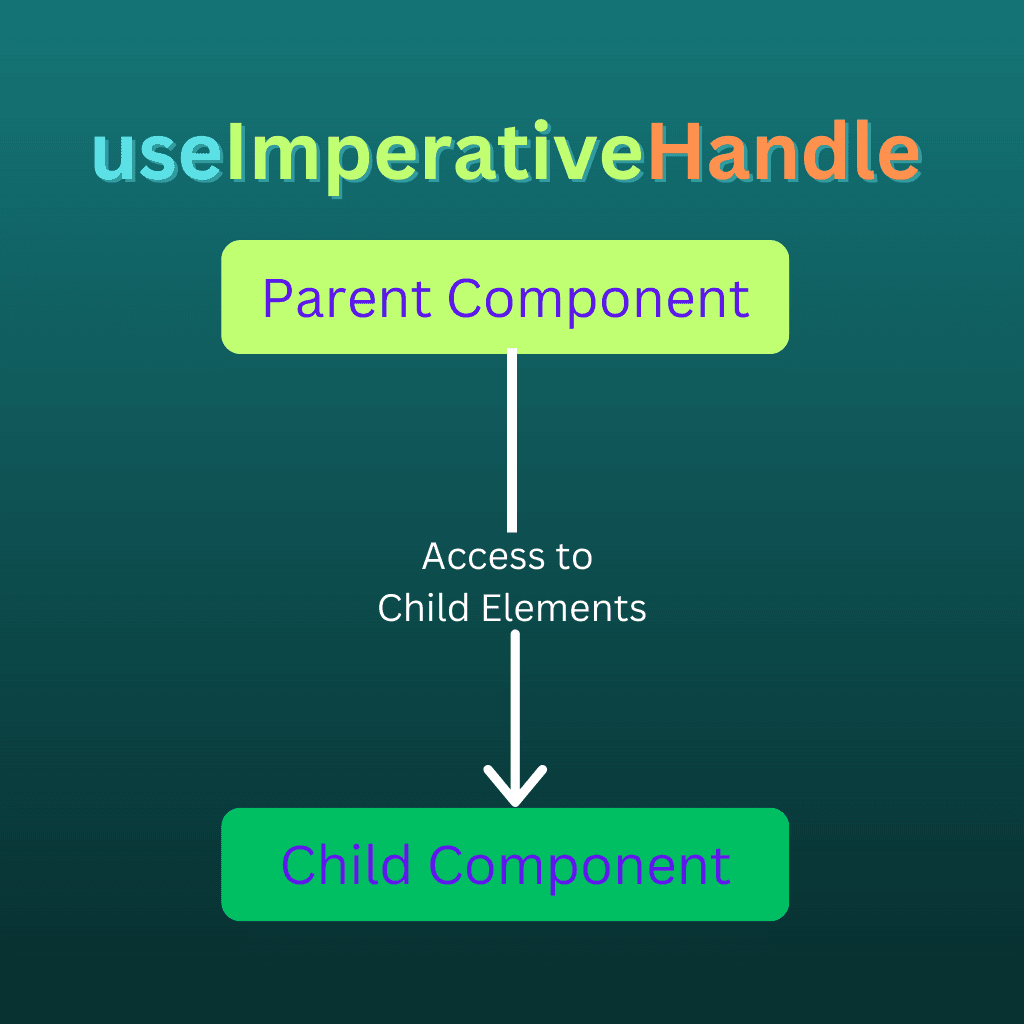
React is known for its clean, declarative style of programming that encourages developers to use props and state to handle data flow and updates. However, there are moments when you need finer control, especially when working with refs to interact with DOM elements or child components. In such cases, the useImperativeHandle
hook comes to the rescue.
We’ll dive deep into what useImperativeHandle
is, how it works, when you should use it, and how to apply it in real-world use cases.
Table of Contents
What is useImperativeHandle
?
useImperativeHandle
is a React hook that allows you to customize the instance value that is exposed to parent components when using ref
. Typically, when a parent component passes a ref
to a child component, it receives access to the child’s DOM element or component instance. However, there may be cases where the child component needs to control what is exposed to the parent. That’s where useImperativeHandle
comes into play.
Syntax
useImperativeHandle(ref, createHandle, [dependencies])
ref
: This is the reference passed from the parent component.createHandle
: A function that returns the value that will be exposed to the parent.[dependencies]
: An optional array of dependencies that re-runscreateHandle
if any of the dependencies change.
Why Do We Need It?
