = useCallback(() => {
console.log('Button clicked');
}, []);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
<ChildComponent onClick={handleClick} />
</div>
);
}
export default ParentComponent;
In this example, the ChildComponent
is wrapped with React.memo
to prevent unnecessary renders. By using it
for the handleClick
function, we ensure that the same function instance is passed to ChildComponent
, preventing it from re-rendering when the parent component updates.
Avoiding Overuse
While useCallback
is a powerful tool, overusing it can lead to more complex code without significant performance benefits. It’s essential to measure and understand your application’s performance bottlenecks before applying it
extensively.
Common Pitfalls
- Incorrect Dependencies: Ensure that the dependencies array includes all variables that the callback function uses. Missing dependencies can lead to stale closures and bugs.
- Unnecessary Memoization: Memoizing callbacks that are inexpensive to create or used infrequently can add unnecessary complexity without improving performance.
Debugging useCallback
Issues
- Check Dependencies: Verify that all dependencies are correctly specified.
- Console Logging: Add console logs to understand when the callback function is being recreated.
- Performance Profiling: Use React DevTools and browser performance profiling tools to analyze renders and measure the impact of
useCallback
.
Conclusion
useCallback
is an essential hook for optimizing performance in React applications by memoizing callback functions. It’s particularly useful when passing callbacks to child components that rely on reference equality. However, like all performance optimizations, it should be used judiciously. Measure and understand your application’s performance needs before introducing it
.
Understanding and effectively using it
can lead to more efficient and responsive React applications.
Interview Questions
Here are some interview questions and answers focused on the useCallback
hook in React:
Basic Questions
Q1: What is useCallback
in React?
A1: It
is a React hook that returns a memoized version of a callback function, which only changes if one of its dependencies has changed. This helps to optimize performance by preventing unnecessary re-creations of the function on every render.
Q2: What arguments does useCallback
take?
A2: It
takes two arguments:
- A function that you want to memoize.
- An array of dependencies that determine when the memoized function should be recomputed.
Intermediate Questions
Q3: When should you use useCallback
?
A3: You should use it
when:
- Passing a callback function to a child component that relies on reference equality to prevent unnecessary renders (e.g., a component wrapped in
React.memo
). - Defining event handlers inside functional components to ensure the same function instance is used unless dependencies change.
Q4: How does useCallback
help with performance optimization?
A4: It
helps with performance optimization by ensuring that the same function instance is reused across renders unless its dependencies change. This prevents unnecessary re-renders of child components that rely on the callback, reducing the overall rendering cost.
Advanced Questions
Q5: Can you provide an example where useCallback
is used to prevent unnecessary re-renders of a child component?
A5:
import React, { useState, useCallback } from 'react';
const ChildComponent = React.memo(({ onClick }) => {
console.log('Child component rendered');
return <button onClick={onClick}>Click me</button>;
});
function ParentComponent() {
const [count, setCount] = useState(0);
const handleClick = useCallback(() => {
console.log('Button clicked');
}, []);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
<ChildComponent onClick={handleClick} />
</div>
);
}
export default ParentComponent;
In this example, useCallback
ensures that handleClick
has the same reference across renders, preventing ChildComponent
from re-rendering unnecessarily.
Q6: How does useCallback
differ from useMemo
?
A6: useCallback
memoizes a function, while useMemo
memoizes the result of a function. useCallback
returns a cached function reference, whereas useMemo
returns a cached value. Both hooks take a dependencies array to determine when to recompute.
Q7: What are some potential pitfalls when using useCallback
?
A7:
- Incorrect Dependencies: Failing to include all necessary dependencies in the array can lead to stale closures and bugs.
- Unnecessary Complexity: Overusing
useCallback
for functions that are inexpensive to recreate or not frequently used can add unnecessary complexity without providing significant performance benefits.
Q8: How would you debug issues related to useCallback
?
A8:
- Check Dependencies: Ensure the dependencies array includes all variables used within the callback function.
- Console Logging: Add console logs to check when the callback function is being recreated.
- Performance Profiling: Use React DevTools and browser performance profiling tools to analyze renders and measure the impact of
useCallback
.
Q9: Can useCallback
be used to memoize asynchronous functions?
A9: Yes, useCallback
can be used to memoize asynchronous functions. However, it’s important to ensure that the dependencies array correctly includes all dependencies to avoid stale closures.
Q10: Explain a scenario where useCallback
does not provide a performance benefit.
A10: It
does not provide a performance benefit if the function being memoized is inexpensive to create or if the component re-renders infrequently. In such cases, the overhead of memoizing the function can outweigh the benefits, leading to unnecessary complexity without significant performance gains.
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
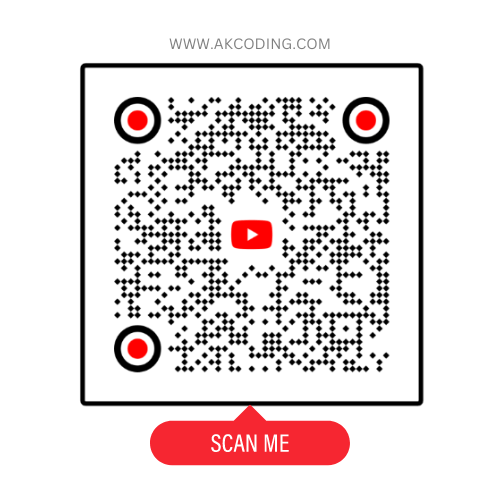