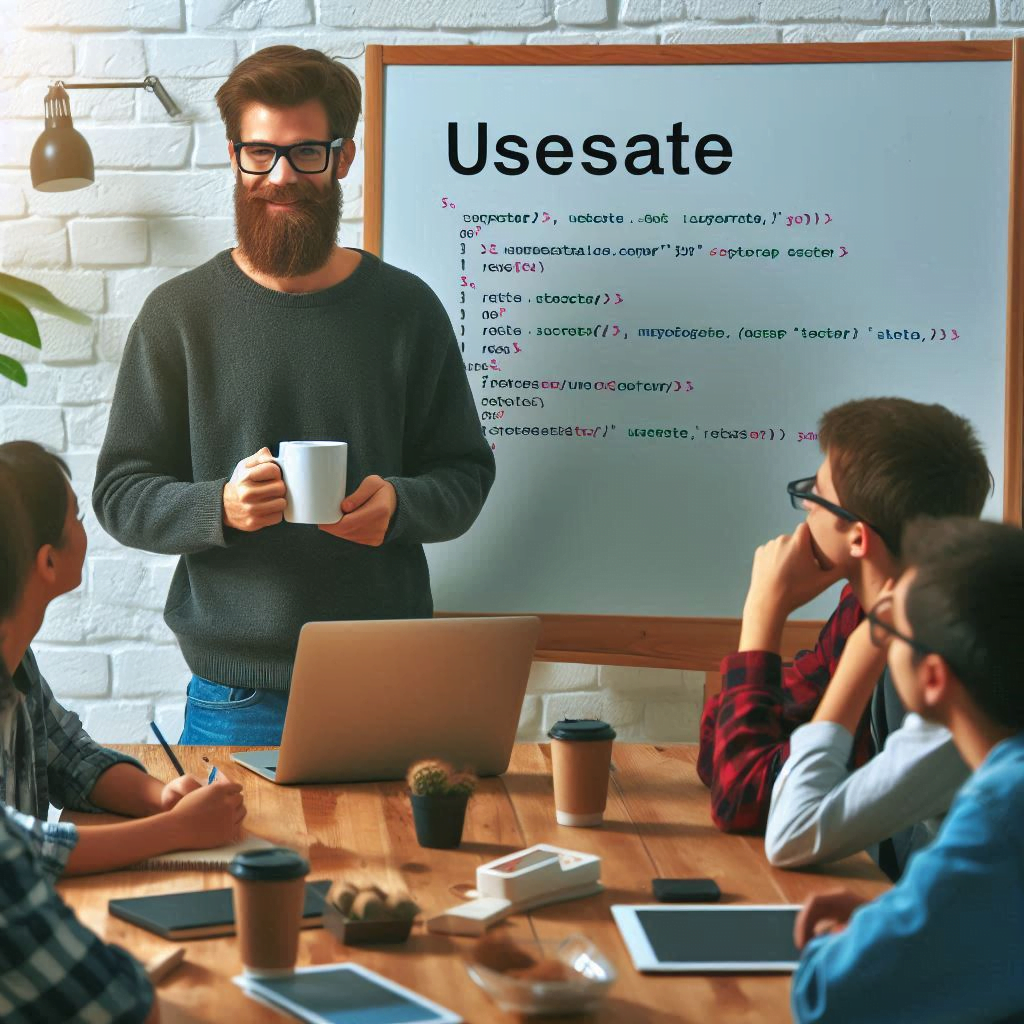
Introduction to useState
in React
React, a popular JavaScript library for building user interfaces, introduced hooks in version 16.8. Hooks allow developers to use state and other React features in functional components. Among the various hooks available, useState
is one of the most fundamental and commonly used. In this blog post, we’ll explore what useState
is, how it works, and provide examples to help you understand its usage.
Table of Contents
What is useState
in react?
useState
is a hook that allows you to add state to your functional components. State is an essential aspect of any React application, enabling components to manage and respond to user input, server requests, and other dynamic interactions.
Syntax and Basic Usage
To use useState
, you need to import it from the react
package. The useState
hook returns an array containing two elements: the current state value and a function to update that value. Here’s the basic syntax:
import React, { useState } from 'react';
function ExampleComponent() {
// Declare a state variable 'count' and a function 'setCount' to update it
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
In this example, useState(0)
initializes the state variable count
with a value of 0
. The setCount
function is used to update the state whenever the button is clicked.
Updating State
Updating state with useState
is straightforward. You call the updater function with the new state value. React will then re-render the component to reflect the updated state. Here’s an example of a component that tracks user input:
import React, { useState } from 'react';
function InputComponent() {
const [text, setText] = useState('');
return (
<div>
<input
type="text"
value={text}
onChange={(e) => setText(e.target.value)}
/>
<p>You typed: {text}</p>
</div>
);
}
In this case, useState('')
initializes the text
state with an empty string. The input field’s onChange
event updates the state with the user’s input.
