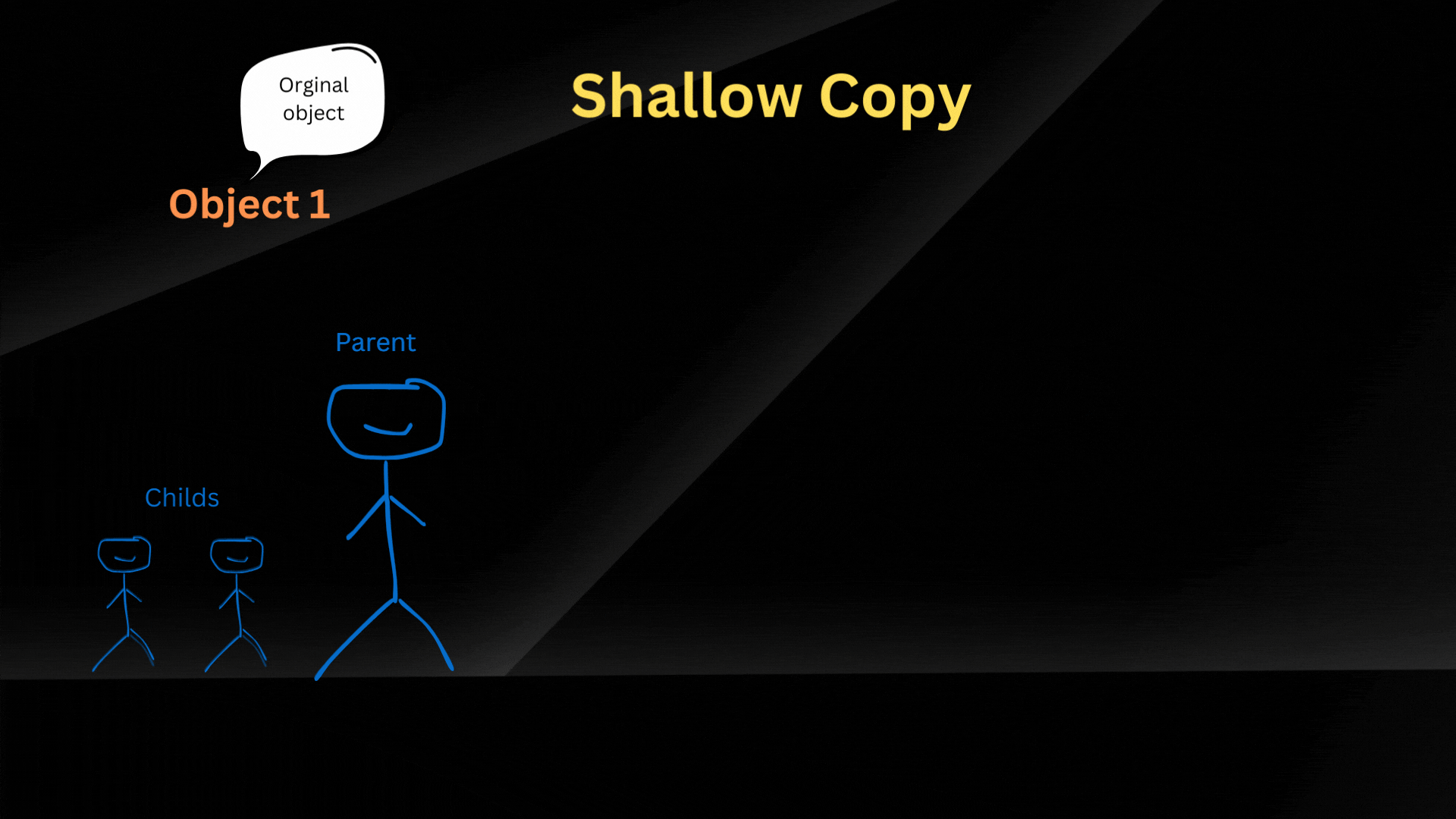
Table of Contents
Shallow Copy vs Deep Copy
Understanding the Shallow Copy vs Deep Copy is crucial for developers working with JavaScript objects and arrays. The distinction impacts how data is copied, manipulated, and managed in various scenarios. In this guide, we’ll explore the definitions of shallow copy and deep copy, examine real-world use cases, and discuss when to use each method. Whether you’re building complex applications or simply need to clone an object, knowing the nuances of these copying techniques can save you from unexpected bugs and ensure your code behaves as intended. Let’s dive into the key concepts and learn how to apply them in your projects.
What is a Shallow Copy?