By default, a ref gives access to a child component’s internal DOM node or instance. While this is sufficient for most use cases, there are instances where you want to expose only specific methods or properties from the child. The useImperativeHandle
hook allows you to customize this behavior, which adds a layer of abstraction and control over what’s exposed to the parent.
For example, if you’re building a reusable component like a modal or a custom input field, you may want the parent component to trigger certain actions (like opening or closing the modal) without giving direct access to the entire component.
When Should You Use useImperativeHandle
?
useImperativeHandle
is particularly useful in the following scenarios:
- Customizing Access to Child Elements: If the child component has several internal methods or states, and you want to expose only a subset of them to the parent component.
- Interacting with DOM Elements: When you need to access or manipulate DOM elements (such as focusing an input field), you can limit what the parent can control.
- Reusable Component Libraries: If you’re building a component that will be used by others, limiting the exposed functionality makes the component easier to maintain and more predictable.
- Avoiding Unnecessary Re-Renders: By using
useImperativeHandle
with dependency arrays, you can prevent unnecessary recalculations or re-renders in child components, thereby improving performance.
A Simple Example: Custom Input Field with Focus
Let’s start with a basic example. Imagine you’re building a custom input field, and you want the parent component to be able to control the focus of that input. Instead of exposing the entire input element via ref
, we’ll use useImperativeHandle
to only expose a focus
method.
Step-by-Step Example
import React, { useRef, useImperativeHandle, forwardRef } from 'react';
// Child Component: CustomInput
const CustomInput = forwardRef((props, ref) => {
const inputRef = useRef();
useImperativeHandle(ref, () => ({
focus: () => {
inputRef.current.focus();
},
}));
return <input ref={inputRef} {...props} />;
});
// Parent Component
const ParentComponent = () => {
const inputRef = useRef();
return (
<div>
<CustomInput ref={inputRef} placeholder="Type something..." />
<button onClick={() => inputRef.current.focus()}>Focus Input</button>
</div>
);
};
export default ParentComponent;
Breakdown of the Example:
CustomInput
: This child component usesforwardRef
to pass the ref from the parent down to the actual input element. Inside the component,useImperativeHandle
is used to expose a single method (focus
), which focuses the input element when called.ParentComponent
: The parent component can now call thefocus
method on the input field via theinputRef
without having access to the entire DOM element.
This pattern is particularly useful because it allows the parent to perform specific actions without knowing or caring about the internal implementation details of the child.
Forwarding Refs with forwardRef
Before you can use useImperativeHandle
, you need to make sure that the ref can be passed down to the child component. By default, ref forwarding doesn’t work with functional components. That’s where forwardRef
comes in—it enables a functional component to accept refs from its parent.
const CustomInput = forwardRef((props, ref) => {
// ref can now be used within the component
});
This makes forwardRef
and useImperativeHandle
a powerful combination, allowing you to build components with controlled access to internal behavior.
Real-World Use Cases
Let’s explore a couple of real-world scenarios where useImperativeHandle
shines:
1. Controlling a Modal Component
Imagine you have a modal component that the parent needs to control (open or close). Instead of exposing the entire modal element, you can expose specific methods like openModal
and closeModal
.
const Modal = forwardRef((props, ref) => {
const [isOpen, setIsOpen] = React.useState(false);
useImperativeHandle(ref, () => ({
openModal: () => setIsOpen(true),
closeModal: () => setIsOpen(false),
}));
return isOpen ? <div className="modal">This is a modal!</div> : null;
});
Now, the parent can open or close the modal without interacting with its internal logic or state.
2. Customizing a Video Player
In a video player component, you may want the parent component to be able to control actions like play, pause, or reset without giving access to the entire DOM element.
const VideoPlayer = forwardRef((props, ref) => {
const videoRef = useRef();
useImperativeHandle(ref, () => ({
play: () => videoRef.current.play(),
pause: () => videoRef.current.pause(),
}));
return <video ref={videoRef} {...props} />;
});
Best Practices
While useImperativeHandle
is a powerful tool, it’s important to use it sparingly. Here are some best practices to follow:
- Use only when necessary: Most of the time, you won’t need
useImperativeHandle
. React’s declarative approach with props and state covers many cases, so only use this hook when you need precise control. - Limit what’s exposed: Be cautious about what you expose to parent components. Only reveal methods that are necessary to avoid breaking encapsulation.
- Handle performance concerns: If the
createHandle
function is computationally expensive, ensure that you pass a dependencies array to avoid re-creating the handle unnecessarily.
