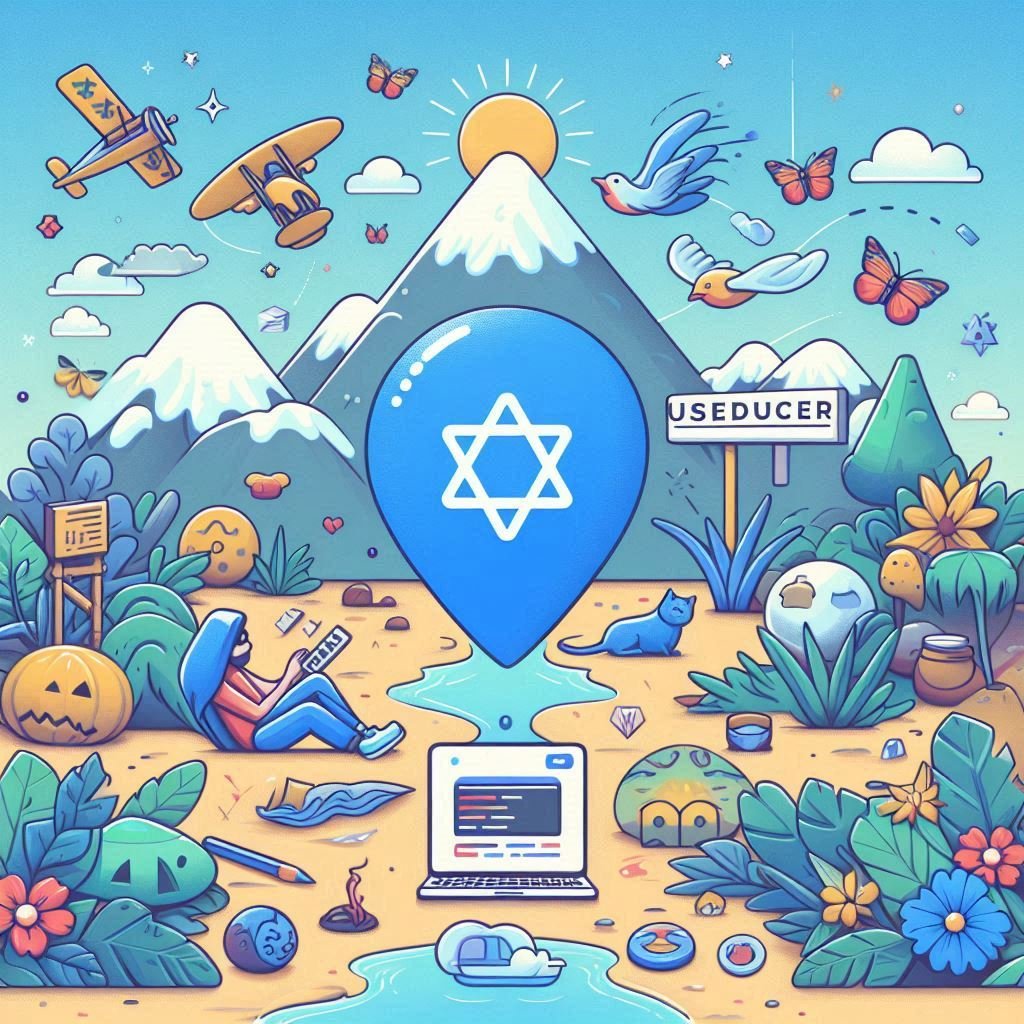
As a React developer, managing complex state logic within your application can be challenging. While useState
is perfect for simpler state management, useReducer
provides a more powerful and flexible solution for complex state logic, akin to Redux. In this blog post, we’ll dive deep into useReducer
, exploring its syntax, use cases, and practical examples to help you master this essential React hook.
Table of Contents
Introduction to useReducer
useReducer
is a hook that helps you manage state in a more predictable and maintainable way, especially when dealing with complex state logic. It’s an alternative to useState
and works similarly to the concept of reducers in Redux.
Syntax and Basic Usage
const [state, dispatch] = useReducer(reducer, initialState);
✅ useReducer()
takes two arguments:
reducer
– A function that determines how state should change based on actions.initialState
– The initial value of the state.
✅ It returns an array with two values:
state
– The current state value.dispatch
– A function used to send actions to the reducer.