A shallow copy is a method of duplicating an object in a way that creates a new object with the same immediate properties as the original, but without recursively copying nested objects or arrays. Instead, a shallow copy references any nested objects or arrays from the original object. This approach can be useful for duplicating simple data structures with primitive values, but it carries some risks when dealing with complex or nested structures. Here’s a more detailed explanation:
How It Works
In a shallow copy, properties that are primitive data types—such as strings, numbers, and booleans—are copied by value. This means that changes to these primitive values in the original object won’t affect the shallow copy. However, if a property is an object or an array, it is copied by reference. This means that changes to the nested object in the original will also reflect in the shallow copy, and vice versa.
Methods for Shallow Copy in JavaScript
Object.assign()
: Copies the properties of one or more source objects into a target object. It creates a new object but does not recursively copy nested structures.- Spread Operator (
...
): When used to copy objects or arrays, it creates a new object but retains references for nested objects or arrays. - Array Methods like
slice()
: Creates a new array with the same elements as the original, but if any elements are objects or arrays, they share references.
Potential Risks and Limitations
Because shallow copy references nested structures, any modification to a nested object or array in one copy can lead to unintended changes in another. This can cause bugs or unexpected behavior when the intention was to create an independent copy.
Common Use Cases
- Shallow copy is suitable when you only need to copy an object’s immediate properties or when the object contains only primitive data types.
- It’s often used for quick duplication tasks where shared references are acceptable or intended.
Shallow copy is a fast and efficient method for duplicating simple data structures, but it requires careful handling when dealing with nested or complex objects to avoid unintended side effects. If the goal is to create a completely independent copy of an object, a deep copy might be more appropriate.
What is a Deep Copy?
A deep copy in JavaScript refers to a copying process that creates a completely independent duplicate of an object, including all its nested objects and arrays. Unlike a shallow copy, which merely copies the references to nested structures, a deep copy replicates every level of the object, ensuring that the new copy doesn’t share any references with the original object. This means that changes made to the deep copy do not affect the original object, and vice versa.
How Deep Copy Works
In a deep copy, every property of the original object is copied recursively. If a property is an object or an array, the deep copy process creates a new instance of that object or array and copies its properties or elements, rather than copying the reference. This recursive approach ensures complete independence between the original object and its deep copy.
Methods for Deep Copy in JavaScript
Deep copying requires more complex methods due to its recursive nature. Some common approaches include:
- Recursive Custom Functions: Manually writing a function that iterates through an object’s properties, creating new instances of nested objects and arrays. This approach gives you full control but can be prone to errors.
JSON.parse(JSON.stringify(object))
: This creates a deep copy by converting the object into a JSON string and then parsing it back into an object. It works for simple structures but has limitations, such as losing functions, circular references, and non-JSON-friendly data types likeundefined
orDate
.- Libraries like
lodash
‘s_.cloneDeep()
:Lodash
provides a reliable_.cloneDeep()
method for deep copying that handles various edge cases, including complex nested structures and circular references.
Advantages of Deep Copy
- Independence: Deep copies ensure complete isolation between the original and the copy, allowing you to modify one without affecting the other.
- Reliability: Deep copy is ideal for cases where changes to nested structures should not impact other copies or the original object.
Common Use Cases
Deep copy is essential in scenarios where you need to ensure data integrity and avoid unexpected behavior due to shared references. Some common use cases include:
- Complex Data Structures: When working with deeply nested objects or arrays, deep copying ensures that each level is independently copied.
- State Management: In frameworks like React or Redux, deep copy helps avoid state mutation issues by ensuring that updates to one part of the state do not affect other parts unintentionally.
- Data Duplication: When creating backups or temporary copies for testing or processing, deep copy helps maintain the original data’s integrity.
Deep copy is a powerful tool for creating independent object duplicates, especially in complex scenarios with nested structures. However, due to its recursive nature, deep copying can be computationally expensive and requires careful consideration in terms of performance. When using deep copy, ensure that the method chosen suits your needs and doesn’t introduce unexpected behavior due to edge cases.
When to Use Shallow Copy?
Scenarios:
- When the object contains only primitive data types.
- When reference sharing is acceptable or intended.
- For simple cloning operations where deep copying isn’t necessary.
Examples:
- Cloning configurations with only primitive data.
- Copying small objects with known references.
When to Use Deep Copy?
Scenarios:
- When objects contain nested objects or arrays.
- When you want complete independence between copies.
- When working with complex data structures that shouldn’t share references.
Examples:
- Deep cloning a complex object structure.
- Copying state in a React or Redux context to avoid mutating the original state.
Performance Considerations
Shallow Copy:
- Generally faster due to limited data copying.
- Ideal for lightweight operations.
Deep Copy:
- Slower due to recursive copying of nested objects.
- Can be computationally expensive for large or deeply nested objects.