Conclusion
The useImperativeHandle
hook is an advanced tool in the React ecosystem that provides granular control over component behavior and interactions. It’s particularly helpful when building reusable components or handling complex DOM manipulations. By using it thoughtfully and combining it with forwardRef
, you can create powerful, flexible, and maintainable components.
While it might seem a bit tricky at first, with practice, you’ll find useImperativeHandle
to be an indispensable part of your React toolkit. Happy coding!
Interview Questions
Here are the top 10 interview questions on the useImperativeHandle
hook in React:
1. What is useImperativeHandle
in React, and why is it used?
- Answer:
It
is a React hook that allows you to customize the ref instance exposed to the parent component. It is primarily used when you need to control or restrict access to certain parts of a child component’s DOM or methods from the parent.
2. How does useImperativeHandle
differ from regular ref
forwarding in React?
- Answer: Normally,
ref
gives access to the child component’s instance or DOM element.
allows you to control what the parent can access by customizing the exposed instance, giving more flexibility and control over the child component.It
3. Explain the syntax of the useImperativeHandle
hook.
- Answer: The syntax is:
useImperativeHandle(ref, createHandle, [dependencies]);
ref
: A ref object forwarded from the parent.createHandle
: A function that returns an object containing the instance or methods you want to expose.[dependencies]
: Optional dependencies array that triggers re-creation of the handle when values change.
4. When should you use useImperativeHandle
in React applications?
- Answer: You should use
It
- You want to expose only specific methods or properties from a child component to the parent.
- You need fine control over interactions with child DOM elements (e.g., focus, scroll).
- You’re building reusable components and want to limit external access to certain internal behaviors.
5. What are the key use cases for useImperativeHandle
in React?
- Answer: Key use cases include:
- Controlling custom input fields or form components (e.g., focus, validation).
- Managing modal open/close behavior from the parent.
- Exposing specific controls in custom UI components like video players, sliders, or animations.
6. What is the role of forwardRef
when using useImperativeHandle
?
- Answer:
forwardRef
is used to forward theref
from the parent to the child component. WithoutforwardRef
,useImperativeHandle
cannot work because functional components don’t automatically acceptref
props like class components.
7. Can you explain a simple use case where useImperativeHandle
is used to expose methods from a child component?
- Answer: Example: A custom input component where the parent can control the focus.
javascript const CustomInput = forwardRef((props, ref) => { const inputRef = useRef(); useImperativeHandle(ref, () => ({ focus: () => inputRef.current.focus(), })); return <input ref={inputRef} {...props} />; });
In this case, only thefocus
method is exposed to the parent.
8. What are some common mistakes developers make when using useImperativeHandle
?
- Answer: Common mistakes include:
- Not using
forwardRef
when needed. - Exposing too much information to the parent, breaking the component’s encapsulation.
- Forgetting to include a dependencies array in performance-critical components.
- Overusing it in scenarios where regular refs or props would suffice.
- Not using
9. How does useImperativeHandle
improve component encapsulation and reusability?
- Answer: By using
useImperativeHandle
, you can expose only specific methods or properties to the parent, keeping the child’s internal logic hidden. This enhances encapsulation, making the component more reusable and preventing the parent from directly manipulating internal states or DOM elements.
10. Can useImperativeHandle
cause performance issues? If so, how can you mitigate them?
- Answer: If the
createHandle
function is complex and frequently re-executes without a proper dependencies array, it can lead to performance issues. To mitigate this, always provide a dependencies array to prevent unnecessary re-creation of the handle unless the dependencies change.
These questions are designed to test a candidate’s understanding of the useImperativeHandle
hook, its use cases, and best practices in real-world applications.
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
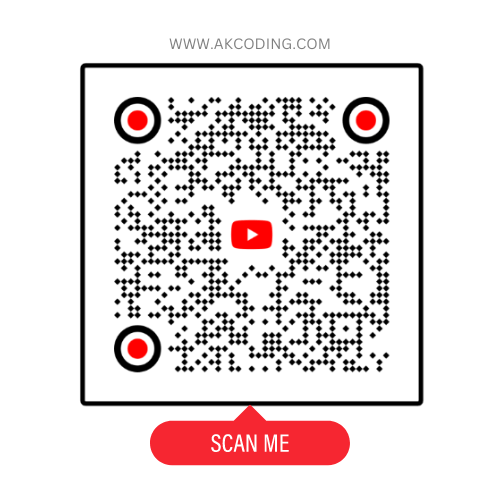