Using Multiple useState
Hooks
You can use multiple useState
hooks to manage different pieces of state within the same component. This approach keeps your state management organized and readable. For example:
import React, { useState } from 'react';
function MultiStateComponent() {
const [name, setName] = useState('');
const [age, setAge] = useState(0);
return (
<div>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
placeholder="Name"
/>
<input
type="number"
value={age}
onChange={(e) => setAge(e.target.value)}
placeholder="Age"
/>
<p>
Name: {name}, Age: {age}
</p>
</div>
);
}
Here, two useState
hooks manage the name
and age
state variables independently.
Initial State with Functions
When the initial state is expensive to compute, you can pass a function to useState
. This function will only run during the initial render:
import React, { useState } from 'react';
function ExpensiveInitialStateComponent() {
const [data, setData] = useState(() => {
// Expensive computation here
return computeInitialState();
});
return <div>{data}</div>;
}
By using a function, you ensure that the expensive computation only happens once, during the initial render, rather than on every render.
Conclusion
The useState
hook is a powerful and essential tool in React for managing state within functional components. It simplifies state management, making your components more readable and maintainable. By understanding and effectively using useState
, you can build dynamic and interactive user interfaces with ease.
Experiment with useState
in your projects and explore its versatility. Whether you’re handling simple counters, complex forms, or dynamic data fetching, useState
provides the foundation for managing state in your React applications.
useState
Interview Questions
The useState
hook is one of the most fundamental hooks in React, enabling functional components to manage local state. When preparing for a React interview, understanding useState
and its nuances is crucial. Here are some top interview questions that you might encounter:
Basic Questions
- What is
useState
in React?
useState
is a hook that allows you to add state to functional components. It returns a state variable and a function to update that state.
- How do you declare a state variable using
useState
?
- Example:
javascript const [count, setCount] = useState(0);
- What arguments does
useState
accept, and what does it return?
useState
accepts the initial state as an argument and returns an array with two elements: the current state and a function to update it.
Intermediate Questions
- How can you update the state using
useState
?
- By calling the setter function returned by
useState
. Example:javascript setCount(count + 1);
- What happens when you call the setter function from
useState
?
- React schedules a re-render of the component, and during this render, the state variable will hold the new value.
- Can you pass a function to the setter function in
useState
? If so, why?
- Yes, you can pass a function to the setter function to ensure you get the most up-to-date state. Example:
javascript setCount(prevCount => prevCount + 1);
Advanced Questions
- What is lazy initialization in
useState
?
- Lazy initialization allows you to pass a function to
useState
to compute the initial state only once, avoiding the computation on every render. - Example:
javascript const [count, setCount] = useState(() => computeExpensiveValue());
- How does
useState
behave with objects or arrays as state variables?
useState
does not perform a deep merge of objects. To update nested state, you must manually merge the changes.- Example:
javascript const [user, setUser] = useState({ name: 'John', age: 30 }); setUser(prevUser => ({ ...prevUser, age: 31 }));
- Can you explain the difference between
useState
and class component state?
useState
is used in functional components, whilethis.state
andthis.setState
are used in class components.useState
allows for more readable and concise state management.
- How do you handle multiple state variables using
useState
?- You can call
useState
multiple times to manage different pieces of state. - Example:
javascript const [name, setName] = useState(''); const [age, setAge] = useState(0);
- You can call
Practical Questions
- What are some common pitfalls when using
useState
?- Forgetting to use a function to update state when the new state depends on the previous state.
- Trying to directly mutate state instead of using the setter function.
- Not initializing state properly, leading to unexpected
undefined
values.
- How would you implement a counter component using
useState
?- import React, { useState } from ‘react’;
- function Counter() {
- const [count, setCount] = useState(0);
- return (
- You clicked {count} times setCount(count + 1)}>Click me
- );
- }
- export default Counter;
- How can you debug issues related to
useState
?- Use
console.log
to track state changes. - Use React Developer Tools to inspect component state.
- Ensure the setter function is called correctly and check for asynchronous issues.
- Use
- Explain a scenario where using
useState
might not be the best choice.- For complex state logic, using
useReducer
might be more appropriate, as it provides a more structured approach to managing state transitions.
- For complex state logic, using
- How do you handle state updates that are triggered by external factors like a WebSocket or API response?
- Ensure the state updates are handled inside an effect (
useEffect
) to capture side effects properly.
- Ensure the state updates are handled inside an effect (
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
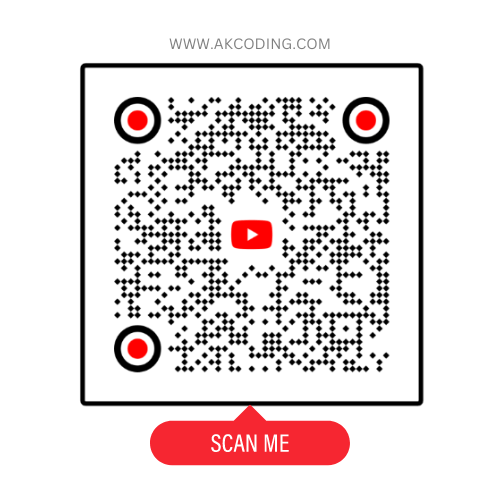