Basic Example:
Let’s start with a simple counter example to understand the basic usage of useReducer
.
import React, { useReducer } from 'react';
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
return state;
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
</div>
);
}
export default Counter;
When to Use useReducer
While useState
is sufficient for simple state management, useReducer
shines in scenarios involving:
- Complex state logic: When state transitions are complex and involve multiple sub-values.
- Centralized state updates: When multiple components need to update the same state.
- Predictable state transitions: When you want more predictable state transitions by using a pure function.
🎯 When to Use useReducer
Instead of useState
?
useState | useReducer |
---|---|
Simple state updates | Complex state logic |
Independent state variables | State transitions depend on previous state |
Fewer actions | Multiple actions affecting state |
useState
vs useReducer
Advanced useReducer Patterns
Using Multiple Reducers
You can use multiple reducers to manage different parts of your state.
const userReducer = (state, action) => {
switch (action.type) {
case 'setUser':
return { ...state, user: action.payload };
default:
return state;
}
};
const postReducer = (state, action) => {
switch (action.type) {
case 'addPost':
return { ...state, posts: [...state.posts, action.payload] };
default:
return state;
}
};
Combining Reducers
Combine multiple reducers to handle different slices of the state in a single useReducer
hook.
const combinedReducer = (state, action) => {
return {
user: userReducer(state.user, action),
posts: postReducer(state.posts, action),
};
};
const initialState = {
user: null,
posts: [],
};
function App() {
const [state, dispatch] = useReducer(combinedReducer, initialState);
return (
<div>
{/* Your components here */}
</div>
);
}
Practical Examples
Todo List
Let’s build a simple todo list application to see useReducer
in action.
const initialState = {
todos: [],
todoCount: 0,
};
function todoReducer(state, action) {
switch (action.type) {
case 'add':
return {
...state,
todos: [...state.todos, action.payload],
todoCount: state.todoCount + 1,
};
case 'remove':
return {
...state,
todos: state.todos.filter((todo, index) => index !== action.payload),
todoCount: state.todoCount - 1,
};
default:
return state;
}
}
function TodoApp() {
const [state, dispatch] = useReducer(todoReducer, initialState);
const [todo, setTodo] = useState('');
const addTodo = () => {
dispatch({ type: 'add', payload: todo });
setTodo('');
};
return (
<div>
<h2>Todo List</h2>
<input value={todo} onChange={(e) => setTodo(e.target.value)} />
<button onClick={addTodo}>Add Todo</button>
<ul>
{state.todos.map((todo, index) => (
<li key={index}>
{todo} <button onClick={() => dispatch({ type: 'remove', payload: index })}>Remove</button>
</li>
))}
</ul>
<p>Total Todos: {state.todoCount}</p>
</div>
);
}
export default TodoApp;
🚀 Benefits of useReducer
✅ Better State Management – Keeps logic centralized.
✅ Predictable State Updates – Follows a structured flow.
✅ Good for Complex State – Useful when handling multiple actions.
Conclusion
useReducer
is a powerful hook that can simplify complex state management in your React applications. By understanding its syntax, use cases, and advanced patterns, you can leverage useReducer
to create more predictable and maintainable state logic. Whether you’re managing a simple counter or a complex application, useReducer
can help you keep your state management clean and efficient.
Embrace the power of useReducer
and take your React skills to the next level!
useReducer
Interview Questions
If you’re preparing for a React interview, understanding useReducer
is essential, especially for positions that require managing complex state logic. Here are some common and insightful interview questions on useReducer
, along with explanations and example answers to help you prepare.
1. What is useReducer and how does it differ from useState?
Explanation:useReducer
is a hook used for managing complex state logic in React. While useState
is suitable for simple state updates, useReducer
is more powerful for handling state transitions that involve multiple actions or intricate state structures.
Example Answer:useReducer
is a hook that allows us to manage complex state logic by using a reducer function. It differs from useState
in that it provides a more predictable and centralized way to handle state transitions. useState
is great for simple state management, while useReducer
is better suited for scenarios where the state changes are dependent on multiple actions and have complex logic.
2. Explain the syntax of useReducer and its parameters.
Explanation:
The useReducer
hook takes two arguments: a reducer function and an initial state. It returns the current state and a dispatch function.