Best Practices for Copying in JavaScript
Copying in JavaScript can involve shallow copy or deep copy, depending on the complexity of the data structure and your intent. To avoid unexpected behavior, performance bottlenecks, or memory leaks, it’s crucial to follow best practices. Here are some key guidelines to consider when copying objects and arrays in JavaScript:
1. Understand the Difference Between Shallow Copy and Deep Copy
- Shallow Copy: Creates a new object with the same properties as the original, but references nested objects. It is suitable for simple structures with primitive values.
- Deep Copy: Creates a new object that recursively copies all nested objects, ensuring complete independence from the original.
2. Choose the Appropriate Copying Method
- Shallow Copy Techniques:
Object.assign()
for copying properties from one object to another.- Spread operator
...
for duplicating objects and arrays. Array.slice()
for creating a shallow copy of an array.- Deep Copy Techniques:
- Custom recursive functions for complex copying scenarios.
JSON.parse(JSON.stringify(object))
for simple deep copies (with limitations).- Libraries like
lodash
with_.cloneDeep()
for reliable deep copying.
3. Avoid Unintended Side Effects
- Be Aware of Shared References: In a shallow copy, changes to nested objects or arrays affect both the original and the copy. Consider using deep copy if shared references could lead to bugs or unexpected behavior.
- Use Deep Copy When Needed: If you need complete independence between objects, choose deep copy to prevent side effects.
4. Consider Performance and Resource Usage
- Shallow Copy Is Faster: It is generally faster because it doesn’t recursively copy nested structures. Use it when performance is critical and references are acceptable.
- Deep Copy Is Resource-Intensive: Deep copying can be slow, especially with large or deeply nested objects. Use it when data integrity is more important than performance.
5. Test and Validate Copying Scenarios
- Write Unit Tests: To ensure that your copying logic works as expected, write tests to validate both shallow and deep copies.
- Check for Edge Cases: Test for scenarios with circular references, complex nested objects, and non-standard data types (like functions or
undefined
).
6. Use Libraries for Complex Scenarios
- Leverage Established Libraries: For deep copying, consider using well-known libraries like
lodash
. They handle many edge cases and reduce the risk of errors. - Stay Updated on Best Practices: Libraries often evolve, so stay informed about updates, new features, and best practices for using them.
7. Document Your Code
- Explain the Copying Strategy: In your code comments or documentation, clarify whether you’re using shallow or deep copy and why.
- Provide Context: Explain why a particular copying method was chosen to help others understand the rationale and maintain the code more easily.
By following these best practices, you can ensure that your copying operations in JavaScript are reliable, maintainable, and less prone to errors. Whether you’re managing state in a React application, duplicating data for processing, or simply creating object copies, these guidelines will help you make the right choices.
Conclusion
Understanding the differences between shallow copy and deep copy in JavaScript is vital for maintaining control over your code’s behavior and avoiding unexpected issues. Shallow copy is a useful technique for duplicating simple objects with primitive properties or when you intend to share references to nested structures. However, it can lead to unintended side effects if you’re not careful about modifying shared references.
Deep copy, on the other hand, provides a robust solution for creating entirely independent copies of complex objects, ensuring that changes to the copy don’t affect the original data. While deep copying is more resource-intensive, it’s often necessary for preserving data integrity in large applications or when dealing with state management in frameworks like React.
As a best practice, always consider the context and requirements of your project before deciding between shallow and deep copy. For small-scale cloning or performance-sensitive scenarios, shallow copy is often sufficient. For cases where you need complete isolation between copies, deep copy is the way to go.
In conclusion, by understanding these concepts, you can better manage your data, prevent unexpected behavior, and write more reliable JavaScript code. Don’t hesitate to use libraries and tools that offer efficient deep copying, and always test your code to ensure the desired behavior.
With this knowledge, you’re now equipped to make informed decisions about copying objects in JavaScript and avoid common pitfalls. Happy coding!
Additional Resources
To deepen your understanding of Shallow Copy vs Deep Copy in JavaScript, explore the following resources. These links include detailed documentation, helpful articles, and popular libraries that offer additional tools for copying objects in various contexts.
- MDN Web Docs – Object.assign()
Mozilla Developer Network (MDN) provides an in-depth explanation of theObject.assign()
method, commonly used for shallow copying. - MDN Web Docs – Spread Operator
Mozilla Developer Network (MDN) also offers a comprehensive guide to the spread operator (...