Example Answer:
The useReducer
hook has the following syntax:
const [state, dispatch] = useReducer(reducer, initialState);
reducer
: A function that takes the current state and an action, and returns the new state.initialState
: The initial value of the state.state
: The current state.dispatch
: A function used to send actions to the reducer.
3. When would you use useReducer over useState?
Explanation:useReducer
is preferable over useState
when dealing with more complex state logic, such as when the state depends on previous states or when there are multiple sub-values in the state.
Example Answer:
I would use useReducer
over useState
when the state logic is complex and involves multiple actions or sub-values. For example, if I have a form with multiple fields and complex validation logic, useReducer
would help manage the state transitions more predictably. Additionally, if multiple components need to dispatch actions to update the same state, useReducer
provides a centralized way to handle it.
4. Can you provide an example of a simple useReducer implementation?
Explanation:
A basic example to illustrate the use of useReducer
in a simple counter component.
Example Answer:
import React, { useReducer } from 'react';
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
return state;
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
</div>
);
}
export default Counter;
5. How do you handle side effects with useReducer?
Explanation:
Side effects (e.g., API calls) are typically handled using useEffect
in conjunction with useReducer
.
Example Answer:
To handle side effects with useReducer
, I would use the useEffect
hook. For example, if I need to fetch data when a component mounts or when certain actions are dispatched, I can use useEffect
to trigger those side effects and then use the dispatch function to update the state based on the results.
import React, { useReducer, useEffect } from 'react';
const initialState = { data: null, loading: true, error: null };
function reducer(state, action) {
switch (action.type) {
case 'fetchSuccess':
return { data: action.payload, loading: false, error: null };
case 'fetchError':
return { data: null, loading: false, error: action.payload };
default:
return state;
}
}
function DataFetchingComponent() {
const [state, dispatch] = useReducer(reducer, initialState);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => dispatch({ type: 'fetchSuccess', payload: data }))
.catch(error => dispatch({ type: 'fetchError', payload: error }));
}, []);
if (state.loading) return <p>Loading...</p>;
if (state.error) return <p>Error: {state.error.message}</p>;
return <div>Data: {JSON.stringify(state.data)}</div>;
}
export default DataFetchingComponent;
6. How can you optimize performance when using useReducer?
Explanation:
Understanding performance optimization techniques is crucial for efficient React applications.
Example Answer:
To optimize performance when using useReducer
, I can:
- Use
useMemo
to memoize expensive computations within the reducer. - Use
React.memo
to prevent unnecessary re-renders of child components. - Ensure the reducer function is pure and avoids side effects.
- Split complex reducers into smaller ones if possible.
import React, { useReducer, useMemo } from 'react';
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
return state;
}
}
const Counter = React.memo(({ count, dispatch }) => (
<div>
<p>Count: {count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
</div>
));
function App() {
const [state, dispatch] = useReducer(reducer, initialState);
const memoizedValue = useMemo(() => state.count, [state.count]);
return <Counter count={memoizedValue} dispatch={dispatch} />;
}
export default App;
Preparing for interview questions on useReducer
requires a solid understanding of its syntax, use cases, and practical applications. By mastering these questions, you’ll be well-equipped to demonstrate your proficiency in managing complex state logic in React during your next interview. Happy coding!
Read other awesome articles in Medium.com or in akcoding’s posts.
OR
Join us on YouTube Channel
OR Scan the QR Code to Directly open the Channel 👉
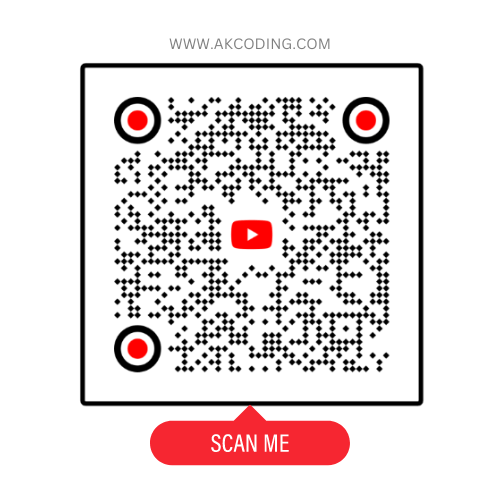