), illustrating how it can be used for shallow copying and other scenarios. - Lodash – _.cloneDeep()
Lodash is a popular utility library in JavaScript, and its_.cloneDeep()
function is a widely used method for deep copying. The official documentation provides examples and additional utilities to facilitate deep copying in your projects. - JavaScript.info – Objects and References
JavaScript.info has a detailed article on object references, illustrating how references work in JavaScript and the impact on copying objects. - React Docs – State and Lifecycle
If you work with React, the React documentation is an excellent resource for understanding state management and how copying mechanisms play a role in React components and state management strategies. - Deep Copy vs Shallow Copy – A Comprehensive Comparison
This freeCodeCamp article provides a comprehensive comparison between shallow copy and deep copy with practical examples, helping you understand the intricacies of each approach.
These resources offer a wealth of information to help you master shallow and deep copying in JavaScript. By exploring them, you can gain further insights, learn new techniques, and enhance your skills in managing JavaScript objects.
Shallow Copy vs Deep Copy comparison in tabular format
Here’s a comparison of Shallow Copy vs Deep Copy in tabular format:
Aspect | Shallow Copy | Deep Copy |
---|---|---|
Definition | Creates a new object with the same properties as the original, but references nested objects. | Creates a new object that recursively copies all nested objects, ensuring complete independence from the original. |
Copying Mechanism | Copies only the immediate properties of the original object. | Recursively copies all levels of nested objects or arrays. |
Independence | Nested objects or arrays share references between original and copy. | Nested objects or arrays are completely independent from the original. |
Performance | Generally faster because it doesn’t recursively copy nested structures. | Can be slower, especially with large or deeply nested objects. |
Use Cases | Suitable for simple structures with primitive values or when shared references are acceptable. | Ideal for scenarios where data integrity is crucial and complete independence is required. |
Methods | – Object.assign() – Spread operator ... – Array.slice() | – Custom recursive functions – JSON.parse(JSON.stringify(object)) – Libraries like lodash ‘s _.cloneDeep() |
Side Effects | Changes to nested objects affect both original and copy. | Changes to nested objects in one copy do not affect others. |
Memory Management | Requires less memory because it shares references. | Can consume more memory due to creating independent copies of nested structures. |
Complexity Handling | Suitable for simple data structures with shallow nesting. | Handles complex nested structures with deep nesting. |
Performance Consideration | Better for performance-sensitive scenarios with large datasets. | Better for ensuring data integrity and preventing side effects. |
Example | javascript<br>const shallowCopy = Object.assign({}, originalObject);<br> | javascript<br>const deepCopy = JSON.parse(JSON.stringify(originalObject));<br> |
This comparison of “Shallow Copy vs Deep Copy” provides a concise overview of the differences between shallow copy and deep copy, helping you choose the appropriate copying mechanism based on your specific requirements and use cases.
FAQs
Here are the top 5 FAQs of Shallow Copy vs Deep Copy :
- What is the difference between shallow copy and deep copy in JavaScript?
- A shallow copy creates a new object and inserts references to the original objects into it. In contrast, a deep copy creates a completely new object with its own copies of the data, independent of the original object.
- When should I use a shallow copy instead of a deep copy?
- Use a shallow copy when you only need to copy the structure of an object or array and are okay with the inner objects or arrays being shared with the original. This is common in situations where immutability isn’t a concern, or performance is critical.
- How can I create a shallow copy of an object or array in JavaScript?
- For arrays, you can use methods like
Array.prototype.slice()
or the spread operator[...array]
. For objects, you can useObject.assign()
or the spread operator{...object}
.
- What are some common methods for creating deep copies in JavaScript?
- A common method is using
JSON.parse(JSON.stringify(object))
, but this has limitations (e.g., it can’t handle functions,undefined
, or circular references). For more complex scenarios, libraries like Lodash’s_.cloneDeep()
are used to perform deep copying.
- What are the potential pitfalls of using shallow copy in JavaScript?
- The main pitfall is that changes to the nested objects in the copied object will affect the original object, leading to unintended side effects. This can cause bugs in situations where independent modifications to the original and copied objects are expected.
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
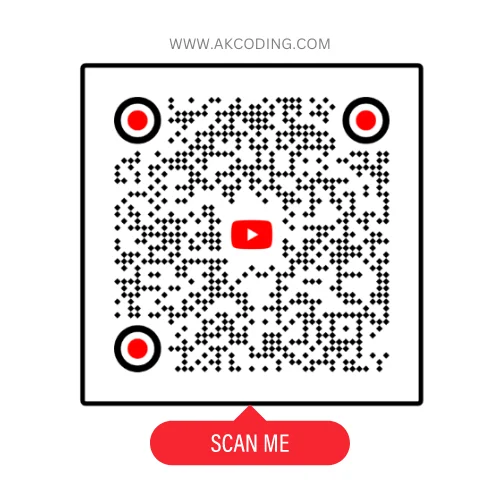